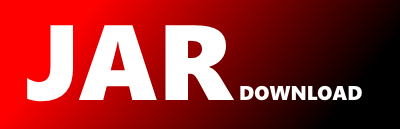
software.amazon.awssdk.services.serverlessapplicationrepository.DefaultServerlessApplicationRepositoryAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessrepo Show documentation
Show all versions of serverlessrepo Show documentation
The AWS Java SDK for AWSServerlessApplicationRepository module holds the client classes that are used for communicating
with AWSServerlessApplicationRepository Service
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.serverlessapplicationrepository;
import java.util.concurrent.CompletableFuture;
import javax.annotation.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.config.AwsAsyncClientConfiguration;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocol;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolMetadata;
import software.amazon.awssdk.core.client.AsyncClientHandler;
import software.amazon.awssdk.core.client.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.protocol.json.JsonClientMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorResponseMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorShapeMetadata;
import software.amazon.awssdk.core.protocol.json.JsonOperationMetadata;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.BadRequestException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ConflictException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationVersionRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationVersionResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateCloudFormationChangeSetRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateCloudFormationChangeSetResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ForbiddenException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationPolicyRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationPolicyResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.InternalServerErrorException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationVersionsRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationVersionsResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationsRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationsResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.NotFoundException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.PutApplicationPolicyRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.PutApplicationPolicyResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.TooManyRequestsException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateApplicationRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateApplicationResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateApplicationVersionRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateApplicationVersionResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateCloudFormationChangeSetRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateCloudFormationChangeSetResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.GetApplicationPolicyRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.GetApplicationPolicyResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.GetApplicationRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.GetApplicationResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.ListApplicationVersionsRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.ListApplicationVersionsResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.ListApplicationsRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.ListApplicationsResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.PutApplicationPolicyRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.PutApplicationPolicyResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.UpdateApplicationRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.UpdateApplicationResponseUnmarshaller;
/**
* Internal implementation of {@link ServerlessApplicationRepositoryAsyncClient}.
*
* @see ServerlessApplicationRepositoryAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultServerlessApplicationRepositoryAsyncClient implements ServerlessApplicationRepositoryAsyncClient {
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
protected DefaultServerlessApplicationRepositoryAsyncClient(AwsAsyncClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration, null);
this.protocolFactory = init();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
* Creates an application, optionally including an AWS SAM file to create the first application version in the same
* call.
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ConflictException 409 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.CreateApplication
* @see AWS API Documentation
*/
@Override
public CompletableFuture createApplication(CreateApplicationRequest createApplicationRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateApplicationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new CreateApplicationRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createApplicationRequest));
}
/**
* Creates an application version.
*
* @param createApplicationVersionRequest
* @return A Java Future containing the result of the CreateApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ConflictException 409 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.CreateApplicationVersion
* @see AWS API Documentation
*/
@Override
public CompletableFuture createApplicationVersion(
CreateApplicationVersionRequest createApplicationVersionRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateApplicationVersionResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withMarshaller(new CreateApplicationVersionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createApplicationVersionRequest));
}
/**
* Creates an AWS CloudFormation ChangeSet for the given application.
*
* @param createCloudFormationChangeSetRequest
* Create application ChangeSet request
* @return A Java Future containing the result of the CreateCloudFormationChangeSet operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.CreateCloudFormationChangeSet
* @see AWS API Documentation
*/
@Override
public CompletableFuture createCloudFormationChangeSet(
CreateCloudFormationChangeSetRequest createCloudFormationChangeSetRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateCloudFormationChangeSetResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withMarshaller(new CreateCloudFormationChangeSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createCloudFormationChangeSetRequest));
}
/**
* Gets the specified application.
*
* @param getApplicationRequest
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.GetApplication
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getApplication(GetApplicationRequest getApplicationRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetApplicationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new GetApplicationRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getApplicationRequest));
}
/**
* Gets the policy for the specified application.
*
* @param getApplicationPolicyRequest
* @return A Java Future containing the result of the GetApplicationPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.GetApplicationPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture getApplicationPolicy(
GetApplicationPolicyRequest getApplicationPolicyRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetApplicationPolicyResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new GetApplicationPolicyRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getApplicationPolicyRequest));
}
/**
* Lists versions for the specified application.
*
* @param listApplicationVersionsRequest
* @return A Java Future containing the result of the ListApplicationVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.ListApplicationVersions
* @see AWS API Documentation
*/
@Override
public CompletableFuture listApplicationVersions(
ListApplicationVersionsRequest listApplicationVersionsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListApplicationVersionsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new ListApplicationVersionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listApplicationVersionsRequest));
}
/**
* Lists applications owned by the requester.
*
* @param listApplicationsRequest
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.ListApplications
* @see AWS API Documentation
*/
@Override
public CompletableFuture listApplications(ListApplicationsRequest listApplicationsRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListApplicationsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new ListApplicationsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listApplicationsRequest));
}
/**
* Puts the policy for the specified application.
*
* @param putApplicationPolicyRequest
* Put policy request
* @return A Java Future containing the result of the PutApplicationPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.PutApplicationPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture putApplicationPolicy(
PutApplicationPolicyRequest putApplicationPolicyRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutApplicationPolicyResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new PutApplicationPolicyRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putApplicationPolicyRequest));
}
/**
* Updates the specified application.
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - ConflictException 409 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.UpdateApplication
* @see AWS API Documentation
*/
@Override
public CompletableFuture updateApplication(UpdateApplicationRequest updateApplicationRequest) {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateApplicationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new UpdateApplicationRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateApplicationRequest));
}
@Override
public void close() {
clientHandler.close();
}
private software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory init() {
return new AwsJsonProtocolFactory(
new JsonClientMetadata()
.withSupportsCbor(false)
.withSupportsIon(false)
.withBaseServiceExceptionClass(
software.amazon.awssdk.services.serverlessapplicationrepository.model.ServerlessApplicationRepositoryException.class)
.withContentTypeOverride("")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withModeledClass(
ConflictException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withModeledClass(
NotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ForbiddenException").withModeledClass(
ForbiddenException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyRequestsException").withModeledClass(
TooManyRequestsException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withModeledClass(
BadRequestException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerErrorException").withModeledClass(
InternalServerErrorException.class)), AwsJsonProtocolMetadata.builder()
.protocolVersion("1.1").protocol(AwsJsonProtocol.REST_JSON).build());
}
private HttpResponseHandler createErrorResponseHandler() {
return protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy