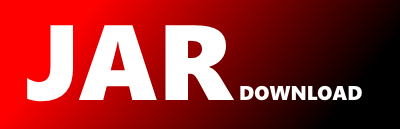
software.amazon.awssdk.core.SdkField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk-core Show documentation
Show all versions of sdk-core Show documentation
The AWS SDK for Java - SDK Core runtime module holds the classes that are used by the individual service
clients to interact with
Amazon Web Services. Users need to depend on aws-java-sdk artifact for accessing individual client classes.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package software.amazon.awssdk.core;
import java.util.Arrays;
import java.util.EnumMap;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import java.util.function.Supplier;
import software.amazon.awssdk.annotations.SdkProtectedApi;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DataTypeConversionFailureHandlingTrait;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.Trait;
import software.amazon.awssdk.core.traits.TraitType;
/**
* Metadata about a member in an {@link SdkPojo}. Contains information about how to marshall/unmarshall.
*
* @param Java Type of member.
*/
@SdkProtectedApi
public final class SdkField {
private final String memberName;
private final MarshallingType super TypeT> marshallingType;
private final MarshallLocation location;
private final String locationName;
private final String unmarshallLocationName;
private final Supplier constructor;
private final BiConsumer
© 2015 - 2024 Weber Informatics LLC | Privacy Policy