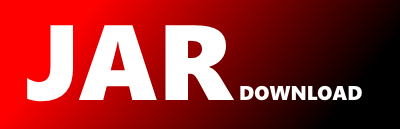
software.amazon.awssdk.services.ses.model.GetSendQuotaResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ses Show documentation
Show all versions of ses Show documentation
The AWS Java SDK for Amazon SES module holds the client classes that are used for communicating with
Amazon Simple Email
Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ses.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents your Amazon SES daily sending quota, maximum send rate, and the number of emails you have sent in the last
* 24 hours.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetSendQuotaResponse extends SesResponse implements
ToCopyableBuilder {
private static final SdkField MAX24_HOUR_SEND_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("Max24HourSend").getter(getter(GetSendQuotaResponse::max24HourSend))
.setter(setter(Builder::max24HourSend))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Max24HourSend").build()).build();
private static final SdkField MAX_SEND_RATE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("MaxSendRate").getter(getter(GetSendQuotaResponse::maxSendRate)).setter(setter(Builder::maxSendRate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxSendRate").build()).build();
private static final SdkField SENT_LAST24_HOURS_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("SentLast24Hours").getter(getter(GetSendQuotaResponse::sentLast24Hours))
.setter(setter(Builder::sentLast24Hours))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SentLast24Hours").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MAX24_HOUR_SEND_FIELD,
MAX_SEND_RATE_FIELD, SENT_LAST24_HOURS_FIELD));
private final Double max24HourSend;
private final Double maxSendRate;
private final Double sentLast24Hours;
private GetSendQuotaResponse(BuilderImpl builder) {
super(builder);
this.max24HourSend = builder.max24HourSend;
this.maxSendRate = builder.maxSendRate;
this.sentLast24Hours = builder.sentLast24Hours;
}
/**
*
* The maximum number of emails the user is allowed to send in a 24-hour interval. A value of -1 signifies an
* unlimited quota.
*
*
* @return The maximum number of emails the user is allowed to send in a 24-hour interval. A value of -1 signifies
* an unlimited quota.
*/
public final Double max24HourSend() {
return max24HourSend;
}
/**
*
* The maximum number of emails that Amazon SES can accept from the user's account per second.
*
*
*
* The rate at which Amazon SES accepts the user's messages might be less than the maximum send rate.
*
*
*
* @return The maximum number of emails that Amazon SES can accept from the user's account per second.
*
* The rate at which Amazon SES accepts the user's messages might be less than the maximum send rate.
*
*/
public final Double maxSendRate() {
return maxSendRate;
}
/**
*
* The number of emails sent during the previous 24 hours.
*
*
* @return The number of emails sent during the previous 24 hours.
*/
public final Double sentLast24Hours() {
return sentLast24Hours;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(max24HourSend());
hashCode = 31 * hashCode + Objects.hashCode(maxSendRate());
hashCode = 31 * hashCode + Objects.hashCode(sentLast24Hours());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetSendQuotaResponse)) {
return false;
}
GetSendQuotaResponse other = (GetSendQuotaResponse) obj;
return Objects.equals(max24HourSend(), other.max24HourSend()) && Objects.equals(maxSendRate(), other.maxSendRate())
&& Objects.equals(sentLast24Hours(), other.sentLast24Hours());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetSendQuotaResponse").add("Max24HourSend", max24HourSend()).add("MaxSendRate", maxSendRate())
.add("SentLast24Hours", sentLast24Hours()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Max24HourSend":
return Optional.ofNullable(clazz.cast(max24HourSend()));
case "MaxSendRate":
return Optional.ofNullable(clazz.cast(maxSendRate()));
case "SentLast24Hours":
return Optional.ofNullable(clazz.cast(sentLast24Hours()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy