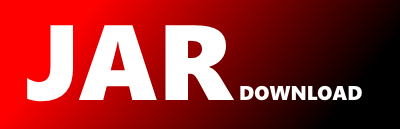
software.amazon.awssdk.services.shield.ShieldClient Maven / Gradle / Ivy
Show all versions of shield Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.shield;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.shield.model.AccessDeniedException;
import software.amazon.awssdk.services.shield.model.AccessDeniedForDependencyException;
import software.amazon.awssdk.services.shield.model.AssociateDrtLogBucketRequest;
import software.amazon.awssdk.services.shield.model.AssociateDrtLogBucketResponse;
import software.amazon.awssdk.services.shield.model.AssociateDrtRoleRequest;
import software.amazon.awssdk.services.shield.model.AssociateDrtRoleResponse;
import software.amazon.awssdk.services.shield.model.CreateProtectionRequest;
import software.amazon.awssdk.services.shield.model.CreateProtectionResponse;
import software.amazon.awssdk.services.shield.model.CreateSubscriptionRequest;
import software.amazon.awssdk.services.shield.model.CreateSubscriptionResponse;
import software.amazon.awssdk.services.shield.model.DeleteProtectionRequest;
import software.amazon.awssdk.services.shield.model.DeleteProtectionResponse;
import software.amazon.awssdk.services.shield.model.DescribeAttackRequest;
import software.amazon.awssdk.services.shield.model.DescribeAttackResponse;
import software.amazon.awssdk.services.shield.model.DescribeDrtAccessRequest;
import software.amazon.awssdk.services.shield.model.DescribeDrtAccessResponse;
import software.amazon.awssdk.services.shield.model.DescribeEmergencyContactSettingsRequest;
import software.amazon.awssdk.services.shield.model.DescribeEmergencyContactSettingsResponse;
import software.amazon.awssdk.services.shield.model.DescribeProtectionRequest;
import software.amazon.awssdk.services.shield.model.DescribeProtectionResponse;
import software.amazon.awssdk.services.shield.model.DescribeSubscriptionRequest;
import software.amazon.awssdk.services.shield.model.DescribeSubscriptionResponse;
import software.amazon.awssdk.services.shield.model.DisassociateDrtLogBucketRequest;
import software.amazon.awssdk.services.shield.model.DisassociateDrtLogBucketResponse;
import software.amazon.awssdk.services.shield.model.DisassociateDrtRoleRequest;
import software.amazon.awssdk.services.shield.model.DisassociateDrtRoleResponse;
import software.amazon.awssdk.services.shield.model.GetSubscriptionStateRequest;
import software.amazon.awssdk.services.shield.model.GetSubscriptionStateResponse;
import software.amazon.awssdk.services.shield.model.InternalErrorException;
import software.amazon.awssdk.services.shield.model.InvalidOperationException;
import software.amazon.awssdk.services.shield.model.InvalidPaginationTokenException;
import software.amazon.awssdk.services.shield.model.InvalidParameterException;
import software.amazon.awssdk.services.shield.model.InvalidResourceException;
import software.amazon.awssdk.services.shield.model.LimitsExceededException;
import software.amazon.awssdk.services.shield.model.ListAttacksRequest;
import software.amazon.awssdk.services.shield.model.ListAttacksResponse;
import software.amazon.awssdk.services.shield.model.ListProtectionsRequest;
import software.amazon.awssdk.services.shield.model.ListProtectionsResponse;
import software.amazon.awssdk.services.shield.model.LockedSubscriptionException;
import software.amazon.awssdk.services.shield.model.NoAssociatedRoleException;
import software.amazon.awssdk.services.shield.model.OptimisticLockException;
import software.amazon.awssdk.services.shield.model.ResourceAlreadyExistsException;
import software.amazon.awssdk.services.shield.model.ResourceNotFoundException;
import software.amazon.awssdk.services.shield.model.ShieldException;
import software.amazon.awssdk.services.shield.model.UpdateEmergencyContactSettingsRequest;
import software.amazon.awssdk.services.shield.model.UpdateEmergencyContactSettingsResponse;
import software.amazon.awssdk.services.shield.model.UpdateSubscriptionRequest;
import software.amazon.awssdk.services.shield.model.UpdateSubscriptionResponse;
/**
* Service client for accessing AWS Shield. This can be created using the static {@link #builder()} method.
*
* AWS Shield Advanced
*
* This is the AWS Shield Advanced API Reference. This guide is for developers who need detailed information
* about the AWS Shield Advanced API actions, data types, and errors. For detailed information about AWS WAF and AWS
* Shield Advanced features and an overview of how to use the AWS WAF and AWS Shield Advanced APIs, see the AWS WAF and AWS Shield Developer Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface ShieldClient extends SdkClient {
String SERVICE_NAME = "shield";
/**
* Create a {@link ShieldClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static ShieldClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link ShieldClient}.
*/
static ShieldClientBuilder builder() {
return new DefaultShieldClientBuilder();
}
/**
*
* Authorizes the DDoS Response team (DRT) to access the specified Amazon S3 bucket containing your AWS WAF logs.
* You can associate up to 10 Amazon S3 buckets with your subscription.
*
*
* To use the services of the DRT and make an AssociateDRTLogBucket
request, you must be subscribed to
* the Business Support plan or the Enterprise Support plan.
*
*
* @param associateDrtLogBucketRequest
* @return Result of the AssociateDRTLogBucket operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws NoAssociatedRoleException
* The ARN of the role that you specifed does not exist.
* @throws LimitsExceededException
* Exception that indicates that the operation would exceed a limit.
*
* Type
is the type of limit that would be exceeded.
*
*
* Limit
is the threshold that would be exceeded.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws AccessDeniedForDependencyException
* In order to grant the necessary access to the DDoS Response Team, the user submitting
* AssociateDRTRole
must have the iam:PassRole
permission. This error indicates
* the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an AWS Service.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.AssociateDRTLogBucket
* @see AWS
* API Documentation
*/
default AssociateDrtLogBucketResponse associateDRTLogBucket(AssociateDrtLogBucketRequest associateDrtLogBucketRequest)
throws InternalErrorException, InvalidOperationException, NoAssociatedRoleException, LimitsExceededException,
InvalidParameterException, AccessDeniedForDependencyException, OptimisticLockException, ResourceNotFoundException,
AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Authorizes the DDoS Response team (DRT) to access the specified Amazon S3 bucket containing your AWS WAF logs.
* You can associate up to 10 Amazon S3 buckets with your subscription.
*
*
* To use the services of the DRT and make an AssociateDRTLogBucket
request, you must be subscribed to
* the Business Support plan or the Enterprise Support plan.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateDrtLogBucketRequest.Builder} avoiding the
* need to create one manually via {@link AssociateDrtLogBucketRequest#builder()}
*
*
* @param associateDrtLogBucketRequest
* A {@link Consumer} that will call methods on {@link AssociateDRTLogBucketRequest.Builder} to create a
* request.
* @return Result of the AssociateDRTLogBucket operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws NoAssociatedRoleException
* The ARN of the role that you specifed does not exist.
* @throws LimitsExceededException
* Exception that indicates that the operation would exceed a limit.
*
* Type
is the type of limit that would be exceeded.
*
*
* Limit
is the threshold that would be exceeded.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws AccessDeniedForDependencyException
* In order to grant the necessary access to the DDoS Response Team, the user submitting
* AssociateDRTRole
must have the iam:PassRole
permission. This error indicates
* the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an AWS Service.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.AssociateDRTLogBucket
* @see AWS
* API Documentation
*/
default AssociateDrtLogBucketResponse associateDRTLogBucket(
Consumer associateDrtLogBucketRequest) throws InternalErrorException,
InvalidOperationException, NoAssociatedRoleException, LimitsExceededException, InvalidParameterException,
AccessDeniedForDependencyException, OptimisticLockException, ResourceNotFoundException, AwsServiceException,
SdkClientException, ShieldException {
return associateDRTLogBucket(AssociateDrtLogBucketRequest.builder().applyMutation(associateDrtLogBucketRequest).build());
}
/**
*
* Authorizes the DDoS Response team (DRT), using the specified role, to access your AWS account to assist with DDoS
* attack mitigation during potential attacks. This enables the DRT to inspect your AWS WAF configuration and create
* or update AWS WAF rules and web ACLs.
*
*
* You can associate only one RoleArn
with your subscription. If you submit an
* AssociateDRTRole
request for an account that already has an associated role, the new
* RoleArn
will replace the existing RoleArn
.
*
*
* Prior to making the AssociateDRTRole
request, you must attach the AWSShieldDRTAccessPolicy managed policy to the role you will specify in the request. For more information
* see Attaching and
* Detaching IAM Policies. The role must also trust the service principal drt.shield.amazonaws.com
* . For more information, see IAM JSON
* Policy Elements: Principal.
*
*
* The DRT will have access only to your AWS WAF and Shield resources. By submitting this request, you authorize the
* DRT to inspect your AWS WAF and Shield configuration and create and update AWS WAF rules and web ACLs on your
* behalf. The DRT takes these actions only if explicitly authorized by you.
*
*
* You must have the iam:PassRole
permission to make an AssociateDRTRole
request. For more
* information, see Granting a
* User Permissions to Pass a Role to an AWS Service.
*
*
* To use the services of the DRT and make an AssociateDRTRole
request, you must be subscribed to the
* Business Support plan or the Enterprise Support plan.
*
*
* @param associateDrtRoleRequest
* @return Result of the AssociateDRTRole operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws AccessDeniedForDependencyException
* In order to grant the necessary access to the DDoS Response Team, the user submitting
* AssociateDRTRole
must have the iam:PassRole
permission. This error indicates
* the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an AWS Service.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.AssociateDRTRole
* @see AWS API
* Documentation
*/
default AssociateDrtRoleResponse associateDRTRole(AssociateDrtRoleRequest associateDrtRoleRequest)
throws InternalErrorException, InvalidOperationException, InvalidParameterException,
AccessDeniedForDependencyException, OptimisticLockException, ResourceNotFoundException, AwsServiceException,
SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Authorizes the DDoS Response team (DRT), using the specified role, to access your AWS account to assist with DDoS
* attack mitigation during potential attacks. This enables the DRT to inspect your AWS WAF configuration and create
* or update AWS WAF rules and web ACLs.
*
*
* You can associate only one RoleArn
with your subscription. If you submit an
* AssociateDRTRole
request for an account that already has an associated role, the new
* RoleArn
will replace the existing RoleArn
.
*
*
* Prior to making the AssociateDRTRole
request, you must attach the AWSShieldDRTAccessPolicy managed policy to the role you will specify in the request. For more information
* see Attaching and
* Detaching IAM Policies. The role must also trust the service principal drt.shield.amazonaws.com
* . For more information, see IAM JSON
* Policy Elements: Principal.
*
*
* The DRT will have access only to your AWS WAF and Shield resources. By submitting this request, you authorize the
* DRT to inspect your AWS WAF and Shield configuration and create and update AWS WAF rules and web ACLs on your
* behalf. The DRT takes these actions only if explicitly authorized by you.
*
*
* You must have the iam:PassRole
permission to make an AssociateDRTRole
request. For more
* information, see Granting a
* User Permissions to Pass a Role to an AWS Service.
*
*
* To use the services of the DRT and make an AssociateDRTRole
request, you must be subscribed to the
* Business Support plan or the Enterprise Support plan.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateDrtRoleRequest.Builder} avoiding the need
* to create one manually via {@link AssociateDrtRoleRequest#builder()}
*
*
* @param associateDrtRoleRequest
* A {@link Consumer} that will call methods on {@link AssociateDRTRoleRequest.Builder} to create a request.
* @return Result of the AssociateDRTRole operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws AccessDeniedForDependencyException
* In order to grant the necessary access to the DDoS Response Team, the user submitting
* AssociateDRTRole
must have the iam:PassRole
permission. This error indicates
* the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an AWS Service.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.AssociateDRTRole
* @see AWS API
* Documentation
*/
default AssociateDrtRoleResponse associateDRTRole(Consumer associateDrtRoleRequest)
throws InternalErrorException, InvalidOperationException, InvalidParameterException,
AccessDeniedForDependencyException, OptimisticLockException, ResourceNotFoundException, AwsServiceException,
SdkClientException, ShieldException {
return associateDRTRole(AssociateDrtRoleRequest.builder().applyMutation(associateDrtRoleRequest).build());
}
/**
*
* Enables AWS Shield Advanced for a specific AWS resource. The resource can be an Amazon CloudFront distribution,
* Elastic Load Balancing load balancer, AWS Global Accelerator accelerator, Elastic IP Address, or an Amazon Route
* 53 hosted zone.
*
*
* You can add protection to only a single resource with each CreateProtection request. If you want to add
* protection to multiple resources at once, use the AWS WAF
* console. For more information see Getting Started with AWS
* Shield Advanced and Add AWS Shield
* Advanced Protection to more AWS Resources.
*
*
* @param createProtectionRequest
* @return Result of the CreateProtection operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidResourceException
* Exception that indicates that the resource is invalid. You might not have access to the resource, or the
* resource might not exist.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws LimitsExceededException
* Exception that indicates that the operation would exceed a limit.
*
* Type
is the type of limit that would be exceeded.
*
*
* Limit
is the threshold that would be exceeded.
* @throws ResourceAlreadyExistsException
* Exception indicating the specified resource already exists.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.CreateProtection
* @see AWS API
* Documentation
*/
default CreateProtectionResponse createProtection(CreateProtectionRequest createProtectionRequest)
throws InternalErrorException, InvalidResourceException, InvalidOperationException, LimitsExceededException,
ResourceAlreadyExistsException, OptimisticLockException, ResourceNotFoundException, AwsServiceException,
SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Enables AWS Shield Advanced for a specific AWS resource. The resource can be an Amazon CloudFront distribution,
* Elastic Load Balancing load balancer, AWS Global Accelerator accelerator, Elastic IP Address, or an Amazon Route
* 53 hosted zone.
*
*
* You can add protection to only a single resource with each CreateProtection request. If you want to add
* protection to multiple resources at once, use the AWS WAF
* console. For more information see Getting Started with AWS
* Shield Advanced and Add AWS Shield
* Advanced Protection to more AWS Resources.
*
*
*
* This is a convenience which creates an instance of the {@link CreateProtectionRequest.Builder} avoiding the need
* to create one manually via {@link CreateProtectionRequest#builder()}
*
*
* @param createProtectionRequest
* A {@link Consumer} that will call methods on {@link CreateProtectionRequest.Builder} to create a request.
* @return Result of the CreateProtection operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidResourceException
* Exception that indicates that the resource is invalid. You might not have access to the resource, or the
* resource might not exist.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws LimitsExceededException
* Exception that indicates that the operation would exceed a limit.
*
* Type
is the type of limit that would be exceeded.
*
*
* Limit
is the threshold that would be exceeded.
* @throws ResourceAlreadyExistsException
* Exception indicating the specified resource already exists.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.CreateProtection
* @see AWS API
* Documentation
*/
default CreateProtectionResponse createProtection(Consumer createProtectionRequest)
throws InternalErrorException, InvalidResourceException, InvalidOperationException, LimitsExceededException,
ResourceAlreadyExistsException, OptimisticLockException, ResourceNotFoundException, AwsServiceException,
SdkClientException, ShieldException {
return createProtection(CreateProtectionRequest.builder().applyMutation(createProtectionRequest).build());
}
/**
*
* Activates AWS Shield Advanced for an account.
*
*
* As part of this request you can specify EmergencySettings
that automaticaly grant the DDoS response
* team (DRT) needed permissions to assist you during a suspected DDoS attack. For more information see Authorize the DDoS Response Team
* to Create Rules and Web ACLs on Your Behalf.
*
*
* To use the services of the DRT, you must be subscribed to the Business Support plan or the Enterprise Support plan.
*
*
* When you initally create a subscription, your subscription is set to be automatically renewed at the end of the
* existing subscription period. You can change this by submitting an UpdateSubscription
request.
*
*
* @return Result of the CreateSubscription operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceAlreadyExistsException
* Exception indicating the specified resource already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.CreateSubscription
* @see #createSubscription(CreateSubscriptionRequest)
* @see AWS API
* Documentation
*/
default CreateSubscriptionResponse createSubscription() throws InternalErrorException, ResourceAlreadyExistsException,
AwsServiceException, SdkClientException, ShieldException {
return createSubscription(CreateSubscriptionRequest.builder().build());
}
/**
*
* Activates AWS Shield Advanced for an account.
*
*
* As part of this request you can specify EmergencySettings
that automaticaly grant the DDoS response
* team (DRT) needed permissions to assist you during a suspected DDoS attack. For more information see Authorize the DDoS Response Team
* to Create Rules and Web ACLs on Your Behalf.
*
*
* To use the services of the DRT, you must be subscribed to the Business Support plan or the Enterprise Support plan.
*
*
* When you initally create a subscription, your subscription is set to be automatically renewed at the end of the
* existing subscription period. You can change this by submitting an UpdateSubscription
request.
*
*
* @param createSubscriptionRequest
* @return Result of the CreateSubscription operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceAlreadyExistsException
* Exception indicating the specified resource already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.CreateSubscription
* @see AWS API
* Documentation
*/
default CreateSubscriptionResponse createSubscription(CreateSubscriptionRequest createSubscriptionRequest)
throws InternalErrorException, ResourceAlreadyExistsException, AwsServiceException, SdkClientException,
ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Activates AWS Shield Advanced for an account.
*
*
* As part of this request you can specify EmergencySettings
that automaticaly grant the DDoS response
* team (DRT) needed permissions to assist you during a suspected DDoS attack. For more information see Authorize the DDoS Response Team
* to Create Rules and Web ACLs on Your Behalf.
*
*
* To use the services of the DRT, you must be subscribed to the Business Support plan or the Enterprise Support plan.
*
*
* When you initally create a subscription, your subscription is set to be automatically renewed at the end of the
* existing subscription period. You can change this by submitting an UpdateSubscription
request.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSubscriptionRequest.Builder} avoiding the
* need to create one manually via {@link CreateSubscriptionRequest#builder()}
*
*
* @param createSubscriptionRequest
* A {@link Consumer} that will call methods on {@link CreateSubscriptionRequest.Builder} to create a
* request.
* @return Result of the CreateSubscription operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceAlreadyExistsException
* Exception indicating the specified resource already exists.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.CreateSubscription
* @see AWS API
* Documentation
*/
default CreateSubscriptionResponse createSubscription(Consumer createSubscriptionRequest)
throws InternalErrorException, ResourceAlreadyExistsException, AwsServiceException, SdkClientException,
ShieldException {
return createSubscription(CreateSubscriptionRequest.builder().applyMutation(createSubscriptionRequest).build());
}
/**
*
* Deletes an AWS Shield Advanced Protection.
*
*
* @param deleteProtectionRequest
* @return Result of the DeleteProtection operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DeleteProtection
* @see AWS API
* Documentation
*/
default DeleteProtectionResponse deleteProtection(DeleteProtectionRequest deleteProtectionRequest)
throws InternalErrorException, ResourceNotFoundException, OptimisticLockException, AwsServiceException,
SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an AWS Shield Advanced Protection.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteProtectionRequest.Builder} avoiding the need
* to create one manually via {@link DeleteProtectionRequest#builder()}
*
*
* @param deleteProtectionRequest
* A {@link Consumer} that will call methods on {@link DeleteProtectionRequest.Builder} to create a request.
* @return Result of the DeleteProtection operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DeleteProtection
* @see AWS API
* Documentation
*/
default DeleteProtectionResponse deleteProtection(Consumer deleteProtectionRequest)
throws InternalErrorException, ResourceNotFoundException, OptimisticLockException, AwsServiceException,
SdkClientException, ShieldException {
return deleteProtection(DeleteProtectionRequest.builder().applyMutation(deleteProtectionRequest).build());
}
/**
*
* Describes the details of a DDoS attack.
*
*
* @param describeAttackRequest
* @return Result of the DescribeAttack operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws AccessDeniedException
* Exception that indicates the specified AttackId
does not exist, or the requester does not
* have the appropriate permissions to access the AttackId
.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeAttack
* @see AWS API
* Documentation
*/
default DescribeAttackResponse describeAttack(DescribeAttackRequest describeAttackRequest) throws InternalErrorException,
AccessDeniedException, AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the details of a DDoS attack.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAttackRequest.Builder} avoiding the need to
* create one manually via {@link DescribeAttackRequest#builder()}
*
*
* @param describeAttackRequest
* A {@link Consumer} that will call methods on {@link DescribeAttackRequest.Builder} to create a request.
* @return Result of the DescribeAttack operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws AccessDeniedException
* Exception that indicates the specified AttackId
does not exist, or the requester does not
* have the appropriate permissions to access the AttackId
.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeAttack
* @see AWS API
* Documentation
*/
default DescribeAttackResponse describeAttack(Consumer describeAttackRequest)
throws InternalErrorException, AccessDeniedException, AwsServiceException, SdkClientException, ShieldException {
return describeAttack(DescribeAttackRequest.builder().applyMutation(describeAttackRequest).build());
}
/**
*
* Returns the current role and list of Amazon S3 log buckets used by the DDoS Response team (DRT) to access your
* AWS account while assisting with attack mitigation.
*
*
* @param describeDrtAccessRequest
* @return Result of the DescribeDRTAccess operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeDRTAccess
* @see AWS API
* Documentation
*/
default DescribeDrtAccessResponse describeDRTAccess(DescribeDrtAccessRequest describeDrtAccessRequest)
throws InternalErrorException, ResourceNotFoundException, AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the current role and list of Amazon S3 log buckets used by the DDoS Response team (DRT) to access your
* AWS account while assisting with attack mitigation.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDrtAccessRequest.Builder} avoiding the need
* to create one manually via {@link DescribeDrtAccessRequest#builder()}
*
*
* @param describeDrtAccessRequest
* A {@link Consumer} that will call methods on {@link DescribeDRTAccessRequest.Builder} to create a request.
* @return Result of the DescribeDRTAccess operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeDRTAccess
* @see AWS API
* Documentation
*/
default DescribeDrtAccessResponse describeDRTAccess(Consumer describeDrtAccessRequest)
throws InternalErrorException, ResourceNotFoundException, AwsServiceException, SdkClientException, ShieldException {
return describeDRTAccess(DescribeDrtAccessRequest.builder().applyMutation(describeDrtAccessRequest).build());
}
/**
*
* Lists the email addresses that the DRT can use to contact you during a suspected attack.
*
*
* @param describeEmergencyContactSettingsRequest
* @return Result of the DescribeEmergencyContactSettings operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeEmergencyContactSettings
* @see AWS API Documentation
*/
default DescribeEmergencyContactSettingsResponse describeEmergencyContactSettings(
DescribeEmergencyContactSettingsRequest describeEmergencyContactSettingsRequest) throws InternalErrorException,
ResourceNotFoundException, AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the email addresses that the DRT can use to contact you during a suspected attack.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEmergencyContactSettingsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeEmergencyContactSettingsRequest#builder()}
*
*
* @param describeEmergencyContactSettingsRequest
* A {@link Consumer} that will call methods on {@link DescribeEmergencyContactSettingsRequest.Builder} to
* create a request.
* @return Result of the DescribeEmergencyContactSettings operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeEmergencyContactSettings
* @see AWS API Documentation
*/
default DescribeEmergencyContactSettingsResponse describeEmergencyContactSettings(
Consumer describeEmergencyContactSettingsRequest)
throws InternalErrorException, ResourceNotFoundException, AwsServiceException, SdkClientException, ShieldException {
return describeEmergencyContactSettings(DescribeEmergencyContactSettingsRequest.builder()
.applyMutation(describeEmergencyContactSettingsRequest).build());
}
/**
*
* Lists the details of a Protection object.
*
*
* @param describeProtectionRequest
* @return Result of the DescribeProtection operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeProtection
* @see AWS API
* Documentation
*/
default DescribeProtectionResponse describeProtection(DescribeProtectionRequest describeProtectionRequest)
throws InternalErrorException, InvalidParameterException, ResourceNotFoundException, AwsServiceException,
SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the details of a Protection object.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeProtectionRequest.Builder} avoiding the
* need to create one manually via {@link DescribeProtectionRequest#builder()}
*
*
* @param describeProtectionRequest
* A {@link Consumer} that will call methods on {@link DescribeProtectionRequest.Builder} to create a
* request.
* @return Result of the DescribeProtection operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeProtection
* @see AWS API
* Documentation
*/
default DescribeProtectionResponse describeProtection(Consumer describeProtectionRequest)
throws InternalErrorException, InvalidParameterException, ResourceNotFoundException, AwsServiceException,
SdkClientException, ShieldException {
return describeProtection(DescribeProtectionRequest.builder().applyMutation(describeProtectionRequest).build());
}
/**
*
* Provides details about the AWS Shield Advanced subscription for an account.
*
*
* @return Result of the DescribeSubscription operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeSubscription
* @see #describeSubscription(DescribeSubscriptionRequest)
* @see AWS
* API Documentation
*/
default DescribeSubscriptionResponse describeSubscription() throws InternalErrorException, ResourceNotFoundException,
AwsServiceException, SdkClientException, ShieldException {
return describeSubscription(DescribeSubscriptionRequest.builder().build());
}
/**
*
* Provides details about the AWS Shield Advanced subscription for an account.
*
*
* @param describeSubscriptionRequest
* @return Result of the DescribeSubscription operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeSubscription
* @see AWS
* API Documentation
*/
default DescribeSubscriptionResponse describeSubscription(DescribeSubscriptionRequest describeSubscriptionRequest)
throws InternalErrorException, ResourceNotFoundException, AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Provides details about the AWS Shield Advanced subscription for an account.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeSubscriptionRequest.Builder} avoiding the
* need to create one manually via {@link DescribeSubscriptionRequest#builder()}
*
*
* @param describeSubscriptionRequest
* A {@link Consumer} that will call methods on {@link DescribeSubscriptionRequest.Builder} to create a
* request.
* @return Result of the DescribeSubscription operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DescribeSubscription
* @see AWS
* API Documentation
*/
default DescribeSubscriptionResponse describeSubscription(
Consumer describeSubscriptionRequest) throws InternalErrorException,
ResourceNotFoundException, AwsServiceException, SdkClientException, ShieldException {
return describeSubscription(DescribeSubscriptionRequest.builder().applyMutation(describeSubscriptionRequest).build());
}
/**
*
* Removes the DDoS Response team's (DRT) access to the specified Amazon S3 bucket containing your AWS WAF logs.
*
*
* To make a DisassociateDRTLogBucket
request, you must be subscribed to the Business Support plan or the Enterprise Support plan. However, if you are
* not subscribed to one of these support plans, but had been previously and had granted the DRT access to your
* account, you can submit a DisassociateDRTLogBucket
request to remove this access.
*
*
* @param disassociateDrtLogBucketRequest
* @return Result of the DisassociateDRTLogBucket operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws NoAssociatedRoleException
* The ARN of the role that you specifed does not exist.
* @throws AccessDeniedForDependencyException
* In order to grant the necessary access to the DDoS Response Team, the user submitting
* AssociateDRTRole
must have the iam:PassRole
permission. This error indicates
* the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an AWS Service.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DisassociateDRTLogBucket
* @see AWS API Documentation
*/
default DisassociateDrtLogBucketResponse disassociateDRTLogBucket(
DisassociateDrtLogBucketRequest disassociateDrtLogBucketRequest) throws InternalErrorException,
InvalidOperationException, NoAssociatedRoleException, AccessDeniedForDependencyException, OptimisticLockException,
ResourceNotFoundException, AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the DDoS Response team's (DRT) access to the specified Amazon S3 bucket containing your AWS WAF logs.
*
*
* To make a DisassociateDRTLogBucket
request, you must be subscribed to the Business Support plan or the Enterprise Support plan. However, if you are
* not subscribed to one of these support plans, but had been previously and had granted the DRT access to your
* account, you can submit a DisassociateDRTLogBucket
request to remove this access.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateDrtLogBucketRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateDrtLogBucketRequest#builder()}
*
*
* @param disassociateDrtLogBucketRequest
* A {@link Consumer} that will call methods on {@link DisassociateDRTLogBucketRequest.Builder} to create a
* request.
* @return Result of the DisassociateDRTLogBucket operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws NoAssociatedRoleException
* The ARN of the role that you specifed does not exist.
* @throws AccessDeniedForDependencyException
* In order to grant the necessary access to the DDoS Response Team, the user submitting
* AssociateDRTRole
must have the iam:PassRole
permission. This error indicates
* the user did not have the appropriate permissions. For more information, see Granting a User
* Permissions to Pass a Role to an AWS Service.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DisassociateDRTLogBucket
* @see AWS API Documentation
*/
default DisassociateDrtLogBucketResponse disassociateDRTLogBucket(
Consumer disassociateDrtLogBucketRequest) throws InternalErrorException,
InvalidOperationException, NoAssociatedRoleException, AccessDeniedForDependencyException, OptimisticLockException,
ResourceNotFoundException, AwsServiceException, SdkClientException, ShieldException {
return disassociateDRTLogBucket(DisassociateDrtLogBucketRequest.builder().applyMutation(disassociateDrtLogBucketRequest)
.build());
}
/**
*
* Removes the DDoS Response team's (DRT) access to your AWS account.
*
*
* To make a DisassociateDRTRole
request, you must be subscribed to the Business Support plan or the Enterprise Support plan. However, if you are
* not subscribed to one of these support plans, but had been previously and had granted the DRT access to your
* account, you can submit a DisassociateDRTRole
request to remove this access.
*
*
* @return Result of the DisassociateDRTRole operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DisassociateDRTRole
* @see #disassociateDRTRole(DisassociateDrtRoleRequest)
* @see AWS API
* Documentation
*/
default DisassociateDrtRoleResponse disassociateDRTRole() throws InternalErrorException, InvalidOperationException,
OptimisticLockException, ResourceNotFoundException, AwsServiceException, SdkClientException, ShieldException {
return disassociateDRTRole(DisassociateDrtRoleRequest.builder().build());
}
/**
*
* Removes the DDoS Response team's (DRT) access to your AWS account.
*
*
* To make a DisassociateDRTRole
request, you must be subscribed to the Business Support plan or the Enterprise Support plan. However, if you are
* not subscribed to one of these support plans, but had been previously and had granted the DRT access to your
* account, you can submit a DisassociateDRTRole
request to remove this access.
*
*
* @param disassociateDrtRoleRequest
* @return Result of the DisassociateDRTRole operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DisassociateDRTRole
* @see AWS API
* Documentation
*/
default DisassociateDrtRoleResponse disassociateDRTRole(DisassociateDrtRoleRequest disassociateDrtRoleRequest)
throws InternalErrorException, InvalidOperationException, OptimisticLockException, ResourceNotFoundException,
AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the DDoS Response team's (DRT) access to your AWS account.
*
*
* To make a DisassociateDRTRole
request, you must be subscribed to the Business Support plan or the Enterprise Support plan. However, if you are
* not subscribed to one of these support plans, but had been previously and had granted the DRT access to your
* account, you can submit a DisassociateDRTRole
request to remove this access.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateDrtRoleRequest.Builder} avoiding the
* need to create one manually via {@link DisassociateDrtRoleRequest#builder()}
*
*
* @param disassociateDrtRoleRequest
* A {@link Consumer} that will call methods on {@link DisassociateDRTRoleRequest.Builder} to create a
* request.
* @return Result of the DisassociateDRTRole operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.DisassociateDRTRole
* @see AWS API
* Documentation
*/
default DisassociateDrtRoleResponse disassociateDRTRole(
Consumer disassociateDrtRoleRequest) throws InternalErrorException,
InvalidOperationException, OptimisticLockException, ResourceNotFoundException, AwsServiceException,
SdkClientException, ShieldException {
return disassociateDRTRole(DisassociateDrtRoleRequest.builder().applyMutation(disassociateDrtRoleRequest).build());
}
/**
*
* Returns the SubscriptionState
, either Active
or Inactive
.
*
*
* @return Result of the GetSubscriptionState operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.GetSubscriptionState
* @see #getSubscriptionState(GetSubscriptionStateRequest)
* @see AWS
* API Documentation
*/
default GetSubscriptionStateResponse getSubscriptionState() throws InternalErrorException, AwsServiceException,
SdkClientException, ShieldException {
return getSubscriptionState(GetSubscriptionStateRequest.builder().build());
}
/**
*
* Returns the SubscriptionState
, either Active
or Inactive
.
*
*
* @param getSubscriptionStateRequest
* @return Result of the GetSubscriptionState operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.GetSubscriptionState
* @see AWS
* API Documentation
*/
default GetSubscriptionStateResponse getSubscriptionState(GetSubscriptionStateRequest getSubscriptionStateRequest)
throws InternalErrorException, AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the SubscriptionState
, either Active
or Inactive
.
*
*
*
* This is a convenience which creates an instance of the {@link GetSubscriptionStateRequest.Builder} avoiding the
* need to create one manually via {@link GetSubscriptionStateRequest#builder()}
*
*
* @param getSubscriptionStateRequest
* A {@link Consumer} that will call methods on {@link GetSubscriptionStateRequest.Builder} to create a
* request.
* @return Result of the GetSubscriptionState operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.GetSubscriptionState
* @see AWS
* API Documentation
*/
default GetSubscriptionStateResponse getSubscriptionState(
Consumer getSubscriptionStateRequest) throws InternalErrorException,
AwsServiceException, SdkClientException, ShieldException {
return getSubscriptionState(GetSubscriptionStateRequest.builder().applyMutation(getSubscriptionStateRequest).build());
}
/**
*
* Returns all ongoing DDoS attacks or all DDoS attacks during a specified time period.
*
*
* @return Result of the ListAttacks operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.ListAttacks
* @see #listAttacks(ListAttacksRequest)
* @see AWS API
* Documentation
*/
default ListAttacksResponse listAttacks() throws InternalErrorException, InvalidParameterException,
InvalidOperationException, AwsServiceException, SdkClientException, ShieldException {
return listAttacks(ListAttacksRequest.builder().build());
}
/**
*
* Returns all ongoing DDoS attacks or all DDoS attacks during a specified time period.
*
*
* @param listAttacksRequest
* @return Result of the ListAttacks operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.ListAttacks
* @see AWS API
* Documentation
*/
default ListAttacksResponse listAttacks(ListAttacksRequest listAttacksRequest) throws InternalErrorException,
InvalidParameterException, InvalidOperationException, AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Returns all ongoing DDoS attacks or all DDoS attacks during a specified time period.
*
*
*
* This is a convenience which creates an instance of the {@link ListAttacksRequest.Builder} avoiding the need to
* create one manually via {@link ListAttacksRequest#builder()}
*
*
* @param listAttacksRequest
* A {@link Consumer} that will call methods on {@link ListAttacksRequest.Builder} to create a request.
* @return Result of the ListAttacks operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws InvalidOperationException
* Exception that indicates that the operation would not cause any change to occur.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.ListAttacks
* @see AWS API
* Documentation
*/
default ListAttacksResponse listAttacks(Consumer listAttacksRequest)
throws InternalErrorException, InvalidParameterException, InvalidOperationException, AwsServiceException,
SdkClientException, ShieldException {
return listAttacks(ListAttacksRequest.builder().applyMutation(listAttacksRequest).build());
}
/**
*
* Lists all Protection objects for the account.
*
*
* @return Result of the ListProtections operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws InvalidPaginationTokenException
* Exception that indicates that the NextToken specified in the request is invalid. Submit the request using
* the NextToken value that was returned in the response.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.ListProtections
* @see #listProtections(ListProtectionsRequest)
* @see AWS API
* Documentation
*/
default ListProtectionsResponse listProtections() throws InternalErrorException, ResourceNotFoundException,
InvalidPaginationTokenException, AwsServiceException, SdkClientException, ShieldException {
return listProtections(ListProtectionsRequest.builder().build());
}
/**
*
* Lists all Protection objects for the account.
*
*
* @param listProtectionsRequest
* @return Result of the ListProtections operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws InvalidPaginationTokenException
* Exception that indicates that the NextToken specified in the request is invalid. Submit the request using
* the NextToken value that was returned in the response.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.ListProtections
* @see AWS API
* Documentation
*/
default ListProtectionsResponse listProtections(ListProtectionsRequest listProtectionsRequest) throws InternalErrorException,
ResourceNotFoundException, InvalidPaginationTokenException, AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all Protection objects for the account.
*
*
*
* This is a convenience which creates an instance of the {@link ListProtectionsRequest.Builder} avoiding the need
* to create one manually via {@link ListProtectionsRequest#builder()}
*
*
* @param listProtectionsRequest
* A {@link Consumer} that will call methods on {@link ListProtectionsRequest.Builder} to create a request.
* @return Result of the ListProtections operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws InvalidPaginationTokenException
* Exception that indicates that the NextToken specified in the request is invalid. Submit the request using
* the NextToken value that was returned in the response.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.ListProtections
* @see AWS API
* Documentation
*/
default ListProtectionsResponse listProtections(Consumer listProtectionsRequest)
throws InternalErrorException, ResourceNotFoundException, InvalidPaginationTokenException, AwsServiceException,
SdkClientException, ShieldException {
return listProtections(ListProtectionsRequest.builder().applyMutation(listProtectionsRequest).build());
}
/**
*
* Updates the details of the list of email addresses that the DRT can use to contact you during a suspected attack.
*
*
* @param updateEmergencyContactSettingsRequest
* @return Result of the UpdateEmergencyContactSettings operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.UpdateEmergencyContactSettings
* @see AWS API Documentation
*/
default UpdateEmergencyContactSettingsResponse updateEmergencyContactSettings(
UpdateEmergencyContactSettingsRequest updateEmergencyContactSettingsRequest) throws InternalErrorException,
InvalidParameterException, OptimisticLockException, ResourceNotFoundException, AwsServiceException,
SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the details of the list of email addresses that the DRT can use to contact you during a suspected attack.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateEmergencyContactSettingsRequest.Builder}
* avoiding the need to create one manually via {@link UpdateEmergencyContactSettingsRequest#builder()}
*
*
* @param updateEmergencyContactSettingsRequest
* A {@link Consumer} that will call methods on {@link UpdateEmergencyContactSettingsRequest.Builder} to
* create a request.
* @return Result of the UpdateEmergencyContactSettings operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.UpdateEmergencyContactSettings
* @see AWS API Documentation
*/
default UpdateEmergencyContactSettingsResponse updateEmergencyContactSettings(
Consumer updateEmergencyContactSettingsRequest)
throws InternalErrorException, InvalidParameterException, OptimisticLockException, ResourceNotFoundException,
AwsServiceException, SdkClientException, ShieldException {
return updateEmergencyContactSettings(UpdateEmergencyContactSettingsRequest.builder()
.applyMutation(updateEmergencyContactSettingsRequest).build());
}
/**
*
* Updates the details of an existing subscription. Only enter values for parameters you want to change. Empty
* parameters are not updated.
*
*
* @param updateSubscriptionRequest
* @return Result of the UpdateSubscription operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws LockedSubscriptionException
* You are trying to update a subscription that has not yet completed the 1-year commitment. You can change
* the AutoRenew
parameter during the last 30 days of your subscription. This exception
* indicates that you are attempting to change AutoRenew
prior to that period.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.UpdateSubscription
* @see AWS API
* Documentation
*/
default UpdateSubscriptionResponse updateSubscription(UpdateSubscriptionRequest updateSubscriptionRequest)
throws InternalErrorException, LockedSubscriptionException, ResourceNotFoundException, InvalidParameterException,
OptimisticLockException, AwsServiceException, SdkClientException, ShieldException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the details of an existing subscription. Only enter values for parameters you want to change. Empty
* parameters are not updated.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSubscriptionRequest.Builder} avoiding the
* need to create one manually via {@link UpdateSubscriptionRequest#builder()}
*
*
* @param updateSubscriptionRequest
* A {@link Consumer} that will call methods on {@link UpdateSubscriptionRequest.Builder} to create a
* request.
* @return Result of the UpdateSubscription operation returned by the service.
* @throws InternalErrorException
* Exception that indicates that a problem occurred with the service infrastructure. You can retry the
* request.
* @throws LockedSubscriptionException
* You are trying to update a subscription that has not yet completed the 1-year commitment. You can change
* the AutoRenew
parameter during the last 30 days of your subscription. This exception
* indicates that you are attempting to change AutoRenew
prior to that period.
* @throws ResourceNotFoundException
* Exception indicating the specified resource does not exist.
* @throws InvalidParameterException
* Exception that indicates that the parameters passed to the API are invalid.
* @throws OptimisticLockException
* Exception that indicates that the protection state has been modified by another client. You can retry the
* request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws ShieldException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample ShieldClient.UpdateSubscription
* @see AWS API
* Documentation
*/
default UpdateSubscriptionResponse updateSubscription(Consumer updateSubscriptionRequest)
throws InternalErrorException, LockedSubscriptionException, ResourceNotFoundException, InvalidParameterException,
OptimisticLockException, AwsServiceException, SdkClientException, ShieldException {
return updateSubscription(UpdateSubscriptionRequest.builder().applyMutation(updateSubscriptionRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of("shield");
}
}