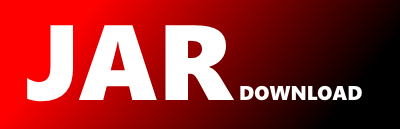
software.amazon.awssdk.services.serverlessapplicationrepository.model.ParameterDefinition Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.serverlessapplicationrepository.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.protocol.ProtocolMarshaller;
import software.amazon.awssdk.core.protocol.StructuredPojo;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.ParameterDefinitionMarshaller;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Parameters supported by the application.
*/
@Generated("software.amazon.awssdk:codegen")
public class ParameterDefinition implements StructuredPojo, ToCopyableBuilder {
private final String allowedPattern;
private final List allowedValues;
private final String constraintDescription;
private final String defaultValue;
private final String description;
private final Integer maxLength;
private final Integer maxValue;
private final Integer minLength;
private final Integer minValue;
private final String name;
private final Boolean noEcho;
private final List referencedByResources;
private final String type;
private ParameterDefinition(BuilderImpl builder) {
this.allowedPattern = builder.allowedPattern;
this.allowedValues = builder.allowedValues;
this.constraintDescription = builder.constraintDescription;
this.defaultValue = builder.defaultValue;
this.description = builder.description;
this.maxLength = builder.maxLength;
this.maxValue = builder.maxValue;
this.minLength = builder.minLength;
this.minValue = builder.minValue;
this.name = builder.name;
this.noEcho = builder.noEcho;
this.referencedByResources = builder.referencedByResources;
this.type = builder.type;
}
/**
* A regular expression that represents the patterns to allow for String types.
*
* @return A regular expression that represents the patterns to allow for String types.
*/
public String allowedPattern() {
return allowedPattern;
}
/**
* Array containing the list of values allowed for the parameter.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Array containing the list of values allowed for the parameter.
*/
public List allowedValues() {
return allowedValues;
}
/**
* A string that explains a constraint when the constraint is violated. For example, without a constraint
* description,\n a parameter that has an allowed pattern of [A-Za-z0-9]+ displays the following error message when
* the user\n specifies an invalid value:\n\n Malformed input-Parameter MyParameter must match pattern [A-Za-z0-9]+
* \n \nBy adding a constraint description, such as
* "must contain only uppercase and lowercase letters, and numbers," you can display\n the following customized
* error message:\n\n Malformed input-Parameter MyParameter must contain only uppercase and lowercase letters and
* numbers.
*
* @return A string that explains a constraint when the constraint is violated. For example, without a constraint
* description,\n a parameter that has an allowed pattern of [A-Za-z0-9]+ displays the following error
* message when the user\n specifies an invalid value:\n\n Malformed input-Parameter MyParameter must match
* pattern [A-Za-z0-9]+ \n \nBy adding a constraint description, such as
* "must contain only uppercase and lowercase letters, and numbers," you can display\n the following
* customized error message:\n\n Malformed input-Parameter MyParameter must contain only uppercase and
* lowercase letters and numbers.
*/
public String constraintDescription() {
return constraintDescription;
}
/**
* A value of the appropriate type for the template to use if no value is specified when a stack is created.\n If
* you define constraints for the parameter, you must specify a value that adheres to those constraints.
*
* @return A value of the appropriate type for the template to use if no value is specified when a stack is
* created.\n If you define constraints for the parameter, you must specify a value that adheres to those
* constraints.
*/
public String defaultValue() {
return defaultValue;
}
/**
* A string of up to 4,000 characters that describes the parameter.
*
* @return A string of up to 4,000 characters that describes the parameter.
*/
public String description() {
return description;
}
/**
* An integer value that determines the largest number of characters you want to allow for String types.
*
* @return An integer value that determines the largest number of characters you want to allow for String types.
*/
public Integer maxLength() {
return maxLength;
}
/**
* A numeric value that determines the largest numeric value you want to allow for Number types.
*
* @return A numeric value that determines the largest numeric value you want to allow for Number types.
*/
public Integer maxValue() {
return maxValue;
}
/**
* An integer value that determines the smallest number of characters you want to allow for String types.
*
* @return An integer value that determines the smallest number of characters you want to allow for String types.
*/
public Integer minLength() {
return minLength;
}
/**
* A numeric value that determines the smallest numeric value you want to allow for Number types.
*
* @return A numeric value that determines the smallest numeric value you want to allow for Number types.
*/
public Integer minValue() {
return minValue;
}
/**
* The name of the parameter.
*
* @return The name of the parameter.
*/
public String name() {
return name;
}
/**
* Whether to mask the parameter value whenever anyone makes a call that describes the stack. If you set the\n value
* to true, the parameter value is masked with asterisks (*****).
*
* @return Whether to mask the parameter value whenever anyone makes a call that describes the stack. If you set
* the\n value to true, the parameter value is masked with asterisks (*****).
*/
public Boolean noEcho() {
return noEcho;
}
/**
* A list of SAM resources that use this parameter.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of SAM resources that use this parameter.
*/
public List referencedByResources() {
return referencedByResources;
}
/**
* The type of the parameter.\nValid values: String | Number | List | CommaDelimitedList \n \n\n String : A
* literal string.\nFor example, users could specify "MyUserName" .\n\n Number : An integer or float. AWS
* CloudFormation validates the parameter value as a number; however, when you use the\n parameter elsewhere in your
* template (for example, by using the Ref intrinsic function), the parameter value becomes a string.\nFor example,
* users could specify "8888" .\n\n List : An array of integers or floats that are separated by commas. AWS
* CloudFormation validates the parameter value as numbers; however, when\n you use the parameter elsewhere in your
* template (for example, by using the Ref intrinsic function), the parameter value becomes a list of strings.\nFor
* example, users could specify "80,20", and a Ref results in ["80","20"] .\n\n CommaDelimitedList : An array of
* literal strings that are separated by commas. The total number of strings should be one more than the total
* number of commas.\n Also, each member string is space-trimmed.\nFor example, users could specify "test,dev,prod",
* and a Ref results in ["test","dev","prod"] .
*
* @return The type of the parameter.\nValid values: String | Number | List | CommaDelimitedList \n \n\n
* String : A literal string.\nFor example, users could specify "MyUserName" .\n\n Number : An integer or
* float. AWS CloudFormation validates the parameter value as a number; however, when you use the\n
* parameter elsewhere in your template (for example, by using the Ref intrinsic function), the parameter
* value becomes a string.\nFor example, users could specify "8888" .\n\n List : An array of
* integers or floats that are separated by commas. AWS CloudFormation validates the parameter value as
* numbers; however, when\n you use the parameter elsewhere in your template (for example, by using the Ref
* intrinsic function), the parameter value becomes a list of strings.\nFor example, users could specify
* "80,20", and a Ref results in ["80","20"] .\n\n CommaDelimitedList : An array of literal strings that are
* separated by commas. The total number of strings should be one more than the total number of commas.\n
* Also, each member string is space-trimmed.\nFor example, users could specify "test,dev,prod", and a Ref
* results in ["test","dev","prod"] .
*/
public String type() {
return type;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(allowedPattern());
hashCode = 31 * hashCode + Objects.hashCode(allowedValues());
hashCode = 31 * hashCode + Objects.hashCode(constraintDescription());
hashCode = 31 * hashCode + Objects.hashCode(defaultValue());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(maxLength());
hashCode = 31 * hashCode + Objects.hashCode(maxValue());
hashCode = 31 * hashCode + Objects.hashCode(minLength());
hashCode = 31 * hashCode + Objects.hashCode(minValue());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(noEcho());
hashCode = 31 * hashCode + Objects.hashCode(referencedByResources());
hashCode = 31 * hashCode + Objects.hashCode(type());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ParameterDefinition)) {
return false;
}
ParameterDefinition other = (ParameterDefinition) obj;
return Objects.equals(allowedPattern(), other.allowedPattern()) && Objects.equals(allowedValues(), other.allowedValues())
&& Objects.equals(constraintDescription(), other.constraintDescription())
&& Objects.equals(defaultValue(), other.defaultValue()) && Objects.equals(description(), other.description())
&& Objects.equals(maxLength(), other.maxLength()) && Objects.equals(maxValue(), other.maxValue())
&& Objects.equals(minLength(), other.minLength()) && Objects.equals(minValue(), other.minValue())
&& Objects.equals(name(), other.name()) && Objects.equals(noEcho(), other.noEcho())
&& Objects.equals(referencedByResources(), other.referencedByResources()) && Objects.equals(type(), other.type());
}
@Override
public String toString() {
return ToString.builder("ParameterDefinition").add("AllowedPattern", allowedPattern())
.add("AllowedValues", allowedValues()).add("ConstraintDescription", constraintDescription())
.add("DefaultValue", defaultValue()).add("Description", description()).add("MaxLength", maxLength())
.add("MaxValue", maxValue()).add("MinLength", minLength()).add("MinValue", minValue()).add("Name", name())
.add("NoEcho", noEcho()).add("ReferencedByResources", referencedByResources()).add("Type", type()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AllowedPattern":
return Optional.of(clazz.cast(allowedPattern()));
case "AllowedValues":
return Optional.of(clazz.cast(allowedValues()));
case "ConstraintDescription":
return Optional.of(clazz.cast(constraintDescription()));
case "DefaultValue":
return Optional.of(clazz.cast(defaultValue()));
case "Description":
return Optional.of(clazz.cast(description()));
case "MaxLength":
return Optional.of(clazz.cast(maxLength()));
case "MaxValue":
return Optional.of(clazz.cast(maxValue()));
case "MinLength":
return Optional.of(clazz.cast(minLength()));
case "MinValue":
return Optional.of(clazz.cast(minValue()));
case "Name":
return Optional.of(clazz.cast(name()));
case "NoEcho":
return Optional.of(clazz.cast(noEcho()));
case "ReferencedByResources":
return Optional.of(clazz.cast(referencedByResources()));
case "Type":
return Optional.of(clazz.cast(type()));
default:
return Optional.empty();
}
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
ParameterDefinitionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
* A regular expression that represents the patterns to allow for String types.
*
* @param allowedPattern
* A regular expression that represents the patterns to allow for String types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder allowedPattern(String allowedPattern);
/**
* Array containing the list of values allowed for the parameter.
*
* @param allowedValues
* Array containing the list of values allowed for the parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder allowedValues(Collection allowedValues);
/**
* Array containing the list of values allowed for the parameter.
*
* @param allowedValues
* Array containing the list of values allowed for the parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder allowedValues(String... allowedValues);
/**
* A string that explains a constraint when the constraint is violated. For example, without a constraint
* description,\n a parameter that has an allowed pattern of [A-Za-z0-9]+ displays the following error message
* when the user\n specifies an invalid value:\n\n Malformed input-Parameter MyParameter must match pattern
* [A-Za-z0-9]+ \n \nBy adding a constraint description, such as
* "must contain only uppercase and lowercase letters, and numbers," you can display\n the following customized
* error message:\n\n Malformed input-Parameter MyParameter must contain only uppercase and lowercase letters
* and numbers.
*
* @param constraintDescription
* A string that explains a constraint when the constraint is violated. For example, without a constraint
* description,\n a parameter that has an allowed pattern of [A-Za-z0-9]+ displays the following error
* message when the user\n specifies an invalid value:\n\n Malformed input-Parameter MyParameter must
* match pattern [A-Za-z0-9]+ \n \nBy adding a constraint description, such as
* "must contain only uppercase and lowercase letters, and numbers," you can display\n the following
* customized error message:\n\n Malformed input-Parameter MyParameter must contain only uppercase and
* lowercase letters and numbers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder constraintDescription(String constraintDescription);
/**
* A value of the appropriate type for the template to use if no value is specified when a stack is created.\n
* If you define constraints for the parameter, you must specify a value that adheres to those constraints.
