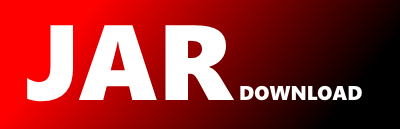
software.amazon.awssdk.services.serverlessapplicationrepository.ServerlessApplicationRepositoryAsyncClient Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.serverlessapplicationrepository;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import javax.annotation.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationVersionRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationVersionResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateCloudFormationChangeSetRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateCloudFormationChangeSetResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationPolicyRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationPolicyResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationVersionsRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationVersionsResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationsRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationsResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.PutApplicationPolicyRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.PutApplicationPolicyResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.UpdateApplicationResponse;
import software.amazon.awssdk.utils.SdkAutoCloseable;
/**
* Service client for accessing null asynchronously. This can be created using the static {@link #builder()} method.
*
* AWS Serverless Repository
*/
@Generated("software.amazon.awssdk:codegen")
public interface ServerlessApplicationRepositoryAsyncClient extends SdkClient, SdkAutoCloseable {
String SERVICE_NAME = "serverlessrepo";
/**
* Create a {@link ServerlessApplicationRepositoryAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ServerlessApplicationRepositoryAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ServerlessApplicationRepositoryAsyncClient}.
*/
static ServerlessApplicationRepositoryAsyncClientBuilder builder() {
return new DefaultServerlessApplicationRepositoryAsyncClientBuilder();
}
/**
* Creates an application, optionally including an AWS SAM file to create the first application version in the same
* call.
*
* @param createApplicationRequest
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ConflictException 409 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.CreateApplication
* @see AWS API Documentation
*/
default CompletableFuture createApplication(CreateApplicationRequest createApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
* Creates an application, optionally including an AWS SAM file to create the first application version in the same
* call.
*
* This is a convenience which creates an instance of the {@link CreateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link CreateApplicationRequest#builder()}
*
*
* @param createApplicationRequest
* A {@link Consumer} that will call methods on {@link CreateApplicationRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ConflictException 409 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.CreateApplication
* @see AWS API Documentation
*/
default CompletableFuture createApplication(
Consumer createApplicationRequest) {
return createApplication(CreateApplicationRequest.builder().apply(createApplicationRequest).build());
}
/**
* Creates an application version.
*
* @param createApplicationVersionRequest
* @return A Java Future containing the result of the CreateApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ConflictException 409 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.CreateApplicationVersion
* @see AWS API Documentation
*/
default CompletableFuture createApplicationVersion(
CreateApplicationVersionRequest createApplicationVersionRequest) {
throw new UnsupportedOperationException();
}
/**
* Creates an application version.
*
* This is a convenience which creates an instance of the {@link CreateApplicationVersionRequest.Builder} avoiding
* the need to create one manually via {@link CreateApplicationVersionRequest#builder()}
*
*
* @param createApplicationVersionRequest
* A {@link Consumer} that will call methods on {@link CreateApplicationVersionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateApplicationVersion operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ConflictException 409 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.CreateApplicationVersion
* @see AWS API Documentation
*/
default CompletableFuture createApplicationVersion(
Consumer createApplicationVersionRequest) {
return createApplicationVersion(CreateApplicationVersionRequest.builder().apply(createApplicationVersionRequest).build());
}
/**
* Creates an AWS CloudFormation ChangeSet for the given application.
*
* @param createCloudFormationChangeSetRequest
* Create application ChangeSet request
* @return A Java Future containing the result of the CreateCloudFormationChangeSet operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.CreateCloudFormationChangeSet
* @see AWS API Documentation
*/
default CompletableFuture createCloudFormationChangeSet(
CreateCloudFormationChangeSetRequest createCloudFormationChangeSetRequest) {
throw new UnsupportedOperationException();
}
/**
* Creates an AWS CloudFormation ChangeSet for the given application.
*
* This is a convenience which creates an instance of the {@link CreateCloudFormationChangeSetRequest.Builder}
* avoiding the need to create one manually via {@link CreateCloudFormationChangeSetRequest#builder()}
*
*
* @param createCloudFormationChangeSetRequest
* A {@link Consumer} that will call methods on {@link CreateCloudFormationChangeSetRequest.Builder} to
* create a request. Create application ChangeSet request
* @return A Java Future containing the result of the CreateCloudFormationChangeSet operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.CreateCloudFormationChangeSet
* @see AWS API Documentation
*/
default CompletableFuture createCloudFormationChangeSet(
Consumer createCloudFormationChangeSetRequest) {
return createCloudFormationChangeSet(CreateCloudFormationChangeSetRequest.builder()
.apply(createCloudFormationChangeSetRequest).build());
}
/**
* Gets the specified application.
*
* @param getApplicationRequest
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.GetApplication
* @see AWS
* API Documentation
*/
default CompletableFuture getApplication(GetApplicationRequest getApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
* Gets the specified application.
*
* This is a convenience which creates an instance of the {@link GetApplicationRequest.Builder} avoiding the need to
* create one manually via {@link GetApplicationRequest#builder()}
*
*
* @param getApplicationRequest
* A {@link Consumer} that will call methods on {@link GetApplicationRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.GetApplication
* @see AWS
* API Documentation
*/
default CompletableFuture getApplication(Consumer getApplicationRequest) {
return getApplication(GetApplicationRequest.builder().apply(getApplicationRequest).build());
}
/**
* Gets the policy for the specified application.
