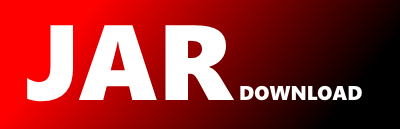
software.amazon.awssdk.services.serverlessapplicationrepository.model.CreateApplicationVersionResponse Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.serverlessapplicationrepository.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateApplicationVersionResponse extends ServerlessApplicationRepositoryResponse implements
ToCopyableBuilder {
private final String applicationId;
private final String creationTime;
private final List parameterDefinitions;
private final String semanticVersion;
private final String sourceCodeUrl;
private final String templateUrl;
private CreateApplicationVersionResponse(BuilderImpl builder) {
super(builder);
this.applicationId = builder.applicationId;
this.creationTime = builder.creationTime;
this.parameterDefinitions = builder.parameterDefinitions;
this.semanticVersion = builder.semanticVersion;
this.sourceCodeUrl = builder.sourceCodeUrl;
this.templateUrl = builder.templateUrl;
}
/**
* The application Amazon Resource Name (ARN).
*
* @return The application Amazon Resource Name (ARN).
*/
public String applicationId() {
return applicationId;
}
/**
* The date/time this resource was created.
*
* @return The date/time this resource was created.
*/
public String creationTime() {
return creationTime;
}
/**
* Array of parameter types supported by the application.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Array of parameter types supported by the application.
*/
public List parameterDefinitions() {
return parameterDefinitions;
}
/**
* The semantic version of the application:\n\n https://semver.org/
*
* @return The semantic version of the application:\n\n https://semver.org/
*/
public String semanticVersion() {
return semanticVersion;
}
/**
* A link to a public repository for the source code of your application.
*
* @return A link to a public repository for the source code of your application.
*/
public String sourceCodeUrl() {
return sourceCodeUrl;
}
/**
* A link to the packaged SAM template of your application.
*
* @return A link to the packaged SAM template of your application.
*/
public String templateUrl() {
return templateUrl;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(applicationId());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(parameterDefinitions());
hashCode = 31 * hashCode + Objects.hashCode(semanticVersion());
hashCode = 31 * hashCode + Objects.hashCode(sourceCodeUrl());
hashCode = 31 * hashCode + Objects.hashCode(templateUrl());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateApplicationVersionResponse)) {
return false;
}
CreateApplicationVersionResponse other = (CreateApplicationVersionResponse) obj;
return Objects.equals(applicationId(), other.applicationId()) && Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(parameterDefinitions(), other.parameterDefinitions())
&& Objects.equals(semanticVersion(), other.semanticVersion())
&& Objects.equals(sourceCodeUrl(), other.sourceCodeUrl()) && Objects.equals(templateUrl(), other.templateUrl());
}
@Override
public String toString() {
return ToString.builder("CreateApplicationVersionResponse").add("ApplicationId", applicationId())
.add("CreationTime", creationTime()).add("ParameterDefinitions", parameterDefinitions())
.add("SemanticVersion", semanticVersion()).add("SourceCodeUrl", sourceCodeUrl())
.add("TemplateUrl", templateUrl()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApplicationId":
return Optional.ofNullable(clazz.cast(applicationId()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "ParameterDefinitions":
return Optional.ofNullable(clazz.cast(parameterDefinitions()));
case "SemanticVersion":
return Optional.ofNullable(clazz.cast(semanticVersion()));
case "SourceCodeUrl":
return Optional.ofNullable(clazz.cast(sourceCodeUrl()));
case "TemplateUrl":
return Optional.ofNullable(clazz.cast(templateUrl()));
default:
return Optional.empty();
}
}
public interface Builder extends ServerlessApplicationRepositoryResponse.Builder,
CopyableBuilder {
/**
* The application Amazon Resource Name (ARN).
*
* @param applicationId
* The application Amazon Resource Name (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder applicationId(String applicationId);
/**
* The date/time this resource was created.
*
* @param creationTime
* The date/time this resource was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder creationTime(String creationTime);
/**
* Array of parameter types supported by the application.
