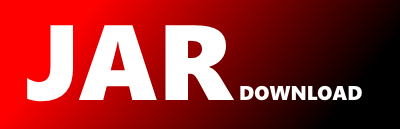
software.amazon.awssdk.services.shield.model.ProtectionGroup Maven / Gradle / Ivy
Show all versions of shield Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.shield.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A grouping of protected resources that you and Shield Advanced can monitor as a collective. This resource grouping
* improves the accuracy of detection and reduces false positives.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ProtectionGroup implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField PROTECTION_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProtectionGroupId").getter(getter(ProtectionGroup::protectionGroupId))
.setter(setter(Builder::protectionGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProtectionGroupId").build()).build();
private static final SdkField AGGREGATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Aggregation").getter(getter(ProtectionGroup::aggregationAsString)).setter(setter(Builder::aggregation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Aggregation").build()).build();
private static final SdkField PATTERN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Pattern")
.getter(getter(ProtectionGroup::patternAsString)).setter(setter(Builder::pattern))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Pattern").build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ResourceType").getter(getter(ProtectionGroup::resourceTypeAsString))
.setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ResourceType").build()).build();
private static final SdkField> MEMBERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Members")
.getter(getter(ProtectionGroup::members))
.setter(setter(Builder::members))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Members").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PROTECTION_GROUP_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProtectionGroupArn").getter(getter(ProtectionGroup::protectionGroupArn))
.setter(setter(Builder::protectionGroupArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProtectionGroupArn").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PROTECTION_GROUP_ID_FIELD,
AGGREGATION_FIELD, PATTERN_FIELD, RESOURCE_TYPE_FIELD, MEMBERS_FIELD, PROTECTION_GROUP_ARN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String protectionGroupId;
private final String aggregation;
private final String pattern;
private final String resourceType;
private final List members;
private final String protectionGroupArn;
private ProtectionGroup(BuilderImpl builder) {
this.protectionGroupId = builder.protectionGroupId;
this.aggregation = builder.aggregation;
this.pattern = builder.pattern;
this.resourceType = builder.resourceType;
this.members = builder.members;
this.protectionGroupArn = builder.protectionGroupArn;
}
/**
*
* The name of the protection group. You use this to identify the protection group in lists and to manage the
* protection group, for example to update, delete, or describe it.
*
*
* @return The name of the protection group. You use this to identify the protection group in lists and to manage
* the protection group, for example to update, delete, or describe it.
*/
public final String protectionGroupId() {
return protectionGroupId;
}
/**
*
* Defines how Shield combines resource data for the group in order to detect, mitigate, and report events.
*
*
* -
*
* Sum - Use the total traffic across the group. This is a good choice for most cases. Examples include Elastic IP
* addresses for EC2 instances that scale manually or automatically.
*
*
* -
*
* Mean - Use the average of the traffic across the group. This is a good choice for resources that share traffic
* uniformly. Examples include accelerators and load balancers.
*
*
* -
*
* Max - Use the highest traffic from each resource. This is useful for resources that don't share traffic and for
* resources that share that traffic in a non-uniform way. Examples include Amazon CloudFront distributions and
* origin resources for CloudFront distributions.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #aggregation} will
* return {@link ProtectionGroupAggregation#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #aggregationAsString}.
*
*
* @return Defines how Shield combines resource data for the group in order to detect, mitigate, and report
* events.
*
* -
*
* Sum - Use the total traffic across the group. This is a good choice for most cases. Examples include
* Elastic IP addresses for EC2 instances that scale manually or automatically.
*
*
* -
*
* Mean - Use the average of the traffic across the group. This is a good choice for resources that share
* traffic uniformly. Examples include accelerators and load balancers.
*
*
* -
*
* Max - Use the highest traffic from each resource. This is useful for resources that don't share traffic
* and for resources that share that traffic in a non-uniform way. Examples include Amazon CloudFront
* distributions and origin resources for CloudFront distributions.
*
*
* @see ProtectionGroupAggregation
*/
public final ProtectionGroupAggregation aggregation() {
return ProtectionGroupAggregation.fromValue(aggregation);
}
/**
*
* Defines how Shield combines resource data for the group in order to detect, mitigate, and report events.
*
*
* -
*
* Sum - Use the total traffic across the group. This is a good choice for most cases. Examples include Elastic IP
* addresses for EC2 instances that scale manually or automatically.
*
*
* -
*
* Mean - Use the average of the traffic across the group. This is a good choice for resources that share traffic
* uniformly. Examples include accelerators and load balancers.
*
*
* -
*
* Max - Use the highest traffic from each resource. This is useful for resources that don't share traffic and for
* resources that share that traffic in a non-uniform way. Examples include Amazon CloudFront distributions and
* origin resources for CloudFront distributions.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #aggregation} will
* return {@link ProtectionGroupAggregation#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #aggregationAsString}.
*
*
* @return Defines how Shield combines resource data for the group in order to detect, mitigate, and report
* events.
*
* -
*
* Sum - Use the total traffic across the group. This is a good choice for most cases. Examples include
* Elastic IP addresses for EC2 instances that scale manually or automatically.
*
*
* -
*
* Mean - Use the average of the traffic across the group. This is a good choice for resources that share
* traffic uniformly. Examples include accelerators and load balancers.
*
*
* -
*
* Max - Use the highest traffic from each resource. This is useful for resources that don't share traffic
* and for resources that share that traffic in a non-uniform way. Examples include Amazon CloudFront
* distributions and origin resources for CloudFront distributions.
*
*
* @see ProtectionGroupAggregation
*/
public final String aggregationAsString() {
return aggregation;
}
/**
*
* The criteria to use to choose the protected resources for inclusion in the group. You can include all resources
* that have protections, provide a list of resource ARNs (Amazon Resource Names), or include all resources of a
* specified resource type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pattern} will
* return {@link ProtectionGroupPattern#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #patternAsString}.
*
*
* @return The criteria to use to choose the protected resources for inclusion in the group. You can include all
* resources that have protections, provide a list of resource ARNs (Amazon Resource Names), or include all
* resources of a specified resource type.
* @see ProtectionGroupPattern
*/
public final ProtectionGroupPattern pattern() {
return ProtectionGroupPattern.fromValue(pattern);
}
/**
*
* The criteria to use to choose the protected resources for inclusion in the group. You can include all resources
* that have protections, provide a list of resource ARNs (Amazon Resource Names), or include all resources of a
* specified resource type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pattern} will
* return {@link ProtectionGroupPattern#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #patternAsString}.
*
*
* @return The criteria to use to choose the protected resources for inclusion in the group. You can include all
* resources that have protections, provide a list of resource ARNs (Amazon Resource Names), or include all
* resources of a specified resource type.
* @see ProtectionGroupPattern
*/
public final String patternAsString() {
return pattern;
}
/**
*
* The resource type to include in the protection group. All protected resources of this type are included in the
* protection group. You must set this when you set Pattern
to BY_RESOURCE_TYPE
and you
* must not set it for any other Pattern
setting.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceType} will
* return {@link ProtectedResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resourceTypeAsString}.
*
*
* @return The resource type to include in the protection group. All protected resources of this type are included
* in the protection group. You must set this when you set Pattern
to
* BY_RESOURCE_TYPE
and you must not set it for any other Pattern
setting.
* @see ProtectedResourceType
*/
public final ProtectedResourceType resourceType() {
return ProtectedResourceType.fromValue(resourceType);
}
/**
*
* The resource type to include in the protection group. All protected resources of this type are included in the
* protection group. You must set this when you set Pattern
to BY_RESOURCE_TYPE
and you
* must not set it for any other Pattern
setting.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceType} will
* return {@link ProtectedResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #resourceTypeAsString}.
*
*
* @return The resource type to include in the protection group. All protected resources of this type are included
* in the protection group. You must set this when you set Pattern
to
* BY_RESOURCE_TYPE
and you must not set it for any other Pattern
setting.
* @see ProtectedResourceType
*/
public final String resourceTypeAsString() {
return resourceType;
}
/**
* For responses, this returns true if the service returned a value for the Members property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasMembers() {
return members != null && !(members instanceof SdkAutoConstructList);
}
/**
*
* The ARNs (Amazon Resource Names) of the resources to include in the protection group. You must set this when you
* set Pattern
to ARBITRARY
and you must not set it for any other Pattern
* setting.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMembers} method.
*
*
* @return The ARNs (Amazon Resource Names) of the resources to include in the protection group. You must set this
* when you set Pattern
to ARBITRARY
and you must not set it for any other
* Pattern
setting.
*/
public final List members() {
return members;
}
/**
*
* The ARN (Amazon Resource Name) of the protection group.
*
*
* @return The ARN (Amazon Resource Name) of the protection group.
*/
public final String protectionGroupArn() {
return protectionGroupArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(protectionGroupId());
hashCode = 31 * hashCode + Objects.hashCode(aggregationAsString());
hashCode = 31 * hashCode + Objects.hashCode(patternAsString());
hashCode = 31 * hashCode + Objects.hashCode(resourceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasMembers() ? members() : null);
hashCode = 31 * hashCode + Objects.hashCode(protectionGroupArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ProtectionGroup)) {
return false;
}
ProtectionGroup other = (ProtectionGroup) obj;
return Objects.equals(protectionGroupId(), other.protectionGroupId())
&& Objects.equals(aggregationAsString(), other.aggregationAsString())
&& Objects.equals(patternAsString(), other.patternAsString())
&& Objects.equals(resourceTypeAsString(), other.resourceTypeAsString()) && hasMembers() == other.hasMembers()
&& Objects.equals(members(), other.members()) && Objects.equals(protectionGroupArn(), other.protectionGroupArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ProtectionGroup").add("ProtectionGroupId", protectionGroupId())
.add("Aggregation", aggregationAsString()).add("Pattern", patternAsString())
.add("ResourceType", resourceTypeAsString()).add("Members", hasMembers() ? members() : null)
.add("ProtectionGroupArn", protectionGroupArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ProtectionGroupId":
return Optional.ofNullable(clazz.cast(protectionGroupId()));
case "Aggregation":
return Optional.ofNullable(clazz.cast(aggregationAsString()));
case "Pattern":
return Optional.ofNullable(clazz.cast(patternAsString()));
case "ResourceType":
return Optional.ofNullable(clazz.cast(resourceTypeAsString()));
case "Members":
return Optional.ofNullable(clazz.cast(members()));
case "ProtectionGroupArn":
return Optional.ofNullable(clazz.cast(protectionGroupArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("ProtectionGroupId", PROTECTION_GROUP_ID_FIELD);
map.put("Aggregation", AGGREGATION_FIELD);
map.put("Pattern", PATTERN_FIELD);
map.put("ResourceType", RESOURCE_TYPE_FIELD);
map.put("Members", MEMBERS_FIELD);
map.put("ProtectionGroupArn", PROTECTION_GROUP_ARN_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function