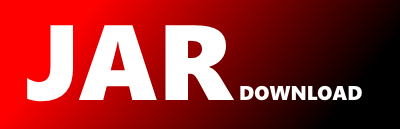
software.amazon.awssdk.services.servicediscovery.model.CreateServiceRequest Maven / Gradle / Ivy
Show all versions of servicediscovery Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.servicediscovery.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateServiceRequest extends ServiceDiscoveryRequest implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(CreateServiceRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField NAMESPACE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NamespaceId").getter(getter(CreateServiceRequest::namespaceId)).setter(setter(Builder::namespaceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NamespaceId").build()).build();
private static final SdkField CREATOR_REQUEST_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CreatorRequestId")
.getter(getter(CreateServiceRequest::creatorRequestId))
.setter(setter(Builder::creatorRequestId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatorRequestId").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateServiceRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField DNS_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("DnsConfig").getter(getter(CreateServiceRequest::dnsConfig)).setter(setter(Builder::dnsConfig))
.constructor(DnsConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DnsConfig").build()).build();
private static final SdkField HEALTH_CHECK_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("HealthCheckConfig")
.getter(getter(CreateServiceRequest::healthCheckConfig)).setter(setter(Builder::healthCheckConfig))
.constructor(HealthCheckConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckConfig").build()).build();
private static final SdkField HEALTH_CHECK_CUSTOM_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("HealthCheckCustomConfig")
.getter(getter(CreateServiceRequest::healthCheckCustomConfig)).setter(setter(Builder::healthCheckCustomConfig))
.constructor(HealthCheckCustomConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckCustomConfig").build())
.build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateServiceRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(CreateServiceRequest::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD,
NAMESPACE_ID_FIELD, CREATOR_REQUEST_ID_FIELD, DESCRIPTION_FIELD, DNS_CONFIG_FIELD, HEALTH_CHECK_CONFIG_FIELD,
HEALTH_CHECK_CUSTOM_CONFIG_FIELD, TAGS_FIELD, TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String name;
private final String namespaceId;
private final String creatorRequestId;
private final String description;
private final DnsConfig dnsConfig;
private final HealthCheckConfig healthCheckConfig;
private final HealthCheckCustomConfig healthCheckCustomConfig;
private final List tags;
private final String type;
private CreateServiceRequest(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.namespaceId = builder.namespaceId;
this.creatorRequestId = builder.creatorRequestId;
this.description = builder.description;
this.dnsConfig = builder.dnsConfig;
this.healthCheckConfig = builder.healthCheckConfig;
this.healthCheckCustomConfig = builder.healthCheckCustomConfig;
this.tags = builder.tags;
this.type = builder.type;
}
/**
*
* The name that you want to assign to the service.
*
*
*
* Do not include sensitive information in the name if the namespace is discoverable by public DNS queries.
*
*
*
* If you want Cloud Map to create an SRV
record when you register an instance and you're using a
* system that requires a specific SRV
format, such as HAProxy,
* specify the following for Name
:
*
*
* -
*
* Start the name with an underscore (_), such as _exampleservice
.
*
*
* -
*
* End the name with ._protocol, such as ._tcp
.
*
*
*
*
* When you register an instance, Cloud Map creates an SRV
record and assigns a name to the record by
* concatenating the service name and the namespace name (for example,
*
*
* _exampleservice._tcp.example.com
).
*
*
*
* For services that are accessible by DNS queries, you can't create multiple services with names that differ only
* by case (such as EXAMPLE and example). Otherwise, these services have the same DNS name and can't be
* distinguished. However, if you use a namespace that's only accessible by API calls, then you can create services
* that with names that differ only by case.
*
*
*
* @return The name that you want to assign to the service.
*
* Do not include sensitive information in the name if the namespace is discoverable by public DNS queries.
*
*
*
* If you want Cloud Map to create an SRV
record when you register an instance and you're using
* a system that requires a specific SRV
format, such as HAProxy, specify the following for Name
:
*
*
* -
*
* Start the name with an underscore (_), such as _exampleservice
.
*
*
* -
*
* End the name with ._protocol, such as ._tcp
.
*
*
*
*
* When you register an instance, Cloud Map creates an SRV
record and assigns a name to the
* record by concatenating the service name and the namespace name (for example,
*
*
* _exampleservice._tcp.example.com
).
*
*
*
* For services that are accessible by DNS queries, you can't create multiple services with names that
* differ only by case (such as EXAMPLE and example). Otherwise, these services have the same DNS name and
* can't be distinguished. However, if you use a namespace that's only accessible by API calls, then you can
* create services that with names that differ only by case.
*
*/
public final String name() {
return name;
}
/**
*
* The ID of the namespace that you want to use to create the service. The namespace ID must be specified, but it
* can be specified either here or in the DnsConfig
object.
*
*
* @return The ID of the namespace that you want to use to create the service. The namespace ID must be specified,
* but it can be specified either here or in the DnsConfig
object.
*/
public final String namespaceId() {
return namespaceId;
}
/**
*
* A unique string that identifies the request and that allows failed CreateService
requests to be
* retried without the risk of running the operation twice. CreatorRequestId
can be any unique string
* (for example, a date/timestamp).
*
*
* @return A unique string that identifies the request and that allows failed CreateService
requests to
* be retried without the risk of running the operation twice. CreatorRequestId
can be any
* unique string (for example, a date/timestamp).
*/
public final String creatorRequestId() {
return creatorRequestId;
}
/**
*
* A description for the service.
*
*
* @return A description for the service.
*/
public final String description() {
return description;
}
/**
*
* A complex type that contains information about the Amazon Route 53 records that you want Cloud Map to create when
* you register an instance.
*
*
* @return A complex type that contains information about the Amazon Route 53 records that you want Cloud Map to
* create when you register an instance.
*/
public final DnsConfig dnsConfig() {
return dnsConfig;
}
/**
*
* Public DNS and HTTP namespaces only. A complex type that contains settings for an optional Route 53 health
* check. If you specify settings for a health check, Cloud Map associates the health check with all the Route 53
* DNS records that you specify in DnsConfig
.
*
*
*
* If you specify a health check configuration, you can specify either HealthCheckCustomConfig
or
* HealthCheckConfig
but not both.
*
*
*
* For information about the charges for health checks, see Cloud
* Map Pricing.
*
*
* @return Public DNS and HTTP namespaces only. A complex type that contains settings for an optional
* Route 53 health check. If you specify settings for a health check, Cloud Map associates the health check
* with all the Route 53 DNS records that you specify in DnsConfig
.
*
* If you specify a health check configuration, you can specify either HealthCheckCustomConfig
* or HealthCheckConfig
but not both.
*
*
*
* For information about the charges for health checks, see Cloud Map Pricing.
*/
public final HealthCheckConfig healthCheckConfig() {
return healthCheckConfig;
}
/**
*
* A complex type that contains information about an optional custom health check.
*
*
*
* If you specify a health check configuration, you can specify either HealthCheckCustomConfig
or
* HealthCheckConfig
but not both.
*
*
*
* You can't add, update, or delete a HealthCheckCustomConfig
configuration from an existing service.
*
*
* @return A complex type that contains information about an optional custom health check.
*
* If you specify a health check configuration, you can specify either HealthCheckCustomConfig
* or HealthCheckConfig
but not both.
*
*
*
* You can't add, update, or delete a HealthCheckCustomConfig
configuration from an existing
* service.
*/
public final HealthCheckCustomConfig healthCheckCustomConfig() {
return healthCheckCustomConfig;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* The tags to add to the service. Each tag consists of a key and an optional value that you define. Tags keys can
* be up to 128 characters in length, and tag values can be up to 256 characters in length.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return The tags to add to the service. Each tag consists of a key and an optional value that you define. Tags
* keys can be up to 128 characters in length, and tag values can be up to 256 characters in length.
*/
public final List tags() {
return tags;
}
/**
*
* If present, specifies that the service instances are only discoverable using the DiscoverInstances
* API operation. No DNS records is registered for the service instances. The only valid value is HTTP
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ServiceTypeOption#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return If present, specifies that the service instances are only discoverable using the
* DiscoverInstances
API operation. No DNS records is registered for the service instances. The
* only valid value is HTTP
.
* @see ServiceTypeOption
*/
public final ServiceTypeOption type() {
return ServiceTypeOption.fromValue(type);
}
/**
*
* If present, specifies that the service instances are only discoverable using the DiscoverInstances
* API operation. No DNS records is registered for the service instances. The only valid value is HTTP
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ServiceTypeOption#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return If present, specifies that the service instances are only discoverable using the
* DiscoverInstances
API operation. No DNS records is registered for the service instances. The
* only valid value is HTTP
.
* @see ServiceTypeOption
*/
public final String typeAsString() {
return type;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(namespaceId());
hashCode = 31 * hashCode + Objects.hashCode(creatorRequestId());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(dnsConfig());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckConfig());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckCustomConfig());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateServiceRequest)) {
return false;
}
CreateServiceRequest other = (CreateServiceRequest) obj;
return Objects.equals(name(), other.name()) && Objects.equals(namespaceId(), other.namespaceId())
&& Objects.equals(creatorRequestId(), other.creatorRequestId())
&& Objects.equals(description(), other.description()) && Objects.equals(dnsConfig(), other.dnsConfig())
&& Objects.equals(healthCheckConfig(), other.healthCheckConfig())
&& Objects.equals(healthCheckCustomConfig(), other.healthCheckCustomConfig()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(typeAsString(), other.typeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateServiceRequest").add("Name", name()).add("NamespaceId", namespaceId())
.add("CreatorRequestId", creatorRequestId()).add("Description", description()).add("DnsConfig", dnsConfig())
.add("HealthCheckConfig", healthCheckConfig()).add("HealthCheckCustomConfig", healthCheckCustomConfig())
.add("Tags", hasTags() ? tags() : null).add("Type", typeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "NamespaceId":
return Optional.ofNullable(clazz.cast(namespaceId()));
case "CreatorRequestId":
return Optional.ofNullable(clazz.cast(creatorRequestId()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "DnsConfig":
return Optional.ofNullable(clazz.cast(dnsConfig()));
case "HealthCheckConfig":
return Optional.ofNullable(clazz.cast(healthCheckConfig()));
case "HealthCheckCustomConfig":
return Optional.ofNullable(clazz.cast(healthCheckCustomConfig()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Name", NAME_FIELD);
map.put("NamespaceId", NAMESPACE_ID_FIELD);
map.put("CreatorRequestId", CREATOR_REQUEST_ID_FIELD);
map.put("Description", DESCRIPTION_FIELD);
map.put("DnsConfig", DNS_CONFIG_FIELD);
map.put("HealthCheckConfig", HEALTH_CHECK_CONFIG_FIELD);
map.put("HealthCheckCustomConfig", HEALTH_CHECK_CUSTOM_CONFIG_FIELD);
map.put("Tags", TAGS_FIELD);
map.put("Type", TYPE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function