*
* @param defaultValue
* A value of the appropriate type for the template to use if no value is specified when a stack is
* created.\n If you define constraints for the parameter, you must specify a value that adheres to those
* constraints.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder defaultValue(String defaultValue);
/**
* A string of up to 4,000 characters that describes the parameter.
*
* @param description
* A string of up to 4,000 characters that describes the parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
* An integer value that determines the largest number of characters you want to allow for String types.
*
* @param maxLength
* An integer value that determines the largest number of characters you want to allow for String types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder maxLength(Integer maxLength);
/**
* A numeric value that determines the largest numeric value you want to allow for Number types.
*
* @param maxValue
* A numeric value that determines the largest numeric value you want to allow for Number types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder maxValue(Integer maxValue);
/**
* An integer value that determines the smallest number of characters you want to allow for String types.
*
* @param minLength
* An integer value that determines the smallest number of characters you want to allow for String types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder minLength(Integer minLength);
/**
* A numeric value that determines the smallest numeric value you want to allow for Number types.
*
* @param minValue
* A numeric value that determines the smallest numeric value you want to allow for Number types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder minValue(Integer minValue);
/**
* The name of the parameter.
*
* @param name
* The name of the parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder name(String name);
/**
* Whether to mask the parameter value whenever anyone makes a call that describes the stack. If you set the\n
* value to true, the parameter value is masked with asterisks (*****).
*
* @param noEcho
* Whether to mask the parameter value whenever anyone makes a call that describes the stack. If you set
* the\n value to true, the parameter value is masked with asterisks (*****).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder noEcho(Boolean noEcho);
/**
* A list of SAM resources that use this parameter.
*
* @param referencedByResources
* A list of SAM resources that use this parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder referencedByResources(Collection referencedByResources);
/**
* A list of SAM resources that use this parameter.
*
* @param referencedByResources
* A list of SAM resources that use this parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder referencedByResources(String... referencedByResources);
/**
* The type of the parameter.\nValid values: String | Number | List | CommaDelimitedList \n \n\n String
* : A literal string.\nFor example, users could specify "MyUserName" .\n\n Number : An integer or float. AWS
* CloudFormation validates the parameter value as a number; however, when you use the\n parameter elsewhere in
* your template (for example, by using the Ref intrinsic function), the parameter value becomes a string.\nFor
* example, users could specify "8888" .\n\n List : An array of integers or floats that are separated by
* commas. AWS CloudFormation validates the parameter value as numbers; however, when\n you use the parameter
* elsewhere in your template (for example, by using the Ref intrinsic function), the parameter value becomes a
* list of strings.\nFor example, users could specify "80,20", and a Ref results in ["80","20"] .\n\n
* CommaDelimitedList : An array of literal strings that are separated by commas. The total number of strings
* should be one more than the total number of commas.\n Also, each member string is space-trimmed.\nFor
* example, users could specify "test,dev,prod", and a Ref results in ["test","dev","prod"] .
*
* @param type
* The type of the parameter.\nValid values: String | Number | List | CommaDelimitedList \n \n\n
* String : A literal string.\nFor example, users could specify "MyUserName" .\n\n Number : An integer or
* float. AWS CloudFormation validates the parameter value as a number; however, when you use the\n
* parameter elsewhere in your template (for example, by using the Ref intrinsic function), the parameter
* value becomes a string.\nFor example, users could specify "8888" .\n\n List : An array of
* integers or floats that are separated by commas. AWS CloudFormation validates the parameter value as
* numbers; however, when\n you use the parameter elsewhere in your template (for example, by using the
* Ref intrinsic function), the parameter value becomes a list of strings.\nFor example, users could
* specify "80,20", and a Ref results in ["80","20"] .\n\n CommaDelimitedList : An array of literal
