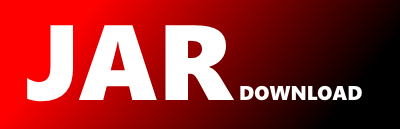
software.amazon.awssdk.services.ses.model.GetCustomVerificationEmailTemplateResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ses Show documentation
Show all versions of ses Show documentation
The AWS Java SDK for Amazon SES module holds the client classes that are used for communicating with
Amazon Simple Email
Service
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ses.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The content of the custom verification email template.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetCustomVerificationEmailTemplateResponse extends SesResponse implements
ToCopyableBuilder {
private static final SdkField TEMPLATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateName").getter(getter(GetCustomVerificationEmailTemplateResponse::templateName))
.setter(setter(Builder::templateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateName").build()).build();
private static final SdkField FROM_EMAIL_ADDRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FromEmailAddress").getter(getter(GetCustomVerificationEmailTemplateResponse::fromEmailAddress))
.setter(setter(Builder::fromEmailAddress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FromEmailAddress").build()).build();
private static final SdkField TEMPLATE_SUBJECT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateSubject").getter(getter(GetCustomVerificationEmailTemplateResponse::templateSubject))
.setter(setter(Builder::templateSubject))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateSubject").build()).build();
private static final SdkField TEMPLATE_CONTENT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TemplateContent").getter(getter(GetCustomVerificationEmailTemplateResponse::templateContent))
.setter(setter(Builder::templateContent))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TemplateContent").build()).build();
private static final SdkField SUCCESS_REDIRECTION_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SuccessRedirectionURL")
.getter(getter(GetCustomVerificationEmailTemplateResponse::successRedirectionURL))
.setter(setter(Builder::successRedirectionURL))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SuccessRedirectionURL").build())
.build();
private static final SdkField FAILURE_REDIRECTION_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureRedirectionURL")
.getter(getter(GetCustomVerificationEmailTemplateResponse::failureRedirectionURL))
.setter(setter(Builder::failureRedirectionURL))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureRedirectionURL").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TEMPLATE_NAME_FIELD,
FROM_EMAIL_ADDRESS_FIELD, TEMPLATE_SUBJECT_FIELD, TEMPLATE_CONTENT_FIELD, SUCCESS_REDIRECTION_URL_FIELD,
FAILURE_REDIRECTION_URL_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("TemplateName", TEMPLATE_NAME_FIELD);
put("FromEmailAddress", FROM_EMAIL_ADDRESS_FIELD);
put("TemplateSubject", TEMPLATE_SUBJECT_FIELD);
put("TemplateContent", TEMPLATE_CONTENT_FIELD);
put("SuccessRedirectionURL", SUCCESS_REDIRECTION_URL_FIELD);
put("FailureRedirectionURL", FAILURE_REDIRECTION_URL_FIELD);
}
});
private final String templateName;
private final String fromEmailAddress;
private final String templateSubject;
private final String templateContent;
private final String successRedirectionURL;
private final String failureRedirectionURL;
private GetCustomVerificationEmailTemplateResponse(BuilderImpl builder) {
super(builder);
this.templateName = builder.templateName;
this.fromEmailAddress = builder.fromEmailAddress;
this.templateSubject = builder.templateSubject;
this.templateContent = builder.templateContent;
this.successRedirectionURL = builder.successRedirectionURL;
this.failureRedirectionURL = builder.failureRedirectionURL;
}
/**
*
* The name of the custom verification email template.
*
*
* @return The name of the custom verification email template.
*/
public final String templateName() {
return templateName;
}
/**
*
* The email address that the custom verification email is sent from.
*
*
* @return The email address that the custom verification email is sent from.
*/
public final String fromEmailAddress() {
return fromEmailAddress;
}
/**
*
* The subject line of the custom verification email.
*
*
* @return The subject line of the custom verification email.
*/
public final String templateSubject() {
return templateSubject;
}
/**
*
* The content of the custom verification email.
*
*
* @return The content of the custom verification email.
*/
public final String templateContent() {
return templateContent;
}
/**
*
* The URL that the recipient of the verification email is sent to if his or her address is successfully verified.
*
*
* @return The URL that the recipient of the verification email is sent to if his or her address is successfully
* verified.
*/
public final String successRedirectionURL() {
return successRedirectionURL;
}
/**
*
* The URL that the recipient of the verification email is sent to if his or her address is not successfully
* verified.
*
*
* @return The URL that the recipient of the verification email is sent to if his or her address is not successfully
* verified.
*/
public final String failureRedirectionURL() {
return failureRedirectionURL;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(templateName());
hashCode = 31 * hashCode + Objects.hashCode(fromEmailAddress());
hashCode = 31 * hashCode + Objects.hashCode(templateSubject());
hashCode = 31 * hashCode + Objects.hashCode(templateContent());
hashCode = 31 * hashCode + Objects.hashCode(successRedirectionURL());
hashCode = 31 * hashCode + Objects.hashCode(failureRedirectionURL());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetCustomVerificationEmailTemplateResponse)) {
return false;
}
GetCustomVerificationEmailTemplateResponse other = (GetCustomVerificationEmailTemplateResponse) obj;
return Objects.equals(templateName(), other.templateName())
&& Objects.equals(fromEmailAddress(), other.fromEmailAddress())
&& Objects.equals(templateSubject(), other.templateSubject())
&& Objects.equals(templateContent(), other.templateContent())
&& Objects.equals(successRedirectionURL(), other.successRedirectionURL())
&& Objects.equals(failureRedirectionURL(), other.failureRedirectionURL());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetCustomVerificationEmailTemplateResponse").add("TemplateName", templateName())
.add("FromEmailAddress", fromEmailAddress()).add("TemplateSubject", templateSubject())
.add("TemplateContent", templateContent()).add("SuccessRedirectionURL", successRedirectionURL())
.add("FailureRedirectionURL", failureRedirectionURL()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TemplateName":
return Optional.ofNullable(clazz.cast(templateName()));
case "FromEmailAddress":
return Optional.ofNullable(clazz.cast(fromEmailAddress()));
case "TemplateSubject":
return Optional.ofNullable(clazz.cast(templateSubject()));
case "TemplateContent":
return Optional.ofNullable(clazz.cast(templateContent()));
case "SuccessRedirectionURL":
return Optional.ofNullable(clazz.cast(successRedirectionURL()));
case "FailureRedirectionURL":
return Optional.ofNullable(clazz.cast(failureRedirectionURL()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy