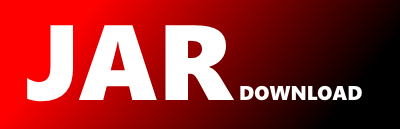
software.amazon.awssdk.services.serverlessapplicationrepository.model.ApplicationPolicyStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessrepo Show documentation
Show all versions of serverlessrepo Show documentation
The AWS Java SDK for AWSServerlessApplicationRepository module holds the client classes that are used for communicating
with AWSServerlessApplicationRepository Service
The newest version!
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.serverlessapplicationrepository.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.protocol.ProtocolMarshaller;
import software.amazon.awssdk.core.protocol.StructuredPojo;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.services.serverlessapplicationrepository.transform.ApplicationPolicyStatementMarshaller;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Policy statement applied to the application.
*/
@Generated("software.amazon.awssdk:codegen")
public final class ApplicationPolicyStatement implements StructuredPojo,
ToCopyableBuilder {
private final List actions;
private final List principals;
private final String statementId;
private ApplicationPolicyStatement(BuilderImpl builder) {
this.actions = builder.actions;
this.principals = builder.principals;
this.statementId = builder.statementId;
}
/**
* A list of supported actions:\n\n GetApplication \n \n\n CreateCloudFormationChangeSet \n \n\n
* ListApplicationVersions \n \n\n SearchApplications \n \n\n Deploy (Note: This action enables all other actions
* above.)
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of supported actions:\n\n GetApplication \n \n\n CreateCloudFormationChangeSet \n \n\n
* ListApplicationVersions \n \n\n SearchApplications \n \n\n Deploy (Note: This action enables all other
* actions above.)
*/
public List actions() {
return actions;
}
/**
* An AWS account ID, or * to make the application public.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return An AWS account ID, or * to make the application public.
*/
public List principals() {
return principals;
}
/**
* A unique ID for the statement.
*
* @return A unique ID for the statement.
*/
public String statementId() {
return statementId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(actions());
hashCode = 31 * hashCode + Objects.hashCode(principals());
hashCode = 31 * hashCode + Objects.hashCode(statementId());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ApplicationPolicyStatement)) {
return false;
}
ApplicationPolicyStatement other = (ApplicationPolicyStatement) obj;
return Objects.equals(actions(), other.actions()) && Objects.equals(principals(), other.principals())
&& Objects.equals(statementId(), other.statementId());
}
@Override
public String toString() {
return ToString.builder("ApplicationPolicyStatement").add("Actions", actions()).add("Principals", principals())
.add("StatementId", statementId()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Actions":
return Optional.ofNullable(clazz.cast(actions()));
case "Principals":
return Optional.ofNullable(clazz.cast(principals()));
case "StatementId":
return Optional.ofNullable(clazz.cast(statementId()));
default:
return Optional.empty();
}
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
ApplicationPolicyStatementMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
* A list of supported actions:\n\n GetApplication \n \n\n CreateCloudFormationChangeSet \n \n\n
* ListApplicationVersions \n \n\n SearchApplications \n \n\n Deploy (Note: This action enables all other
* actions above.)
*
* @param actions
* A list of supported actions:\n\n GetApplication \n \n\n CreateCloudFormationChangeSet \n \n\n
* ListApplicationVersions \n \n\n SearchApplications \n \n\n Deploy (Note: This action enables all other
* actions above.)
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder actions(Collection actions);
/**
* A list of supported actions:\n\n GetApplication \n \n\n CreateCloudFormationChangeSet \n \n\n
* ListApplicationVersions \n \n\n SearchApplications \n \n\n Deploy (Note: This action enables all other
* actions above.)
*
* @param actions
* A list of supported actions:\n\n GetApplication \n \n\n CreateCloudFormationChangeSet \n \n\n
* ListApplicationVersions \n \n\n SearchApplications \n \n\n Deploy (Note: This action enables all other
* actions above.)
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder actions(String... actions);
/**
* An AWS account ID, or * to make the application public.
*
* @param principals
* An AWS account ID, or * to make the application public.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder principals(Collection principals);
/**
* An AWS account ID, or * to make the application public.
*
* @param principals
* An AWS account ID, or * to make the application public.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder principals(String... principals);
/**
* A unique ID for the statement.
*
* @param statementId
* A unique ID for the statement.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder statementId(String statementId);
}
static final class BuilderImpl implements Builder {
private List actions = DefaultSdkAutoConstructList.getInstance();
private List principals = DefaultSdkAutoConstructList.getInstance();
private String statementId;
private BuilderImpl() {
}
private BuilderImpl(ApplicationPolicyStatement model) {
actions(model.actions);
principals(model.principals);
statementId(model.statementId);
}
public final Collection getActions() {
return actions;
}
@Override
public final Builder actions(Collection actions) {
this.actions = ListOf__stringCopier.copy(actions);
return this;
}
@Override
@SafeVarargs
public final Builder actions(String... actions) {
actions(Arrays.asList(actions));
return this;
}
public final void setActions(Collection actions) {
this.actions = ListOf__stringCopier.copy(actions);
}
public final Collection getPrincipals() {
return principals;
}
@Override
public final Builder principals(Collection principals) {
this.principals = ListOf__stringCopier.copy(principals);
return this;
}
@Override
@SafeVarargs
public final Builder principals(String... principals) {
principals(Arrays.asList(principals));
return this;
}
public final void setPrincipals(Collection principals) {
this.principals = ListOf__stringCopier.copy(principals);
}
public final String getStatementId() {
return statementId;
}
@Override
public final Builder statementId(String statementId) {
this.statementId = statementId;
return this;
}
public final void setStatementId(String statementId) {
this.statementId = statementId;
}
@Override
public ApplicationPolicyStatement build() {
return new ApplicationPolicyStatement(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy