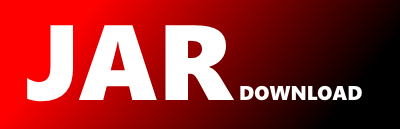
software.amazon.awssdk.services.serverlessapplicationrepository.ServerlessApplicationRepositoryClient Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.serverlessapplicationrepository;
import java.util.function.Consumer;
import javax.annotation.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.BadRequestException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ConflictException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationVersionRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationVersionResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateCloudFormationChangeSetRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateCloudFormationChangeSetResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ForbiddenException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationPolicyRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationPolicyResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.GetApplicationResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.InternalServerErrorException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationVersionsRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationVersionsResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationsRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ListApplicationsResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.NotFoundException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.PutApplicationPolicyRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.PutApplicationPolicyResponse;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.ServerlessApplicationRepositoryException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.TooManyRequestsException;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.UpdateApplicationRequest;
import software.amazon.awssdk.services.serverlessapplicationrepository.model.UpdateApplicationResponse;
import software.amazon.awssdk.utils.SdkAutoCloseable;
/**
* Service client for accessing null. This can be created using the static {@link #builder()} method.
*
* AWS Serverless Repository
*/
@Generated("software.amazon.awssdk:codegen")
public interface ServerlessApplicationRepositoryClient extends SdkClient, SdkAutoCloseable {
String SERVICE_NAME = "serverlessrepo";
/**
* Create a {@link ServerlessApplicationRepositoryClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ServerlessApplicationRepositoryClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ServerlessApplicationRepositoryClient}.
*/
static ServerlessApplicationRepositoryClientBuilder builder() {
return new DefaultServerlessApplicationRepositoryClientBuilder();
}
/**
* Creates an application, optionally including an AWS SAM file to create the first application version in the same
* call.
*
* @param createApplicationRequest
* @return Result of the CreateApplication operation returned by the service.
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ConflictException
* 409 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.CreateApplication
* @see AWS API Documentation
*/
default CreateApplicationResponse createApplication(CreateApplicationRequest createApplicationRequest)
throws TooManyRequestsException, BadRequestException, InternalServerErrorException, ConflictException,
ForbiddenException, AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
throw new UnsupportedOperationException();
}
/**
* Creates an application, optionally including an AWS SAM file to create the first application version in the same
* call.
*
* This is a convenience which creates an instance of the {@link CreateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link CreateApplicationRequest#builder()}
*
*
* @param createApplicationRequest
* A {@link Consumer} that will call methods on {@link CreateApplicationRequest.Builder} to create a request.
* @return Result of the CreateApplication operation returned by the service.
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ConflictException
* 409 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.CreateApplication
* @see AWS API Documentation
*/
default CreateApplicationResponse createApplication(Consumer createApplicationRequest)
throws TooManyRequestsException, BadRequestException, InternalServerErrorException, ConflictException,
ForbiddenException, AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
return createApplication(CreateApplicationRequest.builder().apply(createApplicationRequest).build());
}
/**
* Creates an application version.
*
* @param createApplicationVersionRequest
* @return Result of the CreateApplicationVersion operation returned by the service.
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ConflictException
* 409 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.CreateApplicationVersion
* @see AWS API Documentation
*/
default CreateApplicationVersionResponse createApplicationVersion(
CreateApplicationVersionRequest createApplicationVersionRequest) throws TooManyRequestsException,
BadRequestException, InternalServerErrorException, ConflictException, ForbiddenException, AwsServiceException,
SdkClientException, ServerlessApplicationRepositoryException {
throw new UnsupportedOperationException();
}
/**
* Creates an application version.
*
* This is a convenience which creates an instance of the {@link CreateApplicationVersionRequest.Builder} avoiding
* the need to create one manually via {@link CreateApplicationVersionRequest#builder()}
*
*
* @param createApplicationVersionRequest
* A {@link Consumer} that will call methods on {@link CreateApplicationVersionRequest.Builder} to create a
* request.
* @return Result of the CreateApplicationVersion operation returned by the service.
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ConflictException
* 409 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.CreateApplicationVersion
* @see AWS API Documentation
*/
default CreateApplicationVersionResponse createApplicationVersion(
Consumer createApplicationVersionRequest) throws TooManyRequestsException,
BadRequestException, InternalServerErrorException, ConflictException, ForbiddenException, AwsServiceException,
SdkClientException, ServerlessApplicationRepositoryException {
return createApplicationVersion(CreateApplicationVersionRequest.builder().apply(createApplicationVersionRequest).build());
}
/**
* Creates an AWS CloudFormation ChangeSet for the given application.
*
* @param createCloudFormationChangeSetRequest
* Create application ChangeSet request
* @return Result of the CreateCloudFormationChangeSet operation returned by the service.
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.CreateCloudFormationChangeSet
* @see AWS API Documentation
*/
default CreateCloudFormationChangeSetResponse createCloudFormationChangeSet(
CreateCloudFormationChangeSetRequest createCloudFormationChangeSetRequest) throws TooManyRequestsException,
BadRequestException, InternalServerErrorException, ForbiddenException, AwsServiceException, SdkClientException,
ServerlessApplicationRepositoryException {
throw new UnsupportedOperationException();
}
/**
* Creates an AWS CloudFormation ChangeSet for the given application.
*
* This is a convenience which creates an instance of the {@link CreateCloudFormationChangeSetRequest.Builder}
* avoiding the need to create one manually via {@link CreateCloudFormationChangeSetRequest#builder()}
*
*
* @param createCloudFormationChangeSetRequest
* A {@link Consumer} that will call methods on {@link CreateCloudFormationChangeSetRequest.Builder} to
* create a request. Create application ChangeSet request
* @return Result of the CreateCloudFormationChangeSet operation returned by the service.
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.CreateCloudFormationChangeSet
* @see AWS API Documentation
*/
default CreateCloudFormationChangeSetResponse createCloudFormationChangeSet(
Consumer createCloudFormationChangeSetRequest)
throws TooManyRequestsException, BadRequestException, InternalServerErrorException, ForbiddenException,
AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
return createCloudFormationChangeSet(CreateCloudFormationChangeSetRequest.builder()
.apply(createCloudFormationChangeSetRequest).build());
}
/**
* Gets the specified application.
*
* @param getApplicationRequest
* @return Result of the GetApplication operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.GetApplication
* @see AWS
* API Documentation
*/
default GetApplicationResponse getApplication(GetApplicationRequest getApplicationRequest) throws NotFoundException,
TooManyRequestsException, BadRequestException, InternalServerErrorException, ForbiddenException, AwsServiceException,
SdkClientException, ServerlessApplicationRepositoryException {
throw new UnsupportedOperationException();
}
/**
* Gets the specified application.
*
* This is a convenience which creates an instance of the {@link GetApplicationRequest.Builder} avoiding the need to
* create one manually via {@link GetApplicationRequest#builder()}
*
*
* @param getApplicationRequest
* A {@link Consumer} that will call methods on {@link GetApplicationRequest.Builder} to create a request.
* @return Result of the GetApplication operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.GetApplication
* @see AWS
* API Documentation
*/
default GetApplicationResponse getApplication(Consumer getApplicationRequest)
throws NotFoundException, TooManyRequestsException, BadRequestException, InternalServerErrorException,
ForbiddenException, AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
return getApplication(GetApplicationRequest.builder().apply(getApplicationRequest).build());
}
/**
* Gets the policy for the specified application.
*
* @param getApplicationPolicyRequest
* @return Result of the GetApplicationPolicy operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.GetApplicationPolicy
* @see AWS API Documentation
*/
default GetApplicationPolicyResponse getApplicationPolicy(GetApplicationPolicyRequest getApplicationPolicyRequest)
throws NotFoundException, TooManyRequestsException, BadRequestException, InternalServerErrorException,
ForbiddenException, AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
throw new UnsupportedOperationException();
}
/**
* Gets the policy for the specified application.
*
* This is a convenience which creates an instance of the {@link GetApplicationPolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetApplicationPolicyRequest#builder()}
*
*
* @param getApplicationPolicyRequest
* A {@link Consumer} that will call methods on {@link GetApplicationPolicyRequest.Builder} to create a
* request.
* @return Result of the GetApplicationPolicy operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.GetApplicationPolicy
* @see AWS API Documentation
*/
default GetApplicationPolicyResponse getApplicationPolicy(
Consumer getApplicationPolicyRequest) throws NotFoundException,
TooManyRequestsException, BadRequestException, InternalServerErrorException, ForbiddenException, AwsServiceException,
SdkClientException, ServerlessApplicationRepositoryException {
return getApplicationPolicy(GetApplicationPolicyRequest.builder().apply(getApplicationPolicyRequest).build());
}
/**
* Lists versions for the specified application.
*
* @param listApplicationVersionsRequest
* @return Result of the ListApplicationVersions operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.ListApplicationVersions
* @see AWS API Documentation
*/
default ListApplicationVersionsResponse listApplicationVersions(ListApplicationVersionsRequest listApplicationVersionsRequest)
throws NotFoundException, TooManyRequestsException, BadRequestException, InternalServerErrorException,
ForbiddenException, AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
throw new UnsupportedOperationException();
}
/**
* Lists versions for the specified application.
*
* This is a convenience which creates an instance of the {@link ListApplicationVersionsRequest.Builder} avoiding
* the need to create one manually via {@link ListApplicationVersionsRequest#builder()}
*
*
* @param listApplicationVersionsRequest
* A {@link Consumer} that will call methods on {@link ListApplicationVersionsRequest.Builder} to create a
* request.
* @return Result of the ListApplicationVersions operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.ListApplicationVersions
* @see AWS API Documentation
*/
default ListApplicationVersionsResponse listApplicationVersions(
Consumer listApplicationVersionsRequest) throws NotFoundException,
TooManyRequestsException, BadRequestException, InternalServerErrorException, ForbiddenException, AwsServiceException,
SdkClientException, ServerlessApplicationRepositoryException {
return listApplicationVersions(ListApplicationVersionsRequest.builder().apply(listApplicationVersionsRequest).build());
}
/**
* Lists applications owned by the requester.
*
* @return Result of the ListApplications operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.ListApplications
* @see #listApplications(ListApplicationsRequest)
* @see AWS API Documentation
*/
default ListApplicationsResponse listApplications() throws NotFoundException, BadRequestException,
InternalServerErrorException, ForbiddenException, AwsServiceException, SdkClientException,
ServerlessApplicationRepositoryException {
return listApplications(ListApplicationsRequest.builder().build());
}
/**
* Lists applications owned by the requester.
*
* @param listApplicationsRequest
* @return Result of the ListApplications operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.ListApplications
* @see AWS API Documentation
*/
default ListApplicationsResponse listApplications(ListApplicationsRequest listApplicationsRequest) throws NotFoundException,
BadRequestException, InternalServerErrorException, ForbiddenException, AwsServiceException, SdkClientException,
ServerlessApplicationRepositoryException {
throw new UnsupportedOperationException();
}
/**
* Lists applications owned by the requester.
*
* This is a convenience which creates an instance of the {@link ListApplicationsRequest.Builder} avoiding the need
* to create one manually via {@link ListApplicationsRequest#builder()}
*
*
* @param listApplicationsRequest
* A {@link Consumer} that will call methods on {@link ListApplicationsRequest.Builder} to create a request.
* @return Result of the ListApplications operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.ListApplications
* @see AWS API Documentation
*/
default ListApplicationsResponse listApplications(Consumer listApplicationsRequest)
throws NotFoundException, BadRequestException, InternalServerErrorException, ForbiddenException, AwsServiceException,
SdkClientException, ServerlessApplicationRepositoryException {
return listApplications(ListApplicationsRequest.builder().apply(listApplicationsRequest).build());
}
/**
* Puts the policy for the specified application.
*
* @param putApplicationPolicyRequest
* Put policy request
* @return Result of the PutApplicationPolicy operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.PutApplicationPolicy
* @see AWS API Documentation
*/
default PutApplicationPolicyResponse putApplicationPolicy(PutApplicationPolicyRequest putApplicationPolicyRequest)
throws NotFoundException, TooManyRequestsException, BadRequestException, InternalServerErrorException,
ForbiddenException, AwsServiceException, SdkClientException, ServerlessApplicationRepositoryException {
throw new UnsupportedOperationException();
}
/**
* Puts the policy for the specified application.
*
* This is a convenience which creates an instance of the {@link PutApplicationPolicyRequest.Builder} avoiding the
* need to create one manually via {@link PutApplicationPolicyRequest#builder()}
*
*
* @param putApplicationPolicyRequest
* A {@link Consumer} that will call methods on {@link PutApplicationPolicyRequest.Builder} to create a
* request. Put policy request
* @return Result of the PutApplicationPolicy operation returned by the service.
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.PutApplicationPolicy
* @see AWS API Documentation
*/
default PutApplicationPolicyResponse putApplicationPolicy(
Consumer putApplicationPolicyRequest) throws NotFoundException,
TooManyRequestsException, BadRequestException, InternalServerErrorException, ForbiddenException, AwsServiceException,
SdkClientException, ServerlessApplicationRepositoryException {
return putApplicationPolicy(PutApplicationPolicyRequest.builder().apply(putApplicationPolicyRequest).build());
}
/**
* Updates the specified application.
*
* @param updateApplicationRequest
* @return Result of the UpdateApplication operation returned by the service.
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws ConflictException
* 409 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.UpdateApplication
* @see AWS API Documentation
*/
default UpdateApplicationResponse updateApplication(UpdateApplicationRequest updateApplicationRequest)
throws BadRequestException, InternalServerErrorException, ForbiddenException, NotFoundException,
TooManyRequestsException, ConflictException, AwsServiceException, SdkClientException,
ServerlessApplicationRepositoryException {
throw new UnsupportedOperationException();
}
/**
* Updates the specified application.
*
* This is a convenience which creates an instance of the {@link UpdateApplicationRequest.Builder} avoiding the need
* to create one manually via {@link UpdateApplicationRequest#builder()}
*
*
* @param updateApplicationRequest
* A {@link Consumer} that will call methods on {@link UpdateApplicationRequest.Builder} to create a request.
* @return Result of the UpdateApplication operation returned by the service.
* @throws BadRequestException
* 400 response
* @throws InternalServerErrorException
* 500 response
* @throws ForbiddenException
* 403 response
* @throws NotFoundException
* 404 response
* @throws TooManyRequestsException
* 429 response
* @throws ConflictException
* 409 response
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ServerlessApplicationRepositoryException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ServerlessApplicationRepositoryClient.UpdateApplication
* @see AWS API Documentation
*/
default UpdateApplicationResponse updateApplication(Consumer updateApplicationRequest)
throws BadRequestException, InternalServerErrorException, ForbiddenException, NotFoundException,
TooManyRequestsException, ConflictException, AwsServiceException, SdkClientException,
ServerlessApplicationRepositoryException {
return updateApplication(UpdateApplicationRequest.builder().apply(updateApplicationRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("serverlessrepo");
}
}