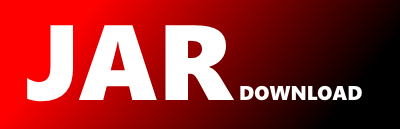
software.amazon.awssdk.services.servicediscovery.model.ServiceSummary Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.servicediscovery.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A complex type that contains information about a specified service.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ServiceSummary implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(ServiceSummary::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(ServiceSummary::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(ServiceSummary::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Type")
.getter(getter(ServiceSummary::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Type").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(ServiceSummary::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField INSTANCE_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("InstanceCount").getter(getter(ServiceSummary::instanceCount)).setter(setter(Builder::instanceCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceCount").build()).build();
private static final SdkField DNS_CONFIG_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("DnsConfig").getter(getter(ServiceSummary::dnsConfig)).setter(setter(Builder::dnsConfig))
.constructor(DnsConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DnsConfig").build()).build();
private static final SdkField HEALTH_CHECK_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("HealthCheckConfig")
.getter(getter(ServiceSummary::healthCheckConfig)).setter(setter(Builder::healthCheckConfig))
.constructor(HealthCheckConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckConfig").build()).build();
private static final SdkField HEALTH_CHECK_CUSTOM_CONFIG_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("HealthCheckCustomConfig")
.getter(getter(ServiceSummary::healthCheckCustomConfig)).setter(setter(Builder::healthCheckCustomConfig))
.constructor(HealthCheckCustomConfig::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HealthCheckCustomConfig").build())
.build();
private static final SdkField CREATE_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreateDate").getter(getter(ServiceSummary::createDate)).setter(setter(Builder::createDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateDate").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, ARN_FIELD,
NAME_FIELD, TYPE_FIELD, DESCRIPTION_FIELD, INSTANCE_COUNT_FIELD, DNS_CONFIG_FIELD, HEALTH_CHECK_CONFIG_FIELD,
HEALTH_CHECK_CUSTOM_CONFIG_FIELD, CREATE_DATE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String id;
private final String arn;
private final String name;
private final String type;
private final String description;
private final Integer instanceCount;
private final DnsConfig dnsConfig;
private final HealthCheckConfig healthCheckConfig;
private final HealthCheckCustomConfig healthCheckCustomConfig;
private final Instant createDate;
private ServiceSummary(BuilderImpl builder) {
this.id = builder.id;
this.arn = builder.arn;
this.name = builder.name;
this.type = builder.type;
this.description = builder.description;
this.instanceCount = builder.instanceCount;
this.dnsConfig = builder.dnsConfig;
this.healthCheckConfig = builder.healthCheckConfig;
this.healthCheckCustomConfig = builder.healthCheckCustomConfig;
this.createDate = builder.createDate;
}
/**
*
* The ID that Cloud Map assigned to the service when you created it.
*
*
* @return The ID that Cloud Map assigned to the service when you created it.
*/
public final String id() {
return id;
}
/**
*
* The Amazon Resource Name (ARN) that Cloud Map assigns to the service when you create it.
*
*
* @return The Amazon Resource Name (ARN) that Cloud Map assigns to the service when you create it.
*/
public final String arn() {
return arn;
}
/**
*
* The name of the service.
*
*
* @return The name of the service.
*/
public final String name() {
return name;
}
/**
*
* Describes the systems that can be used to discover the service instances.
*
*
* - DNS_HTTP
* -
*
* The service instances can be discovered using either DNS queries or the DiscoverInstances
API
* operation.
*
*
* - HTTP
* -
*
* The service instances can only be discovered using the DiscoverInstances
API operation.
*
*
* - DNS
* -
*
* Reserved.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return Describes the systems that can be used to discover the service instances.
*
* - DNS_HTTP
* -
*
* The service instances can be discovered using either DNS queries or the DiscoverInstances
* API operation.
*
*
* - HTTP
* -
*
* The service instances can only be discovered using the DiscoverInstances
API operation.
*
*
* - DNS
* -
*
* Reserved.
*
*
* @see ServiceType
*/
public final ServiceType type() {
return ServiceType.fromValue(type);
}
/**
*
* Describes the systems that can be used to discover the service instances.
*
*
* - DNS_HTTP
* -
*
* The service instances can be discovered using either DNS queries or the DiscoverInstances
API
* operation.
*
*
* - HTTP
* -
*
* The service instances can only be discovered using the DiscoverInstances
API operation.
*
*
* - DNS
* -
*
* Reserved.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link ServiceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return Describes the systems that can be used to discover the service instances.
*
* - DNS_HTTP
* -
*
* The service instances can be discovered using either DNS queries or the DiscoverInstances
* API operation.
*
*
* - HTTP
* -
*
* The service instances can only be discovered using the DiscoverInstances
API operation.
*
*
* - DNS
* -
*
* Reserved.
*
*
* @see ServiceType
*/
public final String typeAsString() {
return type;
}
/**
*
* The description that you specify when you create the service.
*
*
* @return The description that you specify when you create the service.
*/
public final String description() {
return description;
}
/**
*
* The number of instances that are currently associated with the service. Instances that were previously associated
* with the service but that are deleted aren't included in the count. The count might not reflect pending
* registrations and deregistrations.
*
*
* @return The number of instances that are currently associated with the service. Instances that were previously
* associated with the service but that are deleted aren't included in the count. The count might not
* reflect pending registrations and deregistrations.
*/
public final Integer instanceCount() {
return instanceCount;
}
/**
*
* Information about the Route 53 DNS records that you want Cloud Map to create when you register an instance.
*
*
* @return Information about the Route 53 DNS records that you want Cloud Map to create when you register an
* instance.
*/
public final DnsConfig dnsConfig() {
return dnsConfig;
}
/**
*
* Public DNS and HTTP namespaces only. Settings for an optional health check. If you specify settings for a
* health check, Cloud Map associates the health check with the records that you specify in DnsConfig
.
*
*
* @return Public DNS and HTTP namespaces only. Settings for an optional health check. If you specify
* settings for a health check, Cloud Map associates the health check with the records that you specify in
* DnsConfig
.
*/
public final HealthCheckConfig healthCheckConfig() {
return healthCheckConfig;
}
/**
*
* Information about an optional custom health check. A custom health check, which requires that you use a
* third-party health checker to evaluate the health of your resources, is useful in the following circumstances:
*
*
* -
*
* You can't use a health check that's defined by HealthCheckConfig
because the resource isn't
* available over the internet. For example, you can use a custom health check when the instance is in an Amazon
* VPC. (To check the health of resources in a VPC, the health checker must also be in the VPC.)
*
*
* -
*
* You want to use a third-party health checker regardless of where your resources are located.
*
*
*
*
*
* If you specify a health check configuration, you can specify either HealthCheckCustomConfig
or
* HealthCheckConfig
but not both.
*
*
*
* @return Information about an optional custom health check. A custom health check, which requires that you use a
* third-party health checker to evaluate the health of your resources, is useful in the following
* circumstances:
*
* -
*
* You can't use a health check that's defined by HealthCheckConfig
because the resource isn't
* available over the internet. For example, you can use a custom health check when the instance is in an
* Amazon VPC. (To check the health of resources in a VPC, the health checker must also be in the VPC.)
*
*
* -
*
* You want to use a third-party health checker regardless of where your resources are located.
*
*
*
*
*
* If you specify a health check configuration, you can specify either HealthCheckCustomConfig
* or HealthCheckConfig
but not both.
*
*/
public final HealthCheckCustomConfig healthCheckCustomConfig() {
return healthCheckCustomConfig;
}
/**
*
* The date and time that the service was created.
*
*
* @return The date and time that the service was created.
*/
public final Instant createDate() {
return createDate;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(instanceCount());
hashCode = 31 * hashCode + Objects.hashCode(dnsConfig());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckConfig());
hashCode = 31 * hashCode + Objects.hashCode(healthCheckCustomConfig());
hashCode = 31 * hashCode + Objects.hashCode(createDate());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ServiceSummary)) {
return false;
}
ServiceSummary other = (ServiceSummary) obj;
return Objects.equals(id(), other.id()) && Objects.equals(arn(), other.arn()) && Objects.equals(name(), other.name())
&& Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(description(), other.description())
&& Objects.equals(instanceCount(), other.instanceCount()) && Objects.equals(dnsConfig(), other.dnsConfig())
&& Objects.equals(healthCheckConfig(), other.healthCheckConfig())
&& Objects.equals(healthCheckCustomConfig(), other.healthCheckCustomConfig())
&& Objects.equals(createDate(), other.createDate());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ServiceSummary").add("Id", id()).add("Arn", arn()).add("Name", name())
.add("Type", typeAsString()).add("Description", description()).add("InstanceCount", instanceCount())
.add("DnsConfig", dnsConfig()).add("HealthCheckConfig", healthCheckConfig())
.add("HealthCheckCustomConfig", healthCheckCustomConfig()).add("CreateDate", createDate()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "Type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "InstanceCount":
return Optional.ofNullable(clazz.cast(instanceCount()));
case "DnsConfig":
return Optional.ofNullable(clazz.cast(dnsConfig()));
case "HealthCheckConfig":
return Optional.ofNullable(clazz.cast(healthCheckConfig()));
case "HealthCheckCustomConfig":
return Optional.ofNullable(clazz.cast(healthCheckCustomConfig()));
case "CreateDate":
return Optional.ofNullable(clazz.cast(createDate()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Id", ID_FIELD);
map.put("Arn", ARN_FIELD);
map.put("Name", NAME_FIELD);
map.put("Type", TYPE_FIELD);
map.put("Description", DESCRIPTION_FIELD);
map.put("InstanceCount", INSTANCE_COUNT_FIELD);
map.put("DnsConfig", DNS_CONFIG_FIELD);
map.put("HealthCheckConfig", HEALTH_CHECK_CONFIG_FIELD);
map.put("HealthCheckCustomConfig", HEALTH_CHECK_CUSTOM_CONFIG_FIELD);
map.put("CreateDate", CREATE_DATE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function