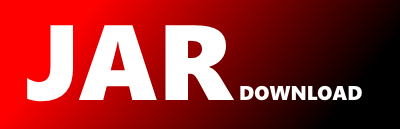
software.amazon.awssdk.services.sagemakerruntime.model.ResponseStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sagemakerruntime Show documentation
Show all versions of sagemakerruntime Show documentation
The AWS Java SDK for SageMaker Runtime module holds the client classes that are used for
communicating with SageMaker Runtime.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sagemakerruntime.model;
import java.util.Collections;
import java.util.EnumSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.services.sagemakerruntime.model.responsestream.DefaultPayloadPart;
import software.amazon.awssdk.utils.internal.EnumUtils;
/**
* Base interface for all event types in ResponseStream.
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
public interface ResponseStream extends SdkPojo {
/**
* Special type of {@link ResponseStream} for unknown types of events that this version of the SDK does not know
* about
*/
ResponseStream UNKNOWN = new ResponseStream() {
@Override
public List> sdkFields() {
return Collections.emptyList();
}
@Override
public void accept(InvokeEndpointWithResponseStreamResponseHandler.Visitor visitor) {
visitor.visitDefault(this);
}
};
/**
* Create a builder for the {@code PayloadPart} event type for this stream.
*/
static PayloadPart.Builder payloadPartBuilder() {
return DefaultPayloadPart.builder();
}
/**
* The type of this event. Corresponds to the {@code :event-type} header on the Message.
*/
default EventType sdkEventType() {
return EventType.UNKNOWN_TO_SDK_VERSION;
}
/**
* Calls the appropriate visit method depending on the subtype of {@link ResponseStream}.
*
* @param visitor
* Visitor to invoke.
*/
void accept(InvokeEndpointWithResponseStreamResponseHandler.Visitor visitor);
/**
* The known possible types of events for {@code ResponseStream}.
*/
@Generated("software.amazon.awssdk:codegen")
enum EventType {
PAYLOAD_PART("PayloadPart"),
UNKNOWN_TO_SDK_VERSION(null);
private static final Map VALUE_MAP = EnumUtils.uniqueIndex(EventType.class, EventType::toString);
private final String value;
private EventType(String value) {
this.value = value;
}
@Override
public String toString() {
return String.valueOf(value);
}
/**
* Use this in place of valueOf to convert the raw string returned by the service into the enum value.
*
* @param value
* real value
* @return EventType corresponding to the value
*/
public static EventType fromValue(String value) {
if (value == null) {
return null;
}
return VALUE_MAP.getOrDefault(value, UNKNOWN_TO_SDK_VERSION);
}
/**
* Use this in place of {@link #values()} to return a {@link Set} of all values known to the SDK. This will
* return all known enum values except {@link #UNKNOWN_TO_SDK_VERSION}.
*
* @return a {@link Set} of known {@link EventType}s
*/
public static Set knownValues() {
Set knownValues = EnumSet.allOf(EventType.class);
knownValues.remove(UNKNOWN_TO_SDK_VERSION);
return knownValues;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy