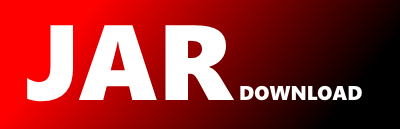
software.amazon.awssdk.services.sms.model.ReplicationRun Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sms.model;
import java.time.Instant;
import java.util.Objects;
import java.util.Optional;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.protocol.ProtocolMarshaller;
import software.amazon.awssdk.core.protocol.StructuredPojo;
import software.amazon.awssdk.services.sms.transform.ReplicationRunMarshaller;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
* Object representing a Replication Run
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReplicationRun implements StructuredPojo, ToCopyableBuilder {
private final String replicationRunId;
private final String state;
private final String type;
private final String statusMessage;
private final String amiId;
private final Instant scheduledStartTime;
private final Instant completedTime;
private final String description;
private ReplicationRun(BuilderImpl builder) {
this.replicationRunId = builder.replicationRunId;
this.state = builder.state;
this.type = builder.type;
this.statusMessage = builder.statusMessage;
this.amiId = builder.amiId;
this.scheduledStartTime = builder.scheduledStartTime;
this.completedTime = builder.completedTime;
this.description = builder.description;
}
/**
* Returns the value of the ReplicationRunId property for this object.
*
* @return The value of the ReplicationRunId property for this object.
*/
public String replicationRunId() {
return replicationRunId;
}
/**
* Returns the value of the State property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link ReplicationRunState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The value of the State property for this object.
* @see ReplicationRunState
*/
public ReplicationRunState state() {
return ReplicationRunState.fromValue(state);
}
/**
* Returns the value of the State property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #state} will return
* {@link ReplicationRunState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #stateAsString}.
*
*
* @return The value of the State property for this object.
* @see ReplicationRunState
*/
public String stateAsString() {
return state;
}
/**
* Returns the value of the Type property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ReplicationRunType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The value of the Type property for this object.
* @see ReplicationRunType
*/
public ReplicationRunType type() {
return ReplicationRunType.fromValue(type);
}
/**
* Returns the value of the Type property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ReplicationRunType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The value of the Type property for this object.
* @see ReplicationRunType
*/
public String typeAsString() {
return type;
}
/**
* Returns the value of the StatusMessage property for this object.
*
* @return The value of the StatusMessage property for this object.
*/
public String statusMessage() {
return statusMessage;
}
/**
* Returns the value of the AmiId property for this object.
*
* @return The value of the AmiId property for this object.
*/
public String amiId() {
return amiId;
}
/**
* Returns the value of the ScheduledStartTime property for this object.
*
* @return The value of the ScheduledStartTime property for this object.
*/
public Instant scheduledStartTime() {
return scheduledStartTime;
}
/**
* Returns the value of the CompletedTime property for this object.
*
* @return The value of the CompletedTime property for this object.
*/
public Instant completedTime() {
return completedTime;
}
/**
* Returns the value of the Description property for this object.
*
* @return The value of the Description property for this object.
*/
public String description() {
return description;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(replicationRunId());
hashCode = 31 * hashCode + Objects.hashCode(stateAsString());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusMessage());
hashCode = 31 * hashCode + Objects.hashCode(amiId());
hashCode = 31 * hashCode + Objects.hashCode(scheduledStartTime());
hashCode = 31 * hashCode + Objects.hashCode(completedTime());
hashCode = 31 * hashCode + Objects.hashCode(description());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReplicationRun)) {
return false;
}
ReplicationRun other = (ReplicationRun) obj;
return Objects.equals(replicationRunId(), other.replicationRunId())
&& Objects.equals(stateAsString(), other.stateAsString()) && Objects.equals(typeAsString(), other.typeAsString())
&& Objects.equals(statusMessage(), other.statusMessage()) && Objects.equals(amiId(), other.amiId())
&& Objects.equals(scheduledStartTime(), other.scheduledStartTime())
&& Objects.equals(completedTime(), other.completedTime()) && Objects.equals(description(), other.description());
}
@Override
public String toString() {
return ToString.builder("ReplicationRun").add("ReplicationRunId", replicationRunId()).add("State", stateAsString())
.add("Type", typeAsString()).add("StatusMessage", statusMessage()).add("AmiId", amiId())
.add("ScheduledStartTime", scheduledStartTime()).add("CompletedTime", completedTime())
.add("Description", description()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "replicationRunId":
return Optional.ofNullable(clazz.cast(replicationRunId()));
case "state":
return Optional.ofNullable(clazz.cast(stateAsString()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "statusMessage":
return Optional.ofNullable(clazz.cast(statusMessage()));
case "amiId":
return Optional.ofNullable(clazz.cast(amiId()));
case "scheduledStartTime":
return Optional.ofNullable(clazz.cast(scheduledStartTime()));
case "completedTime":
return Optional.ofNullable(clazz.cast(completedTime()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
default:
return Optional.empty();
}
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
ReplicationRunMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
* Sets the value of the ReplicationRunId property for this object.
*
* @param replicationRunId
* The new value for the ReplicationRunId property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationRunId(String replicationRunId);
/**
* Sets the value of the State property for this object.
*
* @param state
* The new value for the State property for this object.
* @see ReplicationRunState
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReplicationRunState
*/
Builder state(String state);
/**
* Sets the value of the State property for this object.
*
* @param state
* The new value for the State property for this object.
* @see ReplicationRunState
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReplicationRunState
*/
Builder state(ReplicationRunState state);
/**
* Sets the value of the Type property for this object.
*
* @param type
* The new value for the Type property for this object.
* @see ReplicationRunType
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReplicationRunType
*/
Builder type(String type);
/**
* Sets the value of the Type property for this object.
*
* @param type
* The new value for the Type property for this object.
* @see ReplicationRunType
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReplicationRunType
*/
Builder type(ReplicationRunType type);
/**
* Sets the value of the StatusMessage property for this object.
*
* @param statusMessage
* The new value for the StatusMessage property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder statusMessage(String statusMessage);
/**
* Sets the value of the AmiId property for this object.
*
* @param amiId
* The new value for the AmiId property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder amiId(String amiId);
/**
* Sets the value of the ScheduledStartTime property for this object.
*
* @param scheduledStartTime
* The new value for the ScheduledStartTime property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder scheduledStartTime(Instant scheduledStartTime);
/**
* Sets the value of the CompletedTime property for this object.
*
* @param completedTime
* The new value for the CompletedTime property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder completedTime(Instant completedTime);
/**
* Sets the value of the Description property for this object.
*
* @param description
* The new value for the Description property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
}
static final class BuilderImpl implements Builder {
private String replicationRunId;
private String state;
private String type;
private String statusMessage;
private String amiId;
private Instant scheduledStartTime;
private Instant completedTime;
private String description;
private BuilderImpl() {
}
private BuilderImpl(ReplicationRun model) {
replicationRunId(model.replicationRunId);
state(model.state);
type(model.type);
statusMessage(model.statusMessage);
amiId(model.amiId);
scheduledStartTime(model.scheduledStartTime);
completedTime(model.completedTime);
description(model.description);
}
public final String getReplicationRunId() {
return replicationRunId;
}
@Override
public final Builder replicationRunId(String replicationRunId) {
this.replicationRunId = replicationRunId;
return this;
}
public final void setReplicationRunId(String replicationRunId) {
this.replicationRunId = replicationRunId;
}
public final String getState() {
return state;
}
@Override
public final Builder state(String state) {
this.state = state;
return this;
}
@Override
public final Builder state(ReplicationRunState state) {
this.state(state.toString());
return this;
}
public final void setState(String state) {
this.state = state;
}
public final String getType() {
return type;
}
@Override
public final Builder type(String type) {
this.type = type;
return this;
}
@Override
public final Builder type(ReplicationRunType type) {
this.type(type.toString());
return this;
}
public final void setType(String type) {
this.type = type;
}
public final String getStatusMessage() {
return statusMessage;
}
@Override
public final Builder statusMessage(String statusMessage) {
this.statusMessage = statusMessage;
return this;
}
public final void setStatusMessage(String statusMessage) {
this.statusMessage = statusMessage;
}
public final String getAmiId() {
return amiId;
}
@Override
public final Builder amiId(String amiId) {
this.amiId = amiId;
return this;
}
public final void setAmiId(String amiId) {
this.amiId = amiId;
}
public final Instant getScheduledStartTime() {
return scheduledStartTime;
}
@Override
public final Builder scheduledStartTime(Instant scheduledStartTime) {
this.scheduledStartTime = scheduledStartTime;
return this;
}
public final void setScheduledStartTime(Instant scheduledStartTime) {
this.scheduledStartTime = scheduledStartTime;
}
public final Instant getCompletedTime() {
return completedTime;
}
@Override
public final Builder completedTime(Instant completedTime) {
this.completedTime = completedTime;
return this;
}
public final void setCompletedTime(Instant completedTime) {
this.completedTime = completedTime;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
@Override
public ReplicationRun build() {
return new ReplicationRun(this);
}
}
}