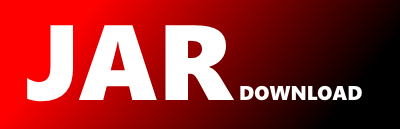
software.amazon.awssdk.services.ses.model.RecipientDsnFields Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ses.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Recipient-related information to include in the Delivery Status Notification (DSN) when an email that Amazon SES
* receives on your behalf bounces.
*
*
* For information about receiving email through Amazon SES, see the Amazon SES Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RecipientDsnFields implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField FINAL_RECIPIENT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FinalRecipient").getter(getter(RecipientDsnFields::finalRecipient))
.setter(setter(Builder::finalRecipient))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FinalRecipient").build()).build();
private static final SdkField ACTION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Action")
.getter(getter(RecipientDsnFields::actionAsString)).setter(setter(Builder::action))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Action").build()).build();
private static final SdkField REMOTE_MTA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RemoteMta").getter(getter(RecipientDsnFields::remoteMta)).setter(setter(Builder::remoteMta))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RemoteMta").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(RecipientDsnFields::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField DIAGNOSTIC_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DiagnosticCode").getter(getter(RecipientDsnFields::diagnosticCode))
.setter(setter(Builder::diagnosticCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DiagnosticCode").build()).build();
private static final SdkField LAST_ATTEMPT_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastAttemptDate").getter(getter(RecipientDsnFields::lastAttemptDate))
.setter(setter(Builder::lastAttemptDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastAttemptDate").build()).build();
private static final SdkField> EXTENSION_FIELDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ExtensionFields")
.getter(getter(RecipientDsnFields::extensionFields))
.setter(setter(Builder::extensionFields))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExtensionFields").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ExtensionField::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections
.unmodifiableList(Arrays.asList(FINAL_RECIPIENT_FIELD, ACTION_FIELD, REMOTE_MTA_FIELD, STATUS_FIELD,
DIAGNOSTIC_CODE_FIELD, LAST_ATTEMPT_DATE_FIELD, EXTENSION_FIELDS_FIELD));
private static final long serialVersionUID = 1L;
private final String finalRecipient;
private final String action;
private final String remoteMta;
private final String status;
private final String diagnosticCode;
private final Instant lastAttemptDate;
private final List extensionFields;
private RecipientDsnFields(BuilderImpl builder) {
this.finalRecipient = builder.finalRecipient;
this.action = builder.action;
this.remoteMta = builder.remoteMta;
this.status = builder.status;
this.diagnosticCode = builder.diagnosticCode;
this.lastAttemptDate = builder.lastAttemptDate;
this.extensionFields = builder.extensionFields;
}
/**
*
* The email address that the message was ultimately delivered to. This corresponds to the
* Final-Recipient
in the DSN. If not specified, FinalRecipient
will be set to the
* Recipient
specified in the BouncedRecipientInfo
structure. Either
* FinalRecipient
or the recipient in BouncedRecipientInfo
must be a recipient of the
* original bounced message.
*
*
*
* Do not prepend the FinalRecipient
email address with rfc 822;
, as described in RFC 3798.
*
*
*
* @return The email address that the message was ultimately delivered to. This corresponds to the
* Final-Recipient
in the DSN. If not specified, FinalRecipient
will be set to the
* Recipient
specified in the BouncedRecipientInfo
structure. Either
* FinalRecipient
or the recipient in BouncedRecipientInfo
must be a recipient of
* the original bounced message.
*
* Do not prepend the FinalRecipient
email address with rfc 822;
, as described in
* RFC 3798.
*
*/
public final String finalRecipient() {
return finalRecipient;
}
/**
*
* The action performed by the reporting mail transfer agent (MTA) as a result of its attempt to deliver the message
* to the recipient address. This is required by RFC 3464.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #action} will
* return {@link DsnAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #actionAsString}.
*
*
* @return The action performed by the reporting mail transfer agent (MTA) as a result of its attempt to deliver the
* message to the recipient address. This is required by RFC
* 3464.
* @see DsnAction
*/
public final DsnAction action() {
return DsnAction.fromValue(action);
}
/**
*
* The action performed by the reporting mail transfer agent (MTA) as a result of its attempt to deliver the message
* to the recipient address. This is required by RFC 3464.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #action} will
* return {@link DsnAction#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #actionAsString}.
*
*
* @return The action performed by the reporting mail transfer agent (MTA) as a result of its attempt to deliver the
* message to the recipient address. This is required by RFC
* 3464.
* @see DsnAction
*/
public final String actionAsString() {
return action;
}
/**
*
* The MTA to which the remote MTA attempted to deliver the message, formatted as specified in RFC 3464 (mta-name-type; mta-name
). This parameter
* typically applies only to propagating synchronous bounces.
*
*
* @return The MTA to which the remote MTA attempted to deliver the message, formatted as specified in RFC 3464 (mta-name-type; mta-name
). This
* parameter typically applies only to propagating synchronous bounces.
*/
public final String remoteMta() {
return remoteMta;
}
/**
*
* The status code that indicates what went wrong. This is required by RFC 3464.
*
*
* @return The status code that indicates what went wrong. This is required by RFC 3464.
*/
public final String status() {
return status;
}
/**
*
* An extended explanation of what went wrong; this is usually an SMTP response. See RFC 3463 for the correct formatting of this parameter.
*
*
* @return An extended explanation of what went wrong; this is usually an SMTP response. See RFC 3463 for the correct formatting of this parameter.
*/
public final String diagnosticCode() {
return diagnosticCode;
}
/**
*
* The time the final delivery attempt was made, in RFC 822
* date-time format.
*
*
* @return The time the final delivery attempt was made, in RFC
* 822 date-time format.
*/
public final Instant lastAttemptDate() {
return lastAttemptDate;
}
/**
* Returns true if the ExtensionFields property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasExtensionFields() {
return extensionFields != null && !(extensionFields instanceof SdkAutoConstructList);
}
/**
*
* Additional X-headers to include in the DSN.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasExtensionFields()} to see if a value was sent in this field.
*
*
* @return Additional X-headers to include in the DSN.
*/
public final List extensionFields() {
return extensionFields;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(finalRecipient());
hashCode = 31 * hashCode + Objects.hashCode(actionAsString());
hashCode = 31 * hashCode + Objects.hashCode(remoteMta());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(diagnosticCode());
hashCode = 31 * hashCode + Objects.hashCode(lastAttemptDate());
hashCode = 31 * hashCode + Objects.hashCode(hasExtensionFields() ? extensionFields() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RecipientDsnFields)) {
return false;
}
RecipientDsnFields other = (RecipientDsnFields) obj;
return Objects.equals(finalRecipient(), other.finalRecipient())
&& Objects.equals(actionAsString(), other.actionAsString()) && Objects.equals(remoteMta(), other.remoteMta())
&& Objects.equals(status(), other.status()) && Objects.equals(diagnosticCode(), other.diagnosticCode())
&& Objects.equals(lastAttemptDate(), other.lastAttemptDate())
&& hasExtensionFields() == other.hasExtensionFields()
&& Objects.equals(extensionFields(), other.extensionFields());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RecipientDsnFields").add("FinalRecipient", finalRecipient()).add("Action", actionAsString())
.add("RemoteMta", remoteMta()).add("Status", status()).add("DiagnosticCode", diagnosticCode())
.add("LastAttemptDate", lastAttemptDate())
.add("ExtensionFields", hasExtensionFields() ? extensionFields() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "FinalRecipient":
return Optional.ofNullable(clazz.cast(finalRecipient()));
case "Action":
return Optional.ofNullable(clazz.cast(actionAsString()));
case "RemoteMta":
return Optional.ofNullable(clazz.cast(remoteMta()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "DiagnosticCode":
return Optional.ofNullable(clazz.cast(diagnosticCode()));
case "LastAttemptDate":
return Optional.ofNullable(clazz.cast(lastAttemptDate()));
case "ExtensionFields":
return Optional.ofNullable(clazz.cast(extensionFields()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function