*
* @param parameterDefinitions
* Array of parameter types supported by the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameterDefinitions(Collection parameterDefinitions);
/**
* Array of parameter types supported by the application.
*
* @param parameterDefinitions
* Array of parameter types supported by the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parameterDefinitions(ParameterDefinition... parameterDefinitions);
/**
* Array of parameter types supported by the application. This is a convenience that creates an instance of the
* {@link List.Builder} avoiding the need to create one manually via {@link List
* #builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately
* and its result is passed to {@link #parameterDefinitions(List)}.
*
* @param parameterDefinitions
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #parameterDefinitions(List)
*/
Builder parameterDefinitions(Consumer... parameterDefinitions);
/**
* The semantic version of the application:\n\n https://semver.org/
*
* @param semanticVersion
* The semantic version of the application:\n\n https://semver.org/
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder semanticVersion(String semanticVersion);
/**
* A link to a public repository for the source code of your application.
*
* @param sourceCodeUrl
* A link to a public repository for the source code of your application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sourceCodeUrl(String sourceCodeUrl);
/**
* A link to the packaged SAM template of your application.
*
* @param templateUrl
* A link to the packaged SAM template of your application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder templateUrl(String templateUrl);
}
static final class BuilderImpl extends ServerlessApplicationRepositoryResponse.BuilderImpl implements Builder {
private String applicationId;
private String creationTime;
private List parameterDefinitions = DefaultSdkAutoConstructList.getInstance();
private String semanticVersion;
private String sourceCodeUrl;
private String templateUrl;
private BuilderImpl() {
}
private BuilderImpl(CreateApplicationVersionResponse model) {
super(model);
applicationId(model.applicationId);
creationTime(model.creationTime);
parameterDefinitions(model.parameterDefinitions);
semanticVersion(model.semanticVersion);
sourceCodeUrl(model.sourceCodeUrl);
templateUrl(model.templateUrl);
}
public final String getApplicationId() {
return applicationId;
}
@Override
public final Builder applicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
public final void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
public final String getCreationTime() {
return creationTime;
}
@Override
public final Builder creationTime(String creationTime) {
this.creationTime = creationTime;
return this;
}
public final void setCreationTime(String creationTime) {
this.creationTime = creationTime;
}
public final Collection getParameterDefinitions() {
return parameterDefinitions != null ? parameterDefinitions.stream().map(ParameterDefinition::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder parameterDefinitions(Collection parameterDefinitions) {
this.parameterDefinitions = ListOfParameterDefinitionCopier.copy(parameterDefinitions);
return this;
}
@Override
@SafeVarargs
public final Builder parameterDefinitions(ParameterDefinition... parameterDefinitions) {
parameterDefinitions(Arrays.asList(parameterDefinitions));
return this;
}
@Override
@SafeVarargs
public final Builder parameterDefinitions(Consumer... parameterDefinitions) {
parameterDefinitions(Stream.of(parameterDefinitions).map(c -> ParameterDefinition.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setParameterDefinitions(Collection parameterDefinitions) {
this.parameterDefinitions = ListOfParameterDefinitionCopier.copyFromBuilder(parameterDefinitions);
}
public final String getSemanticVersion() {
return semanticVersion;
}
@Override
public final Builder semanticVersion(String semanticVersion) {
this.semanticVersion = semanticVersion;
return this;
}
public final void setSemanticVersion(String semanticVersion) {
this.semanticVersion = semanticVersion;
}
public final String getSourceCodeUrl() {
return sourceCodeUrl;
}
@Override
public final Builder sourceCodeUrl(String sourceCodeUrl) {
this.sourceCodeUrl = sourceCodeUrl;
return this;
}
public final void setSourceCodeUrl(String sourceCodeUrl) {
this.sourceCodeUrl = sourceCodeUrl;
}
public final String getTemplateUrl() {
return templateUrl;
}
@Override
public final Builder templateUrl(String templateUrl) {
this.templateUrl = templateUrl;
return this;
}
public final void setTemplateUrl(String templateUrl) {
this.templateUrl = templateUrl;
}
@Override
public CreateApplicationVersionResponse build() {
return new CreateApplicationVersionResponse(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy