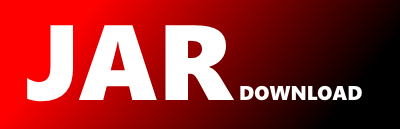
software.amazon.awssdk.services.sms.SmsAsyncClient Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sms;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.sms.model.CreateReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.CreateReplicationJobResponse;
import software.amazon.awssdk.services.sms.model.DeleteReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.DeleteReplicationJobResponse;
import software.amazon.awssdk.services.sms.model.DeleteServerCatalogRequest;
import software.amazon.awssdk.services.sms.model.DeleteServerCatalogResponse;
import software.amazon.awssdk.services.sms.model.DisassociateConnectorRequest;
import software.amazon.awssdk.services.sms.model.DisassociateConnectorResponse;
import software.amazon.awssdk.services.sms.model.GetConnectorsRequest;
import software.amazon.awssdk.services.sms.model.GetConnectorsResponse;
import software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest;
import software.amazon.awssdk.services.sms.model.GetReplicationJobsResponse;
import software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest;
import software.amazon.awssdk.services.sms.model.GetReplicationRunsResponse;
import software.amazon.awssdk.services.sms.model.GetServersRequest;
import software.amazon.awssdk.services.sms.model.GetServersResponse;
import software.amazon.awssdk.services.sms.model.ImportServerCatalogRequest;
import software.amazon.awssdk.services.sms.model.ImportServerCatalogResponse;
import software.amazon.awssdk.services.sms.model.StartOnDemandReplicationRunRequest;
import software.amazon.awssdk.services.sms.model.StartOnDemandReplicationRunResponse;
import software.amazon.awssdk.services.sms.model.UpdateReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.UpdateReplicationJobResponse;
/**
* Service client for accessing SMS asynchronously. This can be created using the static {@link #builder()} method.
*
* Amazon Server Migration Service automates the process of migrating servers to EC2.
*/
@Generated("software.amazon.awssdk:codegen")
public interface SmsAsyncClient extends SdkClient {
String SERVICE_NAME = "sms";
/**
* Create a {@link SmsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static SmsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link SmsAsyncClient}.
*/
static SmsAsyncClientBuilder builder() {
return new DefaultSmsAsyncClientBuilder();
}
/**
* The CreateReplicationJob API is used to create a ReplicationJob to replicate a server on AWS. Call this API to
* first create a ReplicationJob, which will then schedule periodic ReplicationRuns to replicate your server to AWS.
* Each ReplicationRun will result in the creation of an AWS AMI.
*
* @param createReplicationJobRequest
* @return A Java Future containing the result of the CreateReplicationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - ServerCannotBeReplicatedException The provided server cannot be replicated.
* - ReplicationJobAlreadyExistsException An active Replication Job already exists for the specified
* server.
* - NoConnectorsAvailableException No connectors are available to handle this request. Please associate
* connector(s) and verify any existing connectors are healthy and can respond to requests.
* - InternalErrorException An internal error has occured.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.CreateReplicationJob
* @see AWS API
* Documentation
*/
default CompletableFuture createReplicationJob(
CreateReplicationJobRequest createReplicationJobRequest) {
throw new UnsupportedOperationException();
}
/**
* The CreateReplicationJob API is used to create a ReplicationJob to replicate a server on AWS. Call this API to
* first create a ReplicationJob, which will then schedule periodic ReplicationRuns to replicate your server to AWS.
* Each ReplicationRun will result in the creation of an AWS AMI.
*
* This is a convenience which creates an instance of the {@link CreateReplicationJobRequest.Builder} avoiding the
* need to create one manually via {@link CreateReplicationJobRequest#builder()}
*
*
* @param createReplicationJobRequest
* A {@link Consumer} that will call methods on {@link CreateReplicationJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateReplicationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - ServerCannotBeReplicatedException The provided server cannot be replicated.
* - ReplicationJobAlreadyExistsException An active Replication Job already exists for the specified
* server.
* - NoConnectorsAvailableException No connectors are available to handle this request. Please associate
* connector(s) and verify any existing connectors are healthy and can respond to requests.
* - InternalErrorException An internal error has occured.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.CreateReplicationJob
* @see AWS API
* Documentation
*/
default CompletableFuture createReplicationJob(
Consumer createReplicationJobRequest) {
return createReplicationJob(CreateReplicationJobRequest.builder().applyMutation(createReplicationJobRequest).build());
}
/**
* The DeleteReplicationJob API is used to delete a ReplicationJob, resulting in no further ReplicationRuns. This
* will delete the contents of the S3 bucket used to store SMS artifacts, but will not delete any AMIs created by
* the SMS service.
*
* @param deleteReplicationJobRequest
* @return A Java Future containing the result of the DeleteReplicationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - ReplicationJobNotFoundException The specified Replication Job cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.DeleteReplicationJob
* @see AWS API
* Documentation
*/
default CompletableFuture deleteReplicationJob(
DeleteReplicationJobRequest deleteReplicationJobRequest) {
throw new UnsupportedOperationException();
}
/**
* The DeleteReplicationJob API is used to delete a ReplicationJob, resulting in no further ReplicationRuns. This
* will delete the contents of the S3 bucket used to store SMS artifacts, but will not delete any AMIs created by
* the SMS service.
*
* This is a convenience which creates an instance of the {@link DeleteReplicationJobRequest.Builder} avoiding the
* need to create one manually via {@link DeleteReplicationJobRequest#builder()}
*
*
* @param deleteReplicationJobRequest
* A {@link Consumer} that will call methods on {@link DeleteReplicationJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteReplicationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - ReplicationJobNotFoundException The specified Replication Job cannot be found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.DeleteReplicationJob
* @see AWS API
* Documentation
*/
default CompletableFuture deleteReplicationJob(
Consumer deleteReplicationJobRequest) {
return deleteReplicationJob(DeleteReplicationJobRequest.builder().applyMutation(deleteReplicationJobRequest).build());
}
/**
* The DeleteServerCatalog API clears all servers from your server catalog. This means that these servers will no
* longer be accessible to the Server Migration Service.
*
* @param deleteServerCatalogRequest
* @return A Java Future containing the result of the DeleteServerCatalog operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.DeleteServerCatalog
* @see AWS API
* Documentation
*/
default CompletableFuture deleteServerCatalog(
DeleteServerCatalogRequest deleteServerCatalogRequest) {
throw new UnsupportedOperationException();
}
/**
* The DeleteServerCatalog API clears all servers from your server catalog. This means that these servers will no
* longer be accessible to the Server Migration Service.
*
* This is a convenience which creates an instance of the {@link DeleteServerCatalogRequest.Builder} avoiding the
* need to create one manually via {@link DeleteServerCatalogRequest#builder()}
*
*
* @param deleteServerCatalogRequest
* A {@link Consumer} that will call methods on {@link DeleteServerCatalogRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteServerCatalog operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.DeleteServerCatalog
* @see AWS API
* Documentation
*/
default CompletableFuture deleteServerCatalog(
Consumer deleteServerCatalogRequest) {
return deleteServerCatalog(DeleteServerCatalogRequest.builder().applyMutation(deleteServerCatalogRequest).build());
}
/**
* The DeleteServerCatalog API clears all servers from your server catalog. This means that these servers will no
* longer be accessible to the Server Migration Service.
*
* @return A Java Future containing the result of the DeleteServerCatalog operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.DeleteServerCatalog
* @see AWS API
* Documentation
*/
default CompletableFuture deleteServerCatalog() {
return deleteServerCatalog(DeleteServerCatalogRequest.builder().build());
}
/**
* The DisassociateConnector API will disassociate a connector from the Server Migration Service, rendering it
* unavailable to support replication jobs.
*
* @param disassociateConnectorRequest
* @return A Java Future containing the result of the DisassociateConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.DisassociateConnector
* @see AWS API
* Documentation
*/
default CompletableFuture disassociateConnector(
DisassociateConnectorRequest disassociateConnectorRequest) {
throw new UnsupportedOperationException();
}
/**
* The DisassociateConnector API will disassociate a connector from the Server Migration Service, rendering it
* unavailable to support replication jobs.
*
* This is a convenience which creates an instance of the {@link DisassociateConnectorRequest.Builder} avoiding the
* need to create one manually via {@link DisassociateConnectorRequest#builder()}
*
*
* @param disassociateConnectorRequest
* A {@link Consumer} that will call methods on {@link DisassociateConnectorRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateConnector operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.DisassociateConnector
* @see AWS API
* Documentation
*/
default CompletableFuture disassociateConnector(
Consumer disassociateConnectorRequest) {
return disassociateConnector(DisassociateConnectorRequest.builder().applyMutation(disassociateConnectorRequest).build());
}
/**
* The GetConnectors API returns a list of connectors that are registered with the Server Migration Service.
*
* @param getConnectorsRequest
* @return A Java Future containing the result of the GetConnectors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetConnectors
* @see AWS API
* Documentation
*/
default CompletableFuture getConnectors(GetConnectorsRequest getConnectorsRequest) {
throw new UnsupportedOperationException();
}
/**
* The GetConnectors API returns a list of connectors that are registered with the Server Migration Service.
*
* This is a convenience which creates an instance of the {@link GetConnectorsRequest.Builder} avoiding the need to
* create one manually via {@link GetConnectorsRequest#builder()}
*
*
* @param getConnectorsRequest
* A {@link Consumer} that will call methods on {@link GetConnectorsRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetConnectors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetConnectors
* @see AWS API
* Documentation
*/
default CompletableFuture getConnectors(Consumer getConnectorsRequest) {
return getConnectors(GetConnectorsRequest.builder().applyMutation(getConnectorsRequest).build());
}
/**
* The GetConnectors API returns a list of connectors that are registered with the Server Migration Service.
*
* @return A Java Future containing the result of the GetConnectors operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetConnectors
* @see AWS API
* Documentation
*/
default CompletableFuture getConnectors() {
return getConnectors(GetConnectorsRequest.builder().build());
}
/**
* The GetReplicationJobs API will return all of your ReplicationJobs and their details. This API returns a
* paginated list, that may be consecutively called with nextToken to retrieve all ReplicationJobs.
*
* @param getReplicationJobsRequest
* @return A Java Future containing the result of the GetReplicationJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetReplicationJobs
* @see AWS API
* Documentation
*/
default CompletableFuture getReplicationJobs(GetReplicationJobsRequest getReplicationJobsRequest) {
throw new UnsupportedOperationException();
}
/**
* The GetReplicationJobs API will return all of your ReplicationJobs and their details. This API returns a
* paginated list, that may be consecutively called with nextToken to retrieve all ReplicationJobs.
*
* This is a convenience which creates an instance of the {@link GetReplicationJobsRequest.Builder} avoiding the
* need to create one manually via {@link GetReplicationJobsRequest#builder()}
*
*
* @param getReplicationJobsRequest
* A {@link Consumer} that will call methods on {@link GetReplicationJobsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetReplicationJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetReplicationJobs
* @see AWS API
* Documentation
*/
default CompletableFuture getReplicationJobs(
Consumer getReplicationJobsRequest) {
return getReplicationJobs(GetReplicationJobsRequest.builder().applyMutation(getReplicationJobsRequest).build());
}
/**
* The GetReplicationJobs API will return all of your ReplicationJobs and their details. This API returns a
* paginated list, that may be consecutively called with nextToken to retrieve all ReplicationJobs.
*
* @return A Java Future containing the result of the GetReplicationJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetReplicationJobs
* @see AWS API
* Documentation
*/
default CompletableFuture getReplicationJobs() {
return getReplicationJobs(GetReplicationJobsRequest.builder().build());
}
/**
* The GetReplicationRuns API will return all ReplicationRuns for a given ReplicationJob. This API returns a
* paginated list, that may be consecutively called with nextToken to retrieve all ReplicationRuns for a
* ReplicationJob.
*
* @param getReplicationRunsRequest
* @return A Java Future containing the result of the GetReplicationRuns operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetReplicationRuns
* @see AWS API
* Documentation
*/
default CompletableFuture getReplicationRuns(GetReplicationRunsRequest getReplicationRunsRequest) {
throw new UnsupportedOperationException();
}
/**
* The GetReplicationRuns API will return all ReplicationRuns for a given ReplicationJob. This API returns a
* paginated list, that may be consecutively called with nextToken to retrieve all ReplicationRuns for a
* ReplicationJob.
*
* This is a convenience which creates an instance of the {@link GetReplicationRunsRequest.Builder} avoiding the
* need to create one manually via {@link GetReplicationRunsRequest#builder()}
*
*
* @param getReplicationRunsRequest
* A {@link Consumer} that will call methods on {@link GetReplicationRunsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetReplicationRuns operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetReplicationRuns
* @see AWS API
* Documentation
*/
default CompletableFuture getReplicationRuns(
Consumer getReplicationRunsRequest) {
return getReplicationRuns(GetReplicationRunsRequest.builder().applyMutation(getReplicationRunsRequest).build());
}
/**
* The GetServers API returns a list of all servers in your server catalog. For this call to succeed, you must
* previously have called ImportServerCatalog.
*
* @param getServersRequest
* @return A Java Future containing the result of the GetServers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetServers
* @see AWS API
* Documentation
*/
default CompletableFuture getServers(GetServersRequest getServersRequest) {
throw new UnsupportedOperationException();
}
/**
* The GetServers API returns a list of all servers in your server catalog. For this call to succeed, you must
* previously have called ImportServerCatalog.
*
* This is a convenience which creates an instance of the {@link GetServersRequest.Builder} avoiding the need to
* create one manually via {@link GetServersRequest#builder()}
*
*
* @param getServersRequest
* A {@link Consumer} that will call methods on {@link GetServersRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetServers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetServers
* @see AWS API
* Documentation
*/
default CompletableFuture getServers(Consumer getServersRequest) {
return getServers(GetServersRequest.builder().applyMutation(getServersRequest).build());
}
/**
* The GetServers API returns a list of all servers in your server catalog. For this call to succeed, you must
* previously have called ImportServerCatalog.
*
* @return A Java Future containing the result of the GetServers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.GetServers
* @see AWS API
* Documentation
*/
default CompletableFuture getServers() {
return getServers(GetServersRequest.builder().build());
}
/**
* The ImportServerCatalog API is used to gather the complete list of on-premises servers on your premises. This API
* call requires connectors to be installed and monitoring all servers you would like imported. This API call
* returns immediately, but may take some time to retrieve all of the servers.
*
* @param importServerCatalogRequest
* @return A Java Future containing the result of the ImportServerCatalog operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - NoConnectorsAvailableException No connectors are available to handle this request. Please associate
* connector(s) and verify any existing connectors are healthy and can respond to requests.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.ImportServerCatalog
* @see AWS API
* Documentation
*/
default CompletableFuture importServerCatalog(
ImportServerCatalogRequest importServerCatalogRequest) {
throw new UnsupportedOperationException();
}
/**
* The ImportServerCatalog API is used to gather the complete list of on-premises servers on your premises. This API
* call requires connectors to be installed and monitoring all servers you would like imported. This API call
* returns immediately, but may take some time to retrieve all of the servers.
*
* This is a convenience which creates an instance of the {@link ImportServerCatalogRequest.Builder} avoiding the
* need to create one manually via {@link ImportServerCatalogRequest#builder()}
*
*
* @param importServerCatalogRequest
* A {@link Consumer} that will call methods on {@link ImportServerCatalogRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ImportServerCatalog operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - NoConnectorsAvailableException No connectors are available to handle this request. Please associate
* connector(s) and verify any existing connectors are healthy and can respond to requests.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.ImportServerCatalog
* @see AWS API
* Documentation
*/
default CompletableFuture importServerCatalog(
Consumer importServerCatalogRequest) {
return importServerCatalog(ImportServerCatalogRequest.builder().applyMutation(importServerCatalogRequest).build());
}
/**
* The ImportServerCatalog API is used to gather the complete list of on-premises servers on your premises. This API
* call requires connectors to be installed and monitoring all servers you would like imported. This API call
* returns immediately, but may take some time to retrieve all of the servers.
*
* @return A Java Future containing the result of the ImportServerCatalog operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - NoConnectorsAvailableException No connectors are available to handle this request. Please associate
* connector(s) and verify any existing connectors are healthy and can respond to requests.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.ImportServerCatalog
* @see AWS API
* Documentation
*/
default CompletableFuture importServerCatalog() {
return importServerCatalog(ImportServerCatalogRequest.builder().build());
}
/**
* The StartOnDemandReplicationRun API is used to start a ReplicationRun on demand (in addition to those that are
* scheduled based on your frequency). This ReplicationRun will start immediately. StartOnDemandReplicationRun is
* subject to limits on how many on demand ReplicationRuns you may call per 24-hour period.
*
* @param startOnDemandReplicationRunRequest
* @return A Java Future containing the result of the StartOnDemandReplicationRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - ReplicationRunLimitExceededException This user has exceeded the maximum allowed Replication Run
* limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.StartOnDemandReplicationRun
* @see AWS API Documentation
*/
default CompletableFuture startOnDemandReplicationRun(
StartOnDemandReplicationRunRequest startOnDemandReplicationRunRequest) {
throw new UnsupportedOperationException();
}
/**
* The StartOnDemandReplicationRun API is used to start a ReplicationRun on demand (in addition to those that are
* scheduled based on your frequency). This ReplicationRun will start immediately. StartOnDemandReplicationRun is
* subject to limits on how many on demand ReplicationRuns you may call per 24-hour period.
*
* This is a convenience which creates an instance of the {@link StartOnDemandReplicationRunRequest.Builder}
* avoiding the need to create one manually via {@link StartOnDemandReplicationRunRequest#builder()}
*
*
* @param startOnDemandReplicationRunRequest
* A {@link Consumer} that will call methods on {@link StartOnDemandReplicationRunRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the StartOnDemandReplicationRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - ReplicationRunLimitExceededException This user has exceeded the maximum allowed Replication Run
* limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.StartOnDemandReplicationRun
* @see AWS API Documentation
*/
default CompletableFuture startOnDemandReplicationRun(
Consumer startOnDemandReplicationRunRequest) {
return startOnDemandReplicationRun(StartOnDemandReplicationRunRequest.builder()
.applyMutation(startOnDemandReplicationRunRequest).build());
}
/**
* The UpdateReplicationJob API is used to change the settings of your existing ReplicationJob created using
* CreateReplicationJob. Calling this API will affect the next scheduled ReplicationRun.
*
* @param updateReplicationJobRequest
* @return A Java Future containing the result of the UpdateReplicationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - ServerCannotBeReplicatedException The provided server cannot be replicated.
* - ReplicationJobNotFoundException The specified Replication Job cannot be found.
* - InternalErrorException An internal error has occured.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.UpdateReplicationJob
* @see AWS API
* Documentation
*/
default CompletableFuture updateReplicationJob(
UpdateReplicationJobRequest updateReplicationJobRequest) {
throw new UnsupportedOperationException();
}
/**
* The UpdateReplicationJob API is used to change the settings of your existing ReplicationJob created using
* CreateReplicationJob. Calling this API will affect the next scheduled ReplicationRun.
*
* This is a convenience which creates an instance of the {@link UpdateReplicationJobRequest.Builder} avoiding the
* need to create one manually via {@link UpdateReplicationJobRequest#builder()}
*
*
* @param updateReplicationJobRequest
* A {@link Consumer} that will call methods on {@link UpdateReplicationJobRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateReplicationJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidParameterException A parameter specified in the request is not valid, is unsupported, or
* cannot be used.
* - MissingRequiredParameterException The request is missing a required parameter. Ensure that you have
* supplied all the required parameters for the request.
* - OperationNotPermittedException The specified operation is not allowed. This error can occur for a
* number of reasons; for example, you might be trying to start a Replication Run before seed Replication
* Run.
* - UnauthorizedOperationException This user does not have permissions to perform this operation.
* - ServerCannotBeReplicatedException The provided server cannot be replicated.
* - ReplicationJobNotFoundException The specified Replication Job cannot be found.
* - InternalErrorException An internal error has occured.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SmsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SmsAsyncClient.UpdateReplicationJob
* @see AWS API
* Documentation
*/
default CompletableFuture updateReplicationJob(
Consumer updateReplicationJobRequest) {
return updateReplicationJob(UpdateReplicationJobRequest.builder().applyMutation(updateReplicationJobRequest).build());
}
}