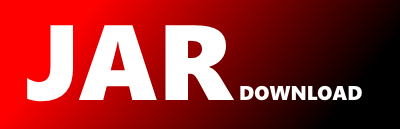
software.amazon.awssdk.services.sms.model.GetReplicationRunsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of servermigration Show documentation
Show all versions of servermigration Show documentation
The AWS Java SDK for AWS Server Migration module holds the client classes that are used for
communicating with AWS Server Migration Service
The newest version!
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sms.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetReplicationRunsResponse extends SmsResponse implements
ToCopyableBuilder {
private final ReplicationJob replicationJob;
private final List replicationRunList;
private final String nextToken;
private GetReplicationRunsResponse(BuilderImpl builder) {
super(builder);
this.replicationJob = builder.replicationJob;
this.replicationRunList = builder.replicationRunList;
this.nextToken = builder.nextToken;
}
/**
* Returns the value of the ReplicationJob property for this object.
*
* @return The value of the ReplicationJob property for this object.
*/
public ReplicationJob replicationJob() {
return replicationJob;
}
/**
* Returns the value of the ReplicationRunList property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The value of the ReplicationRunList property for this object.
*/
public List replicationRunList() {
return replicationRunList;
}
/**
* Returns the value of the NextToken property for this object.
*
* @return The value of the NextToken property for this object.
*/
public String nextToken() {
return nextToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(replicationJob());
hashCode = 31 * hashCode + Objects.hashCode(replicationRunList());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetReplicationRunsResponse)) {
return false;
}
GetReplicationRunsResponse other = (GetReplicationRunsResponse) obj;
return Objects.equals(replicationJob(), other.replicationJob())
&& Objects.equals(replicationRunList(), other.replicationRunList())
&& Objects.equals(nextToken(), other.nextToken());
}
@Override
public String toString() {
return ToString.builder("GetReplicationRunsResponse").add("ReplicationJob", replicationJob())
.add("ReplicationRunList", replicationRunList()).add("NextToken", nextToken()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "replicationJob":
return Optional.ofNullable(clazz.cast(replicationJob()));
case "replicationRunList":
return Optional.ofNullable(clazz.cast(replicationRunList()));
case "nextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
default:
return Optional.empty();
}
}
public interface Builder extends SmsResponse.Builder, CopyableBuilder {
/**
* Sets the value of the ReplicationJob property for this object.
*
* @param replicationJob
* The new value for the ReplicationJob property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationJob(ReplicationJob replicationJob);
/**
* Sets the value of the ReplicationJob property for this object.
*
* This is a convenience that creates an instance of the {@link ReplicationJob.Builder} avoiding the need to
* create one manually via {@link ReplicationJob#builder()}.
*
* When the {@link Consumer} completes, {@link ReplicationJob.Builder#build()} is called immediately and its
* result is passed to {@link #replicationJob(ReplicationJob)}.
*
* @param replicationJob
* a consumer that will call methods on {@link ReplicationJob.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #replicationJob(ReplicationJob)
*/
default Builder replicationJob(Consumer replicationJob) {
return replicationJob(ReplicationJob.builder().applyMutation(replicationJob).build());
}
/**
* Sets the value of the ReplicationRunList property for this object.
*
* @param replicationRunList
* The new value for the ReplicationRunList property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationRunList(Collection replicationRunList);
/**
* Sets the value of the ReplicationRunList property for this object.
*
* @param replicationRunList
* The new value for the ReplicationRunList property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationRunList(ReplicationRun... replicationRunList);
/**
* Sets the value of the ReplicationRunList property for this object.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need
* to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and
* its result is passed to {@link #replicationRunList(List)}.
*
* @param replicationRunList
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #replicationRunList(List)
*/
Builder replicationRunList(Consumer... replicationRunList);
/**
* Sets the value of the NextToken property for this object.
*
* @param nextToken
* The new value for the NextToken property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nextToken(String nextToken);
}
static final class BuilderImpl extends SmsResponse.BuilderImpl implements Builder {
private ReplicationJob replicationJob;
private List replicationRunList = DefaultSdkAutoConstructList.getInstance();
private String nextToken;
private BuilderImpl() {
}
private BuilderImpl(GetReplicationRunsResponse model) {
super(model);
replicationJob(model.replicationJob);
replicationRunList(model.replicationRunList);
nextToken(model.nextToken);
}
public final ReplicationJob.Builder getReplicationJob() {
return replicationJob != null ? replicationJob.toBuilder() : null;
}
@Override
public final Builder replicationJob(ReplicationJob replicationJob) {
this.replicationJob = replicationJob;
return this;
}
public final void setReplicationJob(ReplicationJob.BuilderImpl replicationJob) {
this.replicationJob = replicationJob != null ? replicationJob.build() : null;
}
public final Collection getReplicationRunList() {
return replicationRunList != null ? replicationRunList.stream().map(ReplicationRun::toBuilder)
.collect(Collectors.toList()) : null;
}
@Override
public final Builder replicationRunList(Collection replicationRunList) {
this.replicationRunList = ReplicationRunListCopier.copy(replicationRunList);
return this;
}
@Override
@SafeVarargs
public final Builder replicationRunList(ReplicationRun... replicationRunList) {
replicationRunList(Arrays.asList(replicationRunList));
return this;
}
@Override
@SafeVarargs
public final Builder replicationRunList(Consumer... replicationRunList) {
replicationRunList(Stream.of(replicationRunList).map(c -> ReplicationRun.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setReplicationRunList(Collection replicationRunList) {
this.replicationRunList = ReplicationRunListCopier.copyFromBuilder(replicationRunList);
}
public final String getNextToken() {
return nextToken;
}
@Override
public final Builder nextToken(String nextToken) {
this.nextToken = nextToken;
return this;
}
public final void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
@Override
public GetReplicationRunsResponse build() {
return new GetReplicationRunsResponse(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy