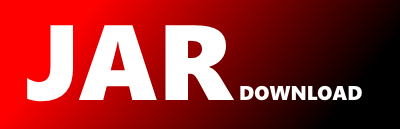
software.amazon.awssdk.services.ses.model.IdentityNotificationAttributes Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ses.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents the notification attributes of an identity, including whether an identity has Amazon Simple Notification
* Service (Amazon SNS) topics set for bounce, complaint, and/or delivery notifications, and whether feedback forwarding
* is enabled for bounce and complaint notifications.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class IdentityNotificationAttributes implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField BOUNCE_TOPIC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BounceTopic").getter(getter(IdentityNotificationAttributes::bounceTopic))
.setter(setter(Builder::bounceTopic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BounceTopic").build()).build();
private static final SdkField COMPLAINT_TOPIC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ComplaintTopic").getter(getter(IdentityNotificationAttributes::complaintTopic))
.setter(setter(Builder::complaintTopic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ComplaintTopic").build()).build();
private static final SdkField DELIVERY_TOPIC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DeliveryTopic").getter(getter(IdentityNotificationAttributes::deliveryTopic))
.setter(setter(Builder::deliveryTopic))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeliveryTopic").build()).build();
private static final SdkField FORWARDING_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ForwardingEnabled").getter(getter(IdentityNotificationAttributes::forwardingEnabled))
.setter(setter(Builder::forwardingEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ForwardingEnabled").build()).build();
private static final SdkField HEADERS_IN_BOUNCE_NOTIFICATIONS_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("HeadersInBounceNotificationsEnabled")
.getter(getter(IdentityNotificationAttributes::headersInBounceNotificationsEnabled))
.setter(setter(Builder::headersInBounceNotificationsEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("HeadersInBounceNotificationsEnabled").build()).build();
private static final SdkField HEADERS_IN_COMPLAINT_NOTIFICATIONS_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("HeadersInComplaintNotificationsEnabled")
.getter(getter(IdentityNotificationAttributes::headersInComplaintNotificationsEnabled))
.setter(setter(Builder::headersInComplaintNotificationsEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("HeadersInComplaintNotificationsEnabled").build()).build();
private static final SdkField HEADERS_IN_DELIVERY_NOTIFICATIONS_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("HeadersInDeliveryNotificationsEnabled")
.getter(getter(IdentityNotificationAttributes::headersInDeliveryNotificationsEnabled))
.setter(setter(Builder::headersInDeliveryNotificationsEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("HeadersInDeliveryNotificationsEnabled").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BOUNCE_TOPIC_FIELD,
COMPLAINT_TOPIC_FIELD, DELIVERY_TOPIC_FIELD, FORWARDING_ENABLED_FIELD, HEADERS_IN_BOUNCE_NOTIFICATIONS_ENABLED_FIELD,
HEADERS_IN_COMPLAINT_NOTIFICATIONS_ENABLED_FIELD, HEADERS_IN_DELIVERY_NOTIFICATIONS_ENABLED_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("BounceTopic", BOUNCE_TOPIC_FIELD);
put("ComplaintTopic", COMPLAINT_TOPIC_FIELD);
put("DeliveryTopic", DELIVERY_TOPIC_FIELD);
put("ForwardingEnabled", FORWARDING_ENABLED_FIELD);
put("HeadersInBounceNotificationsEnabled", HEADERS_IN_BOUNCE_NOTIFICATIONS_ENABLED_FIELD);
put("HeadersInComplaintNotificationsEnabled", HEADERS_IN_COMPLAINT_NOTIFICATIONS_ENABLED_FIELD);
put("HeadersInDeliveryNotificationsEnabled", HEADERS_IN_DELIVERY_NOTIFICATIONS_ENABLED_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String bounceTopic;
private final String complaintTopic;
private final String deliveryTopic;
private final Boolean forwardingEnabled;
private final Boolean headersInBounceNotificationsEnabled;
private final Boolean headersInComplaintNotificationsEnabled;
private final Boolean headersInDeliveryNotificationsEnabled;
private IdentityNotificationAttributes(BuilderImpl builder) {
this.bounceTopic = builder.bounceTopic;
this.complaintTopic = builder.complaintTopic;
this.deliveryTopic = builder.deliveryTopic;
this.forwardingEnabled = builder.forwardingEnabled;
this.headersInBounceNotificationsEnabled = builder.headersInBounceNotificationsEnabled;
this.headersInComplaintNotificationsEnabled = builder.headersInComplaintNotificationsEnabled;
this.headersInDeliveryNotificationsEnabled = builder.headersInDeliveryNotificationsEnabled;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SNS topic where Amazon SES publishes bounce notifications.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon SNS topic where Amazon SES publishes bounce notifications.
*/
public final String bounceTopic() {
return bounceTopic;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SNS topic where Amazon SES publishes complaint notifications.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon SNS topic where Amazon SES publishes complaint
* notifications.
*/
public final String complaintTopic() {
return complaintTopic;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SNS topic where Amazon SES publishes delivery notifications.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon SNS topic where Amazon SES publishes delivery notifications.
*/
public final String deliveryTopic() {
return deliveryTopic;
}
/**
*
* Describes whether Amazon SES forwards bounce and complaint notifications as email. true
indicates
* that Amazon SES forwards bounce and complaint notifications as email, while false
indicates that
* bounce and complaint notifications are published only to the specified bounce and complaint Amazon SNS topics.
*
*
* @return Describes whether Amazon SES forwards bounce and complaint notifications as email. true
* indicates that Amazon SES forwards bounce and complaint notifications as email, while false
* indicates that bounce and complaint notifications are published only to the specified bounce and
* complaint Amazon SNS topics.
*/
public final Boolean forwardingEnabled() {
return forwardingEnabled;
}
/**
*
* Describes whether Amazon SES includes the original email headers in Amazon SNS notifications of type
* Bounce
. A value of true
specifies that Amazon SES includes headers in bounce
* notifications, and a value of false
specifies that Amazon SES does not include headers in bounce
* notifications.
*
*
* @return Describes whether Amazon SES includes the original email headers in Amazon SNS notifications of type
* Bounce
. A value of true
specifies that Amazon SES includes headers in bounce
* notifications, and a value of false
specifies that Amazon SES does not include headers in
* bounce notifications.
*/
public final Boolean headersInBounceNotificationsEnabled() {
return headersInBounceNotificationsEnabled;
}
/**
*
* Describes whether Amazon SES includes the original email headers in Amazon SNS notifications of type
* Complaint
. A value of true
specifies that Amazon SES includes headers in complaint
* notifications, and a value of false
specifies that Amazon SES does not include headers in complaint
* notifications.
*
*
* @return Describes whether Amazon SES includes the original email headers in Amazon SNS notifications of type
* Complaint
. A value of true
specifies that Amazon SES includes headers in
* complaint notifications, and a value of false
specifies that Amazon SES does not include
* headers in complaint notifications.
*/
public final Boolean headersInComplaintNotificationsEnabled() {
return headersInComplaintNotificationsEnabled;
}
/**
*
* Describes whether Amazon SES includes the original email headers in Amazon SNS notifications of type
* Delivery
. A value of true
specifies that Amazon SES includes headers in delivery
* notifications, and a value of false
specifies that Amazon SES does not include headers in delivery
* notifications.
*
*
* @return Describes whether Amazon SES includes the original email headers in Amazon SNS notifications of type
* Delivery
. A value of true
specifies that Amazon SES includes headers in
* delivery notifications, and a value of false
specifies that Amazon SES does not include
* headers in delivery notifications.
*/
public final Boolean headersInDeliveryNotificationsEnabled() {
return headersInDeliveryNotificationsEnabled;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(bounceTopic());
hashCode = 31 * hashCode + Objects.hashCode(complaintTopic());
hashCode = 31 * hashCode + Objects.hashCode(deliveryTopic());
hashCode = 31 * hashCode + Objects.hashCode(forwardingEnabled());
hashCode = 31 * hashCode + Objects.hashCode(headersInBounceNotificationsEnabled());
hashCode = 31 * hashCode + Objects.hashCode(headersInComplaintNotificationsEnabled());
hashCode = 31 * hashCode + Objects.hashCode(headersInDeliveryNotificationsEnabled());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof IdentityNotificationAttributes)) {
return false;
}
IdentityNotificationAttributes other = (IdentityNotificationAttributes) obj;
return Objects.equals(bounceTopic(), other.bounceTopic()) && Objects.equals(complaintTopic(), other.complaintTopic())
&& Objects.equals(deliveryTopic(), other.deliveryTopic())
&& Objects.equals(forwardingEnabled(), other.forwardingEnabled())
&& Objects.equals(headersInBounceNotificationsEnabled(), other.headersInBounceNotificationsEnabled())
&& Objects.equals(headersInComplaintNotificationsEnabled(), other.headersInComplaintNotificationsEnabled())
&& Objects.equals(headersInDeliveryNotificationsEnabled(), other.headersInDeliveryNotificationsEnabled());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("IdentityNotificationAttributes").add("BounceTopic", bounceTopic())
.add("ComplaintTopic", complaintTopic()).add("DeliveryTopic", deliveryTopic())
.add("ForwardingEnabled", forwardingEnabled())
.add("HeadersInBounceNotificationsEnabled", headersInBounceNotificationsEnabled())
.add("HeadersInComplaintNotificationsEnabled", headersInComplaintNotificationsEnabled())
.add("HeadersInDeliveryNotificationsEnabled", headersInDeliveryNotificationsEnabled()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "BounceTopic":
return Optional.ofNullable(clazz.cast(bounceTopic()));
case "ComplaintTopic":
return Optional.ofNullable(clazz.cast(complaintTopic()));
case "DeliveryTopic":
return Optional.ofNullable(clazz.cast(deliveryTopic()));
case "ForwardingEnabled":
return Optional.ofNullable(clazz.cast(forwardingEnabled()));
case "HeadersInBounceNotificationsEnabled":
return Optional.ofNullable(clazz.cast(headersInBounceNotificationsEnabled()));
case "HeadersInComplaintNotificationsEnabled":
return Optional.ofNullable(clazz.cast(headersInComplaintNotificationsEnabled()));
case "HeadersInDeliveryNotificationsEnabled":
return Optional.ofNullable(clazz.cast(headersInDeliveryNotificationsEnabled()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function