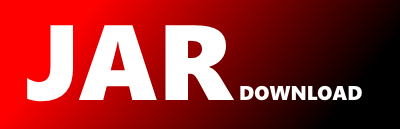
software.amazon.awssdk.services.sms.model.GetServersResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of servermigration Show documentation
Show all versions of servermigration Show documentation
The AWS Java SDK for AWS Server Migration module holds the client classes that are used for
communicating with AWS Server Migration Service
The newest version!
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sms.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetServersResponse extends SmsResponse implements
ToCopyableBuilder {
private final Instant lastModifiedOn;
private final String serverCatalogStatus;
private final List serverList;
private final String nextToken;
private GetServersResponse(BuilderImpl builder) {
super(builder);
this.lastModifiedOn = builder.lastModifiedOn;
this.serverCatalogStatus = builder.serverCatalogStatus;
this.serverList = builder.serverList;
this.nextToken = builder.nextToken;
}
/**
* Returns the value of the LastModifiedOn property for this object.
*
* @return The value of the LastModifiedOn property for this object.
*/
public Instant lastModifiedOn() {
return lastModifiedOn;
}
/**
* Returns the value of the ServerCatalogStatus property for this object.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serverCatalogStatus} will return {@link ServerCatalogStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serverCatalogStatusAsString}.
*
*
* @return The value of the ServerCatalogStatus property for this object.
* @see ServerCatalogStatus
*/
public ServerCatalogStatus serverCatalogStatus() {
return ServerCatalogStatus.fromValue(serverCatalogStatus);
}
/**
* Returns the value of the ServerCatalogStatus property for this object.
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #serverCatalogStatus} will return {@link ServerCatalogStatus#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #serverCatalogStatusAsString}.
*
*
* @return The value of the ServerCatalogStatus property for this object.
* @see ServerCatalogStatus
*/
public String serverCatalogStatusAsString() {
return serverCatalogStatus;
}
/**
* Returns the value of the ServerList property for this object.
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return The value of the ServerList property for this object.
*/
public List serverList() {
return serverList;
}
/**
* Returns the value of the NextToken property for this object.
*
* @return The value of the NextToken property for this object.
*/
public String nextToken() {
return nextToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedOn());
hashCode = 31 * hashCode + Objects.hashCode(serverCatalogStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(serverList());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetServersResponse)) {
return false;
}
GetServersResponse other = (GetServersResponse) obj;
return Objects.equals(lastModifiedOn(), other.lastModifiedOn())
&& Objects.equals(serverCatalogStatusAsString(), other.serverCatalogStatusAsString())
&& Objects.equals(serverList(), other.serverList()) && Objects.equals(nextToken(), other.nextToken());
}
@Override
public String toString() {
return ToString.builder("GetServersResponse").add("LastModifiedOn", lastModifiedOn())
.add("ServerCatalogStatus", serverCatalogStatusAsString()).add("ServerList", serverList())
.add("NextToken", nextToken()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "lastModifiedOn":
return Optional.ofNullable(clazz.cast(lastModifiedOn()));
case "serverCatalogStatus":
return Optional.ofNullable(clazz.cast(serverCatalogStatusAsString()));
case "serverList":
return Optional.ofNullable(clazz.cast(serverList()));
case "nextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
default:
return Optional.empty();
}
}
public interface Builder extends SmsResponse.Builder, CopyableBuilder {
/**
* Sets the value of the LastModifiedOn property for this object.
*
* @param lastModifiedOn
* The new value for the LastModifiedOn property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder lastModifiedOn(Instant lastModifiedOn);
/**
* Sets the value of the ServerCatalogStatus property for this object.
*
* @param serverCatalogStatus
* The new value for the ServerCatalogStatus property for this object.
* @see ServerCatalogStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServerCatalogStatus
*/
Builder serverCatalogStatus(String serverCatalogStatus);
/**
* Sets the value of the ServerCatalogStatus property for this object.
*
* @param serverCatalogStatus
* The new value for the ServerCatalogStatus property for this object.
* @see ServerCatalogStatus
* @return Returns a reference to this object so that method calls can be chained together.
* @see ServerCatalogStatus
*/
Builder serverCatalogStatus(ServerCatalogStatus serverCatalogStatus);
/**
* Sets the value of the ServerList property for this object.
*
* @param serverList
* The new value for the ServerList property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder serverList(Collection serverList);
/**
* Sets the value of the ServerList property for this object.
*
* @param serverList
* The new value for the ServerList property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder serverList(Server... serverList);
/**
* Sets the value of the ServerList property for this object.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to
* create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its
* result is passed to {@link #serverList(List)}.
*
* @param serverList
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #serverList(List)
*/
Builder serverList(Consumer... serverList);
/**
* Sets the value of the NextToken property for this object.
*
* @param nextToken
* The new value for the NextToken property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nextToken(String nextToken);
}
static final class BuilderImpl extends SmsResponse.BuilderImpl implements Builder {
private Instant lastModifiedOn;
private String serverCatalogStatus;
private List serverList = DefaultSdkAutoConstructList.getInstance();
private String nextToken;
private BuilderImpl() {
}
private BuilderImpl(GetServersResponse model) {
super(model);
lastModifiedOn(model.lastModifiedOn);
serverCatalogStatus(model.serverCatalogStatus);
serverList(model.serverList);
nextToken(model.nextToken);
}
public final Instant getLastModifiedOn() {
return lastModifiedOn;
}
@Override
public final Builder lastModifiedOn(Instant lastModifiedOn) {
this.lastModifiedOn = lastModifiedOn;
return this;
}
public final void setLastModifiedOn(Instant lastModifiedOn) {
this.lastModifiedOn = lastModifiedOn;
}
public final String getServerCatalogStatus() {
return serverCatalogStatus;
}
@Override
public final Builder serverCatalogStatus(String serverCatalogStatus) {
this.serverCatalogStatus = serverCatalogStatus;
return this;
}
@Override
public final Builder serverCatalogStatus(ServerCatalogStatus serverCatalogStatus) {
this.serverCatalogStatus(serverCatalogStatus.toString());
return this;
}
public final void setServerCatalogStatus(String serverCatalogStatus) {
this.serverCatalogStatus = serverCatalogStatus;
}
public final Collection getServerList() {
return serverList != null ? serverList.stream().map(Server::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder serverList(Collection serverList) {
this.serverList = ServerListCopier.copy(serverList);
return this;
}
@Override
@SafeVarargs
public final Builder serverList(Server... serverList) {
serverList(Arrays.asList(serverList));
return this;
}
@Override
@SafeVarargs
public final Builder serverList(Consumer... serverList) {
serverList(Stream.of(serverList).map(c -> Server.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setServerList(Collection serverList) {
this.serverList = ServerListCopier.copyFromBuilder(serverList);
}
public final String getNextToken() {
return nextToken;
}
@Override
public final Builder nextToken(String nextToken) {
this.nextToken = nextToken;
return this;
}
public final void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
@Override
public GetServersResponse build() {
return new GetServersResponse(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy