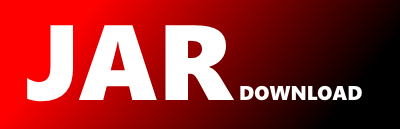
software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationResponse Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.serverlessapplicationrepository.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import javax.annotation.Generated;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public class CreateApplicationResponse extends ServerlessApplicationRepositoryResponse implements
ToCopyableBuilder {
private final String applicationId;
private final String author;
private final String creationTime;
private final String description;
private final List labels;
private final String licenseUrl;
private final String name;
private final String readmeUrl;
private final String spdxLicenseId;
private final Version version;
private CreateApplicationResponse(BuilderImpl builder) {
super(builder);
this.applicationId = builder.applicationId;
this.author = builder.author;
this.creationTime = builder.creationTime;
this.description = builder.description;
this.labels = builder.labels;
this.licenseUrl = builder.licenseUrl;
this.name = builder.name;
this.readmeUrl = builder.readmeUrl;
this.spdxLicenseId = builder.spdxLicenseId;
this.version = builder.version;
}
/**
* The application Amazon Resource Name (ARN).
*
* @return The application Amazon Resource Name (ARN).
*/
public String applicationId() {
return applicationId;
}
/**
* The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*
* @return The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*/
public String author() {
return author;
}
/**
* The date/time this resource was created.
*
* @return The date/time this resource was created.
*/
public String creationTime() {
return creationTime;
}
/**
* The description of the application.\nMin Length=1. Max Length=256
*
* @return The description of the application.\nMin Length=1. Max Length=256
*/
public String description() {
return description;
}
/**
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of labels:
* 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of
* labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*/
public List labels() {
return labels;
}
/**
* A link to a license file of the app that matches the spdxLicenseID of your application.\nMax size 5 MB
*
* @return A link to a license file of the app that matches the spdxLicenseID of your application.\nMax size 5 MB
*/
public String licenseUrl() {
return licenseUrl;
}
/**
* The name of the application.\nMin Length=1. Max Length=140\nPattern: "[a-zA-Z0-9\\-]+";
*
* @return The name of the application.\nMin Length=1. Max Length=140\nPattern: "[a-zA-Z0-9\\-]+";
*/
public String name() {
return name;
}
/**
* A link to the Readme file that contains a more detailed description of the application and how it works in
* markdown language.\nMax size 5 MB
*
* @return A link to the Readme file that contains a more detailed description of the application and how it works
* in markdown language.\nMax size 5 MB
*/
public String readmeUrl() {
return readmeUrl;
}
/**
* A valid identifier from https://spdx.org/licenses/.
*
* @return A valid identifier from https://spdx.org/licenses/.
*/
public String spdxLicenseId() {
return spdxLicenseId;
}
/**
* Version information about the application.
*
* @return Version information about the application.
*/
public Version version() {
return version;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(applicationId());
hashCode = 31 * hashCode + Objects.hashCode(author());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(labels());
hashCode = 31 * hashCode + Objects.hashCode(licenseUrl());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(readmeUrl());
hashCode = 31 * hashCode + Objects.hashCode(spdxLicenseId());
hashCode = 31 * hashCode + Objects.hashCode(version());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateApplicationResponse)) {
return false;
}
CreateApplicationResponse other = (CreateApplicationResponse) obj;
return Objects.equals(applicationId(), other.applicationId()) && Objects.equals(author(), other.author())
&& Objects.equals(creationTime(), other.creationTime()) && Objects.equals(description(), other.description())
&& Objects.equals(labels(), other.labels()) && Objects.equals(licenseUrl(), other.licenseUrl())
&& Objects.equals(name(), other.name()) && Objects.equals(readmeUrl(), other.readmeUrl())
&& Objects.equals(spdxLicenseId(), other.spdxLicenseId()) && Objects.equals(version(), other.version());
}
@Override
public String toString() {
return ToString.builder("CreateApplicationResponse").add("ApplicationId", applicationId()).add("Author", author())
.add("CreationTime", creationTime()).add("Description", description()).add("Labels", labels())
.add("LicenseUrl", licenseUrl()).add("Name", name()).add("ReadmeUrl", readmeUrl())
.add("SpdxLicenseId", spdxLicenseId()).add("Version", version()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApplicationId":
return Optional.of(clazz.cast(applicationId()));
case "Author":
return Optional.of(clazz.cast(author()));
case "CreationTime":
return Optional.of(clazz.cast(creationTime()));
case "Description":
return Optional.of(clazz.cast(description()));
case "Labels":
return Optional.of(clazz.cast(labels()));
case "LicenseUrl":
return Optional.of(clazz.cast(licenseUrl()));
case "Name":
return Optional.of(clazz.cast(name()));
case "ReadmeUrl":
return Optional.of(clazz.cast(readmeUrl()));
case "SpdxLicenseId":
return Optional.of(clazz.cast(spdxLicenseId()));
case "Version":
return Optional.of(clazz.cast(version()));
default:
return Optional.empty();
}
}
public interface Builder extends ServerlessApplicationRepositoryResponse.Builder,
CopyableBuilder {
/**
* The application Amazon Resource Name (ARN).
*
* @param applicationId
* The application Amazon Resource Name (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder applicationId(String applicationId);
/**
* The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*
* @param author
* The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder author(String author);
/**
* The date/time this resource was created.
*
* @param creationTime
* The date/time this resource was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder creationTime(String creationTime);
/**
* The description of the application.\nMin Length=1. Max Length=256
*
* @param description
* The description of the application.\nMin Length=1. Max Length=256
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of
* labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
* @param labels
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number
* of labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder labels(Collection labels);
/**
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of
* labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
* @param labels
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number
* of labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder labels(String... labels);
/**
* A link to a license file of the app that matches the spdxLicenseID of your application.\nMax size 5 MB
*
* @param licenseUrl
* A link to a license file of the app that matches the spdxLicenseID of your application.\nMax size 5 MB
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder licenseUrl(String licenseUrl);
/**
* The name of the application.\nMin Length=1. Max Length=140\nPattern: "[a-zA-Z0-9\\-]+";
*
* @param name
* The name of the application.\nMin Length=1. Max Length=140\nPattern: "[a-zA-Z0-9\\-]+";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder name(String name);
/**
* A link to the Readme file that contains a more detailed description of the application and how it works in
* markdown language.\nMax size 5 MB
*
* @param readmeUrl
* A link to the Readme file that contains a more detailed description of the application and how it
* works in markdown language.\nMax size 5 MB
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder readmeUrl(String readmeUrl);
/**
* A valid identifier from https://spdx.org/licenses/.
*
* @param spdxLicenseId
* A valid identifier from https://spdx.org/licenses/.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder spdxLicenseId(String spdxLicenseId);
/**
* Version information about the application.
*
* @param version
* Version information about the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder version(Version version);
/**
* Version information about the application. This is a convenience that creates an instance of the
* {@link Version.Builder} avoiding the need to create one manually via {@link Version#builder()}.
*
* When the {@link Consumer} completes, {@link Version.Builder#build()} is called immediately and its result is
* passed to {@link #version(Version)}.
*
* @param version
* a consumer that will call methods on {@link Version.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #version(Version)
*/
default Builder version(Consumer version) {
return version(Version.builder().apply(version).build());
}
}
static final class BuilderImpl extends ServerlessApplicationRepositoryResponse.BuilderImpl implements Builder {
private String applicationId;
private String author;
private String creationTime;
private String description;
private List labels;
private String licenseUrl;
private String name;
private String readmeUrl;
private String spdxLicenseId;
private Version version;
private BuilderImpl() {
}
private BuilderImpl(CreateApplicationResponse model) {
super(model);
applicationId(model.applicationId);
author(model.author);
creationTime(model.creationTime);
description(model.description);
labels(model.labels);
licenseUrl(model.licenseUrl);
name(model.name);
readmeUrl(model.readmeUrl);
spdxLicenseId(model.spdxLicenseId);
version(model.version);
}
public final String getApplicationId() {
return applicationId;
}
@Override
public final Builder applicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
public final void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
public final String getAuthor() {
return author;
}
@Override
public final Builder author(String author) {
this.author = author;
return this;
}
public final void setAuthor(String author) {
this.author = author;
}
public final String getCreationTime() {
return creationTime;
}
@Override
public final Builder creationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
public final void setCreationTime(String creationTime) {
this.creationTime = creationTime;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
public final Collection getLabels() {
return labels;
}
@Override
public final Builder labels(Collection labels) {
this.labels = ListOf__stringCopier.copy(labels);
return this;
}
@Override
@SafeVarargs
public final Builder labels(String... labels) {
labels(Arrays.asList(labels));
return this;
}
public final void setLabels(Collection labels) {
this.labels = ListOf__stringCopier.copy(labels);
}
public final String getLicenseUrl() {
return licenseUrl;
}
@Override
public final Builder licenseUrl(String licenseUrl) {
this.licenseUrl = licenseUrl;
return this;
}
public final void setLicenseUrl(String licenseUrl) {
this.licenseUrl = licenseUrl;
}
public final String getName() {
return name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final void setName(String name) {
this.name = name;
}
public final String getReadmeUrl() {
return readmeUrl;
}
@Override
public final Builder readmeUrl(String readmeUrl) {
this.readmeUrl = readmeUrl;
return this;
}
public final void setReadmeUrl(String readmeUrl) {
this.readmeUrl = readmeUrl;
}
public final String getSpdxLicenseId() {
return spdxLicenseId;
}
@Override
public final Builder spdxLicenseId(String spdxLicenseId) {
this.spdxLicenseId = spdxLicenseId;
return this;
}
public final void setSpdxLicenseId(String spdxLicenseId) {
this.spdxLicenseId = spdxLicenseId;
}
public final Version.Builder getVersion() {
return version != null ? version.toBuilder() : null;
}
@Override
public final Builder version(Version version) {
this.version = version;
return this;
}
public final void setVersion(Version.BuilderImpl version) {
this.version = version != null ? version.build() : null;
}
@Override
public CreateApplicationResponse build() {
return new CreateApplicationResponse(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy