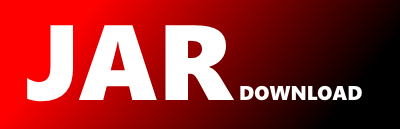
software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessrepo Show documentation
Show all versions of serverlessrepo Show documentation
The AWS Java SDK for AWSServerlessApplicationRepository module holds the client classes that are used for communicating
with AWSServerlessApplicationRepository Service
The newest version!
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.serverlessapplicationrepository.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateApplicationRequest extends ServerlessApplicationRepositoryRequest implements
ToCopyableBuilder {
private final String author;
private final String description;
private final List labels;
private final String licenseBody;
private final String licenseUrl;
private final String name;
private final String readmeBody;
private final String readmeUrl;
private final String semanticVersion;
private final String sourceCodeUrl;
private final String spdxLicenseId;
private final String templateBody;
private final String templateUrl;
private CreateApplicationRequest(BuilderImpl builder) {
super(builder);
this.author = builder.author;
this.description = builder.description;
this.labels = builder.labels;
this.licenseBody = builder.licenseBody;
this.licenseUrl = builder.licenseUrl;
this.name = builder.name;
this.readmeBody = builder.readmeBody;
this.readmeUrl = builder.readmeUrl;
this.semanticVersion = builder.semanticVersion;
this.sourceCodeUrl = builder.sourceCodeUrl;
this.spdxLicenseId = builder.spdxLicenseId;
this.templateBody = builder.templateBody;
this.templateUrl = builder.templateUrl;
}
/**
* The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*
* @return The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*/
public String author() {
return author;
}
/**
* The description of the application.\nMin Length=1. Max Length=256
*
* @return The description of the application.\nMin Length=1. Max Length=256
*/
public String description() {
return description;
}
/**
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of labels:
* 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of
* labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*/
public List labels() {
return labels;
}
/**
* A raw text file that contains the license of the app that matches the spdxLicenseID of your application.\nMax
* size 5 MB
*
* @return A raw text file that contains the license of the app that matches the spdxLicenseID of your
* application.\nMax size 5 MB
*/
public String licenseBody() {
return licenseBody;
}
/**
* A link to a license file of the app that matches the spdxLicenseID of your application.\nMax size 5 MB
*
* @return A link to a license file of the app that matches the spdxLicenseID of your application.\nMax size 5 MB
*/
public String licenseUrl() {
return licenseUrl;
}
/**
* The name of the application you want to publish.\nMin Length=1. Max Length=140\nPattern: "[a-zA-Z0-9\\-]+";
*
* @return The name of the application you want to publish.\nMin Length=1. Max Length=140\nPattern:
* "[a-zA-Z0-9\\-]+";
*/
public String name() {
return name;
}
/**
* A raw text Readme file that contains a more detailed description of the application and how it works in markdown
* language.\nMax size 5 MB
*
* @return A raw text Readme file that contains a more detailed description of the application and how it works in
* markdown language.\nMax size 5 MB
*/
public String readmeBody() {
return readmeBody;
}
/**
* A link to the Readme file that contains a more detailed description of the application and how it works in
* markdown language.\nMax size 5 MB
*
* @return A link to the Readme file that contains a more detailed description of the application and how it works
* in markdown language.\nMax size 5 MB
*/
public String readmeUrl() {
return readmeUrl;
}
/**
* The semantic version of the application:\n\n https://semver.org/
*
* @return The semantic version of the application:\n\n https://semver.org/
*/
public String semanticVersion() {
return semanticVersion;
}
/**
* A link to a public repository for the source code of your application.
*
* @return A link to a public repository for the source code of your application.
*/
public String sourceCodeUrl() {
return sourceCodeUrl;
}
/**
* A valid identifier from https://spdx.org/licenses/ .
*
* @return A valid identifier from https://spdx.org/licenses/ .
*/
public String spdxLicenseId() {
return spdxLicenseId;
}
/**
* The raw packaged SAM template of your application.
*
* @return The raw packaged SAM template of your application.
*/
public String templateBody() {
return templateBody;
}
/**
* A link to the packaged SAM template of your application.
*
* @return A link to the packaged SAM template of your application.
*/
public String templateUrl() {
return templateUrl;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(author());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(labels());
hashCode = 31 * hashCode + Objects.hashCode(licenseBody());
hashCode = 31 * hashCode + Objects.hashCode(licenseUrl());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(readmeBody());
hashCode = 31 * hashCode + Objects.hashCode(readmeUrl());
hashCode = 31 * hashCode + Objects.hashCode(semanticVersion());
hashCode = 31 * hashCode + Objects.hashCode(sourceCodeUrl());
hashCode = 31 * hashCode + Objects.hashCode(spdxLicenseId());
hashCode = 31 * hashCode + Objects.hashCode(templateBody());
hashCode = 31 * hashCode + Objects.hashCode(templateUrl());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateApplicationRequest)) {
return false;
}
CreateApplicationRequest other = (CreateApplicationRequest) obj;
return Objects.equals(author(), other.author()) && Objects.equals(description(), other.description())
&& Objects.equals(labels(), other.labels()) && Objects.equals(licenseBody(), other.licenseBody())
&& Objects.equals(licenseUrl(), other.licenseUrl()) && Objects.equals(name(), other.name())
&& Objects.equals(readmeBody(), other.readmeBody()) && Objects.equals(readmeUrl(), other.readmeUrl())
&& Objects.equals(semanticVersion(), other.semanticVersion())
&& Objects.equals(sourceCodeUrl(), other.sourceCodeUrl())
&& Objects.equals(spdxLicenseId(), other.spdxLicenseId()) && Objects.equals(templateBody(), other.templateBody())
&& Objects.equals(templateUrl(), other.templateUrl());
}
@Override
public String toString() {
return ToString.builder("CreateApplicationRequest").add("Author", author()).add("Description", description())
.add("Labels", labels()).add("LicenseBody", licenseBody()).add("LicenseUrl", licenseUrl()).add("Name", name())
.add("ReadmeBody", readmeBody()).add("ReadmeUrl", readmeUrl()).add("SemanticVersion", semanticVersion())
.add("SourceCodeUrl", sourceCodeUrl()).add("SpdxLicenseId", spdxLicenseId()).add("TemplateBody", templateBody())
.add("TemplateUrl", templateUrl()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Author":
return Optional.ofNullable(clazz.cast(author()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Labels":
return Optional.ofNullable(clazz.cast(labels()));
case "LicenseBody":
return Optional.ofNullable(clazz.cast(licenseBody()));
case "LicenseUrl":
return Optional.ofNullable(clazz.cast(licenseUrl()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "ReadmeBody":
return Optional.ofNullable(clazz.cast(readmeBody()));
case "ReadmeUrl":
return Optional.ofNullable(clazz.cast(readmeUrl()));
case "SemanticVersion":
return Optional.ofNullable(clazz.cast(semanticVersion()));
case "SourceCodeUrl":
return Optional.ofNullable(clazz.cast(sourceCodeUrl()));
case "SpdxLicenseId":
return Optional.ofNullable(clazz.cast(spdxLicenseId()));
case "TemplateBody":
return Optional.ofNullable(clazz.cast(templateBody()));
case "TemplateUrl":
return Optional.ofNullable(clazz.cast(templateUrl()));
default:
return Optional.empty();
}
}
public interface Builder extends ServerlessApplicationRepositoryRequest.Builder,
CopyableBuilder {
/**
* The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*
* @param author
* The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder author(String author);
/**
* The description of the application.\nMin Length=1. Max Length=256
*
* @param description
* The description of the application.\nMin Length=1. Max Length=256
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of
* labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
* @param labels
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number
* of labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder labels(Collection labels);
/**
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of
* labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
* @param labels
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number
* of labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder labels(String... labels);
/**
* A raw text file that contains the license of the app that matches the spdxLicenseID of your application.\nMax
* size 5 MB
*
* @param licenseBody
* A raw text file that contains the license of the app that matches the spdxLicenseID of your
* application.\nMax size 5 MB
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder licenseBody(String licenseBody);
/**
* A link to a license file of the app that matches the spdxLicenseID of your application.\nMax size 5 MB
*
* @param licenseUrl
* A link to a license file of the app that matches the spdxLicenseID of your application.\nMax size 5 MB
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder licenseUrl(String licenseUrl);
/**
* The name of the application you want to publish.\nMin Length=1. Max Length=140\nPattern: "[a-zA-Z0-9\\-]+";
*
* @param name
* The name of the application you want to publish.\nMin Length=1. Max Length=140\nPattern:
* "[a-zA-Z0-9\\-]+";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder name(String name);
/**
* A raw text Readme file that contains a more detailed description of the application and how it works in
* markdown language.\nMax size 5 MB
*
* @param readmeBody
* A raw text Readme file that contains a more detailed description of the application and how it works
* in markdown language.\nMax size 5 MB
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder readmeBody(String readmeBody);
/**
* A link to the Readme file that contains a more detailed description of the application and how it works in
* markdown language.\nMax size 5 MB
*
* @param readmeUrl
* A link to the Readme file that contains a more detailed description of the application and how it
* works in markdown language.\nMax size 5 MB
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder readmeUrl(String readmeUrl);
/**
* The semantic version of the application:\n\n https://semver.org/
*
* @param semanticVersion
* The semantic version of the application:\n\n https://semver.org/
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder semanticVersion(String semanticVersion);
/**
* A link to a public repository for the source code of your application.
*
* @param sourceCodeUrl
* A link to a public repository for the source code of your application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sourceCodeUrl(String sourceCodeUrl);
/**
* A valid identifier from https://spdx.org/licenses/ .
*
* @param spdxLicenseId
* A valid identifier from https://spdx.org/licenses/ .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder spdxLicenseId(String spdxLicenseId);
/**
* The raw packaged SAM template of your application.
*
* @param templateBody
* The raw packaged SAM template of your application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateBody(String templateBody);
/**
* A link to the packaged SAM template of your application.
*
* @param templateUrl
* A link to the packaged SAM template of your application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateUrl(String templateUrl);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends ServerlessApplicationRepositoryRequest.BuilderImpl implements Builder {
private String author;
private String description;
private List labels = DefaultSdkAutoConstructList.getInstance();
private String licenseBody;
private String licenseUrl;
private String name;
private String readmeBody;
private String readmeUrl;
private String semanticVersion;
private String sourceCodeUrl;
private String spdxLicenseId;
private String templateBody;
private String templateUrl;
private BuilderImpl() {
}
private BuilderImpl(CreateApplicationRequest model) {
super(model);
author(model.author);
description(model.description);
labels(model.labels);
licenseBody(model.licenseBody);
licenseUrl(model.licenseUrl);
name(model.name);
readmeBody(model.readmeBody);
readmeUrl(model.readmeUrl);
semanticVersion(model.semanticVersion);
sourceCodeUrl(model.sourceCodeUrl);
spdxLicenseId(model.spdxLicenseId);
templateBody(model.templateBody);
templateUrl(model.templateUrl);
}
public final String getAuthor() {
return author;
}
@Override
public final Builder author(String author) {
this.author = author;
return this;
}
public final void setAuthor(String author) {
this.author = author;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
public final Collection getLabels() {
return labels;
}
@Override
public final Builder labels(Collection labels) {
this.labels = ListOf__stringCopier.copy(labels);
return this;
}
@Override
@SafeVarargs
public final Builder labels(String... labels) {
labels(Arrays.asList(labels));
return this;
}
public final void setLabels(Collection labels) {
this.labels = ListOf__stringCopier.copy(labels);
}
public final String getLicenseBody() {
return licenseBody;
}
@Override
public final Builder licenseBody(String licenseBody) {
this.licenseBody = licenseBody;
return this;
}
public final void setLicenseBody(String licenseBody) {
this.licenseBody = licenseBody;
}
public final String getLicenseUrl() {
return licenseUrl;
}
@Override
public final Builder licenseUrl(String licenseUrl) {
this.licenseUrl = licenseUrl;
return this;
}
public final void setLicenseUrl(String licenseUrl) {
this.licenseUrl = licenseUrl;
}
public final String getName() {
return name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final void setName(String name) {
this.name = name;
}
public final String getReadmeBody() {
return readmeBody;
}
@Override
public final Builder readmeBody(String readmeBody) {
this.readmeBody = readmeBody;
return this;
}
public final void setReadmeBody(String readmeBody) {
this.readmeBody = readmeBody;
}
public final String getReadmeUrl() {
return readmeUrl;
}
@Override
public final Builder readmeUrl(String readmeUrl) {
this.readmeUrl = readmeUrl;
return this;
}
public final void setReadmeUrl(String readmeUrl) {
this.readmeUrl = readmeUrl;
}
public final String getSemanticVersion() {
return semanticVersion;
}
@Override
public final Builder semanticVersion(String semanticVersion) {
this.semanticVersion = semanticVersion;
return this;
}
public final void setSemanticVersion(String semanticVersion) {
this.semanticVersion = semanticVersion;
}
public final String getSourceCodeUrl() {
return sourceCodeUrl;
}
@Override
public final Builder sourceCodeUrl(String sourceCodeUrl) {
this.sourceCodeUrl = sourceCodeUrl;
return this;
}
public final void setSourceCodeUrl(String sourceCodeUrl) {
this.sourceCodeUrl = sourceCodeUrl;
}
public final String getSpdxLicenseId() {
return spdxLicenseId;
}
@Override
public final Builder spdxLicenseId(String spdxLicenseId) {
this.spdxLicenseId = spdxLicenseId;
return this;
}
public final void setSpdxLicenseId(String spdxLicenseId) {
this.spdxLicenseId = spdxLicenseId;
}
public final String getTemplateBody() {
return templateBody;
}
@Override
public final Builder templateBody(String templateBody) {
this.templateBody = templateBody;
return this;
}
public final void setTemplateBody(String templateBody) {
this.templateBody = templateBody;
}
public final String getTemplateUrl() {
return templateUrl;
}
@Override
public final Builder templateUrl(String templateUrl) {
this.templateUrl = templateUrl;
return this;
}
public final void setTemplateUrl(String templateUrl) {
this.templateUrl = templateUrl;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateApplicationRequest build() {
return new CreateApplicationRequest(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy