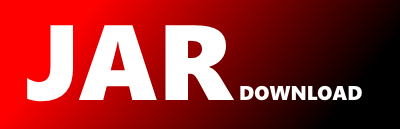
software.amazon.awssdk.services.shield.ShieldAsyncClient Maven / Gradle / Ivy
Show all versions of shield Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.shield;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.shield.model.AssociateDrtLogBucketRequest;
import software.amazon.awssdk.services.shield.model.AssociateDrtLogBucketResponse;
import software.amazon.awssdk.services.shield.model.AssociateDrtRoleRequest;
import software.amazon.awssdk.services.shield.model.AssociateDrtRoleResponse;
import software.amazon.awssdk.services.shield.model.AssociateHealthCheckRequest;
import software.amazon.awssdk.services.shield.model.AssociateHealthCheckResponse;
import software.amazon.awssdk.services.shield.model.AssociateProactiveEngagementDetailsRequest;
import software.amazon.awssdk.services.shield.model.AssociateProactiveEngagementDetailsResponse;
import software.amazon.awssdk.services.shield.model.CreateProtectionGroupRequest;
import software.amazon.awssdk.services.shield.model.CreateProtectionGroupResponse;
import software.amazon.awssdk.services.shield.model.CreateProtectionRequest;
import software.amazon.awssdk.services.shield.model.CreateProtectionResponse;
import software.amazon.awssdk.services.shield.model.CreateSubscriptionRequest;
import software.amazon.awssdk.services.shield.model.CreateSubscriptionResponse;
import software.amazon.awssdk.services.shield.model.DeleteProtectionGroupRequest;
import software.amazon.awssdk.services.shield.model.DeleteProtectionGroupResponse;
import software.amazon.awssdk.services.shield.model.DeleteProtectionRequest;
import software.amazon.awssdk.services.shield.model.DeleteProtectionResponse;
import software.amazon.awssdk.services.shield.model.DescribeAttackRequest;
import software.amazon.awssdk.services.shield.model.DescribeAttackResponse;
import software.amazon.awssdk.services.shield.model.DescribeAttackStatisticsRequest;
import software.amazon.awssdk.services.shield.model.DescribeAttackStatisticsResponse;
import software.amazon.awssdk.services.shield.model.DescribeDrtAccessRequest;
import software.amazon.awssdk.services.shield.model.DescribeDrtAccessResponse;
import software.amazon.awssdk.services.shield.model.DescribeEmergencyContactSettingsRequest;
import software.amazon.awssdk.services.shield.model.DescribeEmergencyContactSettingsResponse;
import software.amazon.awssdk.services.shield.model.DescribeProtectionGroupRequest;
import software.amazon.awssdk.services.shield.model.DescribeProtectionGroupResponse;
import software.amazon.awssdk.services.shield.model.DescribeProtectionRequest;
import software.amazon.awssdk.services.shield.model.DescribeProtectionResponse;
import software.amazon.awssdk.services.shield.model.DescribeSubscriptionRequest;
import software.amazon.awssdk.services.shield.model.DescribeSubscriptionResponse;
import software.amazon.awssdk.services.shield.model.DisableApplicationLayerAutomaticResponseRequest;
import software.amazon.awssdk.services.shield.model.DisableApplicationLayerAutomaticResponseResponse;
import software.amazon.awssdk.services.shield.model.DisableProactiveEngagementRequest;
import software.amazon.awssdk.services.shield.model.DisableProactiveEngagementResponse;
import software.amazon.awssdk.services.shield.model.DisassociateDrtLogBucketRequest;
import software.amazon.awssdk.services.shield.model.DisassociateDrtLogBucketResponse;
import software.amazon.awssdk.services.shield.model.DisassociateDrtRoleRequest;
import software.amazon.awssdk.services.shield.model.DisassociateDrtRoleResponse;
import software.amazon.awssdk.services.shield.model.DisassociateHealthCheckRequest;
import software.amazon.awssdk.services.shield.model.DisassociateHealthCheckResponse;
import software.amazon.awssdk.services.shield.model.EnableApplicationLayerAutomaticResponseRequest;
import software.amazon.awssdk.services.shield.model.EnableApplicationLayerAutomaticResponseResponse;
import software.amazon.awssdk.services.shield.model.EnableProactiveEngagementRequest;
import software.amazon.awssdk.services.shield.model.EnableProactiveEngagementResponse;
import software.amazon.awssdk.services.shield.model.GetSubscriptionStateRequest;
import software.amazon.awssdk.services.shield.model.GetSubscriptionStateResponse;
import software.amazon.awssdk.services.shield.model.ListAttacksRequest;
import software.amazon.awssdk.services.shield.model.ListAttacksResponse;
import software.amazon.awssdk.services.shield.model.ListProtectionGroupsRequest;
import software.amazon.awssdk.services.shield.model.ListProtectionGroupsResponse;
import software.amazon.awssdk.services.shield.model.ListProtectionsRequest;
import software.amazon.awssdk.services.shield.model.ListProtectionsResponse;
import software.amazon.awssdk.services.shield.model.ListResourcesInProtectionGroupRequest;
import software.amazon.awssdk.services.shield.model.ListResourcesInProtectionGroupResponse;
import software.amazon.awssdk.services.shield.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.shield.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.shield.model.TagResourceRequest;
import software.amazon.awssdk.services.shield.model.TagResourceResponse;
import software.amazon.awssdk.services.shield.model.UntagResourceRequest;
import software.amazon.awssdk.services.shield.model.UntagResourceResponse;
import software.amazon.awssdk.services.shield.model.UpdateApplicationLayerAutomaticResponseRequest;
import software.amazon.awssdk.services.shield.model.UpdateApplicationLayerAutomaticResponseResponse;
import software.amazon.awssdk.services.shield.model.UpdateEmergencyContactSettingsRequest;
import software.amazon.awssdk.services.shield.model.UpdateEmergencyContactSettingsResponse;
import software.amazon.awssdk.services.shield.model.UpdateProtectionGroupRequest;
import software.amazon.awssdk.services.shield.model.UpdateProtectionGroupResponse;
import software.amazon.awssdk.services.shield.model.UpdateSubscriptionRequest;
import software.amazon.awssdk.services.shield.model.UpdateSubscriptionResponse;
import software.amazon.awssdk.services.shield.paginators.ListAttacksPublisher;
import software.amazon.awssdk.services.shield.paginators.ListProtectionGroupsPublisher;
import software.amazon.awssdk.services.shield.paginators.ListProtectionsPublisher;
import software.amazon.awssdk.services.shield.paginators.ListResourcesInProtectionGroupPublisher;
/**
* Service client for accessing AWS Shield asynchronously. This can be created using the static {@link #builder()}
* method.
*
* Shield Advanced
*
* This is the Shield Advanced API Reference. This guide is for developers who need detailed information about
* the Shield Advanced API actions, data types, and errors. For detailed information about WAF and Shield Advanced
* features and an overview of how to use the WAF and Shield Advanced APIs, see the WAF and Shield Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface ShieldAsyncClient extends SdkClient {
String SERVICE_NAME = "shield";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "shield";
/**
* Create a {@link ShieldAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ShieldAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ShieldAsyncClient}.
*/
static ShieldAsyncClientBuilder builder() {
return new DefaultShieldAsyncClientBuilder();
}
/**
*
* Authorizes the Shield Response Team (SRT) to access the specified Amazon S3 bucket containing log data such as
* Application Load Balancer access logs, CloudFront logs, or logs from third party sources. You can associate up to
* 10 Amazon S3 buckets with your subscription.
*
*
* To use the services of the SRT and make an AssociateDRTLogBucket
request, you must be subscribed to
* the Business Support plan or the Enterprise Support plan.
*
*
* @param associateDrtLogBucketRequest
* @return A Java Future containing the result of the AssociateDRTLogBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - NoAssociatedRoleException The ARN of the role that you specified does not exist.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - AccessDeniedForDependencyException In order to grant the necessary access to the Shield Response Team
* (SRT) the user submitting the request must have the
iam:PassRole
permission. This error
* indicates the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an Amazon Web Services Service.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateDRTLogBucket
* @see AWS
* API Documentation
*/
default CompletableFuture associateDRTLogBucket(
AssociateDrtLogBucketRequest associateDrtLogBucketRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Authorizes the Shield Response Team (SRT) to access the specified Amazon S3 bucket containing log data such as
* Application Load Balancer access logs, CloudFront logs, or logs from third party sources. You can associate up to
* 10 Amazon S3 buckets with your subscription.
*
*
* To use the services of the SRT and make an AssociateDRTLogBucket
request, you must be subscribed to
* the Business Support plan or the Enterprise Support plan.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateDrtLogBucketRequest.Builder} avoiding the
* need to create one manually via {@link AssociateDrtLogBucketRequest#builder()}
*
*
* @param associateDrtLogBucketRequest
* A {@link Consumer} that will call methods on {@link AssociateDRTLogBucketRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateDRTLogBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - NoAssociatedRoleException The ARN of the role that you specified does not exist.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - AccessDeniedForDependencyException In order to grant the necessary access to the Shield Response Team
* (SRT) the user submitting the request must have the
iam:PassRole
permission. This error
* indicates the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an Amazon Web Services Service.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateDRTLogBucket
* @see AWS
* API Documentation
*/
default CompletableFuture associateDRTLogBucket(
Consumer associateDrtLogBucketRequest) {
return associateDRTLogBucket(AssociateDrtLogBucketRequest.builder().applyMutation(associateDrtLogBucketRequest).build());
}
/**
*
* Authorizes the Shield Response Team (SRT) using the specified role, to access your Amazon Web Services account to
* assist with DDoS attack mitigation during potential attacks. This enables the SRT to inspect your WAF
* configuration and create or update WAF rules and web ACLs.
*
*
* You can associate only one RoleArn
with your subscription. If you submit an
* AssociateDRTRole
request for an account that already has an associated role, the new
* RoleArn
will replace the existing RoleArn
.
*
*
* Prior to making the AssociateDRTRole
request, you must attach the
* AWSShieldDRTAccessPolicy
managed policy to the role that you'll specify in the request. You can
* access this policy in the IAM console at AWSShieldDRTAccessPolicy. For more information see Adding and
* removing IAM identity permissions. The role must also trust the service principal
* drt.shield.amazonaws.com
. For more information, see IAM JSON
* policy elements: Principal.
*
*
* The SRT will have access only to your WAF and Shield resources. By submitting this request, you authorize the SRT
* to inspect your WAF and Shield configuration and create and update WAF rules and web ACLs on your behalf. The SRT
* takes these actions only if explicitly authorized by you.
*
*
* You must have the iam:PassRole
permission to make an AssociateDRTRole
request. For more
* information, see Granting a
* user permissions to pass a role to an Amazon Web Services service.
*
*
* To use the services of the SRT and make an AssociateDRTRole
request, you must be subscribed to the
* Business Support plan or the Enterprise Support plan.
*
*
* @param associateDrtRoleRequest
* @return A Java Future containing the result of the AssociateDRTRole operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - AccessDeniedForDependencyException In order to grant the necessary access to the Shield Response Team
* (SRT) the user submitting the request must have the
iam:PassRole
permission. This error
* indicates the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an Amazon Web Services Service.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateDRTRole
* @see AWS API
* Documentation
*/
default CompletableFuture associateDRTRole(AssociateDrtRoleRequest associateDrtRoleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Authorizes the Shield Response Team (SRT) using the specified role, to access your Amazon Web Services account to
* assist with DDoS attack mitigation during potential attacks. This enables the SRT to inspect your WAF
* configuration and create or update WAF rules and web ACLs.
*
*
* You can associate only one RoleArn
with your subscription. If you submit an
* AssociateDRTRole
request for an account that already has an associated role, the new
* RoleArn
will replace the existing RoleArn
.
*
*
* Prior to making the AssociateDRTRole
request, you must attach the
* AWSShieldDRTAccessPolicy
managed policy to the role that you'll specify in the request. You can
* access this policy in the IAM console at AWSShieldDRTAccessPolicy. For more information see Adding and
* removing IAM identity permissions. The role must also trust the service principal
* drt.shield.amazonaws.com
. For more information, see IAM JSON
* policy elements: Principal.
*
*
* The SRT will have access only to your WAF and Shield resources. By submitting this request, you authorize the SRT
* to inspect your WAF and Shield configuration and create and update WAF rules and web ACLs on your behalf. The SRT
* takes these actions only if explicitly authorized by you.
*
*
* You must have the iam:PassRole
permission to make an AssociateDRTRole
request. For more
* information, see Granting a
* user permissions to pass a role to an Amazon Web Services service.
*
*
* To use the services of the SRT and make an AssociateDRTRole
request, you must be subscribed to the
* Business Support plan or the Enterprise Support plan.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateDrtRoleRequest.Builder} avoiding the need
* to create one manually via {@link AssociateDrtRoleRequest#builder()}
*
*
* @param associateDrtRoleRequest
* A {@link Consumer} that will call methods on {@link AssociateDRTRoleRequest.Builder} to create a request.
* @return A Java Future containing the result of the AssociateDRTRole operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - AccessDeniedForDependencyException In order to grant the necessary access to the Shield Response Team
* (SRT) the user submitting the request must have the
iam:PassRole
permission. This error
* indicates the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an Amazon Web Services Service.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateDRTRole
* @see AWS API
* Documentation
*/
default CompletableFuture associateDRTRole(
Consumer associateDrtRoleRequest) {
return associateDRTRole(AssociateDrtRoleRequest.builder().applyMutation(associateDrtRoleRequest).build());
}
/**
*
* Adds health-based detection to the Shield Advanced protection for a resource. Shield Advanced health-based
* detection uses the health of your Amazon Web Services resource to improve responsiveness and accuracy in attack
* detection and response.
*
*
* You define the health check in Route 53 and then associate it with your Shield Advanced protection. For more
* information, see Shield Advanced Health-Based Detection in the WAF Developer Guide.
*
*
* @param associateHealthCheckRequest
* @return A Java Future containing the result of the AssociateHealthCheck operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateHealthCheck
* @see AWS
* API Documentation
*/
default CompletableFuture associateHealthCheck(
AssociateHealthCheckRequest associateHealthCheckRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds health-based detection to the Shield Advanced protection for a resource. Shield Advanced health-based
* detection uses the health of your Amazon Web Services resource to improve responsiveness and accuracy in attack
* detection and response.
*
*
* You define the health check in Route 53 and then associate it with your Shield Advanced protection. For more
* information, see Shield Advanced Health-Based Detection in the WAF Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateHealthCheckRequest.Builder} avoiding the
* need to create one manually via {@link AssociateHealthCheckRequest#builder()}
*
*
* @param associateHealthCheckRequest
* A {@link Consumer} that will call methods on {@link AssociateHealthCheckRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateHealthCheck operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateHealthCheck
* @see AWS
* API Documentation
*/
default CompletableFuture associateHealthCheck(
Consumer associateHealthCheckRequest) {
return associateHealthCheck(AssociateHealthCheckRequest.builder().applyMutation(associateHealthCheckRequest).build());
}
/**
*
* Initializes proactive engagement and sets the list of contacts for the Shield Response Team (SRT) to use. You
* must provide at least one phone number in the emergency contact list.
*
*
* After you have initialized proactive engagement using this call, to disable or enable proactive engagement, use
* the calls DisableProactiveEngagement
and EnableProactiveEngagement
.
*
*
*
* This call defines the list of email addresses and phone numbers that the SRT can use to contact you for
* escalations to the SRT and to initiate proactive customer support.
*
*
* The contacts that you provide in the request replace any contacts that were already defined. If you already have
* contacts defined and want to use them, retrieve the list using DescribeEmergencyContactSettings
and
* then provide it to this call.
*
*
*
* @param associateProactiveEngagementDetailsRequest
* @return A Java Future containing the result of the AssociateProactiveEngagementDetails operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateProactiveEngagementDetails
* @see AWS API Documentation
*/
default CompletableFuture associateProactiveEngagementDetails(
AssociateProactiveEngagementDetailsRequest associateProactiveEngagementDetailsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Initializes proactive engagement and sets the list of contacts for the Shield Response Team (SRT) to use. You
* must provide at least one phone number in the emergency contact list.
*
*
* After you have initialized proactive engagement using this call, to disable or enable proactive engagement, use
* the calls DisableProactiveEngagement
and EnableProactiveEngagement
.
*
*
*
* This call defines the list of email addresses and phone numbers that the SRT can use to contact you for
* escalations to the SRT and to initiate proactive customer support.
*
*
* The contacts that you provide in the request replace any contacts that were already defined. If you already have
* contacts defined and want to use them, retrieve the list using DescribeEmergencyContactSettings
and
* then provide it to this call.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateProactiveEngagementDetailsRequest.Builder}
* avoiding the need to create one manually via {@link AssociateProactiveEngagementDetailsRequest#builder()}
*
*
* @param associateProactiveEngagementDetailsRequest
* A {@link Consumer} that will call methods on {@link AssociateProactiveEngagementDetailsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateProactiveEngagementDetails operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateProactiveEngagementDetails
* @see AWS API Documentation
*/
default CompletableFuture associateProactiveEngagementDetails(
Consumer associateProactiveEngagementDetailsRequest) {
return associateProactiveEngagementDetails(AssociateProactiveEngagementDetailsRequest.builder()
.applyMutation(associateProactiveEngagementDetailsRequest).build());
}
/**
*
* Enables Shield Advanced for a specific Amazon Web Services resource. The resource can be an Amazon CloudFront
* distribution, Amazon Route 53 hosted zone, Global Accelerator standard accelerator, Elastic IP Address,
* Application Load Balancer, or a Classic Load Balancer. You can protect Amazon EC2 instances and Network Load
* Balancers by association with protected Amazon EC2 Elastic IP addresses.
*
*
* You can add protection to only a single resource with each CreateProtection
request. You can add
* protection to multiple resources at once through the Shield Advanced console at https://console.aws.amazon.com/wafv2/shieldv2#/. For
* more information see Getting Started with
* Shield Advanced and Adding Shield Advanced
* protection to Amazon Web Services resources.
*
*
* @param createProtectionRequest
* @return A Java Future containing the result of the CreateProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - ResourceAlreadyExistsException Exception indicating the specified resource already exists. If
* available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.CreateProtection
* @see AWS API
* Documentation
*/
default CompletableFuture createProtection(CreateProtectionRequest createProtectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Enables Shield Advanced for a specific Amazon Web Services resource. The resource can be an Amazon CloudFront
* distribution, Amazon Route 53 hosted zone, Global Accelerator standard accelerator, Elastic IP Address,
* Application Load Balancer, or a Classic Load Balancer. You can protect Amazon EC2 instances and Network Load
* Balancers by association with protected Amazon EC2 Elastic IP addresses.
*
*
* You can add protection to only a single resource with each CreateProtection
request. You can add
* protection to multiple resources at once through the Shield Advanced console at https://console.aws.amazon.com/wafv2/shieldv2#/. For
* more information see Getting Started with
* Shield Advanced and Adding Shield Advanced
* protection to Amazon Web Services resources.
*
*
*
* This is a convenience which creates an instance of the {@link CreateProtectionRequest.Builder} avoiding the need
* to create one manually via {@link CreateProtectionRequest#builder()}
*
*
* @param createProtectionRequest
* A {@link Consumer} that will call methods on {@link CreateProtectionRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - ResourceAlreadyExistsException Exception indicating the specified resource already exists. If
* available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.CreateProtection
* @see AWS API
* Documentation
*/
default CompletableFuture createProtection(
Consumer createProtectionRequest) {
return createProtection(CreateProtectionRequest.builder().applyMutation(createProtectionRequest).build());
}
/**
*
* Creates a grouping of protected resources so they can be handled as a collective. This resource grouping improves
* the accuracy of detection and reduces false positives.
*
*
* @param createProtectionGroupRequest
* @return A Java Future containing the result of the CreateProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceAlreadyExistsException Exception indicating the specified resource already exists. If
* available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.CreateProtectionGroup
* @see AWS
* API Documentation
*/
default CompletableFuture createProtectionGroup(
CreateProtectionGroupRequest createProtectionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a grouping of protected resources so they can be handled as a collective. This resource grouping improves
* the accuracy of detection and reduces false positives.
*
*
*
* This is a convenience which creates an instance of the {@link CreateProtectionGroupRequest.Builder} avoiding the
* need to create one manually via {@link CreateProtectionGroupRequest#builder()}
*
*
* @param createProtectionGroupRequest
* A {@link Consumer} that will call methods on {@link CreateProtectionGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceAlreadyExistsException Exception indicating the specified resource already exists. If
* available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.CreateProtectionGroup
* @see AWS
* API Documentation
*/
default CompletableFuture createProtectionGroup(
Consumer createProtectionGroupRequest) {
return createProtectionGroup(CreateProtectionGroupRequest.builder().applyMutation(createProtectionGroupRequest).build());
}
/**
*
* Activates Shield Advanced for an account.
*
*
*
* For accounts that are members of an Organizations organization, Shield Advanced subscriptions are billed against
* the organization's payer account, regardless of whether the payer account itself is subscribed.
*
*
*
* When you initially create a subscription, your subscription is set to be automatically renewed at the end of the
* existing subscription period. You can change this by submitting an UpdateSubscription
request.
*
*
* @param createSubscriptionRequest
* @return A Java Future containing the result of the CreateSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceAlreadyExistsException Exception indicating the specified resource already exists. If
* available, this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.CreateSubscription
* @see AWS API
* Documentation
*/
default CompletableFuture createSubscription(CreateSubscriptionRequest createSubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Activates Shield Advanced for an account.
*
*
*
* For accounts that are members of an Organizations organization, Shield Advanced subscriptions are billed against
* the organization's payer account, regardless of whether the payer account itself is subscribed.
*
*
*
* When you initially create a subscription, your subscription is set to be automatically renewed at the end of the
* existing subscription period. You can change this by submitting an UpdateSubscription
request.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSubscriptionRequest.Builder} avoiding the
* need to create one manually via {@link CreateSubscriptionRequest#builder()}
*
*
* @param createSubscriptionRequest
* A {@link Consumer} that will call methods on {@link CreateSubscriptionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceAlreadyExistsException Exception indicating the specified resource already exists. If
* available, this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.CreateSubscription
* @see AWS API
* Documentation
*/
default CompletableFuture createSubscription(
Consumer createSubscriptionRequest) {
return createSubscription(CreateSubscriptionRequest.builder().applyMutation(createSubscriptionRequest).build());
}
/**
*
* Activates Shield Advanced for an account.
*
*
*
* For accounts that are members of an Organizations organization, Shield Advanced subscriptions are billed against
* the organization's payer account, regardless of whether the payer account itself is subscribed.
*
*
*
* When you initially create a subscription, your subscription is set to be automatically renewed at the end of the
* existing subscription period. You can change this by submitting an UpdateSubscription
request.
*
*
* @return A Java Future containing the result of the CreateSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceAlreadyExistsException Exception indicating the specified resource already exists. If
* available, this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.CreateSubscription
* @see AWS API
* Documentation
*/
default CompletableFuture createSubscription() {
return createSubscription(CreateSubscriptionRequest.builder().build());
}
/**
*
* Deletes an Shield Advanced Protection.
*
*
* @param deleteProtectionRequest
* @return A Java Future containing the result of the DeleteProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DeleteProtection
* @see AWS API
* Documentation
*/
default CompletableFuture deleteProtection(DeleteProtectionRequest deleteProtectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Shield Advanced Protection.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProtectionRequest.Builder} avoiding the need
* to create one manually via {@link DeleteProtectionRequest#builder()}
*
*
* @param deleteProtectionRequest
* A {@link Consumer} that will call methods on {@link DeleteProtectionRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DeleteProtection
* @see AWS API
* Documentation
*/
default CompletableFuture deleteProtection(
Consumer deleteProtectionRequest) {
return deleteProtection(DeleteProtectionRequest.builder().applyMutation(deleteProtectionRequest).build());
}
/**
*
* Removes the specified protection group.
*
*
* @param deleteProtectionGroupRequest
* @return A Java Future containing the result of the DeleteProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DeleteProtectionGroup
* @see AWS
* API Documentation
*/
default CompletableFuture deleteProtectionGroup(
DeleteProtectionGroupRequest deleteProtectionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified protection group.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProtectionGroupRequest.Builder} avoiding the
* need to create one manually via {@link DeleteProtectionGroupRequest#builder()}
*
*
* @param deleteProtectionGroupRequest
* A {@link Consumer} that will call methods on {@link DeleteProtectionGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DeleteProtectionGroup
* @see AWS
* API Documentation
*/
default CompletableFuture deleteProtectionGroup(
Consumer deleteProtectionGroupRequest) {
return deleteProtectionGroup(DeleteProtectionGroupRequest.builder().applyMutation(deleteProtectionGroupRequest).build());
}
/**
*
* Describes the details of a DDoS attack.
*
*
* @param describeAttackRequest
* @return A Java Future containing the result of the DescribeAttack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - AccessDeniedException Exception that indicates the specified
AttackId
does not exist, or
* the requester does not have the appropriate permissions to access the AttackId
.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeAttack
* @see AWS API
* Documentation
*/
default CompletableFuture describeAttack(DescribeAttackRequest describeAttackRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the details of a DDoS attack.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAttackRequest.Builder} avoiding the need to
* create one manually via {@link DescribeAttackRequest#builder()}
*
*
* @param describeAttackRequest
* A {@link Consumer} that will call methods on {@link DescribeAttackRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeAttack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - AccessDeniedException Exception that indicates the specified
AttackId
does not exist, or
* the requester does not have the appropriate permissions to access the AttackId
.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeAttack
* @see AWS API
* Documentation
*/
default CompletableFuture describeAttack(Consumer describeAttackRequest) {
return describeAttack(DescribeAttackRequest.builder().applyMutation(describeAttackRequest).build());
}
/**
*
* Provides information about the number and type of attacks Shield has detected in the last year for all resources
* that belong to your account, regardless of whether you've defined Shield protections for them. This operation is
* available to Shield customers as well as to Shield Advanced customers.
*
*
* The operation returns data for the time range of midnight UTC, one year ago, to midnight UTC, today. For example,
* if the current time is 2020-10-26 15:39:32 PDT
, equal to 2020-10-26 22:39:32 UTC
, then
* the time range for the attack data returned is from 2019-10-26 00:00:00 UTC
to
* 2020-10-26 00:00:00 UTC
.
*
*
* The time range indicates the period covered by the attack statistics data items.
*
*
* @param describeAttackStatisticsRequest
* @return A Java Future containing the result of the DescribeAttackStatistics operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeAttackStatistics
* @see AWS API Documentation
*/
default CompletableFuture describeAttackStatistics(
DescribeAttackStatisticsRequest describeAttackStatisticsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides information about the number and type of attacks Shield has detected in the last year for all resources
* that belong to your account, regardless of whether you've defined Shield protections for them. This operation is
* available to Shield customers as well as to Shield Advanced customers.
*
*
* The operation returns data for the time range of midnight UTC, one year ago, to midnight UTC, today. For example,
* if the current time is 2020-10-26 15:39:32 PDT
, equal to 2020-10-26 22:39:32 UTC
, then
* the time range for the attack data returned is from 2019-10-26 00:00:00 UTC
to
* 2020-10-26 00:00:00 UTC
.
*
*
* The time range indicates the period covered by the attack statistics data items.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAttackStatisticsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeAttackStatisticsRequest#builder()}
*
*
* @param describeAttackStatisticsRequest
* A {@link Consumer} that will call methods on {@link DescribeAttackStatisticsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeAttackStatistics operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeAttackStatistics
* @see AWS API Documentation
*/
default CompletableFuture describeAttackStatistics(
Consumer describeAttackStatisticsRequest) {
return describeAttackStatistics(DescribeAttackStatisticsRequest.builder().applyMutation(describeAttackStatisticsRequest)
.build());
}
/**
*
* Returns the current role and list of Amazon S3 log buckets used by the Shield Response Team (SRT) to access your
* Amazon Web Services account while assisting with attack mitigation.
*
*
* @param describeDrtAccessRequest
* @return A Java Future containing the result of the DescribeDRTAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeDRTAccess
* @see AWS API
* Documentation
*/
default CompletableFuture describeDRTAccess(DescribeDrtAccessRequest describeDrtAccessRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the current role and list of Amazon S3 log buckets used by the Shield Response Team (SRT) to access your
* Amazon Web Services account while assisting with attack mitigation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDrtAccessRequest.Builder} avoiding the need
* to create one manually via {@link DescribeDrtAccessRequest#builder()}
*
*
* @param describeDrtAccessRequest
* A {@link Consumer} that will call methods on {@link DescribeDRTAccessRequest.Builder} to create a request.
* @return A Java Future containing the result of the DescribeDRTAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeDRTAccess
* @see AWS API
* Documentation
*/
default CompletableFuture describeDRTAccess(
Consumer describeDrtAccessRequest) {
return describeDRTAccess(DescribeDrtAccessRequest.builder().applyMutation(describeDrtAccessRequest).build());
}
/**
*
* A list of email addresses and phone numbers that the Shield Response Team (SRT) can use to contact you if you
* have proactive engagement enabled, for escalations to the SRT and to initiate proactive customer support.
*
*
* @param describeEmergencyContactSettingsRequest
* @return A Java Future containing the result of the DescribeEmergencyContactSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeEmergencyContactSettings
* @see AWS API Documentation
*/
default CompletableFuture describeEmergencyContactSettings(
DescribeEmergencyContactSettingsRequest describeEmergencyContactSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* A list of email addresses and phone numbers that the Shield Response Team (SRT) can use to contact you if you
* have proactive engagement enabled, for escalations to the SRT and to initiate proactive customer support.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEmergencyContactSettingsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeEmergencyContactSettingsRequest#builder()}
*
*
* @param describeEmergencyContactSettingsRequest
* A {@link Consumer} that will call methods on {@link DescribeEmergencyContactSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DescribeEmergencyContactSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeEmergencyContactSettings
* @see AWS API Documentation
*/
default CompletableFuture describeEmergencyContactSettings(
Consumer describeEmergencyContactSettingsRequest) {
return describeEmergencyContactSettings(DescribeEmergencyContactSettingsRequest.builder()
.applyMutation(describeEmergencyContactSettingsRequest).build());
}
/**
*
* Lists the details of a Protection object.
*
*
* @param describeProtectionRequest
* @return A Java Future containing the result of the DescribeProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeProtection
* @see AWS API
* Documentation
*/
default CompletableFuture describeProtection(DescribeProtectionRequest describeProtectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the details of a Protection object.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeProtectionRequest.Builder} avoiding the
* need to create one manually via {@link DescribeProtectionRequest#builder()}
*
*
* @param describeProtectionRequest
* A {@link Consumer} that will call methods on {@link DescribeProtectionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeProtection
* @see AWS API
* Documentation
*/
default CompletableFuture describeProtection(
Consumer describeProtectionRequest) {
return describeProtection(DescribeProtectionRequest.builder().applyMutation(describeProtectionRequest).build());
}
/**
*
* Returns the specification for the specified protection group.
*
*
* @param describeProtectionGroupRequest
* @return A Java Future containing the result of the DescribeProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeProtectionGroup
* @see AWS API Documentation
*/
default CompletableFuture describeProtectionGroup(
DescribeProtectionGroupRequest describeProtectionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the specification for the specified protection group.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeProtectionGroupRequest.Builder} avoiding
* the need to create one manually via {@link DescribeProtectionGroupRequest#builder()}
*
*
* @param describeProtectionGroupRequest
* A {@link Consumer} that will call methods on {@link DescribeProtectionGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeProtectionGroup
* @see AWS API Documentation
*/
default CompletableFuture describeProtectionGroup(
Consumer describeProtectionGroupRequest) {
return describeProtectionGroup(DescribeProtectionGroupRequest.builder().applyMutation(describeProtectionGroupRequest)
.build());
}
/**
*
* Provides details about the Shield Advanced subscription for an account.
*
*
* @param describeSubscriptionRequest
* @return A Java Future containing the result of the DescribeSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeSubscription
* @see AWS
* API Documentation
*/
default CompletableFuture describeSubscription(
DescribeSubscriptionRequest describeSubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Provides details about the Shield Advanced subscription for an account.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeSubscriptionRequest.Builder} avoiding the
* need to create one manually via {@link DescribeSubscriptionRequest#builder()}
*
*
* @param describeSubscriptionRequest
* A {@link Consumer} that will call methods on {@link DescribeSubscriptionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeSubscription
* @see AWS
* API Documentation
*/
default CompletableFuture describeSubscription(
Consumer describeSubscriptionRequest) {
return describeSubscription(DescribeSubscriptionRequest.builder().applyMutation(describeSubscriptionRequest).build());
}
/**
*
* Provides details about the Shield Advanced subscription for an account.
*
*
* @return A Java Future containing the result of the DescribeSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeSubscription
* @see AWS
* API Documentation
*/
default CompletableFuture describeSubscription() {
return describeSubscription(DescribeSubscriptionRequest.builder().build());
}
/**
*
* Disable the Shield Advanced automatic application layer DDoS mitigation feature for the protected resource. This
* stops Shield Advanced from creating, verifying, and applying WAF rules for attacks that it detects for the
* resource.
*
*
* @param disableApplicationLayerAutomaticResponseRequest
* @return A Java Future containing the result of the DisableApplicationLayerAutomaticResponse operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisableApplicationLayerAutomaticResponse
* @see AWS API Documentation
*/
default CompletableFuture disableApplicationLayerAutomaticResponse(
DisableApplicationLayerAutomaticResponseRequest disableApplicationLayerAutomaticResponseRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disable the Shield Advanced automatic application layer DDoS mitigation feature for the protected resource. This
* stops Shield Advanced from creating, verifying, and applying WAF rules for attacks that it detects for the
* resource.
*
*
*
* This is a convenience which creates an instance of the
* {@link DisableApplicationLayerAutomaticResponseRequest.Builder} avoiding the need to create one manually via
* {@link DisableApplicationLayerAutomaticResponseRequest#builder()}
*
*
* @param disableApplicationLayerAutomaticResponseRequest
* A {@link Consumer} that will call methods on
* {@link DisableApplicationLayerAutomaticResponseRequest.Builder} to create a request.
* @return A Java Future containing the result of the DisableApplicationLayerAutomaticResponse operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisableApplicationLayerAutomaticResponse
* @see AWS API Documentation
*/
default CompletableFuture disableApplicationLayerAutomaticResponse(
Consumer disableApplicationLayerAutomaticResponseRequest) {
return disableApplicationLayerAutomaticResponse(DisableApplicationLayerAutomaticResponseRequest.builder()
.applyMutation(disableApplicationLayerAutomaticResponseRequest).build());
}
/**
*
* Removes authorization from the Shield Response Team (SRT) to notify contacts about escalations to the SRT and to
* initiate proactive customer support.
*
*
* @param disableProactiveEngagementRequest
* @return A Java Future containing the result of the DisableProactiveEngagement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisableProactiveEngagement
* @see AWS API Documentation
*/
default CompletableFuture disableProactiveEngagement(
DisableProactiveEngagementRequest disableProactiveEngagementRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes authorization from the Shield Response Team (SRT) to notify contacts about escalations to the SRT and to
* initiate proactive customer support.
*
*
*
* This is a convenience which creates an instance of the {@link DisableProactiveEngagementRequest.Builder} avoiding
* the need to create one manually via {@link DisableProactiveEngagementRequest#builder()}
*
*
* @param disableProactiveEngagementRequest
* A {@link Consumer} that will call methods on {@link DisableProactiveEngagementRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisableProactiveEngagement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisableProactiveEngagement
* @see AWS API Documentation
*/
default CompletableFuture disableProactiveEngagement(
Consumer disableProactiveEngagementRequest) {
return disableProactiveEngagement(DisableProactiveEngagementRequest.builder()
.applyMutation(disableProactiveEngagementRequest).build());
}
/**
*
* Removes the Shield Response Team's (SRT) access to the specified Amazon S3 bucket containing the logs that you
* shared previously.
*
*
* @param disassociateDrtLogBucketRequest
* @return A Java Future containing the result of the DisassociateDRTLogBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - NoAssociatedRoleException The ARN of the role that you specified does not exist.
* - AccessDeniedForDependencyException In order to grant the necessary access to the Shield Response Team
* (SRT) the user submitting the request must have the
iam:PassRole
permission. This error
* indicates the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an Amazon Web Services Service.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisassociateDRTLogBucket
* @see AWS API Documentation
*/
default CompletableFuture disassociateDRTLogBucket(
DisassociateDrtLogBucketRequest disassociateDrtLogBucketRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the Shield Response Team's (SRT) access to the specified Amazon S3 bucket containing the logs that you
* shared previously.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateDrtLogBucketRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateDrtLogBucketRequest#builder()}
*
*
* @param disassociateDrtLogBucketRequest
* A {@link Consumer} that will call methods on {@link DisassociateDRTLogBucketRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateDRTLogBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - NoAssociatedRoleException The ARN of the role that you specified does not exist.
* - AccessDeniedForDependencyException In order to grant the necessary access to the Shield Response Team
* (SRT) the user submitting the request must have the
iam:PassRole
permission. This error
* indicates the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an Amazon Web Services Service.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisassociateDRTLogBucket
* @see AWS API Documentation
*/
default CompletableFuture disassociateDRTLogBucket(
Consumer disassociateDrtLogBucketRequest) {
return disassociateDRTLogBucket(DisassociateDrtLogBucketRequest.builder().applyMutation(disassociateDrtLogBucketRequest)
.build());
}
/**
*
* Removes the Shield Response Team's (SRT) access to your Amazon Web Services account.
*
*
* @param disassociateDrtRoleRequest
* @return A Java Future containing the result of the DisassociateDRTRole operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisassociateDRTRole
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateDRTRole(
DisassociateDrtRoleRequest disassociateDrtRoleRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the Shield Response Team's (SRT) access to your Amazon Web Services account.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateDrtRoleRequest.Builder} avoiding the
* need to create one manually via {@link DisassociateDrtRoleRequest#builder()}
*
*
* @param disassociateDrtRoleRequest
* A {@link Consumer} that will call methods on {@link DisassociateDRTRoleRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateDRTRole operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisassociateDRTRole
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateDRTRole(
Consumer disassociateDrtRoleRequest) {
return disassociateDRTRole(DisassociateDrtRoleRequest.builder().applyMutation(disassociateDrtRoleRequest).build());
}
/**
*
* Removes the Shield Response Team's (SRT) access to your Amazon Web Services account.
*
*
* @return A Java Future containing the result of the DisassociateDRTRole operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisassociateDRTRole
* @see AWS
* API Documentation
*/
default CompletableFuture disassociateDRTRole() {
return disassociateDRTRole(DisassociateDrtRoleRequest.builder().build());
}
/**
*
* Removes health-based detection from the Shield Advanced protection for a resource. Shield Advanced health-based
* detection uses the health of your Amazon Web Services resource to improve responsiveness and accuracy in attack
* detection and response.
*
*
* You define the health check in Route 53 and then associate or disassociate it with your Shield Advanced
* protection. For more information, see Shield Advanced Health-Based Detection in the WAF Developer Guide.
*
*
* @param disassociateHealthCheckRequest
* @return A Java Future containing the result of the DisassociateHealthCheck operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisassociateHealthCheck
* @see AWS API Documentation
*/
default CompletableFuture disassociateHealthCheck(
DisassociateHealthCheckRequest disassociateHealthCheckRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes health-based detection from the Shield Advanced protection for a resource. Shield Advanced health-based
* detection uses the health of your Amazon Web Services resource to improve responsiveness and accuracy in attack
* detection and response.
*
*
* You define the health check in Route 53 and then associate or disassociate it with your Shield Advanced
* protection. For more information, see Shield Advanced Health-Based Detection in the WAF Developer Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateHealthCheckRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateHealthCheckRequest#builder()}
*
*
* @param disassociateHealthCheckRequest
* A {@link Consumer} that will call methods on {@link DisassociateHealthCheckRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateHealthCheck operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisassociateHealthCheck
* @see AWS API Documentation
*/
default CompletableFuture disassociateHealthCheck(
Consumer disassociateHealthCheckRequest) {
return disassociateHealthCheck(DisassociateHealthCheckRequest.builder().applyMutation(disassociateHealthCheckRequest)
.build());
}
/**
*
* Enable the Shield Advanced automatic application layer DDoS mitigation for the protected resource.
*
*
*
* This feature is available for Amazon CloudFront distributions and Application Load Balancers only.
*
*
*
* This causes Shield Advanced to create, verify, and apply WAF rules for DDoS attacks that it detects for the
* resource. Shield Advanced applies the rules in a Shield rule group inside the web ACL that you've associated with
* the resource. For information about how automatic mitigation works and the requirements for using it, see Shield
* Advanced automatic application layer DDoS mitigation.
*
*
*
* Don't use this action to make changes to automatic mitigation settings when it's already enabled for a resource.
* Instead, use UpdateApplicationLayerAutomaticResponse.
*
*
*
* To use this feature, you must associate a web ACL with the protected resource. The web ACL must be created using
* the latest version of WAF (v2). You can associate the web ACL through the Shield Advanced console at https://console.aws.amazon.com/wafv2/shieldv2#/. For
* more information, see Getting Started with
* Shield Advanced. You can also associate the web ACL to the resource through the WAF console or the WAF API,
* but you must manage Shield Advanced automatic mitigation through Shield Advanced. For information about WAF, see
* WAF Developer Guide.
*
*
* @param enableApplicationLayerAutomaticResponseRequest
* @return A Java Future containing the result of the EnableApplicationLayerAutomaticResponse operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.EnableApplicationLayerAutomaticResponse
* @see AWS API Documentation
*/
default CompletableFuture enableApplicationLayerAutomaticResponse(
EnableApplicationLayerAutomaticResponseRequest enableApplicationLayerAutomaticResponseRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Enable the Shield Advanced automatic application layer DDoS mitigation for the protected resource.
*
*
*
* This feature is available for Amazon CloudFront distributions and Application Load Balancers only.
*
*
*
* This causes Shield Advanced to create, verify, and apply WAF rules for DDoS attacks that it detects for the
* resource. Shield Advanced applies the rules in a Shield rule group inside the web ACL that you've associated with
* the resource. For information about how automatic mitigation works and the requirements for using it, see Shield
* Advanced automatic application layer DDoS mitigation.
*
*
*
* Don't use this action to make changes to automatic mitigation settings when it's already enabled for a resource.
* Instead, use UpdateApplicationLayerAutomaticResponse.
*
*
*
* To use this feature, you must associate a web ACL with the protected resource. The web ACL must be created using
* the latest version of WAF (v2). You can associate the web ACL through the Shield Advanced console at https://console.aws.amazon.com/wafv2/shieldv2#/. For
* more information, see Getting Started with
* Shield Advanced. You can also associate the web ACL to the resource through the WAF console or the WAF API,
* but you must manage Shield Advanced automatic mitigation through Shield Advanced. For information about WAF, see
* WAF Developer Guide.
*
*
*
* This is a convenience which creates an instance of the
* {@link EnableApplicationLayerAutomaticResponseRequest.Builder} avoiding the need to create one manually via
* {@link EnableApplicationLayerAutomaticResponseRequest#builder()}
*
*
* @param enableApplicationLayerAutomaticResponseRequest
* A {@link Consumer} that will call methods on
* {@link EnableApplicationLayerAutomaticResponseRequest.Builder} to create a request.
* @return A Java Future containing the result of the EnableApplicationLayerAutomaticResponse operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.EnableApplicationLayerAutomaticResponse
* @see AWS API Documentation
*/
default CompletableFuture enableApplicationLayerAutomaticResponse(
Consumer enableApplicationLayerAutomaticResponseRequest) {
return enableApplicationLayerAutomaticResponse(EnableApplicationLayerAutomaticResponseRequest.builder()
.applyMutation(enableApplicationLayerAutomaticResponseRequest).build());
}
/**
*
* Authorizes the Shield Response Team (SRT) to use email and phone to notify contacts about escalations to the SRT
* and to initiate proactive customer support.
*
*
* @param enableProactiveEngagementRequest
* @return A Java Future containing the result of the EnableProactiveEngagement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.EnableProactiveEngagement
* @see AWS API Documentation
*/
default CompletableFuture enableProactiveEngagement(
EnableProactiveEngagementRequest enableProactiveEngagementRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Authorizes the Shield Response Team (SRT) to use email and phone to notify contacts about escalations to the SRT
* and to initiate proactive customer support.
*
*
*
* This is a convenience which creates an instance of the {@link EnableProactiveEngagementRequest.Builder} avoiding
* the need to create one manually via {@link EnableProactiveEngagementRequest#builder()}
*
*
* @param enableProactiveEngagementRequest
* A {@link Consumer} that will call methods on {@link EnableProactiveEngagementRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the EnableProactiveEngagement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.EnableProactiveEngagement
* @see AWS API Documentation
*/
default CompletableFuture enableProactiveEngagement(
Consumer enableProactiveEngagementRequest) {
return enableProactiveEngagement(EnableProactiveEngagementRequest.builder()
.applyMutation(enableProactiveEngagementRequest).build());
}
/**
*
* Returns the SubscriptionState
, either Active
or Inactive
.
*
*
* @param getSubscriptionStateRequest
* @return A Java Future containing the result of the GetSubscriptionState operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.GetSubscriptionState
* @see AWS
* API Documentation
*/
default CompletableFuture getSubscriptionState(
GetSubscriptionStateRequest getSubscriptionStateRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the SubscriptionState
, either Active
or Inactive
.
*
*
*
* This is a convenience which creates an instance of the {@link GetSubscriptionStateRequest.Builder} avoiding the
* need to create one manually via {@link GetSubscriptionStateRequest#builder()}
*
*
* @param getSubscriptionStateRequest
* A {@link Consumer} that will call methods on {@link GetSubscriptionStateRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetSubscriptionState operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.GetSubscriptionState
* @see AWS
* API Documentation
*/
default CompletableFuture getSubscriptionState(
Consumer getSubscriptionStateRequest) {
return getSubscriptionState(GetSubscriptionStateRequest.builder().applyMutation(getSubscriptionStateRequest).build());
}
/**
*
* Returns the SubscriptionState
, either Active
or Inactive
.
*
*
* @return A Java Future containing the result of the GetSubscriptionState operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.GetSubscriptionState
* @see AWS
* API Documentation
*/
default CompletableFuture getSubscriptionState() {
return getSubscriptionState(GetSubscriptionStateRequest.builder().build());
}
/**
*
* Returns all ongoing DDoS attacks or all DDoS attacks during a specified time period.
*
*
* @param listAttacksRequest
* @return A Java Future containing the result of the ListAttacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListAttacks
* @see AWS API
* Documentation
*/
default CompletableFuture listAttacks(ListAttacksRequest listAttacksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns all ongoing DDoS attacks or all DDoS attacks during a specified time period.
*
*
*
* This is a convenience which creates an instance of the {@link ListAttacksRequest.Builder} avoiding the need to
* create one manually via {@link ListAttacksRequest#builder()}
*
*
* @param listAttacksRequest
* A {@link Consumer} that will call methods on {@link ListAttacksRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListAttacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListAttacks
* @see AWS API
* Documentation
*/
default CompletableFuture listAttacks(Consumer listAttacksRequest) {
return listAttacks(ListAttacksRequest.builder().applyMutation(listAttacksRequest).build());
}
/**
*
* Returns all ongoing DDoS attacks or all DDoS attacks during a specified time period.
*
*
* @return A Java Future containing the result of the ListAttacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListAttacks
* @see AWS API
* Documentation
*/
default CompletableFuture listAttacks() {
return listAttacks(ListAttacksRequest.builder().build());
}
/**
*
* Returns all ongoing DDoS attacks or all DDoS attacks during a specified time period.
*
*
*
* This is a variant of {@link #listAttacks(software.amazon.awssdk.services.shield.model.ListAttacksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListAttacksPublisher publisher = client.listAttacksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListAttacksPublisher publisher = client.listAttacksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.shield.model.ListAttacksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttacks(software.amazon.awssdk.services.shield.model.ListAttacksRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListAttacks
* @see AWS API
* Documentation
*/
default ListAttacksPublisher listAttacksPaginator() {
return listAttacksPaginator(ListAttacksRequest.builder().build());
}
/**
*
* Returns all ongoing DDoS attacks or all DDoS attacks during a specified time period.
*
*
*
* This is a variant of {@link #listAttacks(software.amazon.awssdk.services.shield.model.ListAttacksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListAttacksPublisher publisher = client.listAttacksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListAttacksPublisher publisher = client.listAttacksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.shield.model.ListAttacksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttacks(software.amazon.awssdk.services.shield.model.ListAttacksRequest)} operation.
*
*
* @param listAttacksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListAttacks
* @see AWS API
* Documentation
*/
default ListAttacksPublisher listAttacksPaginator(ListAttacksRequest listAttacksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns all ongoing DDoS attacks or all DDoS attacks during a specified time period.
*
*
*
* This is a variant of {@link #listAttacks(software.amazon.awssdk.services.shield.model.ListAttacksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListAttacksPublisher publisher = client.listAttacksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListAttacksPublisher publisher = client.listAttacksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.shield.model.ListAttacksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttacks(software.amazon.awssdk.services.shield.model.ListAttacksRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListAttacksRequest.Builder} avoiding the need to
* create one manually via {@link ListAttacksRequest#builder()}
*
*
* @param listAttacksRequest
* A {@link Consumer} that will call methods on {@link ListAttacksRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListAttacks
* @see AWS API
* Documentation
*/
default ListAttacksPublisher listAttacksPaginator(Consumer listAttacksRequest) {
return listAttacksPaginator(ListAttacksRequest.builder().applyMutation(listAttacksRequest).build());
}
/**
*
* Retrieves ProtectionGroup objects for the account. You can retrieve all protection groups or you can
* provide filtering criteria and retrieve just the subset of protection groups that match the criteria.
*
*
* @param listProtectionGroupsRequest
* @return A Java Future containing the result of the ListProtectionGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtectionGroups
* @see AWS
* API Documentation
*/
default CompletableFuture listProtectionGroups(
ListProtectionGroupsRequest listProtectionGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves ProtectionGroup objects for the account. You can retrieve all protection groups or you can
* provide filtering criteria and retrieve just the subset of protection groups that match the criteria.
*
*
*
* This is a convenience which creates an instance of the {@link ListProtectionGroupsRequest.Builder} avoiding the
* need to create one manually via {@link ListProtectionGroupsRequest#builder()}
*
*
* @param listProtectionGroupsRequest
* A {@link Consumer} that will call methods on {@link ListProtectionGroupsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListProtectionGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtectionGroups
* @see AWS
* API Documentation
*/
default CompletableFuture listProtectionGroups(
Consumer listProtectionGroupsRequest) {
return listProtectionGroups(ListProtectionGroupsRequest.builder().applyMutation(listProtectionGroupsRequest).build());
}
/**
*
* Retrieves ProtectionGroup objects for the account. You can retrieve all protection groups or you can
* provide filtering criteria and retrieve just the subset of protection groups that match the criteria.
*
*
*
* This is a variant of
* {@link #listProtectionGroups(software.amazon.awssdk.services.shield.model.ListProtectionGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListProtectionGroupsPublisher publisher = client.listProtectionGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListProtectionGroupsPublisher publisher = client.listProtectionGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.shield.model.ListProtectionGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProtectionGroups(software.amazon.awssdk.services.shield.model.ListProtectionGroupsRequest)}
* operation.
*
*
* @param listProtectionGroupsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtectionGroups
* @see AWS
* API Documentation
*/
default ListProtectionGroupsPublisher listProtectionGroupsPaginator(ListProtectionGroupsRequest listProtectionGroupsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves ProtectionGroup objects for the account. You can retrieve all protection groups or you can
* provide filtering criteria and retrieve just the subset of protection groups that match the criteria.
*
*
*
* This is a variant of
* {@link #listProtectionGroups(software.amazon.awssdk.services.shield.model.ListProtectionGroupsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListProtectionGroupsPublisher publisher = client.listProtectionGroupsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListProtectionGroupsPublisher publisher = client.listProtectionGroupsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.shield.model.ListProtectionGroupsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProtectionGroups(software.amazon.awssdk.services.shield.model.ListProtectionGroupsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListProtectionGroupsRequest.Builder} avoiding the
* need to create one manually via {@link ListProtectionGroupsRequest#builder()}
*
*
* @param listProtectionGroupsRequest
* A {@link Consumer} that will call methods on {@link ListProtectionGroupsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtectionGroups
* @see AWS
* API Documentation
*/
default ListProtectionGroupsPublisher listProtectionGroupsPaginator(
Consumer listProtectionGroupsRequest) {
return listProtectionGroupsPaginator(ListProtectionGroupsRequest.builder().applyMutation(listProtectionGroupsRequest)
.build());
}
/**
*
* Retrieves Protection objects for the account. You can retrieve all protections or you can provide
* filtering criteria and retrieve just the subset of protections that match the criteria.
*
*
* @param listProtectionsRequest
* @return A Java Future containing the result of the ListProtections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtections
* @see AWS API
* Documentation
*/
default CompletableFuture listProtections(ListProtectionsRequest listProtectionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves Protection objects for the account. You can retrieve all protections or you can provide
* filtering criteria and retrieve just the subset of protections that match the criteria.
*
*
*
* This is a convenience which creates an instance of the {@link ListProtectionsRequest.Builder} avoiding the need
* to create one manually via {@link ListProtectionsRequest#builder()}
*
*
* @param listProtectionsRequest
* A {@link Consumer} that will call methods on {@link ListProtectionsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListProtections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtections
* @see AWS API
* Documentation
*/
default CompletableFuture listProtections(
Consumer listProtectionsRequest) {
return listProtections(ListProtectionsRequest.builder().applyMutation(listProtectionsRequest).build());
}
/**
*
* Retrieves Protection objects for the account. You can retrieve all protections or you can provide
* filtering criteria and retrieve just the subset of protections that match the criteria.
*
*
* @return A Java Future containing the result of the ListProtections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtections
* @see AWS API
* Documentation
*/
default CompletableFuture listProtections() {
return listProtections(ListProtectionsRequest.builder().build());
}
/**
*
* Retrieves Protection objects for the account. You can retrieve all protections or you can provide
* filtering criteria and retrieve just the subset of protections that match the criteria.
*
*
*
* This is a variant of
* {@link #listProtections(software.amazon.awssdk.services.shield.model.ListProtectionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListProtectionsPublisher publisher = client.listProtectionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListProtectionsPublisher publisher = client.listProtectionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.shield.model.ListProtectionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProtections(software.amazon.awssdk.services.shield.model.ListProtectionsRequest)} operation.
*
*
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtections
* @see AWS API
* Documentation
*/
default ListProtectionsPublisher listProtectionsPaginator() {
return listProtectionsPaginator(ListProtectionsRequest.builder().build());
}
/**
*
* Retrieves Protection objects for the account. You can retrieve all protections or you can provide
* filtering criteria and retrieve just the subset of protections that match the criteria.
*
*
*
* This is a variant of
* {@link #listProtections(software.amazon.awssdk.services.shield.model.ListProtectionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListProtectionsPublisher publisher = client.listProtectionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListProtectionsPublisher publisher = client.listProtectionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.shield.model.ListProtectionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProtections(software.amazon.awssdk.services.shield.model.ListProtectionsRequest)} operation.
*
*
* @param listProtectionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtections
* @see AWS API
* Documentation
*/
default ListProtectionsPublisher listProtectionsPaginator(ListProtectionsRequest listProtectionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves Protection objects for the account. You can retrieve all protections or you can provide
* filtering criteria and retrieve just the subset of protections that match the criteria.
*
*
*
* This is a variant of
* {@link #listProtections(software.amazon.awssdk.services.shield.model.ListProtectionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListProtectionsPublisher publisher = client.listProtectionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListProtectionsPublisher publisher = client.listProtectionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.shield.model.ListProtectionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listProtections(software.amazon.awssdk.services.shield.model.ListProtectionsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListProtectionsRequest.Builder} avoiding the need
* to create one manually via {@link ListProtectionsRequest#builder()}
*
*
* @param listProtectionsRequest
* A {@link Consumer} that will call methods on {@link ListProtectionsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtections
* @see AWS API
* Documentation
*/
default ListProtectionsPublisher listProtectionsPaginator(Consumer listProtectionsRequest) {
return listProtectionsPaginator(ListProtectionsRequest.builder().applyMutation(listProtectionsRequest).build());
}
/**
*
* Retrieves the resources that are included in the protection group.
*
*
* @param listResourcesInProtectionGroupRequest
* @return A Java Future containing the result of the ListResourcesInProtectionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListResourcesInProtectionGroup
* @see AWS API Documentation
*/
default CompletableFuture listResourcesInProtectionGroup(
ListResourcesInProtectionGroupRequest listResourcesInProtectionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the resources that are included in the protection group.
*
*
*
* This is a convenience which creates an instance of the {@link ListResourcesInProtectionGroupRequest.Builder}
* avoiding the need to create one manually via {@link ListResourcesInProtectionGroupRequest#builder()}
*
*
* @param listResourcesInProtectionGroupRequest
* A {@link Consumer} that will call methods on {@link ListResourcesInProtectionGroupRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListResourcesInProtectionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListResourcesInProtectionGroup
* @see AWS API Documentation
*/
default CompletableFuture listResourcesInProtectionGroup(
Consumer listResourcesInProtectionGroupRequest) {
return listResourcesInProtectionGroup(ListResourcesInProtectionGroupRequest.builder()
.applyMutation(listResourcesInProtectionGroupRequest).build());
}
/**
*
* Retrieves the resources that are included in the protection group.
*
*
*
* This is a variant of
* {@link #listResourcesInProtectionGroup(software.amazon.awssdk.services.shield.model.ListResourcesInProtectionGroupRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListResourcesInProtectionGroupPublisher publisher = client.listResourcesInProtectionGroupPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListResourcesInProtectionGroupPublisher publisher = client.listResourcesInProtectionGroupPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.shield.model.ListResourcesInProtectionGroupResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listResourcesInProtectionGroup(software.amazon.awssdk.services.shield.model.ListResourcesInProtectionGroupRequest)}
* operation.
*
*
* @param listResourcesInProtectionGroupRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListResourcesInProtectionGroup
* @see AWS API Documentation
*/
default ListResourcesInProtectionGroupPublisher listResourcesInProtectionGroupPaginator(
ListResourcesInProtectionGroupRequest listResourcesInProtectionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the resources that are included in the protection group.
*
*
*
* This is a variant of
* {@link #listResourcesInProtectionGroup(software.amazon.awssdk.services.shield.model.ListResourcesInProtectionGroupRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListResourcesInProtectionGroupPublisher publisher = client.listResourcesInProtectionGroupPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.shield.paginators.ListResourcesInProtectionGroupPublisher publisher = client.listResourcesInProtectionGroupPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.shield.model.ListResourcesInProtectionGroupResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listResourcesInProtectionGroup(software.amazon.awssdk.services.shield.model.ListResourcesInProtectionGroupRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListResourcesInProtectionGroupRequest.Builder}
* avoiding the need to create one manually via {@link ListResourcesInProtectionGroupRequest#builder()}
*
*
* @param listResourcesInProtectionGroupRequest
* A {@link Consumer} that will call methods on {@link ListResourcesInProtectionGroupRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListResourcesInProtectionGroup
* @see AWS API Documentation
*/
default ListResourcesInProtectionGroupPublisher listResourcesInProtectionGroupPaginator(
Consumer listResourcesInProtectionGroupRequest) {
return listResourcesInProtectionGroupPaginator(ListResourcesInProtectionGroupRequest.builder()
.applyMutation(listResourcesInProtectionGroupRequest).build());
}
/**
*
* Gets information about Amazon Web Services tags for a specified Amazon Resource Name (ARN) in Shield.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about Amazon Web Services tags for a specified Amazon Resource Name (ARN) in Shield.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Adds or updates tags for a resource in Shield.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds or updates tags for a resource in Shield.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from a resource in Shield.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from a resource in Shield.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates an existing Shield Advanced automatic application layer DDoS mitigation configuration for the specified
* resource.
*
*
* @param updateApplicationLayerAutomaticResponseRequest
* @return A Java Future containing the result of the UpdateApplicationLayerAutomaticResponse operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateApplicationLayerAutomaticResponse
* @see AWS API Documentation
*/
default CompletableFuture updateApplicationLayerAutomaticResponse(
UpdateApplicationLayerAutomaticResponseRequest updateApplicationLayerAutomaticResponseRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing Shield Advanced automatic application layer DDoS mitigation configuration for the specified
* resource.
*
*
*
* This is a convenience which creates an instance of the
* {@link UpdateApplicationLayerAutomaticResponseRequest.Builder} avoiding the need to create one manually via
* {@link UpdateApplicationLayerAutomaticResponseRequest#builder()}
*
*
* @param updateApplicationLayerAutomaticResponseRequest
* A {@link Consumer} that will call methods on
* {@link UpdateApplicationLayerAutomaticResponseRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateApplicationLayerAutomaticResponse operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateApplicationLayerAutomaticResponse
* @see AWS API Documentation
*/
default CompletableFuture updateApplicationLayerAutomaticResponse(
Consumer updateApplicationLayerAutomaticResponseRequest) {
return updateApplicationLayerAutomaticResponse(UpdateApplicationLayerAutomaticResponseRequest.builder()
.applyMutation(updateApplicationLayerAutomaticResponseRequest).build());
}
/**
*
* Updates the details of the list of email addresses and phone numbers that the Shield Response Team (SRT) can use
* to contact you if you have proactive engagement enabled, for escalations to the SRT and to initiate proactive
* customer support.
*
*
* @param updateEmergencyContactSettingsRequest
* @return A Java Future containing the result of the UpdateEmergencyContactSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateEmergencyContactSettings
* @see AWS API Documentation
*/
default CompletableFuture updateEmergencyContactSettings(
UpdateEmergencyContactSettingsRequest updateEmergencyContactSettingsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the details of the list of email addresses and phone numbers that the Shield Response Team (SRT) can use
* to contact you if you have proactive engagement enabled, for escalations to the SRT and to initiate proactive
* customer support.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateEmergencyContactSettingsRequest.Builder}
* avoiding the need to create one manually via {@link UpdateEmergencyContactSettingsRequest#builder()}
*
*
* @param updateEmergencyContactSettingsRequest
* A {@link Consumer} that will call methods on {@link UpdateEmergencyContactSettingsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateEmergencyContactSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateEmergencyContactSettings
* @see AWS API Documentation
*/
default CompletableFuture updateEmergencyContactSettings(
Consumer updateEmergencyContactSettingsRequest) {
return updateEmergencyContactSettings(UpdateEmergencyContactSettingsRequest.builder()
.applyMutation(updateEmergencyContactSettingsRequest).build());
}
/**
*
* Updates an existing protection group. A protection group is a grouping of protected resources so they can be
* handled as a collective. This resource grouping improves the accuracy of detection and reduces false positives.
*
*
* @param updateProtectionGroupRequest
* @return A Java Future containing the result of the UpdateProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateProtectionGroup
* @see AWS
* API Documentation
*/
default CompletableFuture updateProtectionGroup(
UpdateProtectionGroupRequest updateProtectionGroupRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing protection group. A protection group is a grouping of protected resources so they can be
* handled as a collective. This resource grouping improves the accuracy of detection and reduces false positives.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateProtectionGroupRequest.Builder} avoiding the
* need to create one manually via {@link UpdateProtectionGroupRequest#builder()}
*
*
* @param updateProtectionGroupRequest
* A {@link Consumer} that will call methods on {@link UpdateProtectionGroupRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateProtectionGroup
* @see AWS
* API Documentation
*/
default CompletableFuture updateProtectionGroup(
Consumer updateProtectionGroupRequest) {
return updateProtectionGroup(UpdateProtectionGroupRequest.builder().applyMutation(updateProtectionGroupRequest).build());
}
/**
*
* Updates the details of an existing subscription. Only enter values for parameters you want to change. Empty
* parameters are not updated.
*
*
*
* For accounts that are members of an Organizations organization, Shield Advanced subscriptions are billed against
* the organization's payer account, regardless of whether the payer account itself is subscribed.
*
*
*
* @param updateSubscriptionRequest
* @return A Java Future containing the result of the UpdateSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - LockedSubscriptionException You are trying to update a subscription that has not yet completed the
* 1-year commitment. You can change the
AutoRenew
parameter during the last 30 days of your
* subscription. This exception indicates that you are attempting to change AutoRenew
prior to
* that period.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateSubscription
* @see AWS API
* Documentation
*/
default CompletableFuture updateSubscription(UpdateSubscriptionRequest updateSubscriptionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the details of an existing subscription. Only enter values for parameters you want to change. Empty
* parameters are not updated.
*
*
*
* For accounts that are members of an Organizations organization, Shield Advanced subscriptions are billed against
* the organization's payer account, regardless of whether the payer account itself is subscribed.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSubscriptionRequest.Builder} avoiding the
* need to create one manually via {@link UpdateSubscriptionRequest#builder()}
*
*
* @param updateSubscriptionRequest
* A {@link Consumer} that will call methods on {@link UpdateSubscriptionRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - LockedSubscriptionException You are trying to update a subscription that has not yet completed the
* 1-year commitment. You can change the
AutoRenew
parameter during the last 30 days of your
* subscription. This exception indicates that you are attempting to change AutoRenew
prior to
* that period.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateSubscription
* @see AWS API
* Documentation
*/
default CompletableFuture updateSubscription(
Consumer updateSubscriptionRequest) {
return updateSubscription(UpdateSubscriptionRequest.builder().applyMutation(updateSubscriptionRequest).build());
}
}