* strings that are separated by commas. The total number of strings should be one more than the total
* number of commas.\n Also, each member string is space-trimmed.\nFor example, users could specify
* "test,dev,prod", and a Ref results in ["test","dev","prod"] .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder type(String type);
}
static final class BuilderImpl implements Builder {
private String allowedPattern;
private List allowedValues;
private String constraintDescription;
private String defaultValue;
private String description;
private Integer maxLength;
private Integer maxValue;
private Integer minLength;
private Integer minValue;
private String name;
private Boolean noEcho;
private List referencedByResources;
private String type;
private BuilderImpl() {
}
private BuilderImpl(ParameterDefinition model) {
allowedPattern(model.allowedPattern);
allowedValues(model.allowedValues);
constraintDescription(model.constraintDescription);
defaultValue(model.defaultValue);
description(model.description);
maxLength(model.maxLength);
maxValue(model.maxValue);
minLength(model.minLength);
minValue(model.minValue);
name(model.name);
noEcho(model.noEcho);
referencedByResources(model.referencedByResources);
type(model.type);
}
public final String getAllowedPattern() {
return allowedPattern;
}
@Override
public final Builder allowedPattern(String allowedPattern) {
this.allowedPattern = allowedPattern;
return this;
}
public final void setAllowedPattern(String allowedPattern) {
this.allowedPattern = allowedPattern;
}
public final Collection getAllowedValues() {
return allowedValues;
}
@Override
public final Builder allowedValues(Collection allowedValues) {
this.allowedValues = ListOf__stringCopier.copy(allowedValues);
return this;
}
@Override
@SafeVarargs
public final Builder allowedValues(String... allowedValues) {
allowedValues(Arrays.asList(allowedValues));
return this;
}
public final void setAllowedValues(Collection allowedValues) {
this.allowedValues = ListOf__stringCopier.copy(allowedValues);
}
public final String getConstraintDescription() {
return constraintDescription;
}
@Override
public final Builder constraintDescription(String constraintDescription) {
this.constraintDescription = constraintDescription;
return this;
}
public final void setConstraintDescription(String constraintDescription) {
this.constraintDescription = constraintDescription;
}
public final String getDefaultValue() {
return defaultValue;
}
@Override
public final Builder defaultValue(String defaultValue) {
this.defaultValue = defaultValue;
return this;
}
public final void setDefaultValue(String defaultValue) {
this.defaultValue = defaultValue;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
public final Integer getMaxLength() {
return maxLength;
}
@Override
public final Builder maxLength(Integer maxLength) {
this.maxLength = maxLength;
return this;
}
public final void setMaxLength(Integer maxLength) {
this.maxLength = maxLength;
}
public final Integer getMaxValue() {
return maxValue;
}
@Override
public final Builder maxValue(Integer maxValue) {
this.maxValue = maxValue;
return this;
}
public final void setMaxValue(Integer maxValue) {
this.maxValue = maxValue;
}
public final Integer getMinLength() {
return minLength;
}
@Override
public final Builder minLength(Integer minLength) {
this.minLength = minLength;
return this;
}
public final void setMinLength(Integer minLength) {
this.minLength = minLength;
}
public final Integer getMinValue() {
return minValue;
}
@Override
public final Builder minValue(Integer minValue) {
this.minValue = minValue;
return this;
}
public final void setMinValue(Integer minValue) {
this.minValue = minValue;
}
public final String getName() {
return name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final void setName(String name) {
this.name = name;
}
public final Boolean getNoEcho() {
return noEcho;
}
@Override
public final Builder noEcho(Boolean noEcho) {
this.noEcho = noEcho;
return this;
}
public final void setNoEcho(Boolean noEcho) {
this.noEcho = noEcho;
}
public final Collection getReferencedByResources() {
return referencedByResources;
}
@Override
public final Builder referencedByResources(Collection referencedByResources) {
this.referencedByResources = ListOf__stringCopier.copy(referencedByResources);
return this;
}
@Override
@SafeVarargs
public final Builder referencedByResources(String... referencedByResources) {
referencedByResources(Arrays.asList(referencedByResources));
return this;
}
public final void setReferencedByResources(Collection referencedByResources) {
this.referencedByResources = ListOf__stringCopier.copy(referencedByResources);
}
public final String getType() {
return type;
}
@Override
public final Builder type(String type) {
this.type = type;
return this;
}
public final void setType(String type) {
this.type = type;
}
@Override
public ParameterDefinition build() {
return new ParameterDefinition(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy