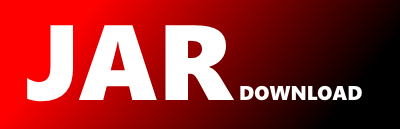
software.amazon.awssdk.services.sms.DefaultSmsClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of servermigration Show documentation
Show all versions of servermigration Show documentation
The AWS Java SDK for AWS Server Migration module holds the client classes that are used for
communicating with AWS Server Migration Service
The newest version!
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sms;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.protocol.json.AwsJsonProtocol;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolMetadata;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.internal.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.protocol.json.JsonClientMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorResponseMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorShapeMetadata;
import software.amazon.awssdk.core.protocol.json.JsonOperationMetadata;
import software.amazon.awssdk.services.sms.model.CreateReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.CreateReplicationJobResponse;
import software.amazon.awssdk.services.sms.model.DeleteReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.DeleteReplicationJobResponse;
import software.amazon.awssdk.services.sms.model.DeleteServerCatalogRequest;
import software.amazon.awssdk.services.sms.model.DeleteServerCatalogResponse;
import software.amazon.awssdk.services.sms.model.DisassociateConnectorRequest;
import software.amazon.awssdk.services.sms.model.DisassociateConnectorResponse;
import software.amazon.awssdk.services.sms.model.GetConnectorsRequest;
import software.amazon.awssdk.services.sms.model.GetConnectorsResponse;
import software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest;
import software.amazon.awssdk.services.sms.model.GetReplicationJobsResponse;
import software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest;
import software.amazon.awssdk.services.sms.model.GetReplicationRunsResponse;
import software.amazon.awssdk.services.sms.model.GetServersRequest;
import software.amazon.awssdk.services.sms.model.GetServersResponse;
import software.amazon.awssdk.services.sms.model.ImportServerCatalogRequest;
import software.amazon.awssdk.services.sms.model.ImportServerCatalogResponse;
import software.amazon.awssdk.services.sms.model.InternalErrorException;
import software.amazon.awssdk.services.sms.model.InvalidParameterException;
import software.amazon.awssdk.services.sms.model.MissingRequiredParameterException;
import software.amazon.awssdk.services.sms.model.NoConnectorsAvailableException;
import software.amazon.awssdk.services.sms.model.OperationNotPermittedException;
import software.amazon.awssdk.services.sms.model.ReplicationJobAlreadyExistsException;
import software.amazon.awssdk.services.sms.model.ReplicationJobNotFoundException;
import software.amazon.awssdk.services.sms.model.ReplicationRunLimitExceededException;
import software.amazon.awssdk.services.sms.model.ServerCannotBeReplicatedException;
import software.amazon.awssdk.services.sms.model.SmsException;
import software.amazon.awssdk.services.sms.model.StartOnDemandReplicationRunRequest;
import software.amazon.awssdk.services.sms.model.StartOnDemandReplicationRunResponse;
import software.amazon.awssdk.services.sms.model.UnauthorizedOperationException;
import software.amazon.awssdk.services.sms.model.UpdateReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.UpdateReplicationJobResponse;
import software.amazon.awssdk.services.sms.transform.CreateReplicationJobRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.CreateReplicationJobResponseUnmarshaller;
import software.amazon.awssdk.services.sms.transform.DeleteReplicationJobRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.DeleteReplicationJobResponseUnmarshaller;
import software.amazon.awssdk.services.sms.transform.DeleteServerCatalogRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.DeleteServerCatalogResponseUnmarshaller;
import software.amazon.awssdk.services.sms.transform.DisassociateConnectorRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.DisassociateConnectorResponseUnmarshaller;
import software.amazon.awssdk.services.sms.transform.GetConnectorsRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetConnectorsResponseUnmarshaller;
import software.amazon.awssdk.services.sms.transform.GetReplicationJobsRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetReplicationJobsResponseUnmarshaller;
import software.amazon.awssdk.services.sms.transform.GetReplicationRunsRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetReplicationRunsResponseUnmarshaller;
import software.amazon.awssdk.services.sms.transform.GetServersRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetServersResponseUnmarshaller;
import software.amazon.awssdk.services.sms.transform.ImportServerCatalogRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.ImportServerCatalogResponseUnmarshaller;
import software.amazon.awssdk.services.sms.transform.StartOnDemandReplicationRunRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.StartOnDemandReplicationRunResponseUnmarshaller;
import software.amazon.awssdk.services.sms.transform.UpdateReplicationJobRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.UpdateReplicationJobResponseUnmarshaller;
/**
* Internal implementation of {@link SmsClient}.
*
* @see SmsClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultSmsClient implements SmsClient {
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultSmsClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.protocolFactory = init();
this.clientConfiguration = clientConfiguration;
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
* The CreateReplicationJob API is used to create a ReplicationJob to replicate a server on AWS. Call this API to
* first create a ReplicationJob, which will then schedule periodic ReplicationRuns to replicate your server to AWS.
* Each ReplicationRun will result in the creation of an AWS AMI.
*
* @param createReplicationJobRequest
* @return Result of the CreateReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A parameter specified in the request is not valid, is unsupported, or cannot be used.
* @throws MissingRequiredParameterException
* The request is missing a required parameter. Ensure that you have supplied all the required parameters
* for the request.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws OperationNotPermittedException
* The specified operation is not allowed. This error can occur for a number of reasons; for example, you
* might be trying to start a Replication Run before seed Replication Run.
* @throws ServerCannotBeReplicatedException
* The provided server cannot be replicated.
* @throws ReplicationJobAlreadyExistsException
* An active Replication Job already exists for the specified server.
* @throws NoConnectorsAvailableException
* No connectors are available to handle this request. Please associate connector(s) and verify any existing
* connectors are healthy and can respond to requests.
* @throws InternalErrorException
* An internal error has occured.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.CreateReplicationJob
* @see AWS API
* Documentation
*/
@Override
public CreateReplicationJobResponse createReplicationJob(CreateReplicationJobRequest createReplicationJobRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
OperationNotPermittedException, ServerCannotBeReplicatedException, ReplicationJobAlreadyExistsException,
NoConnectorsAvailableException, InternalErrorException, AwsServiceException, SdkClientException, SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateReplicationJobResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createReplicationJobRequest)
.withMarshaller(new CreateReplicationJobRequestMarshaller(protocolFactory)));
}
/**
* The DeleteReplicationJob API is used to delete a ReplicationJob, resulting in no further ReplicationRuns. This
* will delete the contents of the S3 bucket used to store SMS artifacts, but will not delete any AMIs created by
* the SMS service.
*
* @param deleteReplicationJobRequest
* @return Result of the DeleteReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A parameter specified in the request is not valid, is unsupported, or cannot be used.
* @throws MissingRequiredParameterException
* The request is missing a required parameter. Ensure that you have supplied all the required parameters
* for the request.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws OperationNotPermittedException
* The specified operation is not allowed. This error can occur for a number of reasons; for example, you
* might be trying to start a Replication Run before seed Replication Run.
* @throws ReplicationJobNotFoundException
* The specified Replication Job cannot be found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteReplicationJob
* @see AWS API
* Documentation
*/
@Override
public DeleteReplicationJobResponse deleteReplicationJob(DeleteReplicationJobRequest deleteReplicationJobRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
OperationNotPermittedException, ReplicationJobNotFoundException, AwsServiceException, SdkClientException,
SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteReplicationJobResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteReplicationJobRequest)
.withMarshaller(new DeleteReplicationJobRequestMarshaller(protocolFactory)));
}
/**
* The DeleteServerCatalog API clears all servers from your server catalog. This means that these servers will no
* longer be accessible to the Server Migration Service.
*
* @param deleteServerCatalogRequest
* @return Result of the DeleteServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws OperationNotPermittedException
* The specified operation is not allowed. This error can occur for a number of reasons; for example, you
* might be trying to start a Replication Run before seed Replication Run.
* @throws InvalidParameterException
* A parameter specified in the request is not valid, is unsupported, or cannot be used.
* @throws MissingRequiredParameterException
* The request is missing a required parameter. Ensure that you have supplied all the required parameters
* for the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteServerCatalog
* @see AWS API
* Documentation
*/
@Override
public DeleteServerCatalogResponse deleteServerCatalog(DeleteServerCatalogRequest deleteServerCatalogRequest)
throws UnauthorizedOperationException, OperationNotPermittedException, InvalidParameterException,
MissingRequiredParameterException, AwsServiceException, SdkClientException, SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteServerCatalogResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteServerCatalogRequest).withMarshaller(new DeleteServerCatalogRequestMarshaller(protocolFactory)));
}
/**
* The DisassociateConnector API will disassociate a connector from the Server Migration Service, rendering it
* unavailable to support replication jobs.
*
* @param disassociateConnectorRequest
* @return Result of the DisassociateConnector operation returned by the service.
* @throws MissingRequiredParameterException
* The request is missing a required parameter. Ensure that you have supplied all the required parameters
* for the request.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws OperationNotPermittedException
* The specified operation is not allowed. This error can occur for a number of reasons; for example, you
* might be trying to start a Replication Run before seed Replication Run.
* @throws InvalidParameterException
* A parameter specified in the request is not valid, is unsupported, or cannot be used.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DisassociateConnector
* @see AWS API
* Documentation
*/
@Override
public DisassociateConnectorResponse disassociateConnector(DisassociateConnectorRequest disassociateConnectorRequest)
throws MissingRequiredParameterException, UnauthorizedOperationException, OperationNotPermittedException,
InvalidParameterException, AwsServiceException, SdkClientException, SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateConnectorResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(disassociateConnectorRequest)
.withMarshaller(new DisassociateConnectorRequestMarshaller(protocolFactory)));
}
/**
* The GetConnectors API returns a list of connectors that are registered with the Server Migration Service.
*
* @param getConnectorsRequest
* @return Result of the GetConnectors operation returned by the service.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetConnectors
* @see AWS API
* Documentation
*/
@Override
public GetConnectorsResponse getConnectors(GetConnectorsRequest getConnectorsRequest) throws UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetConnectorsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getConnectorsRequest).withMarshaller(new GetConnectorsRequestMarshaller(protocolFactory)));
}
/**
* The GetReplicationJobs API will return all of your ReplicationJobs and their details. This API returns a
* paginated list, that may be consecutively called with nextToken to retrieve all ReplicationJobs.
*
* @param getReplicationJobsRequest
* @return Result of the GetReplicationJobs operation returned by the service.
* @throws InvalidParameterException
* A parameter specified in the request is not valid, is unsupported, or cannot be used.
* @throws MissingRequiredParameterException
* The request is missing a required parameter. Ensure that you have supplied all the required parameters
* for the request.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationJobs
* @see AWS API
* Documentation
*/
@Override
public GetReplicationJobsResponse getReplicationJobs(GetReplicationJobsRequest getReplicationJobsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetReplicationJobsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getReplicationJobsRequest).withMarshaller(new GetReplicationJobsRequestMarshaller(protocolFactory)));
}
/**
* The GetReplicationRuns API will return all ReplicationRuns for a given ReplicationJob. This API returns a
* paginated list, that may be consecutively called with nextToken to retrieve all ReplicationRuns for a
* ReplicationJob.
*
* @param getReplicationRunsRequest
* @return Result of the GetReplicationRuns operation returned by the service.
* @throws InvalidParameterException
* A parameter specified in the request is not valid, is unsupported, or cannot be used.
* @throws MissingRequiredParameterException
* The request is missing a required parameter. Ensure that you have supplied all the required parameters
* for the request.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationRuns
* @see AWS API
* Documentation
*/
@Override
public GetReplicationRunsResponse getReplicationRuns(GetReplicationRunsRequest getReplicationRunsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetReplicationRunsResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getReplicationRunsRequest).withMarshaller(new GetReplicationRunsRequestMarshaller(protocolFactory)));
}
/**
* The GetServers API returns a list of all servers in your server catalog. For this call to succeed, you must
* previously have called ImportServerCatalog.
*
* @param getServersRequest
* @return Result of the GetServers operation returned by the service.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetServers
* @see AWS API
* Documentation
*/
@Override
public GetServersResponse getServers(GetServersRequest getServersRequest) throws UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetServersResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler).withInput(getServersRequest)
.withMarshaller(new GetServersRequestMarshaller(protocolFactory)));
}
/**
* The ImportServerCatalog API is used to gather the complete list of on-premises servers on your premises. This API
* call requires connectors to be installed and monitoring all servers you would like imported. This API call
* returns immediately, but may take some time to retrieve all of the servers.
*
* @param importServerCatalogRequest
* @return Result of the ImportServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws OperationNotPermittedException
* The specified operation is not allowed. This error can occur for a number of reasons; for example, you
* might be trying to start a Replication Run before seed Replication Run.
* @throws InvalidParameterException
* A parameter specified in the request is not valid, is unsupported, or cannot be used.
* @throws MissingRequiredParameterException
* The request is missing a required parameter. Ensure that you have supplied all the required parameters
* for the request.
* @throws NoConnectorsAvailableException
* No connectors are available to handle this request. Please associate connector(s) and verify any existing
* connectors are healthy and can respond to requests.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ImportServerCatalog
* @see AWS API
* Documentation
*/
@Override
public ImportServerCatalogResponse importServerCatalog(ImportServerCatalogRequest importServerCatalogRequest)
throws UnauthorizedOperationException, OperationNotPermittedException, InvalidParameterException,
MissingRequiredParameterException, NoConnectorsAvailableException, AwsServiceException, SdkClientException,
SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ImportServerCatalogResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(importServerCatalogRequest).withMarshaller(new ImportServerCatalogRequestMarshaller(protocolFactory)));
}
/**
* The StartOnDemandReplicationRun API is used to start a ReplicationRun on demand (in addition to those that are
* scheduled based on your frequency). This ReplicationRun will start immediately. StartOnDemandReplicationRun is
* subject to limits on how many on demand ReplicationRuns you may call per 24-hour period.
*
* @param startOnDemandReplicationRunRequest
* @return Result of the StartOnDemandReplicationRun operation returned by the service.
* @throws InvalidParameterException
* A parameter specified in the request is not valid, is unsupported, or cannot be used.
* @throws MissingRequiredParameterException
* The request is missing a required parameter. Ensure that you have supplied all the required parameters
* for the request.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws OperationNotPermittedException
* The specified operation is not allowed. This error can occur for a number of reasons; for example, you
* might be trying to start a Replication Run before seed Replication Run.
* @throws ReplicationRunLimitExceededException
* This user has exceeded the maximum allowed Replication Run limit.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StartOnDemandReplicationRun
* @see AWS API Documentation
*/
@Override
public StartOnDemandReplicationRunResponse startOnDemandReplicationRun(
StartOnDemandReplicationRunRequest startOnDemandReplicationRunRequest) throws InvalidParameterException,
MissingRequiredParameterException, UnauthorizedOperationException, OperationNotPermittedException,
ReplicationRunLimitExceededException, AwsServiceException, SdkClientException, SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartOnDemandReplicationRunResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler
.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(startOnDemandReplicationRunRequest)
.withMarshaller(new StartOnDemandReplicationRunRequestMarshaller(protocolFactory)));
}
/**
* The UpdateReplicationJob API is used to change the settings of your existing ReplicationJob created using
* CreateReplicationJob. Calling this API will affect the next scheduled ReplicationRun.
*
* @param updateReplicationJobRequest
* @return Result of the UpdateReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A parameter specified in the request is not valid, is unsupported, or cannot be used.
* @throws MissingRequiredParameterException
* The request is missing a required parameter. Ensure that you have supplied all the required parameters
* for the request.
* @throws OperationNotPermittedException
* The specified operation is not allowed. This error can occur for a number of reasons; for example, you
* might be trying to start a Replication Run before seed Replication Run.
* @throws UnauthorizedOperationException
* This user does not have permissions to perform this operation.
* @throws ServerCannotBeReplicatedException
* The provided server cannot be replicated.
* @throws ReplicationJobNotFoundException
* The specified Replication Job cannot be found.
* @throws InternalErrorException
* An internal error has occured.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.UpdateReplicationJob
* @see AWS API
* Documentation
*/
@Override
public UpdateReplicationJobResponse updateReplicationJob(UpdateReplicationJobRequest updateReplicationJobRequest)
throws InvalidParameterException, MissingRequiredParameterException, OperationNotPermittedException,
UnauthorizedOperationException, ServerCannotBeReplicatedException, ReplicationJobNotFoundException,
InternalErrorException, AwsServiceException, SdkClientException, SmsException {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateReplicationJobResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(updateReplicationJobRequest)
.withMarshaller(new UpdateReplicationJobRequestMarshaller(protocolFactory)));
}
private HttpResponseHandler createErrorResponseHandler() {
return protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
}
private software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory init() {
return new AwsJsonProtocolFactory(new JsonClientMetadata()
.withSupportsCbor(false)
.withSupportsIon(false)
.withBaseServiceExceptionClass(software.amazon.awssdk.services.sms.model.SmsException.class)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ReplicationJobAlreadyExistsException").withModeledClass(
ReplicationJobAlreadyExistsException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MissingRequiredParameterException").withModeledClass(
MissingRequiredParameterException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServerCannotBeReplicatedException").withModeledClass(
ServerCannotBeReplicatedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterException").withModeledClass(
InvalidParameterException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ReplicationJobNotFoundException").withModeledClass(
ReplicationJobNotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ReplicationRunLimitExceededException").withModeledClass(
ReplicationRunLimitExceededException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("OperationNotPermittedException").withModeledClass(
OperationNotPermittedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedOperationException").withModeledClass(
UnauthorizedOperationException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalError")
.withModeledClass(InternalErrorException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NoConnectorsAvailableException").withModeledClass(
NoConnectorsAvailableException.class)), AwsJsonProtocolMetadata.builder().protocolVersion("1.1")
.protocol(AwsJsonProtocol.AWS_JSON).build());
}
@Override
public void close() {
clientHandler.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy