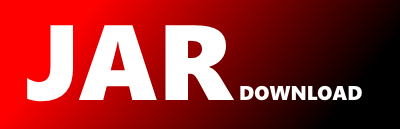
software.amazon.awssdk.services.shield.DefaultShieldAsyncClient Maven / Gradle / Ivy
Show all versions of shield Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.shield;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import java.util.function.Function;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.shield.internal.ShieldServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.shield.model.AccessDeniedException;
import software.amazon.awssdk.services.shield.model.AccessDeniedForDependencyException;
import software.amazon.awssdk.services.shield.model.AssociateDrtLogBucketRequest;
import software.amazon.awssdk.services.shield.model.AssociateDrtLogBucketResponse;
import software.amazon.awssdk.services.shield.model.AssociateDrtRoleRequest;
import software.amazon.awssdk.services.shield.model.AssociateDrtRoleResponse;
import software.amazon.awssdk.services.shield.model.AssociateHealthCheckRequest;
import software.amazon.awssdk.services.shield.model.AssociateHealthCheckResponse;
import software.amazon.awssdk.services.shield.model.AssociateProactiveEngagementDetailsRequest;
import software.amazon.awssdk.services.shield.model.AssociateProactiveEngagementDetailsResponse;
import software.amazon.awssdk.services.shield.model.CreateProtectionGroupRequest;
import software.amazon.awssdk.services.shield.model.CreateProtectionGroupResponse;
import software.amazon.awssdk.services.shield.model.CreateProtectionRequest;
import software.amazon.awssdk.services.shield.model.CreateProtectionResponse;
import software.amazon.awssdk.services.shield.model.CreateSubscriptionRequest;
import software.amazon.awssdk.services.shield.model.CreateSubscriptionResponse;
import software.amazon.awssdk.services.shield.model.DeleteProtectionGroupRequest;
import software.amazon.awssdk.services.shield.model.DeleteProtectionGroupResponse;
import software.amazon.awssdk.services.shield.model.DeleteProtectionRequest;
import software.amazon.awssdk.services.shield.model.DeleteProtectionResponse;
import software.amazon.awssdk.services.shield.model.DescribeAttackRequest;
import software.amazon.awssdk.services.shield.model.DescribeAttackResponse;
import software.amazon.awssdk.services.shield.model.DescribeAttackStatisticsRequest;
import software.amazon.awssdk.services.shield.model.DescribeAttackStatisticsResponse;
import software.amazon.awssdk.services.shield.model.DescribeDrtAccessRequest;
import software.amazon.awssdk.services.shield.model.DescribeDrtAccessResponse;
import software.amazon.awssdk.services.shield.model.DescribeEmergencyContactSettingsRequest;
import software.amazon.awssdk.services.shield.model.DescribeEmergencyContactSettingsResponse;
import software.amazon.awssdk.services.shield.model.DescribeProtectionGroupRequest;
import software.amazon.awssdk.services.shield.model.DescribeProtectionGroupResponse;
import software.amazon.awssdk.services.shield.model.DescribeProtectionRequest;
import software.amazon.awssdk.services.shield.model.DescribeProtectionResponse;
import software.amazon.awssdk.services.shield.model.DescribeSubscriptionRequest;
import software.amazon.awssdk.services.shield.model.DescribeSubscriptionResponse;
import software.amazon.awssdk.services.shield.model.DisableApplicationLayerAutomaticResponseRequest;
import software.amazon.awssdk.services.shield.model.DisableApplicationLayerAutomaticResponseResponse;
import software.amazon.awssdk.services.shield.model.DisableProactiveEngagementRequest;
import software.amazon.awssdk.services.shield.model.DisableProactiveEngagementResponse;
import software.amazon.awssdk.services.shield.model.DisassociateDrtLogBucketRequest;
import software.amazon.awssdk.services.shield.model.DisassociateDrtLogBucketResponse;
import software.amazon.awssdk.services.shield.model.DisassociateDrtRoleRequest;
import software.amazon.awssdk.services.shield.model.DisassociateDrtRoleResponse;
import software.amazon.awssdk.services.shield.model.DisassociateHealthCheckRequest;
import software.amazon.awssdk.services.shield.model.DisassociateHealthCheckResponse;
import software.amazon.awssdk.services.shield.model.EnableApplicationLayerAutomaticResponseRequest;
import software.amazon.awssdk.services.shield.model.EnableApplicationLayerAutomaticResponseResponse;
import software.amazon.awssdk.services.shield.model.EnableProactiveEngagementRequest;
import software.amazon.awssdk.services.shield.model.EnableProactiveEngagementResponse;
import software.amazon.awssdk.services.shield.model.GetSubscriptionStateRequest;
import software.amazon.awssdk.services.shield.model.GetSubscriptionStateResponse;
import software.amazon.awssdk.services.shield.model.InternalErrorException;
import software.amazon.awssdk.services.shield.model.InvalidOperationException;
import software.amazon.awssdk.services.shield.model.InvalidPaginationTokenException;
import software.amazon.awssdk.services.shield.model.InvalidParameterException;
import software.amazon.awssdk.services.shield.model.InvalidResourceException;
import software.amazon.awssdk.services.shield.model.LimitsExceededException;
import software.amazon.awssdk.services.shield.model.ListAttacksRequest;
import software.amazon.awssdk.services.shield.model.ListAttacksResponse;
import software.amazon.awssdk.services.shield.model.ListProtectionGroupsRequest;
import software.amazon.awssdk.services.shield.model.ListProtectionGroupsResponse;
import software.amazon.awssdk.services.shield.model.ListProtectionsRequest;
import software.amazon.awssdk.services.shield.model.ListProtectionsResponse;
import software.amazon.awssdk.services.shield.model.ListResourcesInProtectionGroupRequest;
import software.amazon.awssdk.services.shield.model.ListResourcesInProtectionGroupResponse;
import software.amazon.awssdk.services.shield.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.shield.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.shield.model.LockedSubscriptionException;
import software.amazon.awssdk.services.shield.model.NoAssociatedRoleException;
import software.amazon.awssdk.services.shield.model.OptimisticLockException;
import software.amazon.awssdk.services.shield.model.ResourceAlreadyExistsException;
import software.amazon.awssdk.services.shield.model.ResourceNotFoundException;
import software.amazon.awssdk.services.shield.model.ShieldException;
import software.amazon.awssdk.services.shield.model.TagResourceRequest;
import software.amazon.awssdk.services.shield.model.TagResourceResponse;
import software.amazon.awssdk.services.shield.model.UntagResourceRequest;
import software.amazon.awssdk.services.shield.model.UntagResourceResponse;
import software.amazon.awssdk.services.shield.model.UpdateApplicationLayerAutomaticResponseRequest;
import software.amazon.awssdk.services.shield.model.UpdateApplicationLayerAutomaticResponseResponse;
import software.amazon.awssdk.services.shield.model.UpdateEmergencyContactSettingsRequest;
import software.amazon.awssdk.services.shield.model.UpdateEmergencyContactSettingsResponse;
import software.amazon.awssdk.services.shield.model.UpdateProtectionGroupRequest;
import software.amazon.awssdk.services.shield.model.UpdateProtectionGroupResponse;
import software.amazon.awssdk.services.shield.model.UpdateSubscriptionRequest;
import software.amazon.awssdk.services.shield.model.UpdateSubscriptionResponse;
import software.amazon.awssdk.services.shield.transform.AssociateDrtLogBucketRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.AssociateDrtRoleRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.AssociateHealthCheckRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.AssociateProactiveEngagementDetailsRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.CreateProtectionGroupRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.CreateProtectionRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.CreateSubscriptionRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DeleteProtectionGroupRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DeleteProtectionRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DescribeAttackRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DescribeAttackStatisticsRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DescribeDrtAccessRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DescribeEmergencyContactSettingsRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DescribeProtectionGroupRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DescribeProtectionRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DescribeSubscriptionRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DisableApplicationLayerAutomaticResponseRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DisableProactiveEngagementRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DisassociateDrtLogBucketRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DisassociateDrtRoleRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.DisassociateHealthCheckRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.EnableApplicationLayerAutomaticResponseRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.EnableProactiveEngagementRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.GetSubscriptionStateRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.ListAttacksRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.ListProtectionGroupsRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.ListProtectionsRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.ListResourcesInProtectionGroupRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.UpdateApplicationLayerAutomaticResponseRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.UpdateEmergencyContactSettingsRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.UpdateProtectionGroupRequestMarshaller;
import software.amazon.awssdk.services.shield.transform.UpdateSubscriptionRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link ShieldAsyncClient}.
*
* @see ShieldAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultShieldAsyncClient implements ShieldAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultShieldAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultShieldAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Authorizes the Shield Response Team (SRT) to access the specified Amazon S3 bucket containing log data such as
* Application Load Balancer access logs, CloudFront logs, or logs from third party sources. You can associate up to
* 10 Amazon S3 buckets with your subscription.
*
*
* To use the services of the SRT and make an AssociateDRTLogBucket
request, you must be subscribed to
* the Business Support plan or the Enterprise Support plan.
*
*
* @param associateDrtLogBucketRequest
* @return A Java Future containing the result of the AssociateDRTLogBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - NoAssociatedRoleException The ARN of the role that you specified does not exist.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - AccessDeniedForDependencyException In order to grant the necessary access to the Shield Response Team
* (SRT) the user submitting the request must have the
iam:PassRole
permission. This error
* indicates the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an Amazon Web Services Service.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateDRTLogBucket
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture associateDRTLogBucket(
AssociateDrtLogBucketRequest associateDrtLogBucketRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateDrtLogBucketRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateDrtLogBucketRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateDRTLogBucket");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateDrtLogBucketResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateDRTLogBucket").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateDrtLogBucketRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateDrtLogBucketRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Authorizes the Shield Response Team (SRT) using the specified role, to access your Amazon Web Services account to
* assist with DDoS attack mitigation during potential attacks. This enables the SRT to inspect your WAF
* configuration and create or update WAF rules and web ACLs.
*
*
* You can associate only one RoleArn
with your subscription. If you submit an
* AssociateDRTRole
request for an account that already has an associated role, the new
* RoleArn
will replace the existing RoleArn
.
*
*
* Prior to making the AssociateDRTRole
request, you must attach the
* AWSShieldDRTAccessPolicy
managed policy to the role that you'll specify in the request. You can
* access this policy in the IAM console at AWSShieldDRTAccessPolicy. For more information see Adding and
* removing IAM identity permissions. The role must also trust the service principal
* drt.shield.amazonaws.com
. For more information, see IAM JSON
* policy elements: Principal.
*
*
* The SRT will have access only to your WAF and Shield resources. By submitting this request, you authorize the SRT
* to inspect your WAF and Shield configuration and create and update WAF rules and web ACLs on your behalf. The SRT
* takes these actions only if explicitly authorized by you.
*
*
* You must have the iam:PassRole
permission to make an AssociateDRTRole
request. For more
* information, see Granting a
* user permissions to pass a role to an Amazon Web Services service.
*
*
* To use the services of the SRT and make an AssociateDRTRole
request, you must be subscribed to the
* Business Support plan or the Enterprise Support plan.
*
*
* @param associateDrtRoleRequest
* @return A Java Future containing the result of the AssociateDRTRole operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - AccessDeniedForDependencyException In order to grant the necessary access to the Shield Response Team
* (SRT) the user submitting the request must have the
iam:PassRole
permission. This error
* indicates the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an Amazon Web Services Service.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateDRTRole
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture associateDRTRole(AssociateDrtRoleRequest associateDrtRoleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateDrtRoleRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateDrtRoleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateDRTRole");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateDrtRoleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateDRTRole").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateDrtRoleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateDrtRoleRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds health-based detection to the Shield Advanced protection for a resource. Shield Advanced health-based
* detection uses the health of your Amazon Web Services resource to improve responsiveness and accuracy in attack
* detection and response.
*
*
* You define the health check in Route 53 and then associate it with your Shield Advanced protection. For more
* information, see Shield Advanced Health-Based Detection in the WAF Developer Guide.
*
*
* @param associateHealthCheckRequest
* @return A Java Future containing the result of the AssociateHealthCheck operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateHealthCheck
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture associateHealthCheck(
AssociateHealthCheckRequest associateHealthCheckRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateHealthCheckRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateHealthCheckRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateHealthCheck");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateHealthCheckResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateHealthCheck").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateHealthCheckRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateHealthCheckRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Initializes proactive engagement and sets the list of contacts for the Shield Response Team (SRT) to use. You
* must provide at least one phone number in the emergency contact list.
*
*
* After you have initialized proactive engagement using this call, to disable or enable proactive engagement, use
* the calls DisableProactiveEngagement
and EnableProactiveEngagement
.
*
*
*
* This call defines the list of email addresses and phone numbers that the SRT can use to contact you for
* escalations to the SRT and to initiate proactive customer support.
*
*
* The contacts that you provide in the request replace any contacts that were already defined. If you already have
* contacts defined and want to use them, retrieve the list using DescribeEmergencyContactSettings
and
* then provide it to this call.
*
*
*
* @param associateProactiveEngagementDetailsRequest
* @return A Java Future containing the result of the AssociateProactiveEngagementDetails operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.AssociateProactiveEngagementDetails
* @see AWS API Documentation
*/
@Override
public CompletableFuture associateProactiveEngagementDetails(
AssociateProactiveEngagementDetailsRequest associateProactiveEngagementDetailsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateProactiveEngagementDetailsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
associateProactiveEngagementDetailsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateProactiveEngagementDetails");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, AssociateProactiveEngagementDetailsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateProactiveEngagementDetails").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateProactiveEngagementDetailsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateProactiveEngagementDetailsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enables Shield Advanced for a specific Amazon Web Services resource. The resource can be an Amazon CloudFront
* distribution, Amazon Route 53 hosted zone, Global Accelerator standard accelerator, Elastic IP Address,
* Application Load Balancer, or a Classic Load Balancer. You can protect Amazon EC2 instances and Network Load
* Balancers by association with protected Amazon EC2 Elastic IP addresses.
*
*
* You can add protection to only a single resource with each CreateProtection
request. You can add
* protection to multiple resources at once through the Shield Advanced console at https://console.aws.amazon.com/wafv2/shieldv2#/. For
* more information see Getting Started with
* Shield Advanced and Adding Shield Advanced
* protection to Amazon Web Services resources.
*
*
* @param createProtectionRequest
* @return A Java Future containing the result of the CreateProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - ResourceAlreadyExistsException Exception indicating the specified resource already exists. If
* available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.CreateProtection
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createProtection(CreateProtectionRequest createProtectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createProtectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createProtectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateProtection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateProtectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateProtection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateProtectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createProtectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a grouping of protected resources so they can be handled as a collective. This resource grouping improves
* the accuracy of detection and reduces false positives.
*
*
* @param createProtectionGroupRequest
* @return A Java Future containing the result of the CreateProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceAlreadyExistsException Exception indicating the specified resource already exists. If
* available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.CreateProtectionGroup
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createProtectionGroup(
CreateProtectionGroupRequest createProtectionGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createProtectionGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createProtectionGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateProtectionGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateProtectionGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateProtectionGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateProtectionGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createProtectionGroupRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Activates Shield Advanced for an account.
*
*
*
* For accounts that are members of an Organizations organization, Shield Advanced subscriptions are billed against
* the organization's payer account, regardless of whether the payer account itself is subscribed.
*
*
*
* When you initially create a subscription, your subscription is set to be automatically renewed at the end of the
* existing subscription period. You can change this by submitting an UpdateSubscription
request.
*
*
* @param createSubscriptionRequest
* @return A Java Future containing the result of the CreateSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceAlreadyExistsException Exception indicating the specified resource already exists. If
* available, this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.CreateSubscription
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createSubscription(CreateSubscriptionRequest createSubscriptionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createSubscriptionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSubscriptionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSubscription");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateSubscriptionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateSubscription").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateSubscriptionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createSubscriptionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an Shield Advanced Protection.
*
*
* @param deleteProtectionRequest
* @return A Java Future containing the result of the DeleteProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DeleteProtection
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteProtection(DeleteProtectionRequest deleteProtectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteProtectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteProtectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteProtection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteProtectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteProtection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteProtectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteProtectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the specified protection group.
*
*
* @param deleteProtectionGroupRequest
* @return A Java Future containing the result of the DeleteProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DeleteProtectionGroup
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteProtectionGroup(
DeleteProtectionGroupRequest deleteProtectionGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteProtectionGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteProtectionGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteProtectionGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteProtectionGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteProtectionGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteProtectionGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteProtectionGroupRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the details of a DDoS attack.
*
*
* @param describeAttackRequest
* @return A Java Future containing the result of the DescribeAttack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - AccessDeniedException Exception that indicates the specified
AttackId
does not exist, or
* the requester does not have the appropriate permissions to access the AttackId
.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeAttack
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeAttack(DescribeAttackRequest describeAttackRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAttackRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAttackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAttack");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAttackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAttack").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeAttackRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeAttackRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides information about the number and type of attacks Shield has detected in the last year for all resources
* that belong to your account, regardless of whether you've defined Shield protections for them. This operation is
* available to Shield customers as well as to Shield Advanced customers.
*
*
* The operation returns data for the time range of midnight UTC, one year ago, to midnight UTC, today. For example,
* if the current time is 2020-10-26 15:39:32 PDT
, equal to 2020-10-26 22:39:32 UTC
, then
* the time range for the attack data returned is from 2019-10-26 00:00:00 UTC
to
* 2020-10-26 00:00:00 UTC
.
*
*
* The time range indicates the period covered by the attack statistics data items.
*
*
* @param describeAttackStatisticsRequest
* @return A Java Future containing the result of the DescribeAttackStatistics operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeAttackStatistics
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeAttackStatistics(
DescribeAttackStatisticsRequest describeAttackStatisticsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAttackStatisticsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAttackStatisticsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAttackStatistics");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAttackStatisticsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAttackStatistics").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeAttackStatisticsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeAttackStatisticsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the current role and list of Amazon S3 log buckets used by the Shield Response Team (SRT) to access your
* Amazon Web Services account while assisting with attack mitigation.
*
*
* @param describeDrtAccessRequest
* @return A Java Future containing the result of the DescribeDRTAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeDRTAccess
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeDRTAccess(DescribeDrtAccessRequest describeDrtAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDrtAccessRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDrtAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDRTAccess");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDrtAccessResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDRTAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDrtAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDrtAccessRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* A list of email addresses and phone numbers that the Shield Response Team (SRT) can use to contact you if you
* have proactive engagement enabled, for escalations to the SRT and to initiate proactive customer support.
*
*
* @param describeEmergencyContactSettingsRequest
* @return A Java Future containing the result of the DescribeEmergencyContactSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeEmergencyContactSettings
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeEmergencyContactSettings(
DescribeEmergencyContactSettingsRequest describeEmergencyContactSettingsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeEmergencyContactSettingsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeEmergencyContactSettingsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEmergencyContactSettings");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DescribeEmergencyContactSettingsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeEmergencyContactSettings").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeEmergencyContactSettingsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeEmergencyContactSettingsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the details of a Protection object.
*
*
* @param describeProtectionRequest
* @return A Java Future containing the result of the DescribeProtection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeProtection
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeProtection(DescribeProtectionRequest describeProtectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeProtectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeProtectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeProtection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeProtectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeProtection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeProtectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeProtectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the specification for the specified protection group.
*
*
* @param describeProtectionGroupRequest
* @return A Java Future containing the result of the DescribeProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeProtectionGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeProtectionGroup(
DescribeProtectionGroupRequest describeProtectionGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeProtectionGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeProtectionGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeProtectionGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeProtectionGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeProtectionGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeProtectionGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeProtectionGroupRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides details about the Shield Advanced subscription for an account.
*
*
* @param describeSubscriptionRequest
* @return A Java Future containing the result of the DescribeSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DescribeSubscription
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeSubscription(
DescribeSubscriptionRequest describeSubscriptionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeSubscriptionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeSubscriptionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSubscription");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSubscriptionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeSubscription").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeSubscriptionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeSubscriptionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disable the Shield Advanced automatic application layer DDoS mitigation feature for the protected resource. This
* stops Shield Advanced from creating, verifying, and applying WAF rules for attacks that it detects for the
* resource.
*
*
* @param disableApplicationLayerAutomaticResponseRequest
* @return A Java Future containing the result of the DisableApplicationLayerAutomaticResponse operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisableApplicationLayerAutomaticResponse
* @see AWS API Documentation
*/
@Override
public CompletableFuture disableApplicationLayerAutomaticResponse(
DisableApplicationLayerAutomaticResponseRequest disableApplicationLayerAutomaticResponseRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(
disableApplicationLayerAutomaticResponseRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disableApplicationLayerAutomaticResponseRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisableApplicationLayerAutomaticResponse");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DisableApplicationLayerAutomaticResponseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisableApplicationLayerAutomaticResponse").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisableApplicationLayerAutomaticResponseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disableApplicationLayerAutomaticResponseRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes authorization from the Shield Response Team (SRT) to notify contacts about escalations to the SRT and to
* initiate proactive customer support.
*
*
* @param disableProactiveEngagementRequest
* @return A Java Future containing the result of the DisableProactiveEngagement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisableProactiveEngagement
* @see AWS API Documentation
*/
@Override
public CompletableFuture disableProactiveEngagement(
DisableProactiveEngagementRequest disableProactiveEngagementRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disableProactiveEngagementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disableProactiveEngagementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisableProactiveEngagement");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisableProactiveEngagementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisableProactiveEngagement").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisableProactiveEngagementRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disableProactiveEngagementRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the Shield Response Team's (SRT) access to the specified Amazon S3 bucket containing the logs that you
* shared previously.
*
*
* @param disassociateDrtLogBucketRequest
* @return A Java Future containing the result of the DisassociateDRTLogBucket operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - NoAssociatedRoleException The ARN of the role that you specified does not exist.
* - AccessDeniedForDependencyException In order to grant the necessary access to the Shield Response Team
* (SRT) the user submitting the request must have the
iam:PassRole
permission. This error
* indicates the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an Amazon Web Services Service.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisassociateDRTLogBucket
* @see AWS API Documentation
*/
@Override
public CompletableFuture disassociateDRTLogBucket(
DisassociateDrtLogBucketRequest disassociateDrtLogBucketRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateDrtLogBucketRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateDrtLogBucketRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateDRTLogBucket");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateDrtLogBucketResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateDRTLogBucket").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisassociateDrtLogBucketRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disassociateDrtLogBucketRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the Shield Response Team's (SRT) access to your Amazon Web Services account.
*
*
* @param disassociateDrtRoleRequest
* @return A Java Future containing the result of the DisassociateDRTRole operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisassociateDRTRole
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture disassociateDRTRole(
DisassociateDrtRoleRequest disassociateDrtRoleRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateDrtRoleRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateDrtRoleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateDRTRole");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateDrtRoleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateDRTRole").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisassociateDrtRoleRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disassociateDrtRoleRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes health-based detection from the Shield Advanced protection for a resource. Shield Advanced health-based
* detection uses the health of your Amazon Web Services resource to improve responsiveness and accuracy in attack
* detection and response.
*
*
* You define the health check in Route 53 and then associate or disassociate it with your Shield Advanced
* protection. For more information, see Shield Advanced Health-Based Detection in the WAF Developer Guide.
*
*
* @param disassociateHealthCheckRequest
* @return A Java Future containing the result of the DisassociateHealthCheck operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.DisassociateHealthCheck
* @see AWS API Documentation
*/
@Override
public CompletableFuture disassociateHealthCheck(
DisassociateHealthCheckRequest disassociateHealthCheckRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateHealthCheckRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateHealthCheckRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateHealthCheck");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateHealthCheckResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateHealthCheck").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisassociateHealthCheckRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disassociateHealthCheckRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enable the Shield Advanced automatic application layer DDoS mitigation for the protected resource.
*
*
*
* This feature is available for Amazon CloudFront distributions and Application Load Balancers only.
*
*
*
* This causes Shield Advanced to create, verify, and apply WAF rules for DDoS attacks that it detects for the
* resource. Shield Advanced applies the rules in a Shield rule group inside the web ACL that you've associated with
* the resource. For information about how automatic mitigation works and the requirements for using it, see Shield
* Advanced automatic application layer DDoS mitigation.
*
*
*
* Don't use this action to make changes to automatic mitigation settings when it's already enabled for a resource.
* Instead, use UpdateApplicationLayerAutomaticResponse.
*
*
*
* To use this feature, you must associate a web ACL with the protected resource. The web ACL must be created using
* the latest version of WAF (v2). You can associate the web ACL through the Shield Advanced console at https://console.aws.amazon.com/wafv2/shieldv2#/. For
* more information, see Getting Started with
* Shield Advanced. You can also associate the web ACL to the resource through the WAF console or the WAF API,
* but you must manage Shield Advanced automatic mitigation through Shield Advanced. For information about WAF, see
* WAF Developer Guide.
*
*
* @param enableApplicationLayerAutomaticResponseRequest
* @return A Java Future containing the result of the EnableApplicationLayerAutomaticResponse operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitsExceededException Exception that indicates that the operation would exceed a limit.
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.EnableApplicationLayerAutomaticResponse
* @see AWS API Documentation
*/
@Override
public CompletableFuture enableApplicationLayerAutomaticResponse(
EnableApplicationLayerAutomaticResponseRequest enableApplicationLayerAutomaticResponseRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(enableApplicationLayerAutomaticResponseRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
enableApplicationLayerAutomaticResponseRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "EnableApplicationLayerAutomaticResponse");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, EnableApplicationLayerAutomaticResponseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("EnableApplicationLayerAutomaticResponse").withProtocolMetadata(protocolMetadata)
.withMarshaller(new EnableApplicationLayerAutomaticResponseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(enableApplicationLayerAutomaticResponseRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Authorizes the Shield Response Team (SRT) to use email and phone to notify contacts about escalations to the SRT
* and to initiate proactive customer support.
*
*
* @param enableProactiveEngagementRequest
* @return A Java Future containing the result of the EnableProactiveEngagement operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.EnableProactiveEngagement
* @see AWS API Documentation
*/
@Override
public CompletableFuture enableProactiveEngagement(
EnableProactiveEngagementRequest enableProactiveEngagementRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(enableProactiveEngagementRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, enableProactiveEngagementRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "EnableProactiveEngagement");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, EnableProactiveEngagementResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("EnableProactiveEngagement").withProtocolMetadata(protocolMetadata)
.withMarshaller(new EnableProactiveEngagementRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(enableProactiveEngagementRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the SubscriptionState
, either Active
or Inactive
.
*
*
* @param getSubscriptionStateRequest
* @return A Java Future containing the result of the GetSubscriptionState operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.GetSubscriptionState
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getSubscriptionState(
GetSubscriptionStateRequest getSubscriptionStateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getSubscriptionStateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSubscriptionStateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSubscriptionState");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetSubscriptionStateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSubscriptionState").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetSubscriptionStateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getSubscriptionStateRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns all ongoing DDoS attacks or all DDoS attacks during a specified time period.
*
*
* @param listAttacksRequest
* @return A Java Future containing the result of the ListAttacks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListAttacks
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listAttacks(ListAttacksRequest listAttacksRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAttacksRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAttacksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAttacks");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListAttacksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAttacks").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAttacksRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAttacksRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves ProtectionGroup objects for the account. You can retrieve all protection groups or you can
* provide filtering criteria and retrieve just the subset of protection groups that match the criteria.
*
*
* @param listProtectionGroupsRequest
* @return A Java Future containing the result of the ListProtectionGroups operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtectionGroups
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listProtectionGroups(
ListProtectionGroupsRequest listProtectionGroupsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listProtectionGroupsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listProtectionGroupsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListProtectionGroups");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListProtectionGroupsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListProtectionGroups").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListProtectionGroupsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listProtectionGroupsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves Protection objects for the account. You can retrieve all protections or you can provide
* filtering criteria and retrieve just the subset of protections that match the criteria.
*
*
* @param listProtectionsRequest
* @return A Java Future containing the result of the ListProtections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListProtections
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listProtections(ListProtectionsRequest listProtectionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listProtectionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listProtectionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListProtections");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListProtectionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListProtections").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListProtectionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listProtectionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the resources that are included in the protection group.
*
*
* @param listResourcesInProtectionGroupRequest
* @return A Java Future containing the result of the ListResourcesInProtectionGroup operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidPaginationTokenException Exception that indicates that the
NextToken
specified in
* the request is invalid. Submit the request using the NextToken
value that was returned in
* the prior response.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListResourcesInProtectionGroup
* @see AWS API Documentation
*/
@Override
public CompletableFuture listResourcesInProtectionGroup(
ListResourcesInProtectionGroupRequest listResourcesInProtectionGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listResourcesInProtectionGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listResourcesInProtectionGroupRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListResourcesInProtectionGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListResourcesInProtectionGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListResourcesInProtectionGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListResourcesInProtectionGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listResourcesInProtectionGroupRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about Amazon Web Services tags for a specified Amazon Resource Name (ARN) in Shield.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds or updates tags for a resource in Shield.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(tagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes tags from a resource in Shield.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidResourceException Exception that indicates that the resource is invalid. You might not have
* access to the resource, or the resource might not exist.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(untagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(untagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates an existing Shield Advanced automatic application layer DDoS mitigation configuration for the specified
* resource.
*
*
* @param updateApplicationLayerAutomaticResponseRequest
* @return A Java Future containing the result of the UpdateApplicationLayerAutomaticResponse operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidOperationException Exception that indicates that the operation would not cause any change to
* occur.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateApplicationLayerAutomaticResponse
* @see AWS API Documentation
*/
@Override
public CompletableFuture updateApplicationLayerAutomaticResponse(
UpdateApplicationLayerAutomaticResponseRequest updateApplicationLayerAutomaticResponseRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateApplicationLayerAutomaticResponseRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
updateApplicationLayerAutomaticResponseRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateApplicationLayerAutomaticResponse");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, UpdateApplicationLayerAutomaticResponseResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateApplicationLayerAutomaticResponse").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateApplicationLayerAutomaticResponseRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateApplicationLayerAutomaticResponseRequest));
CompletableFuture whenCompleted = executeFuture
.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates the details of the list of email addresses and phone numbers that the Shield Response Team (SRT) can use
* to contact you if you have proactive engagement enabled, for escalations to the SRT and to initiate proactive
* customer support.
*
*
* @param updateEmergencyContactSettingsRequest
* @return A Java Future containing the result of the UpdateEmergencyContactSettings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateEmergencyContactSettings
* @see AWS API Documentation
*/
@Override
public CompletableFuture updateEmergencyContactSettings(
UpdateEmergencyContactSettingsRequest updateEmergencyContactSettingsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateEmergencyContactSettingsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
updateEmergencyContactSettingsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateEmergencyContactSettings");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateEmergencyContactSettingsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateEmergencyContactSettings").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateEmergencyContactSettingsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateEmergencyContactSettingsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates an existing protection group. A protection group is a grouping of protected resources so they can be
* handled as a collective. This resource grouping improves the accuracy of detection and reduces false positives.
*
*
* @param updateProtectionGroupRequest
* @return A Java Future containing the result of the UpdateProtectionGroup operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateProtectionGroup
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture updateProtectionGroup(
UpdateProtectionGroupRequest updateProtectionGroupRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateProtectionGroupRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateProtectionGroupRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateProtectionGroup");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateProtectionGroupResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateProtectionGroup").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateProtectionGroupRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateProtectionGroupRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates the details of an existing subscription. Only enter values for parameters you want to change. Empty
* parameters are not updated.
*
*
*
* For accounts that are members of an Organizations organization, Shield Advanced subscriptions are billed against
* the organization's payer account, regardless of whether the payer account itself is subscribed.
*
*
*
* @param updateSubscriptionRequest
* @return A Java Future containing the result of the UpdateSubscription operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalErrorException Exception that indicates that a problem occurred with the service
* infrastructure. You can retry the request.
* - LockedSubscriptionException You are trying to update a subscription that has not yet completed the
* 1-year commitment. You can change the
AutoRenew
parameter during the last 30 days of your
* subscription. This exception indicates that you are attempting to change AutoRenew
prior to
* that period.
* - ResourceNotFoundException Exception indicating the specified resource does not exist. If available,
* this exception includes details in additional properties.
* - InvalidParameterException Exception that indicates that the parameters passed to the API are invalid.
* If available, this exception includes details in additional properties.
* - OptimisticLockException Exception that indicates that the resource state has been modified by another
* client. Retrieve the resource and then retry your request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - ShieldException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample ShieldAsyncClient.UpdateSubscription
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture updateSubscription(UpdateSubscriptionRequest updateSubscriptionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateSubscriptionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateSubscriptionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Shield");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateSubscription");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateSubscriptionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateSubscription").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateSubscriptionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateSubscriptionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public final ShieldServiceClientConfiguration serviceClientConfiguration() {
return new ShieldServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(ShieldException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("OptimisticLockException")
.exceptionBuilderSupplier(OptimisticLockException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidParameterException")
.exceptionBuilderSupplier(InvalidParameterException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidOperationException")
.exceptionBuilderSupplier(InvalidOperationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalErrorException")
.exceptionBuilderSupplier(InternalErrorException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NoAssociatedRoleException")
.exceptionBuilderSupplier(NoAssociatedRoleException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedForDependencyException")
.exceptionBuilderSupplier(AccessDeniedForDependencyException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LockedSubscriptionException")
.exceptionBuilderSupplier(LockedSubscriptionException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidResourceException")
.exceptionBuilderSupplier(InvalidResourceException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceAlreadyExistsException")
.exceptionBuilderSupplier(ResourceAlreadyExistsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidPaginationTokenException")
.exceptionBuilderSupplier(InvalidPaginationTokenException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LimitsExceededException")
.exceptionBuilderSupplier(LimitsExceededException::builder).httpStatusCode(400).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
ShieldServiceClientConfigurationBuilder serviceConfigBuilder = new ShieldServiceClientConfigurationBuilder(configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private HttpResponseHandler