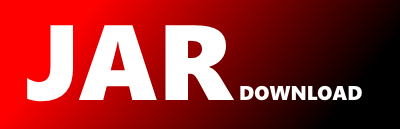
software.amazon.awssdk.services.ses.model.SendEmailRequest Maven / Gradle / Ivy
Show all versions of ses Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ses.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a request to send a single formatted email using Amazon SES. For more information, see the Amazon SES Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SendEmailRequest extends SesRequest implements ToCopyableBuilder {
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Source")
.getter(getter(SendEmailRequest::source)).setter(setter(Builder::source))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Source").build()).build();
private static final SdkField DESTINATION_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Destination").getter(getter(SendEmailRequest::destination)).setter(setter(Builder::destination))
.constructor(Destination::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Destination").build()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Message").getter(getter(SendEmailRequest::message)).setter(setter(Builder::message))
.constructor(Message::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Message").build()).build();
private static final SdkField> REPLY_TO_ADDRESSES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ReplyToAddresses")
.getter(getter(SendEmailRequest::replyToAddresses))
.setter(setter(Builder::replyToAddresses))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplyToAddresses").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField RETURN_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReturnPath").getter(getter(SendEmailRequest::returnPath)).setter(setter(Builder::returnPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReturnPath").build()).build();
private static final SdkField SOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceArn").getter(getter(SendEmailRequest::sourceArn)).setter(setter(Builder::sourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceArn").build()).build();
private static final SdkField RETURN_PATH_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReturnPathArn").getter(getter(SendEmailRequest::returnPathArn)).setter(setter(Builder::returnPathArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReturnPathArn").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(SendEmailRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MessageTag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CONFIGURATION_SET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ConfigurationSetName").getter(getter(SendEmailRequest::configurationSetName))
.setter(setter(Builder::configurationSetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfigurationSetName").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SOURCE_FIELD,
DESTINATION_FIELD, MESSAGE_FIELD, REPLY_TO_ADDRESSES_FIELD, RETURN_PATH_FIELD, SOURCE_ARN_FIELD,
RETURN_PATH_ARN_FIELD, TAGS_FIELD, CONFIGURATION_SET_NAME_FIELD));
private final String source;
private final Destination destination;
private final Message message;
private final List replyToAddresses;
private final String returnPath;
private final String sourceArn;
private final String returnPathArn;
private final List tags;
private final String configurationSetName;
private SendEmailRequest(BuilderImpl builder) {
super(builder);
this.source = builder.source;
this.destination = builder.destination;
this.message = builder.message;
this.replyToAddresses = builder.replyToAddresses;
this.returnPath = builder.returnPath;
this.sourceArn = builder.sourceArn;
this.returnPathArn = builder.returnPathArn;
this.tags = builder.tags;
this.configurationSetName = builder.configurationSetName;
}
/**
*
* The email address that is sending the email. This email address must be either individually verified with Amazon
* SES, or from a domain that has been verified with Amazon SES. For information about verifying identities, see the
* Amazon SES Developer Guide.
*
*
* If you are sending on behalf of another user and have been permitted to do so by a sending authorization policy,
* then you must also specify the SourceArn
parameter. For more information about sending
* authorization, see the Amazon SES
* Developer Guide.
*
*
*
* Amazon SES does not support the SMTPUTF8 extension, as described in RFC6531. For this reason, the email address string must be 7-bit
* ASCII. If you want to send to or from email addresses that contain Unicode characters in the domain part of an
* address, you must encode the domain using Punycode. Punycode is not permitted in the local part of the email
* address (the part before the @ sign) nor in the "friendly from" name. If you want to use Unicode characters in
* the "friendly from" name, you must encode the "friendly from" name using MIME encoded-word syntax, as described
* in Sending raw email using the Amazon SES
* API. For more information about Punycode, see RFC 3492.
*
*
*
* @return The email address that is sending the email. This email address must be either individually verified with
* Amazon SES, or from a domain that has been verified with Amazon SES. For information about verifying
* identities, see the Amazon
* SES Developer Guide.
*
* If you are sending on behalf of another user and have been permitted to do so by a sending authorization
* policy, then you must also specify the SourceArn
parameter. For more information about
* sending authorization, see the Amazon SES Developer
* Guide.
*
*
*
* Amazon SES does not support the SMTPUTF8 extension, as described in RFC6531. For this reason, the email address string must be
* 7-bit ASCII. If you want to send to or from email addresses that contain Unicode characters in the domain
* part of an address, you must encode the domain using Punycode. Punycode is not permitted in the local
* part of the email address (the part before the @ sign) nor in the "friendly from" name. If you want to
* use Unicode characters in the "friendly from" name, you must encode the "friendly from" name using MIME
* encoded-word syntax, as described in Sending raw email using the Amazon
* SES API. For more information about Punycode, see RFC
* 3492.
*
*/
public final String source() {
return source;
}
/**
*
* The destination for this email, composed of To:, CC:, and BCC: fields.
*
*
* @return The destination for this email, composed of To:, CC:, and BCC: fields.
*/
public final Destination destination() {
return destination;
}
/**
*
* The message to be sent.
*
*
* @return The message to be sent.
*/
public final Message message() {
return message;
}
/**
* For responses, this returns true if the service returned a value for the ReplyToAddresses property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasReplyToAddresses() {
return replyToAddresses != null && !(replyToAddresses instanceof SdkAutoConstructList);
}
/**
*
* The reply-to email address(es) for the message. If the recipient replies to the message, each reply-to address
* receives the reply.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasReplyToAddresses} method.
*
*
* @return The reply-to email address(es) for the message. If the recipient replies to the message, each reply-to
* address receives the reply.
*/
public final List replyToAddresses() {
return replyToAddresses;
}
/**
*
* The email address that bounces and complaints are forwarded to when feedback forwarding is enabled. If the
* message cannot be delivered to the recipient, then an error message is returned from the recipient's ISP; this
* message is forwarded to the email address specified by the ReturnPath
parameter. The
* ReturnPath
parameter is never overwritten. This email address must be either individually verified
* with Amazon SES, or from a domain that has been verified with Amazon SES.
*
*
* @return The email address that bounces and complaints are forwarded to when feedback forwarding is enabled. If
* the message cannot be delivered to the recipient, then an error message is returned from the recipient's
* ISP; this message is forwarded to the email address specified by the ReturnPath
parameter.
* The ReturnPath
parameter is never overwritten. This email address must be either
* individually verified with Amazon SES, or from a domain that has been verified with Amazon SES.
*/
public final String returnPath() {
return returnPath;
}
/**
*
* This parameter is used only for sending authorization. It is the ARN of the identity that is associated with the
* sending authorization policy that permits you to send for the email address specified in the Source
* parameter.
*
*
* For example, if the owner of example.com
(which has ARN
* arn:aws:ses:us-east-1:123456789012:identity/example.com
) attaches a policy to it that authorizes you
* to send from [email protected]
, then you would specify the SourceArn
to be
* arn:aws:ses:us-east-1:123456789012:identity/example.com
, and the Source
to be
* [email protected]
.
*
*
* For more information about sending authorization, see the Amazon SES Developer Guide.
*
*
* @return This parameter is used only for sending authorization. It is the ARN of the identity that is associated
* with the sending authorization policy that permits you to send for the email address specified in the
* Source
parameter.
*
* For example, if the owner of example.com
(which has ARN
* arn:aws:ses:us-east-1:123456789012:identity/example.com
) attaches a policy to it that
* authorizes you to send from [email protected]
, then you would specify the
* SourceArn
to be arn:aws:ses:us-east-1:123456789012:identity/example.com
, and
* the Source
to be [email protected]
.
*
*
* For more information about sending authorization, see the Amazon SES Developer
* Guide.
*/
public final String sourceArn() {
return sourceArn;
}
/**
*
* This parameter is used only for sending authorization. It is the ARN of the identity that is associated with the
* sending authorization policy that permits you to use the email address specified in the ReturnPath
* parameter.
*
*
* For example, if the owner of example.com
(which has ARN
* arn:aws:ses:us-east-1:123456789012:identity/example.com
) attaches a policy to it that authorizes you
* to use [email protected]
, then you would specify the ReturnPathArn
to be
* arn:aws:ses:us-east-1:123456789012:identity/example.com
, and the ReturnPath
to be
* [email protected]
.
*
*
* For more information about sending authorization, see the Amazon SES Developer Guide.
*
*
* @return This parameter is used only for sending authorization. It is the ARN of the identity that is associated
* with the sending authorization policy that permits you to use the email address specified in the
* ReturnPath
parameter.
*
* For example, if the owner of example.com
(which has ARN
* arn:aws:ses:us-east-1:123456789012:identity/example.com
) attaches a policy to it that
* authorizes you to use [email protected]
, then you would specify the
* ReturnPathArn
to be arn:aws:ses:us-east-1:123456789012:identity/example.com
,
* and the ReturnPath
to be [email protected]
.
*
*
* For more information about sending authorization, see the Amazon SES Developer
* Guide.
*/
public final String returnPathArn() {
return returnPathArn;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of tags, in the form of name/value pairs, to apply to an email that you send using SendEmail
.
* Tags correspond to characteristics of the email that you define, so that you can publish email sending events.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return A list of tags, in the form of name/value pairs, to apply to an email that you send using
* SendEmail
. Tags correspond to characteristics of the email that you define, so that you can
* publish email sending events.
*/
public final List tags() {
return tags;
}
/**
*
* The name of the configuration set to use when you send an email using SendEmail
.
*
*
* @return The name of the configuration set to use when you send an email using SendEmail
.
*/
public final String configurationSetName() {
return configurationSetName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(destination());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(hasReplyToAddresses() ? replyToAddresses() : null);
hashCode = 31 * hashCode + Objects.hashCode(returnPath());
hashCode = 31 * hashCode + Objects.hashCode(sourceArn());
hashCode = 31 * hashCode + Objects.hashCode(returnPathArn());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(configurationSetName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SendEmailRequest)) {
return false;
}
SendEmailRequest other = (SendEmailRequest) obj;
return Objects.equals(source(), other.source()) && Objects.equals(destination(), other.destination())
&& Objects.equals(message(), other.message()) && hasReplyToAddresses() == other.hasReplyToAddresses()
&& Objects.equals(replyToAddresses(), other.replyToAddresses())
&& Objects.equals(returnPath(), other.returnPath()) && Objects.equals(sourceArn(), other.sourceArn())
&& Objects.equals(returnPathArn(), other.returnPathArn()) && hasTags() == other.hasTags()
&& Objects.equals(tags(), other.tags()) && Objects.equals(configurationSetName(), other.configurationSetName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SendEmailRequest").add("Source", source()).add("Destination", destination())
.add("Message", message()).add("ReplyToAddresses", hasReplyToAddresses() ? replyToAddresses() : null)
.add("ReturnPath", returnPath()).add("SourceArn", sourceArn()).add("ReturnPathArn", returnPathArn())
.add("Tags", hasTags() ? tags() : null).add("ConfigurationSetName", configurationSetName()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Source":
return Optional.ofNullable(clazz.cast(source()));
case "Destination":
return Optional.ofNullable(clazz.cast(destination()));
case "Message":
return Optional.ofNullable(clazz.cast(message()));
case "ReplyToAddresses":
return Optional.ofNullable(clazz.cast(replyToAddresses()));
case "ReturnPath":
return Optional.ofNullable(clazz.cast(returnPath()));
case "SourceArn":
return Optional.ofNullable(clazz.cast(sourceArn()));
case "ReturnPathArn":
return Optional.ofNullable(clazz.cast(returnPathArn()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "ConfigurationSetName":
return Optional.ofNullable(clazz.cast(configurationSetName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function