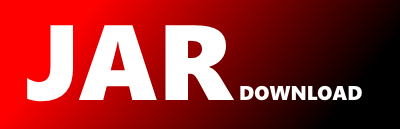
software.amazon.awssdk.services.serverlessapplicationrepository.DefaultServerlessApplicationRepositoryClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessrepo Show documentation
Show all versions of serverlessrepo Show documentation
The AWS Java SDK for AWSServerlessApplicationRepository module holds the client classes that are used for communicating
with AWSServerlessApplicationRepository Service
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.serverlessapplicationrepository;
import javax.annotation.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.config.AwsSyncClientConfiguration;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocol;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolMetadata;
import software.amazon.awssdk.core.client.ClientExecutionParams;
import software.amazon.awssdk.core.client.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.protocol.json.JsonClientMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorResponseMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorShapeMetadata;
import software.amazon.awssdk.core.protocol.json.JsonOperationMetadata;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.BadRequestException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ConflictException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationVersionRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationVersionResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateCloudFormationChangeSetRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateCloudFormationChangeSetResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ForbiddenException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationPolicyRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationPolicyResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.InternalServerErrorException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationVersionsRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationVersionsResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationsRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationsResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.NotFoundException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.PutApplicationPolicyRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.PutApplicationPolicyResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ServerlessApplicationRepositoryException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.TooManyRequestsException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.UpdateApplicationResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateApplicationRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateApplicationResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateApplicationVersionRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateApplicationVersionResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateCloudFormationChangeSetRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.CreateCloudFormationChangeSetResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.GetApplicationPolicyRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.GetApplicationPolicyResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.GetApplicationRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.GetApplicationResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.ListApplicationVersionsRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.ListApplicationVersionsResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.ListApplicationsRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.ListApplicationsResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.PutApplicationPolicyRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.PutApplicationPolicyResponseUnmarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.UpdateApplicationRequestMarshaller;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.UpdateApplicationResponseUnmarshaller;
/**
* Internal implementation of {@link ServerlessApplicationRepositoryClient}.
*
* @see ServerlessApplicationRepositoryClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultServerlessApplicationRepositoryClient implements ServerlessApplicationRepositoryClient {
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final AwsSyncClientConfiguration clientConfiguration;
protected DefaultServerlessApplicationRepositoryClient(AwsSyncClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration, null);
this.protocolFactory = init();
this.clientConfiguration = clientConfiguration;
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
* Creates an application, optionally including an AWS SAM file to create the first application version in the same
* call.
*
* @param createApplicationRequest
* @return Result of the CreateApplication operation returned by the service.
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ConflictException
* 409 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.CreateApplication
* @see AWS API Documentation
*/
@Override
public CreateApplicationResponse createApplication(CreateApplicationRequest createApplicationRequest)
throws TooManyRequestsException, BadRequestException, InternalServerErrorException, ConflictException,
ForbiddenException, AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateApplicationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createApplicationRequest).withMarshaller(new CreateApplicationRequestMarshaller(protocolFactory)));
}
/**
* Creates an application version.
*
* @param createApplicationVersionRequest
* @return Result of the CreateApplicationVersion operation returned by the service.
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ConflictException
* 409 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.CreateApplicationVersion
* @see AWS API Documentation
*/
@Override
public CreateApplicationVersionResponse createApplicationVersion(
CreateApplicationVersionRequest createApplicationVersionRequest) throws TooManyRequestsException,
BadRequestException, InternalServerErrorException, ConflictException, ForbiddenException, AwsServiceException,
SdkClientException, ServerlessApplicationRepositoryException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateApplicationVersionResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createApplicationVersionRequest)
.withMarshaller(new CreateApplicationVersionRequestMarshaller(protocolFactory)));
}
/**
* Creates an AWS CloudFormation ChangeSet for the given application.
*
* @param createCloudFormationChangeSetRequest
* Create application ChangeSet request
* @return Result of the CreateCloudFormationChangeSet operation returned by the service.
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.CreateCloudFormationChangeSet
* @see AWS API Documentation
*/
@Override
public CreateCloudFormationChangeSetResponse createCloudFormationChangeSet(
CreateCloudFormationChangeSetRequest createCloudFormationChangeSetRequest) throws TooManyRequestsException,
BadRequestException, InternalServerErrorException, ForbiddenException, AwsServiceException, SdkClientException,
ServerlessApplicationRepositoryException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateCloudFormationChangeSetResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createCloudFormationChangeSetRequest)
.withMarshaller(new CreateCloudFormationChangeSetRequestMarshaller(protocolFactory)));
}
/**
* Gets the specified application.
*
* @param getApplicationRequest
* @return Result of the GetApplication operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.GetApplication
* @see AWS
* API Documentation
*/
@Override
public GetApplicationResponse getApplication(GetApplicationRequest getApplicationRequest) throws NotFoundException,
TooManyRequestsException, BadRequestException, InternalServerErrorException, ForbiddenException, AwsServiceException,
SdkClientException, ServerlessApplicationRepositoryException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetApplicationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getApplicationRequest).withMarshaller(new GetApplicationRequestMarshaller(protocolFactory)));
}
/**
* Gets the policy for the specified application.
*
* @param getApplicationPolicyRequest
* @return Result of the GetApplicationPolicy operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.GetApplicationPolicy
* @see AWS API Documentation
*/
@Override
public GetApplicationPolicyResponse getApplicationPolicy(GetApplicationPolicyRequest getApplicationPolicyRequest)
throws NotFoundException, TooManyRequestsException, BadRequestException, InternalServerErrorException,
ForbiddenException, AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetApplicationPolicyResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getApplicationPolicyRequest)
.withMarshaller(new GetApplicationPolicyRequestMarshaller(protocolFactory)));
}
/**
* Lists versions for the specified application.
*
* @param listApplicationVersionsRequest
* @return Result of the ListApplicationVersions operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.ListApplicationVersions
* @see AWS API Documentation
*/
@Override
public ListApplicationVersionsResponse listApplicationVersions(ListApplicationVersionsRequest listApplicationVersionsRequest)
throws NotFoundException, TooManyRequestsException, BadRequestException, InternalServerErrorException,
ForbiddenException, AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListApplicationVersionsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listApplicationVersionsRequest)
.withMarshaller(new ListApplicationVersionsRequestMarshaller(protocolFactory)));
}
/**
* Lists applications owned by the requester.
*
* @param listApplicationsRequest
* @return Result of the ListApplications operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.ListApplications
* @see AWS API Documentation
*/
@Override
public ListApplicationsResponse listApplications(ListApplicationsRequest listApplicationsRequest) throws NotFoundException,
BadRequestException, InternalServerErrorException, ForbiddenException, AwsServiceException, SdkClientException,
ServerlessApplicationRepositoryException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListApplicationsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listApplicationsRequest).withMarshaller(new ListApplicationsRequestMarshaller(protocolFactory)));
}
/**
* Puts the policy for the specified application.
*
* @param putApplicationPolicyRequest
* Put policy request
* @return Result of the PutApplicationPolicy operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.PutApplicationPolicy
* @see AWS API Documentation
*/
@Override
public PutApplicationPolicyResponse putApplicationPolicy(PutApplicationPolicyRequest putApplicationPolicyRequest)
throws NotFoundException, TooManyRequestsException, BadRequestException, InternalServerErrorException,
ForbiddenException, AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutApplicationPolicyResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(putApplicationPolicyRequest)
.withMarshaller(new PutApplicationPolicyRequestMarshaller(protocolFactory)));
}
/**
* Updates the specified application.
*
* @param updateApplicationRequest
* @return Result of the UpdateApplication operation returned by the service.
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws ConflictException
* 409 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.UpdateApplication
* @see AWS API Documentation
*/
@Override
public UpdateApplicationResponse updateApplication(UpdateApplicationRequest updateApplicationRequest)
throws BadRequestException, InternalServerErrorException, ForbiddenException, NotFoundException,
TooManyRequestsException, ConflictException, AwsServiceException, SdkClientException,
ServerlessApplicationRepositoryException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateApplicationResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(updateApplicationRequest).withMarshaller(new UpdateApplicationRequestMarshaller(protocolFactory)));
}
private HttpResponseHandler createErrorResponseHandler() {
return protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
}
private software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory init() {
return new AwsJsonProtocolFactory(
new JsonClientMetadata()
.withSupportsCbor(false)
.withSupportsIon(false)
.withBaseServiceExceptionClass(
software.amazon.awssdk.services.serverlessapplicationrepository.model.ServerlessApplicationRepositoryException.class)
.withContentTypeOverride("")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withModeledClass(
ConflictException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withModeledClass(
NotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ForbiddenException").withModeledClass(
ForbiddenException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyRequestsException").withModeledClass(
TooManyRequestsException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withModeledClass(
BadRequestException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerErrorException").withModeledClass(
InternalServerErrorException.class)), AwsJsonProtocolMetadata.builder()
.protocolVersion("1.1").protocol(AwsJsonProtocol.REST_JSON).build());
}
@Override
public void close() {
clientHandler.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy