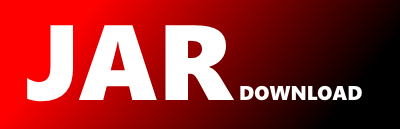
software.amazon.awssdk.services.sms.model.UpdateReplicationJobRequest Maven / Gradle / Ivy
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sms.model;
import java.time.Instant;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateReplicationJobRequest extends SmsRequest implements
ToCopyableBuilder {
private final String replicationJobId;
private final Integer frequency;
private final Instant nextReplicationRunStartTime;
private final String licenseType;
private final String roleName;
private final String description;
private UpdateReplicationJobRequest(BuilderImpl builder) {
super(builder);
this.replicationJobId = builder.replicationJobId;
this.frequency = builder.frequency;
this.nextReplicationRunStartTime = builder.nextReplicationRunStartTime;
this.licenseType = builder.licenseType;
this.roleName = builder.roleName;
this.description = builder.description;
}
/**
* Returns the value of the ReplicationJobId property for this object.
*
* @return The value of the ReplicationJobId property for this object.
*/
public String replicationJobId() {
return replicationJobId;
}
/**
* Returns the value of the Frequency property for this object.
*
* @return The value of the Frequency property for this object.
*/
public Integer frequency() {
return frequency;
}
/**
* Returns the value of the NextReplicationRunStartTime property for this object.
*
* @return The value of the NextReplicationRunStartTime property for this object.
*/
public Instant nextReplicationRunStartTime() {
return nextReplicationRunStartTime;
}
/**
* Returns the value of the LicenseType property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #licenseType} will
* return {@link LicenseType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #licenseTypeAsString}.
*
*
* @return The value of the LicenseType property for this object.
* @see LicenseType
*/
public LicenseType licenseType() {
return LicenseType.fromValue(licenseType);
}
/**
* Returns the value of the LicenseType property for this object.
*
* If the service returns an enum value that is not available in the current SDK version, {@link #licenseType} will
* return {@link LicenseType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #licenseTypeAsString}.
*
*
* @return The value of the LicenseType property for this object.
* @see LicenseType
*/
public String licenseTypeAsString() {
return licenseType;
}
/**
* Returns the value of the RoleName property for this object.
*
* @return The value of the RoleName property for this object.
*/
public String roleName() {
return roleName;
}
/**
* Returns the value of the Description property for this object.
*
* @return The value of the Description property for this object.
*/
public String description() {
return description;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(replicationJobId());
hashCode = 31 * hashCode + Objects.hashCode(frequency());
hashCode = 31 * hashCode + Objects.hashCode(nextReplicationRunStartTime());
hashCode = 31 * hashCode + Objects.hashCode(licenseTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(roleName());
hashCode = 31 * hashCode + Objects.hashCode(description());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateReplicationJobRequest)) {
return false;
}
UpdateReplicationJobRequest other = (UpdateReplicationJobRequest) obj;
return Objects.equals(replicationJobId(), other.replicationJobId()) && Objects.equals(frequency(), other.frequency())
&& Objects.equals(nextReplicationRunStartTime(), other.nextReplicationRunStartTime())
&& Objects.equals(licenseTypeAsString(), other.licenseTypeAsString())
&& Objects.equals(roleName(), other.roleName()) && Objects.equals(description(), other.description());
}
@Override
public String toString() {
return ToString.builder("UpdateReplicationJobRequest").add("ReplicationJobId", replicationJobId())
.add("Frequency", frequency()).add("NextReplicationRunStartTime", nextReplicationRunStartTime())
.add("LicenseType", licenseTypeAsString()).add("RoleName", roleName()).add("Description", description()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "replicationJobId":
return Optional.ofNullable(clazz.cast(replicationJobId()));
case "frequency":
return Optional.ofNullable(clazz.cast(frequency()));
case "nextReplicationRunStartTime":
return Optional.ofNullable(clazz.cast(nextReplicationRunStartTime()));
case "licenseType":
return Optional.ofNullable(clazz.cast(licenseTypeAsString()));
case "roleName":
return Optional.ofNullable(clazz.cast(roleName()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
default:
return Optional.empty();
}
}
public interface Builder extends SmsRequest.Builder, CopyableBuilder {
/**
* Sets the value of the ReplicationJobId property for this object.
*
* @param replicationJobId
* The new value for the ReplicationJobId property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder replicationJobId(String replicationJobId);
/**
* Sets the value of the Frequency property for this object.
*
* @param frequency
* The new value for the Frequency property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder frequency(Integer frequency);
/**
* Sets the value of the NextReplicationRunStartTime property for this object.
*
* @param nextReplicationRunStartTime
* The new value for the NextReplicationRunStartTime property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder nextReplicationRunStartTime(Instant nextReplicationRunStartTime);
/**
* Sets the value of the LicenseType property for this object.
*
* @param licenseType
* The new value for the LicenseType property for this object.
* @see LicenseType
* @return Returns a reference to this object so that method calls can be chained together.
* @see LicenseType
*/
Builder licenseType(String licenseType);
/**
* Sets the value of the LicenseType property for this object.
*
* @param licenseType
* The new value for the LicenseType property for this object.
* @see LicenseType
* @return Returns a reference to this object so that method calls can be chained together.
* @see LicenseType
*/
Builder licenseType(LicenseType licenseType);
/**
* Sets the value of the RoleName property for this object.
*
* @param roleName
* The new value for the RoleName property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder roleName(String roleName);
/**
* Sets the value of the Description property for this object.
*
* @param description
* The new value for the Description property for this object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends SmsRequest.BuilderImpl implements Builder {
private String replicationJobId;
private Integer frequency;
private Instant nextReplicationRunStartTime;
private String licenseType;
private String roleName;
private String description;
private BuilderImpl() {
}
private BuilderImpl(UpdateReplicationJobRequest model) {
super(model);
replicationJobId(model.replicationJobId);
frequency(model.frequency);
nextReplicationRunStartTime(model.nextReplicationRunStartTime);
licenseType(model.licenseType);
roleName(model.roleName);
description(model.description);
}
public final String getReplicationJobId() {
return replicationJobId;
}
@Override
public final Builder replicationJobId(String replicationJobId) {
this.replicationJobId = replicationJobId;
return this;
}
public final void setReplicationJobId(String replicationJobId) {
this.replicationJobId = replicationJobId;
}
public final Integer getFrequency() {
return frequency;
}
@Override
public final Builder frequency(Integer frequency) {
this.frequency = frequency;
return this;
}
public final void setFrequency(Integer frequency) {
this.frequency = frequency;
}
public final Instant getNextReplicationRunStartTime() {
return nextReplicationRunStartTime;
}
@Override
public final Builder nextReplicationRunStartTime(Instant nextReplicationRunStartTime) {
this.nextReplicationRunStartTime = nextReplicationRunStartTime;
return this;
}
public final void setNextReplicationRunStartTime(Instant nextReplicationRunStartTime) {
this.nextReplicationRunStartTime = nextReplicationRunStartTime;
}
public final String getLicenseType() {
return licenseType;
}
@Override
public final Builder licenseType(String licenseType) {
this.licenseType = licenseType;
return this;
}
@Override
public final Builder licenseType(LicenseType licenseType) {
this.licenseType(licenseType.toString());
return this;
}
public final void setLicenseType(String licenseType) {
this.licenseType = licenseType;
}
public final String getRoleName() {
return roleName;
}
@Override
public final Builder roleName(String roleName) {
this.roleName = roleName;
return this;
}
public final void setRoleName(String roleName) {
this.roleName = roleName;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public UpdateReplicationJobRequest build() {
return new UpdateReplicationJobRequest(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy