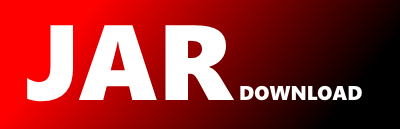
software.amazon.awssdk.services.ses.model.SendRawEmailRequest Maven / Gradle / Ivy
Show all versions of ses Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ses.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a request to send a single raw email using Amazon SES. For more information, see the Amazon SES Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SendRawEmailRequest extends SesRequest implements
ToCopyableBuilder {
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Source")
.getter(getter(SendRawEmailRequest::source)).setter(setter(Builder::source))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Source").build()).build();
private static final SdkField> DESTINATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Destinations")
.getter(getter(SendRawEmailRequest::destinations))
.setter(setter(Builder::destinations))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Destinations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField RAW_MESSAGE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("RawMessage").getter(getter(SendRawEmailRequest::rawMessage)).setter(setter(Builder::rawMessage))
.constructor(RawMessage::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RawMessage").build()).build();
private static final SdkField FROM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FromArn").getter(getter(SendRawEmailRequest::fromArn)).setter(setter(Builder::fromArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FromArn").build()).build();
private static final SdkField SOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceArn").getter(getter(SendRawEmailRequest::sourceArn)).setter(setter(Builder::sourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceArn").build()).build();
private static final SdkField RETURN_PATH_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReturnPathArn").getter(getter(SendRawEmailRequest::returnPathArn))
.setter(setter(Builder::returnPathArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReturnPathArn").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(SendRawEmailRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MessageTag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CONFIGURATION_SET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ConfigurationSetName").getter(getter(SendRawEmailRequest::configurationSetName))
.setter(setter(Builder::configurationSetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationSetName").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SOURCE_FIELD,
DESTINATIONS_FIELD, RAW_MESSAGE_FIELD, FROM_ARN_FIELD, SOURCE_ARN_FIELD, RETURN_PATH_ARN_FIELD, TAGS_FIELD,
CONFIGURATION_SET_NAME_FIELD));
private final String source;
private final List destinations;
private final RawMessage rawMessage;
private final String fromArn;
private final String sourceArn;
private final String returnPathArn;
private final List tags;
private final String configurationSetName;
private SendRawEmailRequest(BuilderImpl builder) {
super(builder);
this.source = builder.source;
this.destinations = builder.destinations;
this.rawMessage = builder.rawMessage;
this.fromArn = builder.fromArn;
this.sourceArn = builder.sourceArn;
this.returnPathArn = builder.returnPathArn;
this.tags = builder.tags;
this.configurationSetName = builder.configurationSetName;
}
/**
*
* The identity's email address. If you do not provide a value for this parameter, you must specify a "From" address
* in the raw text of the message. (You can also specify both.)
*
*
*
* Amazon SES does not support the SMTPUTF8 extension, as described inRFC6531. For this reason, the local part of a source email
* address (the part of the email address that precedes the @ sign) may only contain 7-bit ASCII characters. If the domain
* part of an address (the part after the @ sign) contains non-ASCII characters, they must be encoded using
* Punycode, as described in RFC3492. The sender name (also
* known as the friendly name) may contain non-ASCII characters. These characters must be encoded using MIME
* encoded-word syntax, as described in RFC 2047. MIME
* encoded-word syntax uses the following form: =?charset?encoding?encoded-text?=
.
*
*
*
* If you specify the Source
parameter and have feedback forwarding enabled, then bounces and
* complaints will be sent to this email address. This takes precedence over any Return-Path header that you might
* include in the raw text of the message.
*
*
* @return The identity's email address. If you do not provide a value for this parameter, you must specify a "From"
* address in the raw text of the message. (You can also specify both.)
*
* Amazon SES does not support the SMTPUTF8 extension, as described inRFC6531. For this reason, the local part of a
* source email address (the part of the email address that precedes the @ sign) may only contain 7-bit ASCII characters. If the
* domain part of an address (the part after the @ sign) contains non-ASCII characters, they must be
* encoded using Punycode, as described in RFC3492.
* The sender name (also known as the friendly name) may contain non-ASCII characters. These
* characters must be encoded using MIME encoded-word syntax, as described in RFC 2047. MIME encoded-word syntax uses the following
* form: =?charset?encoding?encoded-text?=
.
*
*
*
* If you specify the Source
parameter and have feedback forwarding enabled, then bounces and
* complaints will be sent to this email address. This takes precedence over any Return-Path header that you
* might include in the raw text of the message.
*/
public String source() {
return source;
}
/**
* Returns true if the Destinations property was specified by the sender (it may be empty), or false if the sender
* did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasDestinations() {
return destinations != null && !(destinations instanceof SdkAutoConstructList);
}
/**
*
* A list of destinations for the message, consisting of To:, CC:, and BCC: addresses.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasDestinations()} to see if a value was sent in this field.
*
*
* @return A list of destinations for the message, consisting of To:, CC:, and BCC: addresses.
*/
public List destinations() {
return destinations;
}
/**
*
* The raw email message itself. The message has to meet the following criteria:
*
*
* -
*
* The message has to contain a header and a body, separated by a blank line.
*
*
* -
*
* All of the required header fields must be present in the message.
*
*
* -
*
* Each part of a multipart MIME message must be formatted properly.
*
*
* -
*
* Attachments must be of a content type that Amazon SES supports. For a list on unsupported content types, see Unsupported Attachment Types in
* the Amazon SES Developer Guide.
*
*
* -
*
* The entire message must be base64-encoded.
*
*
* -
*
* If any of the MIME parts in your message contain content that is outside of the 7-bit ASCII character range, we
* highly recommend that you encode that content. For more information, see Sending Raw Email in the
* Amazon SES Developer Guide.
*
*
* -
*
* Per RFC 5321, the maximum length of each line
* of text, including the <CRLF>, must not exceed 1,000 characters.
*
*
*
*
* @return The raw email message itself. The message has to meet the following criteria:
*
* -
*
* The message has to contain a header and a body, separated by a blank line.
*
*
* -
*
* All of the required header fields must be present in the message.
*
*
* -
*
* Each part of a multipart MIME message must be formatted properly.
*
*
* -
*
* Attachments must be of a content type that Amazon SES supports. For a list on unsupported content types,
* see Unsupported
* Attachment Types in the Amazon SES Developer Guide.
*
*
* -
*
* The entire message must be base64-encoded.
*
*
* -
*
* If any of the MIME parts in your message contain content that is outside of the 7-bit ASCII character
* range, we highly recommend that you encode that content. For more information, see Sending Raw Email in
* the Amazon SES Developer Guide.
*
*
* -
*
* Per RFC 5321, the maximum length of
* each line of text, including the <CRLF>, must not exceed 1,000 characters.
*
*
*/
public RawMessage rawMessage() {
return rawMessage;
}
/**
*
* This parameter is used only for sending authorization. It is the ARN of the identity that is associated with the
* sending authorization policy that permits you to specify a particular "From" address in the header of the raw
* email.
*
*
* Instead of using this parameter, you can use the X-header X-SES-FROM-ARN
in the raw message of the
* email. If you use both the FromArn
parameter and the corresponding X-header, Amazon SES uses the
* value of the FromArn
parameter.
*
*
*
* For information about when to use this parameter, see the description of SendRawEmail
in this guide,
* or see the Amazon SES Developer Guide.
*
*
*
* @return This parameter is used only for sending authorization. It is the ARN of the identity that is associated
* with the sending authorization policy that permits you to specify a particular "From" address in the
* header of the raw email.
*
* Instead of using this parameter, you can use the X-header X-SES-FROM-ARN
in the raw message
* of the email. If you use both the FromArn
parameter and the corresponding X-header, Amazon
* SES uses the value of the FromArn
parameter.
*
*
*
* For information about when to use this parameter, see the description of SendRawEmail
in
* this guide, or see the Amazon SES Developer Guide.
*
*/
public String fromArn() {
return fromArn;
}
/**
*
* This parameter is used only for sending authorization. It is the ARN of the identity that is associated with the
* sending authorization policy that permits you to send for the email address specified in the Source
* parameter.
*
*
* For example, if the owner of example.com
(which has ARN
* arn:aws:ses:us-east-1:123456789012:identity/example.com
) attaches a policy to it that authorizes you
* to send from [email protected]
, then you would specify the SourceArn
to be
* arn:aws:ses:us-east-1:123456789012:identity/example.com
, and the Source
to be
* [email protected]
.
*
*
* Instead of using this parameter, you can use the X-header X-SES-SOURCE-ARN
in the raw message of the
* email. If you use both the SourceArn
parameter and the corresponding X-header, Amazon SES uses the
* value of the SourceArn
parameter.
*
*
*
* For information about when to use this parameter, see the description of SendRawEmail
in this guide,
* or see the Amazon SES Developer Guide.
*
*
*
* @return This parameter is used only for sending authorization. It is the ARN of the identity that is associated
* with the sending authorization policy that permits you to send for the email address specified in the
* Source
parameter.
*
* For example, if the owner of example.com
(which has ARN
* arn:aws:ses:us-east-1:123456789012:identity/example.com
) attaches a policy to it that
* authorizes you to send from [email protected]
, then you would specify the
* SourceArn
to be arn:aws:ses:us-east-1:123456789012:identity/example.com
, and
* the Source
to be [email protected]
.
*
*
* Instead of using this parameter, you can use the X-header X-SES-SOURCE-ARN
in the raw
* message of the email. If you use both the SourceArn
parameter and the corresponding
* X-header, Amazon SES uses the value of the SourceArn
parameter.
*
*
*
* For information about when to use this parameter, see the description of SendRawEmail
in
* this guide, or see the Amazon SES Developer Guide.
*
*/
public String sourceArn() {
return sourceArn;
}
/**
*
* This parameter is used only for sending authorization. It is the ARN of the identity that is associated with the
* sending authorization policy that permits you to use the email address specified in the ReturnPath
* parameter.
*
*
* For example, if the owner of example.com
(which has ARN
* arn:aws:ses:us-east-1:123456789012:identity/example.com
) attaches a policy to it that authorizes you
* to use [email protected]
, then you would specify the ReturnPathArn
to be
* arn:aws:ses:us-east-1:123456789012:identity/example.com
, and the ReturnPath
to be
* [email protected]
.
*
*
* Instead of using this parameter, you can use the X-header X-SES-RETURN-PATH-ARN
in the raw message
* of the email. If you use both the ReturnPathArn
parameter and the corresponding X-header, Amazon SES
* uses the value of the ReturnPathArn
parameter.
*
*
*
* For information about when to use this parameter, see the description of SendRawEmail
in this guide,
* or see the Amazon SES Developer Guide.
*
*
*
* @return This parameter is used only for sending authorization. It is the ARN of the identity that is associated
* with the sending authorization policy that permits you to use the email address specified in the
* ReturnPath
parameter.
*
* For example, if the owner of example.com
(which has ARN
* arn:aws:ses:us-east-1:123456789012:identity/example.com
) attaches a policy to it that
* authorizes you to use [email protected]
, then you would specify the
* ReturnPathArn
to be arn:aws:ses:us-east-1:123456789012:identity/example.com
,
* and the ReturnPath
to be [email protected]
.
*
*
* Instead of using this parameter, you can use the X-header X-SES-RETURN-PATH-ARN
in the raw
* message of the email. If you use both the ReturnPathArn
parameter and the corresponding
* X-header, Amazon SES uses the value of the ReturnPathArn
parameter.
*
*
*
* For information about when to use this parameter, see the description of SendRawEmail
in
* this guide, or see the Amazon SES Developer Guide.
*
*/
public String returnPathArn() {
return returnPathArn;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tags, in the form of name/value pairs, to apply to an email that you send using
* SendRawEmail
. Tags correspond to characteristics of the email that you define, so that you can
* publish email sending events.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return A list of tags, in the form of name/value pairs, to apply to an email that you send using
* SendRawEmail
. Tags correspond to characteristics of the email that you define, so that you
* can publish email sending events.
*/
public List tags() {
return tags;
}
/**
*
* The name of the configuration set to use when you send an email using SendRawEmail
.
*
*
* @return The name of the configuration set to use when you send an email using SendRawEmail
.
*/
public String configurationSetName() {
return configurationSetName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(hasDestinations() ? destinations() : null);
hashCode = 31 * hashCode + Objects.hashCode(rawMessage());
hashCode = 31 * hashCode + Objects.hashCode(fromArn());
hashCode = 31 * hashCode + Objects.hashCode(sourceArn());
hashCode = 31 * hashCode + Objects.hashCode(returnPathArn());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(configurationSetName());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SendRawEmailRequest)) {
return false;
}
SendRawEmailRequest other = (SendRawEmailRequest) obj;
return Objects.equals(source(), other.source()) && hasDestinations() == other.hasDestinations()
&& Objects.equals(destinations(), other.destinations()) && Objects.equals(rawMessage(), other.rawMessage())
&& Objects.equals(fromArn(), other.fromArn()) && Objects.equals(sourceArn(), other.sourceArn())
&& Objects.equals(returnPathArn(), other.returnPathArn()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(configurationSetName(), other.configurationSetName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("SendRawEmailRequest").add("Source", source())
.add("Destinations", hasDestinations() ? destinations() : null).add("RawMessage", rawMessage())
.add("FromArn", fromArn()).add("SourceArn", sourceArn()).add("ReturnPathArn", returnPathArn())
.add("Tags", hasTags() ? tags() : null).add("ConfigurationSetName", configurationSetName()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Source":
return Optional.ofNullable(clazz.cast(source()));
case "Destinations":
return Optional.ofNullable(clazz.cast(destinations()));
case "RawMessage":
return Optional.ofNullable(clazz.cast(rawMessage()));
case "FromArn":
return Optional.ofNullable(clazz.cast(fromArn()));
case "SourceArn":
return Optional.ofNullable(clazz.cast(sourceArn()));
case "ReturnPathArn":
return Optional.ofNullable(clazz.cast(returnPathArn()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ConfigurationSetName":
return Optional.ofNullable(clazz.cast(configurationSetName()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function