*
* @param getApplicationPolicyRequest
* @return A Java Future containing the result of the GetApplicationPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.GetApplicationPolicy
* @see AWS API Documentation
*/
default CompletableFuture getApplicationPolicy(
GetApplicationPolicyRequest getApplicationPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
* Gets the policy for the specified application.
*
* This is a convenience which creates an instance of the {@link GetApplicationPolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetApplicationPolicyRequest#builder()}
*
*
* @param getApplicationPolicyRequest
* A {@link Consumer} that will call methods on {@link GetApplicationPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetApplicationPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.GetApplicationPolicy
* @see AWS API Documentation
*/
default CompletableFuture getApplicationPolicy(
Consumer getApplicationPolicyRequest) {
return getApplicationPolicy(GetApplicationPolicyRequest.builder().apply(getApplicationPolicyRequest).build());
}
/**
* Lists versions for the specified application.
*
* @param listApplicationVersionsRequest
* @return A Java Future containing the result of the ListApplicationVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.ListApplicationVersions
* @see AWS API Documentation
*/
default CompletableFuture listApplicationVersions(
ListApplicationVersionsRequest listApplicationVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
* Lists versions for the specified application.
*
* This is a convenience which creates an instance of the {@link ListApplicationVersionsRequest.Builder} avoiding
* the need to create one manually via {@link ListApplicationVersionsRequest#builder()}
*
*
* @param listApplicationVersionsRequest
* A {@link Consumer} that will call methods on {@link ListApplicationVersionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListApplicationVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.ListApplicationVersions
* @see AWS API Documentation
*/
default CompletableFuture listApplicationVersions(
Consumer listApplicationVersionsRequest) {
return listApplicationVersions(ListApplicationVersionsRequest.builder().apply(listApplicationVersionsRequest).build());
}
/**
* Lists applications owned by the requester.
*
* @param listApplicationsRequest
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.ListApplications
* @see AWS API Documentation
*/
default CompletableFuture listApplications(ListApplicationsRequest listApplicationsRequest) {
throw new UnsupportedOperationException();
}
/**
* Lists applications owned by the requester.
*
* This is a convenience which creates an instance of the {@link ListApplicationsRequest.Builder} avoiding the need
* to create one manually via {@link ListApplicationsRequest#builder()}
*
*
* @param listApplicationsRequest
* A {@link Consumer} that will call methods on {@link ListApplicationsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.ListApplications
* @see AWS API Documentation
*/
default CompletableFuture listApplications(
Consumer listApplicationsRequest) {
return listApplications(ListApplicationsRequest.builder().apply(listApplicationsRequest).build());
}
/**
* Lists applications owned by the requester.
*
* @return A Java Future containing the result of the ListApplications operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.ListApplications
* @see AWS API Documentation
*/
default CompletableFuture listApplications() {
return listApplications(ListApplicationsRequest.builder().build());
}
/**
* Puts the policy for the specified application.
*
* @param putApplicationPolicyRequest
* Put policy request
* @return A Java Future containing the result of the PutApplicationPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.PutApplicationPolicy
* @see AWS API Documentation
*/
default CompletableFuture putApplicationPolicy(
PutApplicationPolicyRequest putApplicationPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
* Puts the policy for the specified application.
*
* This is a convenience which creates an instance of the {@link PutApplicationPolicyRequest.Builder} avoiding the
* need to create one manually via {@link PutApplicationPolicyRequest#builder()}
*
*
* @param putApplicationPolicyRequest
* A {@link Consumer} that will call methods on {@link PutApplicationPolicyRequest.Builder} to create a
* request. Put policy request
* @return A Java Future containing the result of the PutApplicationPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.PutApplicationPolicy
* @see AWS API Documentation
*/
default CompletableFuture putApplicationPolicy(
Consumer putApplicationPolicyRequest) {
return putApplicationPolicy(PutApplicationPolicyRequest.builder().apply(putApplicationPolicyRequest).build());
}
/**
* Updates the specified application.
*
* @param updateApplicationRequest
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - ConflictException 409 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.UpdateApplication
* @see AWS API Documentation
*/
default CompletableFuture updateApplication(UpdateApplicationRequest updateApplicationRequest) {
throw new UnsupportedOperationException();
}
/**
* Updates the specified application.
*
* This is a convenience which creates an instance of the {@link UpdateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link UpdateApplicationRequest#builder()}
*
*
* @param updateApplicationRequest
* A {@link Consumer} that will call methods on {@link UpdateApplicationRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateApplication operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - BadRequestException 400 response
* - InternalServerErrorException 500 response
* - ForbiddenException 403 response
* - NotFoundException 404 response
* - TooManyRequestsException 429 response
* - ConflictException 409 response
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ServerlessApplicationRepositoryException Base class for all service exceptions. Unknown exceptions
* will be thrown as an instance of this type.
*
* @sample ServerlessApplicationRepositoryAsyncClient.UpdateApplication
* @see AWS API Documentation
*/
default CompletableFuture updateApplication(
Consumer updateApplicationRequest) {
return updateApplication(UpdateApplicationRequest.builder().apply(updateApplicationRequest).build());
}
}