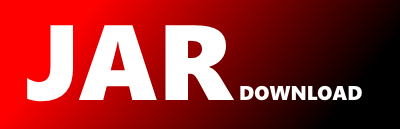
software.amazon.awssdk.services.serverlessapplicationrepository.model.UpdateApplicationRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessrepo Show documentation
Show all versions of serverlessrepo Show documentation
The AWS Java SDK for AWSServerlessApplicationRepository module holds the client classes that are used for communicating
with AWSServerlessApplicationRepository Service
The newest version!
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.serverlessapplicationrepository.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateApplicationRequest extends ServerlessApplicationRepositoryRequest implements
ToCopyableBuilder {
private final String applicationId;
private final String author;
private final String description;
private final List labels;
private final String readmeBody;
private final String readmeUrl;
private UpdateApplicationRequest(BuilderImpl builder) {
super(builder);
this.applicationId = builder.applicationId;
this.author = builder.author;
this.description = builder.description;
this.labels = builder.labels;
this.readmeBody = builder.readmeBody;
this.readmeUrl = builder.readmeUrl;
}
/**
* The id of the application to update
*
* @return The id of the application to update
*/
public String applicationId() {
return applicationId;
}
/**
* The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*
* @return The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*/
public String author() {
return author;
}
/**
* The description of the application.\nMin Length=1. Max Length=256
*
* @return The description of the application.\nMin Length=1. Max Length=256
*/
public String description() {
return description;
}
/**
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of labels:
* 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of
* labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*/
public List labels() {
return labels;
}
/**
* A raw text Readme file that contains a more detailed description of the application and how it works in markdown
* language.\nMax size 5 MB
*
* @return A raw text Readme file that contains a more detailed description of the application and how it works in
* markdown language.\nMax size 5 MB
*/
public String readmeBody() {
return readmeBody;
}
/**
* A link to the Readme file that contains a more detailed description of the application and how it works in
* markdown language.\nMax size 5 MB
*
* @return A link to the Readme file that contains a more detailed description of the application and how it works
* in markdown language.\nMax size 5 MB
*/
public String readmeUrl() {
return readmeUrl;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(applicationId());
hashCode = 31 * hashCode + Objects.hashCode(author());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(labels());
hashCode = 31 * hashCode + Objects.hashCode(readmeBody());
hashCode = 31 * hashCode + Objects.hashCode(readmeUrl());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateApplicationRequest)) {
return false;
}
UpdateApplicationRequest other = (UpdateApplicationRequest) obj;
return Objects.equals(applicationId(), other.applicationId()) && Objects.equals(author(), other.author())
&& Objects.equals(description(), other.description()) && Objects.equals(labels(), other.labels())
&& Objects.equals(readmeBody(), other.readmeBody()) && Objects.equals(readmeUrl(), other.readmeUrl());
}
@Override
public String toString() {
return ToString.builder("UpdateApplicationRequest").add("ApplicationId", applicationId()).add("Author", author())
.add("Description", description()).add("Labels", labels()).add("ReadmeBody", readmeBody())
.add("ReadmeUrl", readmeUrl()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApplicationId":
return Optional.ofNullable(clazz.cast(applicationId()));
case "Author":
return Optional.ofNullable(clazz.cast(author()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Labels":
return Optional.ofNullable(clazz.cast(labels()));
case "ReadmeBody":
return Optional.ofNullable(clazz.cast(readmeBody()));
case "ReadmeUrl":
return Optional.ofNullable(clazz.cast(readmeUrl()));
default:
return Optional.empty();
}
}
public interface Builder extends ServerlessApplicationRepositoryRequest.Builder,
CopyableBuilder {
/**
* The id of the application to update
*
* @param applicationId
* The id of the application to update
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder applicationId(String applicationId);
/**
* The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
*
* @param author
* The name of the author publishing the app.\nMin Length=1. Max Length=127.\nPattern
* "^[a-z0-9](([a-z0-9]|-(?!-))*[a-z0-9])?$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder author(String author);
/**
* The description of the application.\nMin Length=1. Max Length=256
*
* @param description
* The description of the application.\nMin Length=1. Max Length=256
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of
* labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
* @param labels
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number
* of labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder labels(Collection labels);
/**
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number of
* labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
*
* @param labels
* Labels to improve discovery of apps in search results.\nMin Length=1. Max Length=127. Maximum number
* of labels: 10\nPattern: "^[a-zA-Z0-9+\\-_:\\/@]+$";
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder labels(String... labels);
/**
* A raw text Readme file that contains a more detailed description of the application and how it works in
* markdown language.\nMax size 5 MB
*
* @param readmeBody
* A raw text Readme file that contains a more detailed description of the application and how it works
* in markdown language.\nMax size 5 MB
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder readmeBody(String readmeBody);
/**
* A link to the Readme file that contains a more detailed description of the application and how it works in
* markdown language.\nMax size 5 MB
*
* @param readmeUrl
* A link to the Readme file that contains a more detailed description of the application and how it
* works in markdown language.\nMax size 5 MB
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder readmeUrl(String readmeUrl);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends ServerlessApplicationRepositoryRequest.BuilderImpl implements Builder {
private String applicationId;
private String author;
private String description;
private List labels = DefaultSdkAutoConstructList.getInstance();
private String readmeBody;
private String readmeUrl;
private BuilderImpl() {
}
private BuilderImpl(UpdateApplicationRequest model) {
super(model);
applicationId(model.applicationId);
author(model.author);
description(model.description);
labels(model.labels);
readmeBody(model.readmeBody);
readmeUrl(model.readmeUrl);
}
public final String getApplicationId() {
return applicationId;
}
@Override
public final Builder applicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
public final void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
public final String getAuthor() {
return author;
}
@Override
public final Builder author(String author) {
this.author = author;
return this;
}
public final void setAuthor(String author) {
this.author = author;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
public final Collection getLabels() {
return labels;
}
@Override
public final Builder labels(Collection labels) {
this.labels = ListOf__stringCopier.copy(labels);
return this;
}
@Override
@SafeVarargs
public final Builder labels(String... labels) {
labels(Arrays.asList(labels));
return this;
}
public final void setLabels(Collection labels) {
this.labels = ListOf__stringCopier.copy(labels);
}
public final String getReadmeBody() {
return readmeBody;
}
@Override
public final Builder readmeBody(String readmeBody) {
this.readmeBody = readmeBody;
return this;
}
public final void setReadmeBody(String readmeBody) {
this.readmeBody = readmeBody;
}
public final String getReadmeUrl() {
return readmeUrl;
}
@Override
public final Builder readmeUrl(String readmeUrl) {
this.readmeUrl = readmeUrl;
return this;
}
public final void setReadmeUrl(String readmeUrl) {
this.readmeUrl = readmeUrl;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public UpdateApplicationRequest build() {
return new UpdateApplicationRequest(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy