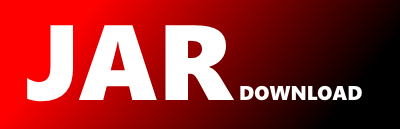
software.amazon.awssdk.services.ses.model.ReceiptAction Maven / Gradle / Ivy
Show all versions of ses Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ses.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* An action that Amazon SES can take when it receives an email on behalf of one or more email addresses or domains that
* you own. An instance of this data type can represent only one action.
*
*
* For information about setting up receipt rules, see the Amazon SES Developer
* Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReceiptAction implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField S3_ACTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("S3Action").getter(getter(ReceiptAction::s3Action)).setter(setter(Builder::s3Action))
.constructor(S3Action::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("S3Action").build()).build();
private static final SdkField BOUNCE_ACTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("BounceAction").getter(getter(ReceiptAction::bounceAction)).setter(setter(Builder::bounceAction))
.constructor(BounceAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BounceAction").build()).build();
private static final SdkField WORKMAIL_ACTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("WorkmailAction")
.getter(getter(ReceiptAction::workmailAction)).setter(setter(Builder::workmailAction))
.constructor(WorkmailAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkmailAction").build()).build();
private static final SdkField LAMBDA_ACTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("LambdaAction").getter(getter(ReceiptAction::lambdaAction)).setter(setter(Builder::lambdaAction))
.constructor(LambdaAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LambdaAction").build()).build();
private static final SdkField STOP_ACTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("StopAction").getter(getter(ReceiptAction::stopAction)).setter(setter(Builder::stopAction))
.constructor(StopAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StopAction").build()).build();
private static final SdkField ADD_HEADER_ACTION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AddHeaderAction")
.getter(getter(ReceiptAction::addHeaderAction)).setter(setter(Builder::addHeaderAction))
.constructor(AddHeaderAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AddHeaderAction").build()).build();
private static final SdkField SNS_ACTION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("SNSAction").getter(getter(ReceiptAction::snsAction)).setter(setter(Builder::snsAction))
.constructor(SNSAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SNSAction").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(S3_ACTION_FIELD,
BOUNCE_ACTION_FIELD, WORKMAIL_ACTION_FIELD, LAMBDA_ACTION_FIELD, STOP_ACTION_FIELD, ADD_HEADER_ACTION_FIELD,
SNS_ACTION_FIELD));
private static final long serialVersionUID = 1L;
private final S3Action s3Action;
private final BounceAction bounceAction;
private final WorkmailAction workmailAction;
private final LambdaAction lambdaAction;
private final StopAction stopAction;
private final AddHeaderAction addHeaderAction;
private final SNSAction snsAction;
private ReceiptAction(BuilderImpl builder) {
this.s3Action = builder.s3Action;
this.bounceAction = builder.bounceAction;
this.workmailAction = builder.workmailAction;
this.lambdaAction = builder.lambdaAction;
this.stopAction = builder.stopAction;
this.addHeaderAction = builder.addHeaderAction;
this.snsAction = builder.snsAction;
}
/**
*
* Saves the received message to an Amazon Simple Storage Service (Amazon S3) bucket and, optionally, publishes a
* notification to Amazon SNS.
*
*
* @return Saves the received message to an Amazon Simple Storage Service (Amazon S3) bucket and, optionally,
* publishes a notification to Amazon SNS.
*/
public final S3Action s3Action() {
return s3Action;
}
/**
*
* Rejects the received email by returning a bounce response to the sender and, optionally, publishes a notification
* to Amazon Simple Notification Service (Amazon SNS).
*
*
* @return Rejects the received email by returning a bounce response to the sender and, optionally, publishes a
* notification to Amazon Simple Notification Service (Amazon SNS).
*/
public final BounceAction bounceAction() {
return bounceAction;
}
/**
*
* Calls Amazon WorkMail and, optionally, publishes a notification to Amazon Amazon SNS.
*
*
* @return Calls Amazon WorkMail and, optionally, publishes a notification to Amazon Amazon SNS.
*/
public final WorkmailAction workmailAction() {
return workmailAction;
}
/**
*
* Calls an AWS Lambda function, and optionally, publishes a notification to Amazon SNS.
*
*
* @return Calls an AWS Lambda function, and optionally, publishes a notification to Amazon SNS.
*/
public final LambdaAction lambdaAction() {
return lambdaAction;
}
/**
*
* Terminates the evaluation of the receipt rule set and optionally publishes a notification to Amazon SNS.
*
*
* @return Terminates the evaluation of the receipt rule set and optionally publishes a notification to Amazon SNS.
*/
public final StopAction stopAction() {
return stopAction;
}
/**
*
* Adds a header to the received email.
*
*
* @return Adds a header to the received email.
*/
public final AddHeaderAction addHeaderAction() {
return addHeaderAction;
}
/**
*
* Publishes the email content within a notification to Amazon SNS.
*
*
* @return Publishes the email content within a notification to Amazon SNS.
*/
public final SNSAction snsAction() {
return snsAction;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(s3Action());
hashCode = 31 * hashCode + Objects.hashCode(bounceAction());
hashCode = 31 * hashCode + Objects.hashCode(workmailAction());
hashCode = 31 * hashCode + Objects.hashCode(lambdaAction());
hashCode = 31 * hashCode + Objects.hashCode(stopAction());
hashCode = 31 * hashCode + Objects.hashCode(addHeaderAction());
hashCode = 31 * hashCode + Objects.hashCode(snsAction());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReceiptAction)) {
return false;
}
ReceiptAction other = (ReceiptAction) obj;
return Objects.equals(s3Action(), other.s3Action()) && Objects.equals(bounceAction(), other.bounceAction())
&& Objects.equals(workmailAction(), other.workmailAction())
&& Objects.equals(lambdaAction(), other.lambdaAction()) && Objects.equals(stopAction(), other.stopAction())
&& Objects.equals(addHeaderAction(), other.addHeaderAction()) && Objects.equals(snsAction(), other.snsAction());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ReceiptAction").add("S3Action", s3Action()).add("BounceAction", bounceAction())
.add("WorkmailAction", workmailAction()).add("LambdaAction", lambdaAction()).add("StopAction", stopAction())
.add("AddHeaderAction", addHeaderAction()).add("SNSAction", snsAction()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "S3Action":
return Optional.ofNullable(clazz.cast(s3Action()));
case "BounceAction":
return Optional.ofNullable(clazz.cast(bounceAction()));
case "WorkmailAction":
return Optional.ofNullable(clazz.cast(workmailAction()));
case "LambdaAction":
return Optional.ofNullable(clazz.cast(lambdaAction()));
case "StopAction":
return Optional.ofNullable(clazz.cast(stopAction()));
case "AddHeaderAction":
return Optional.ofNullable(clazz.cast(addHeaderAction()));
case "SNSAction":
return Optional.ofNullable(clazz.cast(snsAction()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function