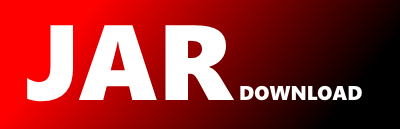
software.amazon.awssdk.services.secretsmanager.model.GetSecretValueResponse Maven / Gradle / Ivy
Show all versions of secretsmanager Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.secretsmanager.model;
import java.nio.ByteBuffer;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkBytes;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetSecretValueResponse extends SecretsManagerResponse implements
ToCopyableBuilder {
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("ARN")
.getter(getter(GetSecretValueResponse::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ARN").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(GetSecretValueResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField VERSION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VersionId").getter(getter(GetSecretValueResponse::versionId)).setter(setter(Builder::versionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionId").build()).build();
private static final SdkField SECRET_BINARY_FIELD = SdkField. builder(MarshallingType.SDK_BYTES)
.memberName("SecretBinary").getter(getter(GetSecretValueResponse::secretBinary))
.setter(setter(Builder::secretBinary))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretBinary").build()).build();
private static final SdkField SECRET_STRING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SecretString").getter(getter(GetSecretValueResponse::secretString))
.setter(setter(Builder::secretString))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecretString").build()).build();
private static final SdkField> VERSION_STAGES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("VersionStages")
.getter(getter(GetSecretValueResponse::versionStages))
.setter(setter(Builder::versionStages))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VersionStages").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CREATED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreatedDate").getter(getter(GetSecretValueResponse::createdDate)).setter(setter(Builder::createdDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedDate").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ARN_FIELD, NAME_FIELD,
VERSION_ID_FIELD, SECRET_BINARY_FIELD, SECRET_STRING_FIELD, VERSION_STAGES_FIELD, CREATED_DATE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("ARN", ARN_FIELD);
put("Name", NAME_FIELD);
put("VersionId", VERSION_ID_FIELD);
put("SecretBinary", SECRET_BINARY_FIELD);
put("SecretString", SECRET_STRING_FIELD);
put("VersionStages", VERSION_STAGES_FIELD);
put("CreatedDate", CREATED_DATE_FIELD);
}
});
private final String arn;
private final String name;
private final String versionId;
private final SdkBytes secretBinary;
private final String secretString;
private final List versionStages;
private final Instant createdDate;
private GetSecretValueResponse(BuilderImpl builder) {
super(builder);
this.arn = builder.arn;
this.name = builder.name;
this.versionId = builder.versionId;
this.secretBinary = builder.secretBinary;
this.secretString = builder.secretString;
this.versionStages = builder.versionStages;
this.createdDate = builder.createdDate;
}
/**
*
* The ARN of the secret.
*
*
* @return The ARN of the secret.
*/
public final String arn() {
return arn;
}
/**
*
* The friendly name of the secret.
*
*
* @return The friendly name of the secret.
*/
public final String name() {
return name;
}
/**
*
* The unique identifier of this version of the secret.
*
*
* @return The unique identifier of this version of the secret.
*/
public final String versionId() {
return versionId;
}
/**
*
* The decrypted secret value, if the secret value was originally provided as binary data in the form of a byte
* array. When you retrieve a SecretBinary
using the HTTP API, the Python SDK, or the Amazon Web
* Services CLI, the value is Base64-encoded. Otherwise, it is not encoded.
*
*
* If the secret was created by using the Secrets Manager console, or if the secret value was originally provided as
* a string, then this field is omitted. The secret value appears in SecretString
instead.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @return The decrypted secret value, if the secret value was originally provided as binary data in the form of a
* byte array. When you retrieve a SecretBinary
using the HTTP API, the Python SDK, or the
* Amazon Web Services CLI, the value is Base64-encoded. Otherwise, it is not encoded.
*
* If the secret was created by using the Secrets Manager console, or if the secret value was originally
* provided as a string, then this field is omitted. The secret value appears in SecretString
* instead.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail
* log entries. If you create your own log entries, you must also avoid logging the information in this
* field.
*/
public final SdkBytes secretBinary() {
return secretBinary;
}
/**
*
* The decrypted secret value, if the secret value was originally provided as a string or through the Secrets
* Manager console.
*
*
* If this secret was created by using the console, then Secrets Manager stores the information as a JSON structure
* of key/value pairs.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @return The decrypted secret value, if the secret value was originally provided as a string or through the
* Secrets Manager console.
*
* If this secret was created by using the console, then Secrets Manager stores the information as a JSON
* structure of key/value pairs.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail
* log entries. If you create your own log entries, you must also avoid logging the information in this
* field.
*/
public final String secretString() {
return secretString;
}
/**
* For responses, this returns true if the service returned a value for the VersionStages property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVersionStages() {
return versionStages != null && !(versionStages instanceof SdkAutoConstructList);
}
/**
*
* A list of all of the staging labels currently attached to this version of the secret.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVersionStages} method.
*
*
* @return A list of all of the staging labels currently attached to this version of the secret.
*/
public final List versionStages() {
return versionStages;
}
/**
*
* The date and time that this version of the secret was created. If you don't specify which version in
* VersionId
or VersionStage
, then Secrets Manager uses the AWSCURRENT
* version.
*
*
* @return The date and time that this version of the secret was created. If you don't specify which version in
* VersionId
or VersionStage
, then Secrets Manager uses the
* AWSCURRENT
version.
*/
public final Instant createdDate() {
return createdDate;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(versionId());
hashCode = 31 * hashCode + Objects.hashCode(secretBinary());
hashCode = 31 * hashCode + Objects.hashCode(secretString());
hashCode = 31 * hashCode + Objects.hashCode(hasVersionStages() ? versionStages() : null);
hashCode = 31 * hashCode + Objects.hashCode(createdDate());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetSecretValueResponse)) {
return false;
}
GetSecretValueResponse other = (GetSecretValueResponse) obj;
return Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(versionId(), other.versionId()) && Objects.equals(secretBinary(), other.secretBinary())
&& Objects.equals(secretString(), other.secretString()) && hasVersionStages() == other.hasVersionStages()
&& Objects.equals(versionStages(), other.versionStages()) && Objects.equals(createdDate(), other.createdDate());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetSecretValueResponse").add("ARN", arn()).add("Name", name()).add("VersionId", versionId())
.add("SecretBinary", secretBinary() == null ? null : "*** Sensitive Data Redacted ***")
.add("SecretString", secretString() == null ? null : "*** Sensitive Data Redacted ***")
.add("VersionStages", hasVersionStages() ? versionStages() : null).add("CreatedDate", createdDate()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ARN":
return Optional.ofNullable(clazz.cast(arn()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "VersionId":
return Optional.ofNullable(clazz.cast(versionId()));
case "SecretBinary":
return Optional.ofNullable(clazz.cast(secretBinary()));
case "SecretString":
return Optional.ofNullable(clazz.cast(secretString()));
case "VersionStages":
return Optional.ofNullable(clazz.cast(versionStages()));
case "CreatedDate":
return Optional.ofNullable(clazz.cast(createdDate()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function