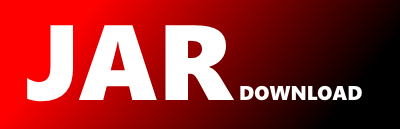
software.amazon.awssdk.services.ses.DefaultSesAsyncClient Maven / Gradle / Ivy
Show all versions of ses Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ses;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.query.AwsQueryProtocolFactory;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.ses.internal.SesServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.ses.model.AccountSendingPausedException;
import software.amazon.awssdk.services.ses.model.AlreadyExistsException;
import software.amazon.awssdk.services.ses.model.CannotDeleteException;
import software.amazon.awssdk.services.ses.model.CloneReceiptRuleSetRequest;
import software.amazon.awssdk.services.ses.model.CloneReceiptRuleSetResponse;
import software.amazon.awssdk.services.ses.model.ConfigurationSetAlreadyExistsException;
import software.amazon.awssdk.services.ses.model.ConfigurationSetDoesNotExistException;
import software.amazon.awssdk.services.ses.model.ConfigurationSetSendingPausedException;
import software.amazon.awssdk.services.ses.model.CreateConfigurationSetEventDestinationRequest;
import software.amazon.awssdk.services.ses.model.CreateConfigurationSetEventDestinationResponse;
import software.amazon.awssdk.services.ses.model.CreateConfigurationSetRequest;
import software.amazon.awssdk.services.ses.model.CreateConfigurationSetResponse;
import software.amazon.awssdk.services.ses.model.CreateConfigurationSetTrackingOptionsRequest;
import software.amazon.awssdk.services.ses.model.CreateConfigurationSetTrackingOptionsResponse;
import software.amazon.awssdk.services.ses.model.CreateCustomVerificationEmailTemplateRequest;
import software.amazon.awssdk.services.ses.model.CreateCustomVerificationEmailTemplateResponse;
import software.amazon.awssdk.services.ses.model.CreateReceiptFilterRequest;
import software.amazon.awssdk.services.ses.model.CreateReceiptFilterResponse;
import software.amazon.awssdk.services.ses.model.CreateReceiptRuleRequest;
import software.amazon.awssdk.services.ses.model.CreateReceiptRuleResponse;
import software.amazon.awssdk.services.ses.model.CreateReceiptRuleSetRequest;
import software.amazon.awssdk.services.ses.model.CreateReceiptRuleSetResponse;
import software.amazon.awssdk.services.ses.model.CreateTemplateRequest;
import software.amazon.awssdk.services.ses.model.CreateTemplateResponse;
import software.amazon.awssdk.services.ses.model.CustomVerificationEmailInvalidContentException;
import software.amazon.awssdk.services.ses.model.CustomVerificationEmailTemplateAlreadyExistsException;
import software.amazon.awssdk.services.ses.model.CustomVerificationEmailTemplateDoesNotExistException;
import software.amazon.awssdk.services.ses.model.DeleteConfigurationSetEventDestinationRequest;
import software.amazon.awssdk.services.ses.model.DeleteConfigurationSetEventDestinationResponse;
import software.amazon.awssdk.services.ses.model.DeleteConfigurationSetRequest;
import software.amazon.awssdk.services.ses.model.DeleteConfigurationSetResponse;
import software.amazon.awssdk.services.ses.model.DeleteConfigurationSetTrackingOptionsRequest;
import software.amazon.awssdk.services.ses.model.DeleteConfigurationSetTrackingOptionsResponse;
import software.amazon.awssdk.services.ses.model.DeleteCustomVerificationEmailTemplateRequest;
import software.amazon.awssdk.services.ses.model.DeleteCustomVerificationEmailTemplateResponse;
import software.amazon.awssdk.services.ses.model.DeleteIdentityPolicyRequest;
import software.amazon.awssdk.services.ses.model.DeleteIdentityPolicyResponse;
import software.amazon.awssdk.services.ses.model.DeleteIdentityRequest;
import software.amazon.awssdk.services.ses.model.DeleteIdentityResponse;
import software.amazon.awssdk.services.ses.model.DeleteReceiptFilterRequest;
import software.amazon.awssdk.services.ses.model.DeleteReceiptFilterResponse;
import software.amazon.awssdk.services.ses.model.DeleteReceiptRuleRequest;
import software.amazon.awssdk.services.ses.model.DeleteReceiptRuleResponse;
import software.amazon.awssdk.services.ses.model.DeleteReceiptRuleSetRequest;
import software.amazon.awssdk.services.ses.model.DeleteReceiptRuleSetResponse;
import software.amazon.awssdk.services.ses.model.DeleteTemplateRequest;
import software.amazon.awssdk.services.ses.model.DeleteTemplateResponse;
import software.amazon.awssdk.services.ses.model.DeleteVerifiedEmailAddressRequest;
import software.amazon.awssdk.services.ses.model.DeleteVerifiedEmailAddressResponse;
import software.amazon.awssdk.services.ses.model.DescribeActiveReceiptRuleSetRequest;
import software.amazon.awssdk.services.ses.model.DescribeActiveReceiptRuleSetResponse;
import software.amazon.awssdk.services.ses.model.DescribeConfigurationSetRequest;
import software.amazon.awssdk.services.ses.model.DescribeConfigurationSetResponse;
import software.amazon.awssdk.services.ses.model.DescribeReceiptRuleRequest;
import software.amazon.awssdk.services.ses.model.DescribeReceiptRuleResponse;
import software.amazon.awssdk.services.ses.model.DescribeReceiptRuleSetRequest;
import software.amazon.awssdk.services.ses.model.DescribeReceiptRuleSetResponse;
import software.amazon.awssdk.services.ses.model.EventDestinationAlreadyExistsException;
import software.amazon.awssdk.services.ses.model.EventDestinationDoesNotExistException;
import software.amazon.awssdk.services.ses.model.FromEmailAddressNotVerifiedException;
import software.amazon.awssdk.services.ses.model.GetAccountSendingEnabledRequest;
import software.amazon.awssdk.services.ses.model.GetAccountSendingEnabledResponse;
import software.amazon.awssdk.services.ses.model.GetCustomVerificationEmailTemplateRequest;
import software.amazon.awssdk.services.ses.model.GetCustomVerificationEmailTemplateResponse;
import software.amazon.awssdk.services.ses.model.GetIdentityDkimAttributesRequest;
import software.amazon.awssdk.services.ses.model.GetIdentityDkimAttributesResponse;
import software.amazon.awssdk.services.ses.model.GetIdentityMailFromDomainAttributesRequest;
import software.amazon.awssdk.services.ses.model.GetIdentityMailFromDomainAttributesResponse;
import software.amazon.awssdk.services.ses.model.GetIdentityNotificationAttributesRequest;
import software.amazon.awssdk.services.ses.model.GetIdentityNotificationAttributesResponse;
import software.amazon.awssdk.services.ses.model.GetIdentityPoliciesRequest;
import software.amazon.awssdk.services.ses.model.GetIdentityPoliciesResponse;
import software.amazon.awssdk.services.ses.model.GetIdentityVerificationAttributesRequest;
import software.amazon.awssdk.services.ses.model.GetIdentityVerificationAttributesResponse;
import software.amazon.awssdk.services.ses.model.GetSendQuotaRequest;
import software.amazon.awssdk.services.ses.model.GetSendQuotaResponse;
import software.amazon.awssdk.services.ses.model.GetSendStatisticsRequest;
import software.amazon.awssdk.services.ses.model.GetSendStatisticsResponse;
import software.amazon.awssdk.services.ses.model.GetTemplateRequest;
import software.amazon.awssdk.services.ses.model.GetTemplateResponse;
import software.amazon.awssdk.services.ses.model.InvalidCloudWatchDestinationException;
import software.amazon.awssdk.services.ses.model.InvalidConfigurationSetException;
import software.amazon.awssdk.services.ses.model.InvalidDeliveryOptionsException;
import software.amazon.awssdk.services.ses.model.InvalidFirehoseDestinationException;
import software.amazon.awssdk.services.ses.model.InvalidLambdaFunctionException;
import software.amazon.awssdk.services.ses.model.InvalidPolicyException;
import software.amazon.awssdk.services.ses.model.InvalidRenderingParameterException;
import software.amazon.awssdk.services.ses.model.InvalidS3ConfigurationException;
import software.amazon.awssdk.services.ses.model.InvalidSnsDestinationException;
import software.amazon.awssdk.services.ses.model.InvalidSnsTopicException;
import software.amazon.awssdk.services.ses.model.InvalidTemplateException;
import software.amazon.awssdk.services.ses.model.InvalidTrackingOptionsException;
import software.amazon.awssdk.services.ses.model.LimitExceededException;
import software.amazon.awssdk.services.ses.model.ListConfigurationSetsRequest;
import software.amazon.awssdk.services.ses.model.ListConfigurationSetsResponse;
import software.amazon.awssdk.services.ses.model.ListCustomVerificationEmailTemplatesRequest;
import software.amazon.awssdk.services.ses.model.ListCustomVerificationEmailTemplatesResponse;
import software.amazon.awssdk.services.ses.model.ListIdentitiesRequest;
import software.amazon.awssdk.services.ses.model.ListIdentitiesResponse;
import software.amazon.awssdk.services.ses.model.ListIdentityPoliciesRequest;
import software.amazon.awssdk.services.ses.model.ListIdentityPoliciesResponse;
import software.amazon.awssdk.services.ses.model.ListReceiptFiltersRequest;
import software.amazon.awssdk.services.ses.model.ListReceiptFiltersResponse;
import software.amazon.awssdk.services.ses.model.ListReceiptRuleSetsRequest;
import software.amazon.awssdk.services.ses.model.ListReceiptRuleSetsResponse;
import software.amazon.awssdk.services.ses.model.ListTemplatesRequest;
import software.amazon.awssdk.services.ses.model.ListTemplatesResponse;
import software.amazon.awssdk.services.ses.model.ListVerifiedEmailAddressesRequest;
import software.amazon.awssdk.services.ses.model.ListVerifiedEmailAddressesResponse;
import software.amazon.awssdk.services.ses.model.MailFromDomainNotVerifiedException;
import software.amazon.awssdk.services.ses.model.MessageRejectedException;
import software.amazon.awssdk.services.ses.model.MissingRenderingAttributeException;
import software.amazon.awssdk.services.ses.model.ProductionAccessNotGrantedException;
import software.amazon.awssdk.services.ses.model.PutConfigurationSetDeliveryOptionsRequest;
import software.amazon.awssdk.services.ses.model.PutConfigurationSetDeliveryOptionsResponse;
import software.amazon.awssdk.services.ses.model.PutIdentityPolicyRequest;
import software.amazon.awssdk.services.ses.model.PutIdentityPolicyResponse;
import software.amazon.awssdk.services.ses.model.ReorderReceiptRuleSetRequest;
import software.amazon.awssdk.services.ses.model.ReorderReceiptRuleSetResponse;
import software.amazon.awssdk.services.ses.model.RuleDoesNotExistException;
import software.amazon.awssdk.services.ses.model.RuleSetDoesNotExistException;
import software.amazon.awssdk.services.ses.model.SendBounceRequest;
import software.amazon.awssdk.services.ses.model.SendBounceResponse;
import software.amazon.awssdk.services.ses.model.SendBulkTemplatedEmailRequest;
import software.amazon.awssdk.services.ses.model.SendBulkTemplatedEmailResponse;
import software.amazon.awssdk.services.ses.model.SendCustomVerificationEmailRequest;
import software.amazon.awssdk.services.ses.model.SendCustomVerificationEmailResponse;
import software.amazon.awssdk.services.ses.model.SendEmailRequest;
import software.amazon.awssdk.services.ses.model.SendEmailResponse;
import software.amazon.awssdk.services.ses.model.SendRawEmailRequest;
import software.amazon.awssdk.services.ses.model.SendRawEmailResponse;
import software.amazon.awssdk.services.ses.model.SendTemplatedEmailRequest;
import software.amazon.awssdk.services.ses.model.SendTemplatedEmailResponse;
import software.amazon.awssdk.services.ses.model.SesException;
import software.amazon.awssdk.services.ses.model.SetActiveReceiptRuleSetRequest;
import software.amazon.awssdk.services.ses.model.SetActiveReceiptRuleSetResponse;
import software.amazon.awssdk.services.ses.model.SetIdentityDkimEnabledRequest;
import software.amazon.awssdk.services.ses.model.SetIdentityDkimEnabledResponse;
import software.amazon.awssdk.services.ses.model.SetIdentityFeedbackForwardingEnabledRequest;
import software.amazon.awssdk.services.ses.model.SetIdentityFeedbackForwardingEnabledResponse;
import software.amazon.awssdk.services.ses.model.SetIdentityHeadersInNotificationsEnabledRequest;
import software.amazon.awssdk.services.ses.model.SetIdentityHeadersInNotificationsEnabledResponse;
import software.amazon.awssdk.services.ses.model.SetIdentityMailFromDomainRequest;
import software.amazon.awssdk.services.ses.model.SetIdentityMailFromDomainResponse;
import software.amazon.awssdk.services.ses.model.SetIdentityNotificationTopicRequest;
import software.amazon.awssdk.services.ses.model.SetIdentityNotificationTopicResponse;
import software.amazon.awssdk.services.ses.model.SetReceiptRulePositionRequest;
import software.amazon.awssdk.services.ses.model.SetReceiptRulePositionResponse;
import software.amazon.awssdk.services.ses.model.TemplateDoesNotExistException;
import software.amazon.awssdk.services.ses.model.TestRenderTemplateRequest;
import software.amazon.awssdk.services.ses.model.TestRenderTemplateResponse;
import software.amazon.awssdk.services.ses.model.TrackingOptionsAlreadyExistsException;
import software.amazon.awssdk.services.ses.model.TrackingOptionsDoesNotExistException;
import software.amazon.awssdk.services.ses.model.UpdateAccountSendingEnabledRequest;
import software.amazon.awssdk.services.ses.model.UpdateAccountSendingEnabledResponse;
import software.amazon.awssdk.services.ses.model.UpdateConfigurationSetEventDestinationRequest;
import software.amazon.awssdk.services.ses.model.UpdateConfigurationSetEventDestinationResponse;
import software.amazon.awssdk.services.ses.model.UpdateConfigurationSetReputationMetricsEnabledRequest;
import software.amazon.awssdk.services.ses.model.UpdateConfigurationSetReputationMetricsEnabledResponse;
import software.amazon.awssdk.services.ses.model.UpdateConfigurationSetSendingEnabledRequest;
import software.amazon.awssdk.services.ses.model.UpdateConfigurationSetSendingEnabledResponse;
import software.amazon.awssdk.services.ses.model.UpdateConfigurationSetTrackingOptionsRequest;
import software.amazon.awssdk.services.ses.model.UpdateConfigurationSetTrackingOptionsResponse;
import software.amazon.awssdk.services.ses.model.UpdateCustomVerificationEmailTemplateRequest;
import software.amazon.awssdk.services.ses.model.UpdateCustomVerificationEmailTemplateResponse;
import software.amazon.awssdk.services.ses.model.UpdateReceiptRuleRequest;
import software.amazon.awssdk.services.ses.model.UpdateReceiptRuleResponse;
import software.amazon.awssdk.services.ses.model.UpdateTemplateRequest;
import software.amazon.awssdk.services.ses.model.UpdateTemplateResponse;
import software.amazon.awssdk.services.ses.model.VerifyDomainDkimRequest;
import software.amazon.awssdk.services.ses.model.VerifyDomainDkimResponse;
import software.amazon.awssdk.services.ses.model.VerifyDomainIdentityRequest;
import software.amazon.awssdk.services.ses.model.VerifyDomainIdentityResponse;
import software.amazon.awssdk.services.ses.model.VerifyEmailAddressRequest;
import software.amazon.awssdk.services.ses.model.VerifyEmailAddressResponse;
import software.amazon.awssdk.services.ses.model.VerifyEmailIdentityRequest;
import software.amazon.awssdk.services.ses.model.VerifyEmailIdentityResponse;
import software.amazon.awssdk.services.ses.transform.CloneReceiptRuleSetRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.CreateConfigurationSetEventDestinationRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.CreateConfigurationSetRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.CreateConfigurationSetTrackingOptionsRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.CreateCustomVerificationEmailTemplateRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.CreateReceiptFilterRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.CreateReceiptRuleRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.CreateReceiptRuleSetRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.CreateTemplateRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteConfigurationSetEventDestinationRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteConfigurationSetRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteConfigurationSetTrackingOptionsRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteCustomVerificationEmailTemplateRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteIdentityPolicyRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteIdentityRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteReceiptFilterRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteReceiptRuleRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteReceiptRuleSetRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteTemplateRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DeleteVerifiedEmailAddressRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DescribeActiveReceiptRuleSetRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DescribeConfigurationSetRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DescribeReceiptRuleRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.DescribeReceiptRuleSetRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.GetAccountSendingEnabledRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.GetCustomVerificationEmailTemplateRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.GetIdentityDkimAttributesRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.GetIdentityMailFromDomainAttributesRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.GetIdentityNotificationAttributesRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.GetIdentityPoliciesRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.GetIdentityVerificationAttributesRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.GetSendQuotaRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.GetSendStatisticsRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.GetTemplateRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.ListConfigurationSetsRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.ListCustomVerificationEmailTemplatesRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.ListIdentitiesRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.ListIdentityPoliciesRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.ListReceiptFiltersRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.ListReceiptRuleSetsRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.ListTemplatesRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.ListVerifiedEmailAddressesRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.PutConfigurationSetDeliveryOptionsRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.PutIdentityPolicyRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.ReorderReceiptRuleSetRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SendBounceRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SendBulkTemplatedEmailRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SendCustomVerificationEmailRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SendEmailRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SendRawEmailRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SendTemplatedEmailRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SetActiveReceiptRuleSetRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SetIdentityDkimEnabledRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SetIdentityFeedbackForwardingEnabledRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SetIdentityHeadersInNotificationsEnabledRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SetIdentityMailFromDomainRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SetIdentityNotificationTopicRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.SetReceiptRulePositionRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.TestRenderTemplateRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.UpdateAccountSendingEnabledRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.UpdateConfigurationSetEventDestinationRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.UpdateConfigurationSetReputationMetricsEnabledRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.UpdateConfigurationSetSendingEnabledRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.UpdateConfigurationSetTrackingOptionsRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.UpdateCustomVerificationEmailTemplateRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.UpdateReceiptRuleRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.UpdateTemplateRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.VerifyDomainDkimRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.VerifyDomainIdentityRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.VerifyEmailAddressRequestMarshaller;
import software.amazon.awssdk.services.ses.transform.VerifyEmailIdentityRequestMarshaller;
import software.amazon.awssdk.services.ses.waiters.SesAsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link SesAsyncClient}.
*
* @see SesAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultSesAsyncClient implements SesAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultSesAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.QUERY).build();
private final AsyncClientHandler clientHandler;
private final AwsQueryProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultSesAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
/**
*
* Creates a receipt rule set by cloning an existing one. All receipt rules and configurations are copied to the new
* receipt rule set and are completely independent of the source rule set.
*
*
* For information about setting up rule sets, see the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param cloneReceiptRuleSetRequest
* Represents a request to create a receipt rule set by cloning an existing one. You use receipt rule sets to
* receive email with Amazon SES. For more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the CloneReceiptRuleSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RuleSetDoesNotExistException Indicates that the provided receipt rule set does not exist.
* - AlreadyExistsException Indicates that a resource could not be created because of a naming conflict.
* - LimitExceededException Indicates that a resource could not be created because of service limits. For
* a list of Amazon SES limits, see the Amazon SES Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.CloneReceiptRuleSet
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture cloneReceiptRuleSet(
CloneReceiptRuleSetRequest cloneReceiptRuleSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cloneReceiptRuleSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cloneReceiptRuleSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CloneReceiptRuleSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CloneReceiptRuleSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CloneReceiptRuleSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CloneReceiptRuleSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cloneReceiptRuleSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a configuration set.
*
*
* Configuration sets enable you to publish email sending events. For information about using configuration sets,
* see the Amazon SES Developer
* Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createConfigurationSetRequest
* Represents a request to create a configuration set. Configuration sets enable you to publish email sending
* events. For information about using configuration sets, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the CreateConfigurationSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConfigurationSetAlreadyExistsException Indicates that the configuration set could not be created
* because of a naming conflict.
* - InvalidConfigurationSetException Indicates that the configuration set is invalid. See the error
* message for details.
* - LimitExceededException Indicates that a resource could not be created because of service limits. For
* a list of Amazon SES limits, see the Amazon SES Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.CreateConfigurationSet
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createConfigurationSet(
CreateConfigurationSetRequest createConfigurationSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createConfigurationSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createConfigurationSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateConfigurationSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateConfigurationSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateConfigurationSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateConfigurationSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createConfigurationSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a configuration set event destination.
*
*
*
* When you create or update an event destination, you must provide one, and only one, destination. The destination
* can be CloudWatch, Amazon Kinesis Firehose, or Amazon Simple Notification Service (Amazon SNS).
*
*
*
* An event destination is the Amazon Web Services service to which Amazon SES publishes the email sending events
* associated with a configuration set. For information about using configuration sets, see the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createConfigurationSetEventDestinationRequest
* Represents a request to create a configuration set event destination. A configuration set event
* destination, which can be either Amazon CloudWatch or Amazon Kinesis Firehose, describes an Amazon Web
* Services service in which Amazon SES publishes the email sending events associated with a configuration
* set. For information about using configuration sets, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the CreateConfigurationSetEventDestination operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConfigurationSetDoesNotExistException Indicates that the configuration set does not exist.
* - EventDestinationAlreadyExistsException Indicates that the event destination could not be created
* because of a naming conflict.
* - InvalidCloudWatchDestinationException Indicates that the Amazon CloudWatch destination is invalid.
* See the error message for details.
* - InvalidFirehoseDestinationException Indicates that the Amazon Kinesis Firehose destination is
* invalid. See the error message for details.
* - InvalidSnsDestinationException Indicates that the Amazon Simple Notification Service (Amazon SNS)
* destination is invalid. See the error message for details.
* - LimitExceededException Indicates that a resource could not be created because of service limits. For
* a list of Amazon SES limits, see the Amazon SES Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.CreateConfigurationSetEventDestination
* @see AWS API Documentation
*/
@Override
public CompletableFuture createConfigurationSetEventDestination(
CreateConfigurationSetEventDestinationRequest createConfigurationSetEventDestinationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createConfigurationSetEventDestinationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createConfigurationSetEventDestinationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateConfigurationSetEventDestination");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateConfigurationSetEventDestinationResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateConfigurationSetEventDestination").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateConfigurationSetEventDestinationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createConfigurationSetEventDestinationRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an association between a configuration set and a custom domain for open and click event tracking.
*
*
* By default, images and links used for tracking open and click events are hosted on domains operated by Amazon
* SES. You can configure a subdomain of your own to handle these events. For information about using custom
* domains, see the Amazon SES Developer
* Guide.
*
*
* @param createConfigurationSetTrackingOptionsRequest
* Represents a request to create an open and click tracking option object in a configuration set.
* @return A Java Future containing the result of the CreateConfigurationSetTrackingOptions operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConfigurationSetDoesNotExistException Indicates that the configuration set does not exist.
* - TrackingOptionsAlreadyExistsException Indicates that the configuration set you specified already
* contains a TrackingOptions object.
* - InvalidTrackingOptionsException Indicates that the custom domain to be used for open and click
* tracking redirects is invalid. This error appears most often in the following situations:
*
* -
*
* When the tracking domain you specified is not verified in Amazon SES.
*
*
* -
*
* When the tracking domain you specified is not a valid domain or subdomain.
*
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.CreateConfigurationSetTrackingOptions
* @see AWS API Documentation
*/
@Override
public CompletableFuture createConfigurationSetTrackingOptions(
CreateConfigurationSetTrackingOptionsRequest createConfigurationSetTrackingOptionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createConfigurationSetTrackingOptionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createConfigurationSetTrackingOptionsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateConfigurationSetTrackingOptions");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateConfigurationSetTrackingOptionsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateConfigurationSetTrackingOptions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateConfigurationSetTrackingOptionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createConfigurationSetTrackingOptionsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new custom verification email template.
*
*
* For more information about custom verification email templates, see Using
* Custom Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createCustomVerificationEmailTemplateRequest
* Represents a request to create a custom verification email template.
* @return A Java Future containing the result of the CreateCustomVerificationEmailTemplate operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CustomVerificationEmailTemplateAlreadyExistsException Indicates that a custom verification email
* template with the name you specified already exists.
* - FromEmailAddressNotVerifiedException Indicates that the sender address specified for a custom
* verification email is not verified, and is therefore not eligible to send the custom verification email.
* - CustomVerificationEmailInvalidContentException Indicates that custom verification email template
* provided content is invalid.
* - LimitExceededException Indicates that a resource could not be created because of service limits. For
* a list of Amazon SES limits, see the Amazon SES Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.CreateCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
@Override
public CompletableFuture createCustomVerificationEmailTemplate(
CreateCustomVerificationEmailTemplateRequest createCustomVerificationEmailTemplateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createCustomVerificationEmailTemplateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createCustomVerificationEmailTemplateRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCustomVerificationEmailTemplate");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateCustomVerificationEmailTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateCustomVerificationEmailTemplate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateCustomVerificationEmailTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createCustomVerificationEmailTemplateRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new IP address filter.
*
*
* For information about setting up IP address filters, see the Amazon SES
* Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createReceiptFilterRequest
* Represents a request to create a new IP address filter. You use IP address filters when you receive email
* with Amazon SES. For more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the CreateReceiptFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException Indicates that a resource could not be created because of service limits. For
* a list of Amazon SES limits, see the Amazon SES Developer Guide.
* - AlreadyExistsException Indicates that a resource could not be created because of a naming conflict.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.CreateReceiptFilter
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createReceiptFilter(
CreateReceiptFilterRequest createReceiptFilterRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createReceiptFilterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createReceiptFilterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateReceiptFilter");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateReceiptFilterResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateReceiptFilter").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateReceiptFilterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createReceiptFilterRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a receipt rule.
*
*
* For information about setting up receipt rules, see the Amazon
* SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createReceiptRuleRequest
* Represents a request to create a receipt rule. You use receipt rules to receive email with Amazon SES. For
* more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the CreateReceiptRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidSnsTopicException Indicates that the provided Amazon SNS topic is invalid, or that Amazon SES
* could not publish to the topic, possibly due to permissions issues. For information about giving
* permissions, see the Amazon SES
* Developer Guide.
* - InvalidS3ConfigurationException Indicates that the provided Amazon S3 bucket or Amazon Web Services
* KMS encryption key is invalid, or that Amazon SES could not publish to the bucket, possibly due to
* permissions issues. For information about giving permissions, see the Amazon SES
* Developer Guide.
* - InvalidLambdaFunctionException Indicates that the provided Amazon Web Services Lambda function is
* invalid, or that Amazon SES could not execute the provided function, possibly due to permissions issues.
* For information about giving permissions, see the Amazon SES
* Developer Guide.
* - AlreadyExistsException Indicates that a resource could not be created because of a naming conflict.
* - RuleDoesNotExistException Indicates that the provided receipt rule does not exist.
* - RuleSetDoesNotExistException Indicates that the provided receipt rule set does not exist.
* - LimitExceededException Indicates that a resource could not be created because of service limits. For
* a list of Amazon SES limits, see the Amazon SES Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.CreateReceiptRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createReceiptRule(CreateReceiptRuleRequest createReceiptRuleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createReceiptRuleRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createReceiptRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateReceiptRule");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateReceiptRuleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateReceiptRule").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateReceiptRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createReceiptRuleRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an empty receipt rule set.
*
*
* For information about setting up receipt rule sets, see the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createReceiptRuleSetRequest
* Represents a request to create an empty receipt rule set. You use receipt rule sets to receive email with
* Amazon SES. For more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the CreateReceiptRuleSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException Indicates that a resource could not be created because of a naming conflict.
* - LimitExceededException Indicates that a resource could not be created because of service limits. For
* a list of Amazon SES limits, see the Amazon SES Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.CreateReceiptRuleSet
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createReceiptRuleSet(
CreateReceiptRuleSetRequest createReceiptRuleSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createReceiptRuleSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createReceiptRuleSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateReceiptRuleSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateReceiptRuleSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateReceiptRuleSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateReceiptRuleSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createReceiptRuleSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an email template. Email templates enable you to send personalized email to one or more destinations in a
* single operation. For more information, see the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param createTemplateRequest
* Represents a request to create an email template. For more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the CreateTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AlreadyExistsException Indicates that a resource could not be created because of a naming conflict.
* - InvalidTemplateException Indicates that the template that you specified could not be rendered. This
* issue may occur when a template refers to a partial that does not exist.
* - LimitExceededException Indicates that a resource could not be created because of service limits. For
* a list of Amazon SES limits, see the Amazon SES Developer Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.CreateTemplate
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createTemplate(CreateTemplateRequest createTemplateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createTemplateRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateTemplate");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(CreateTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateTemplate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createTemplateRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a configuration set. Configuration sets enable you to publish email sending events. For information about
* using configuration sets, see the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteConfigurationSetRequest
* Represents a request to delete a configuration set. Configuration sets enable you to publish email sending
* events. For information about using configuration sets, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the DeleteConfigurationSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConfigurationSetDoesNotExistException Indicates that the configuration set does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteConfigurationSet
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteConfigurationSet(
DeleteConfigurationSetRequest deleteConfigurationSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteConfigurationSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteConfigurationSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteConfigurationSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteConfigurationSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteConfigurationSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteConfigurationSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteConfigurationSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a configuration set event destination. Configuration set event destinations are associated with
* configuration sets, which enable you to publish email sending events. For information about using configuration
* sets, see the Amazon SES
* Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteConfigurationSetEventDestinationRequest
* Represents a request to delete a configuration set event destination. Configuration set event destinations
* are associated with configuration sets, which enable you to publish email sending events. For information
* about using configuration sets, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the DeleteConfigurationSetEventDestination operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConfigurationSetDoesNotExistException Indicates that the configuration set does not exist.
* - EventDestinationDoesNotExistException Indicates that the event destination does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteConfigurationSetEventDestination
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteConfigurationSetEventDestination(
DeleteConfigurationSetEventDestinationRequest deleteConfigurationSetEventDestinationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteConfigurationSetEventDestinationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteConfigurationSetEventDestinationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteConfigurationSetEventDestination");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteConfigurationSetEventDestinationResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteConfigurationSetEventDestination").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteConfigurationSetEventDestinationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteConfigurationSetEventDestinationRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an association between a configuration set and a custom domain for open and click event tracking.
*
*
* By default, images and links used for tracking open and click events are hosted on domains operated by Amazon
* SES. You can configure a subdomain of your own to handle these events. For information about using custom
* domains, see the Amazon SES Developer
* Guide.
*
*
*
* Deleting this kind of association results in emails sent using the specified configuration set to capture open
* and click events using the standard, Amazon SES-operated domains.
*
*
*
* @param deleteConfigurationSetTrackingOptionsRequest
* Represents a request to delete open and click tracking options in a configuration set.
* @return A Java Future containing the result of the DeleteConfigurationSetTrackingOptions operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConfigurationSetDoesNotExistException Indicates that the configuration set does not exist.
* - TrackingOptionsDoesNotExistException Indicates that the TrackingOptions object you specified does not
* exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteConfigurationSetTrackingOptions
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteConfigurationSetTrackingOptions(
DeleteConfigurationSetTrackingOptionsRequest deleteConfigurationSetTrackingOptionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteConfigurationSetTrackingOptionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteConfigurationSetTrackingOptionsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteConfigurationSetTrackingOptions");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteConfigurationSetTrackingOptionsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteConfigurationSetTrackingOptions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteConfigurationSetTrackingOptionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteConfigurationSetTrackingOptionsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an existing custom verification email template.
*
*
* For more information about custom verification email templates, see Using
* Custom Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteCustomVerificationEmailTemplateRequest
* Represents a request to delete an existing custom verification email template.
* @return A Java Future containing the result of the DeleteCustomVerificationEmailTemplate operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteCustomVerificationEmailTemplate(
DeleteCustomVerificationEmailTemplateRequest deleteCustomVerificationEmailTemplateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteCustomVerificationEmailTemplateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteCustomVerificationEmailTemplateRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCustomVerificationEmailTemplate");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteCustomVerificationEmailTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteCustomVerificationEmailTemplate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteCustomVerificationEmailTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteCustomVerificationEmailTemplateRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified identity (an email address or a domain) from the list of verified identities.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteIdentityRequest
* Represents a request to delete one of your Amazon SES identities (an email address or domain).
* @return A Java Future containing the result of the DeleteIdentity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteIdentity
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteIdentity(DeleteIdentityRequest deleteIdentityRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteIdentityRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteIdentityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteIdentity");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteIdentityResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteIdentity").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteIdentityRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteIdentityRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified sending authorization policy for the given identity (an email address or a domain). This
* operation returns successfully even if a policy with the specified name does not exist.
*
*
*
* This operation is for the identity owner only. If you have not verified the identity, it returns an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteIdentityPolicyRequest
* Represents a request to delete a sending authorization policy for an identity. Sending authorization is an
* Amazon SES feature that enables you to authorize other senders to use your identities. For information,
* see the Amazon SES
* Developer Guide.
* @return A Java Future containing the result of the DeleteIdentityPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteIdentityPolicy
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteIdentityPolicy(
DeleteIdentityPolicyRequest deleteIdentityPolicyRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteIdentityPolicyRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteIdentityPolicyRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteIdentityPolicy");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteIdentityPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteIdentityPolicy").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteIdentityPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteIdentityPolicyRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified IP address filter.
*
*
* For information about managing IP address filters, see the Amazon SES
* Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteReceiptFilterRequest
* Represents a request to delete an IP address filter. You use IP address filters when you receive email
* with Amazon SES. For more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the DeleteReceiptFilter operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteReceiptFilter
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteReceiptFilter(
DeleteReceiptFilterRequest deleteReceiptFilterRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteReceiptFilterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteReceiptFilterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteReceiptFilter");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteReceiptFilterResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteReceiptFilter").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteReceiptFilterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteReceiptFilterRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified receipt rule.
*
*
* For information about managing receipt rules, see the Amazon
* SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteReceiptRuleRequest
* Represents a request to delete a receipt rule. You use receipt rules to receive email with Amazon SES. For
* more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the DeleteReceiptRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RuleSetDoesNotExistException Indicates that the provided receipt rule set does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteReceiptRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteReceiptRule(DeleteReceiptRuleRequest deleteReceiptRuleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteReceiptRuleRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteReceiptRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteReceiptRule");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteReceiptRuleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteReceiptRule").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteReceiptRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteReceiptRuleRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified receipt rule set and all of the receipt rules it contains.
*
*
*
* The currently active rule set cannot be deleted.
*
*
*
* For information about managing receipt rule sets, see the Amazon
* SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteReceiptRuleSetRequest
* Represents a request to delete a receipt rule set and all of the receipt rules it contains. You use
* receipt rule sets to receive email with Amazon SES. For more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the DeleteReceiptRuleSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CannotDeleteException Indicates that the delete operation could not be completed.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteReceiptRuleSet
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteReceiptRuleSet(
DeleteReceiptRuleSetRequest deleteReceiptRuleSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteReceiptRuleSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteReceiptRuleSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteReceiptRuleSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteReceiptRuleSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteReceiptRuleSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteReceiptRuleSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteReceiptRuleSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an email template.
*
*
* You can execute this operation no more than once per second.
*
*
* @param deleteTemplateRequest
* Represents a request to delete an email template. For more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the DeleteTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteTemplate
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteTemplate(DeleteTemplateRequest deleteTemplateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteTemplateRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteTemplate");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteTemplate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteTemplateRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deprecated. Use the DeleteIdentity
operation to delete email addresses and domains.
*
*
* @param deleteVerifiedEmailAddressRequest
* Represents a request to delete an email address from the list of email addresses you have attempted to
* verify under your Amazon Web Services account.
* @return A Java Future containing the result of the DeleteVerifiedEmailAddress operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DeleteVerifiedEmailAddress
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteVerifiedEmailAddress(
DeleteVerifiedEmailAddressRequest deleteVerifiedEmailAddressRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteVerifiedEmailAddressRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVerifiedEmailAddressRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVerifiedEmailAddress");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DeleteVerifiedEmailAddressResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteVerifiedEmailAddress").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteVerifiedEmailAddressRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteVerifiedEmailAddressRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the metadata and receipt rules for the receipt rule set that is currently active.
*
*
* For information about setting up receipt rule sets, see the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param describeActiveReceiptRuleSetRequest
* Represents a request to return the metadata and receipt rules for the receipt rule set that is currently
* active. You use receipt rule sets to receive email with Amazon SES. For more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the DescribeActiveReceiptRuleSet operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DescribeActiveReceiptRuleSet
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeActiveReceiptRuleSet(
DescribeActiveReceiptRuleSetRequest describeActiveReceiptRuleSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeActiveReceiptRuleSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeActiveReceiptRuleSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeActiveReceiptRuleSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeActiveReceiptRuleSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeActiveReceiptRuleSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeActiveReceiptRuleSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeActiveReceiptRuleSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the details of the specified configuration set. For information about using configuration sets, see the
* Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param describeConfigurationSetRequest
* Represents a request to return the details of a configuration set. Configuration sets enable you to
* publish email sending events. For information about using configuration sets, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the DescribeConfigurationSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConfigurationSetDoesNotExistException Indicates that the configuration set does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DescribeConfigurationSet
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeConfigurationSet(
DescribeConfigurationSetRequest describeConfigurationSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeConfigurationSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeConfigurationSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeConfigurationSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeConfigurationSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeConfigurationSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeConfigurationSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeConfigurationSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the details of the specified receipt rule.
*
*
* For information about setting up receipt rules, see the Amazon
* SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param describeReceiptRuleRequest
* Represents a request to return the details of a receipt rule. You use receipt rules to receive email with
* Amazon SES. For more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the DescribeReceiptRule operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RuleDoesNotExistException Indicates that the provided receipt rule does not exist.
* - RuleSetDoesNotExistException Indicates that the provided receipt rule set does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DescribeReceiptRule
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeReceiptRule(
DescribeReceiptRuleRequest describeReceiptRuleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeReceiptRuleRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeReceiptRuleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReceiptRule");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeReceiptRuleResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeReceiptRule").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeReceiptRuleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeReceiptRuleRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the details of the specified receipt rule set.
*
*
* For information about managing receipt rule sets, see the Amazon
* SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param describeReceiptRuleSetRequest
* Represents a request to return the details of a receipt rule set. You use receipt rule sets to receive
* email with Amazon SES. For more information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the DescribeReceiptRuleSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RuleSetDoesNotExistException Indicates that the provided receipt rule set does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.DescribeReceiptRuleSet
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeReceiptRuleSet(
DescribeReceiptRuleSetRequest describeReceiptRuleSetRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeReceiptRuleSetRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeReceiptRuleSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReceiptRuleSet");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(DescribeReceiptRuleSetResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeReceiptRuleSet").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeReceiptRuleSetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeReceiptRuleSetRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the email sending status of the Amazon SES account for the current Region.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getAccountSendingEnabledRequest
* @return A Java Future containing the result of the GetAccountSendingEnabled operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.GetAccountSendingEnabled
* @see AWS API Documentation
*/
@Override
public CompletableFuture getAccountSendingEnabled(
GetAccountSendingEnabledRequest getAccountSendingEnabledRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAccountSendingEnabledRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAccountSendingEnabledRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccountSendingEnabled");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetAccountSendingEnabledResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAccountSendingEnabled").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetAccountSendingEnabledRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getAccountSendingEnabledRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the custom email verification template for the template name you specify.
*
*
* For more information about custom verification email templates, see Using
* Custom Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getCustomVerificationEmailTemplateRequest
* Represents a request to retrieve an existing custom verification email template.
* @return A Java Future containing the result of the GetCustomVerificationEmailTemplate operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - CustomVerificationEmailTemplateDoesNotExistException Indicates that a custom verification email
* template with the name you specified does not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.GetCustomVerificationEmailTemplate
* @see AWS API Documentation
*/
@Override
public CompletableFuture getCustomVerificationEmailTemplate(
GetCustomVerificationEmailTemplateRequest getCustomVerificationEmailTemplateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getCustomVerificationEmailTemplateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getCustomVerificationEmailTemplateRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCustomVerificationEmailTemplate");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetCustomVerificationEmailTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetCustomVerificationEmailTemplate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetCustomVerificationEmailTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getCustomVerificationEmailTemplateRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the current status of Easy DKIM signing for an entity. For domain name identities, this operation also
* returns the DKIM tokens that are required for Easy DKIM signing, and whether Amazon SES has successfully verified
* that these tokens have been published.
*
*
* This operation takes a list of identities as input and returns the following information for each:
*
*
* -
*
* Whether Easy DKIM signing is enabled or disabled.
*
*
* -
*
* A set of DKIM tokens that represent the identity. If the identity is an email address, the tokens represent the
* domain of that address.
*
*
* -
*
* Whether Amazon SES has successfully verified the DKIM tokens published in the domain's DNS. This information is
* only returned for domain name identities, not for email addresses.
*
*
*
*
* This operation is throttled at one request per second and can only get DKIM attributes for up to 100 identities
* at a time.
*
*
* For more information about creating DNS records using DKIM tokens, go to the Amazon SES
* Developer Guide.
*
*
* @param getIdentityDkimAttributesRequest
* Represents a request for the status of Amazon SES Easy DKIM signing for an identity. For domain
* identities, this request also returns the DKIM tokens that are required for Easy DKIM signing, and whether
* Amazon SES successfully verified that these tokens were published. For more information about Easy DKIM,
* see the Amazon SES
* Developer Guide.
* @return A Java Future containing the result of the GetIdentityDkimAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.GetIdentityDkimAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture getIdentityDkimAttributes(
GetIdentityDkimAttributesRequest getIdentityDkimAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getIdentityDkimAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getIdentityDkimAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetIdentityDkimAttributes");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetIdentityDkimAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetIdentityDkimAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetIdentityDkimAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getIdentityDkimAttributesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the custom MAIL FROM attributes for a list of identities (email addresses : domains).
*
*
* This operation is throttled at one request per second and can only get custom MAIL FROM attributes for up to 100
* identities at a time.
*
*
* @param getIdentityMailFromDomainAttributesRequest
* Represents a request to return the Amazon SES custom MAIL FROM attributes for a list of identities. For
* information about using a custom MAIL FROM domain, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the GetIdentityMailFromDomainAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.GetIdentityMailFromDomainAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture getIdentityMailFromDomainAttributes(
GetIdentityMailFromDomainAttributesRequest getIdentityMailFromDomainAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getIdentityMailFromDomainAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getIdentityMailFromDomainAttributesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetIdentityMailFromDomainAttributes");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetIdentityMailFromDomainAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetIdentityMailFromDomainAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetIdentityMailFromDomainAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getIdentityMailFromDomainAttributesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Given a list of verified identities (email addresses and/or domains), returns a structure describing identity
* notification attributes.
*
*
* This operation is throttled at one request per second and can only get notification attributes for up to 100
* identities at a time.
*
*
* For more information about using notifications with Amazon SES, see the Amazon SES
* Developer Guide.
*
*
* @param getIdentityNotificationAttributesRequest
* Represents a request to return the notification attributes for a list of identities you verified with
* Amazon SES. For information about Amazon SES notifications, see the Amazon
* SES Developer Guide.
* @return A Java Future containing the result of the GetIdentityNotificationAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.GetIdentityNotificationAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture getIdentityNotificationAttributes(
GetIdentityNotificationAttributesRequest getIdentityNotificationAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getIdentityNotificationAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getIdentityNotificationAttributesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetIdentityNotificationAttributes");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetIdentityNotificationAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetIdentityNotificationAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetIdentityNotificationAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getIdentityNotificationAttributesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the requested sending authorization policies for the given identity (an email address or a domain). The
* policies are returned as a map of policy names to policy contents. You can retrieve a maximum of 20 policies at a
* time.
*
*
*
* This operation is for the identity owner only. If you have not verified the identity, it returns an error.
*
*
*
* Sending authorization is a feature that enables an identity owner to authorize other senders to use its
* identities. For information about using sending authorization, see the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getIdentityPoliciesRequest
* Represents a request to return the requested sending authorization policies for an identity. Sending
* authorization is an Amazon SES feature that enables you to authorize other senders to use your identities.
* For information, see the Amazon SES Developer
* Guide.
* @return A Java Future containing the result of the GetIdentityPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.GetIdentityPolicies
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getIdentityPolicies(
GetIdentityPoliciesRequest getIdentityPoliciesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getIdentityPoliciesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getIdentityPoliciesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetIdentityPolicies");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetIdentityPoliciesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetIdentityPolicies").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetIdentityPoliciesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getIdentityPoliciesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Given a list of identities (email addresses and/or domains), returns the verification status and (for domain
* identities) the verification token for each identity.
*
*
* The verification status of an email address is "Pending" until the email address owner clicks the link within the
* verification email that Amazon SES sent to that address. If the email address owner clicks the link within 24
* hours, the verification status of the email address changes to "Success". If the link is not clicked within 24
* hours, the verification status changes to "Failed." In that case, to verify the email address, you must restart
* the verification process from the beginning.
*
*
* For domain identities, the domain's verification status is "Pending" as Amazon SES searches for the required TXT
* record in the DNS settings of the domain. When Amazon SES detects the record, the domain's verification status
* changes to "Success". If Amazon SES is unable to detect the record within 72 hours, the domain's verification
* status changes to "Failed." In that case, to verify the domain, you must restart the verification process from
* the beginning.
*
*
* This operation is throttled at one request per second and can only get verification attributes for up to 100
* identities at a time.
*
*
* @param getIdentityVerificationAttributesRequest
* Represents a request to return the Amazon SES verification status of a list of identities. For domain
* identities, this request also returns the verification token. For information about verifying identities
* with Amazon SES, see the Amazon SES Developer Guide.
* @return A Java Future containing the result of the GetIdentityVerificationAttributes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.GetIdentityVerificationAttributes
* @see AWS API Documentation
*/
@Override
public CompletableFuture getIdentityVerificationAttributes(
GetIdentityVerificationAttributesRequest getIdentityVerificationAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getIdentityVerificationAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getIdentityVerificationAttributesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetIdentityVerificationAttributes");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetIdentityVerificationAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetIdentityVerificationAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetIdentityVerificationAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getIdentityVerificationAttributesRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides the sending limits for the Amazon SES account.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getSendQuotaRequest
* @return A Java Future containing the result of the GetSendQuota operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.GetSendQuota
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getSendQuota(GetSendQuotaRequest getSendQuotaRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getSendQuotaRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSendQuotaRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSendQuota");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetSendQuotaResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSendQuota").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetSendQuotaRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getSendQuotaRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides sending statistics for the current Amazon Web Services Region. The result is a list of data points,
* representing the last two weeks of sending activity. Each data point in the list contains statistics for a
* 15-minute period of time.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getSendStatisticsRequest
* @return A Java Future containing the result of the GetSendStatistics operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.GetSendStatistics
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getSendStatistics(GetSendStatisticsRequest getSendStatisticsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getSendStatisticsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSendStatisticsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSendStatistics");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetSendStatisticsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSendStatistics").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetSendStatisticsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getSendStatisticsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Displays the template object (which includes the Subject line, HTML part and text part) for the template you
* specify.
*
*
* You can execute this operation no more than once per second.
*
*
* @param getTemplateRequest
* @return A Java Future containing the result of the GetTemplate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TemplateDoesNotExistException Indicates that the Template object you specified does not exist in your
* Amazon SES account.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.GetTemplate
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getTemplate(GetTemplateRequest getTemplateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getTemplateRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetTemplate");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(GetTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetTemplate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetTemplateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getTemplateRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides a list of the configuration sets associated with your Amazon SES account in the current Amazon Web
* Services Region. For information about using configuration sets, see Monitoring Your Amazon SES Sending
* Activity in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second. This operation returns up to 1,000 configuration
* sets each time it is run. If your Amazon SES account has more than 1,000 configuration sets, this operation also
* returns NextToken
. You can then execute the ListConfigurationSets
operation again,
* passing the NextToken
parameter and the value of the NextToken element to retrieve additional
* results.
*
*
* @param listConfigurationSetsRequest
* Represents a request to list the configuration sets associated with your Amazon Web Services account.
* Configuration sets enable you to publish email sending events. For information about using configuration
* sets, see the Amazon SES
* Developer Guide.
* @return A Java Future containing the result of the ListConfigurationSets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SesException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SesAsyncClient.ListConfigurationSets
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listConfigurationSets(
ListConfigurationSetsRequest listConfigurationSetsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listConfigurationSetsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listConfigurationSetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListConfigurationSets");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ListConfigurationSetsResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListConfigurationSets").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListConfigurationSetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listConfigurationSetsRequest));
CompletableFuture whenCompleteFuture = null;
whenCompleteFuture = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
return CompletableFutureUtils.forwardExceptionTo(whenCompleteFuture, executeFuture);
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the existing custom verification email templates for your account in the current Amazon Web Services
* Region.
*
*
* For more information about custom verification email templates, see Using
* Custom Verification Email Templates in the Amazon SES Developer Guide.
*
*
* You can execute this operation no more than once per second.
*
*
* @param listCustomVerificationEmailTemplatesRequest
* Represents a request to list the existing custom verification email templates for your account.
*
* For more information about custom verification email templates, see Using Custom Verification Email Templates in the Amazon SES Developer Guide.
* @return A Java Future containing the result of the ListCustomVerificationEmailTemplates operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
- SdkClientException If any client side error occurs such as
* an IO related failure, failure to get credentials, etc.
- SesException Base class for all service
* exceptions. Unknown exceptions will be thrown as an instance of this type.
*
* @sample SesAsyncClient.ListCustomVerificationEmailTemplates
* @see AWS API Documentation
*/
@Override
public CompletableFuture listCustomVerificationEmailTemplates(
ListCustomVerificationEmailTemplatesRequest listCustomVerificationEmailTemplatesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listCustomVerificationEmailTemplatesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listCustomVerificationEmailTemplatesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SES");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListCustomVerificationEmailTemplates");
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(ListCustomVerificationEmailTemplatesResponse::builder);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler();
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams