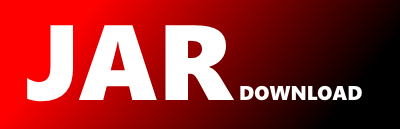
software.amazon.awssdk.services.signer.DefaultSignerClient Maven / Gradle / Ivy
Show all versions of signer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.signer;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.signer.model.AccessDeniedException;
import software.amazon.awssdk.services.signer.model.AddProfilePermissionRequest;
import software.amazon.awssdk.services.signer.model.AddProfilePermissionResponse;
import software.amazon.awssdk.services.signer.model.BadRequestException;
import software.amazon.awssdk.services.signer.model.CancelSigningProfileRequest;
import software.amazon.awssdk.services.signer.model.CancelSigningProfileResponse;
import software.amazon.awssdk.services.signer.model.ConflictException;
import software.amazon.awssdk.services.signer.model.DescribeSigningJobRequest;
import software.amazon.awssdk.services.signer.model.DescribeSigningJobResponse;
import software.amazon.awssdk.services.signer.model.GetSigningPlatformRequest;
import software.amazon.awssdk.services.signer.model.GetSigningPlatformResponse;
import software.amazon.awssdk.services.signer.model.GetSigningProfileRequest;
import software.amazon.awssdk.services.signer.model.GetSigningProfileResponse;
import software.amazon.awssdk.services.signer.model.InternalServiceErrorException;
import software.amazon.awssdk.services.signer.model.ListProfilePermissionsRequest;
import software.amazon.awssdk.services.signer.model.ListProfilePermissionsResponse;
import software.amazon.awssdk.services.signer.model.ListSigningJobsRequest;
import software.amazon.awssdk.services.signer.model.ListSigningJobsResponse;
import software.amazon.awssdk.services.signer.model.ListSigningPlatformsRequest;
import software.amazon.awssdk.services.signer.model.ListSigningPlatformsResponse;
import software.amazon.awssdk.services.signer.model.ListSigningProfilesRequest;
import software.amazon.awssdk.services.signer.model.ListSigningProfilesResponse;
import software.amazon.awssdk.services.signer.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.signer.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.signer.model.NotFoundException;
import software.amazon.awssdk.services.signer.model.PutSigningProfileRequest;
import software.amazon.awssdk.services.signer.model.PutSigningProfileResponse;
import software.amazon.awssdk.services.signer.model.RemoveProfilePermissionRequest;
import software.amazon.awssdk.services.signer.model.RemoveProfilePermissionResponse;
import software.amazon.awssdk.services.signer.model.ResourceNotFoundException;
import software.amazon.awssdk.services.signer.model.RevokeSignatureRequest;
import software.amazon.awssdk.services.signer.model.RevokeSignatureResponse;
import software.amazon.awssdk.services.signer.model.RevokeSigningProfileRequest;
import software.amazon.awssdk.services.signer.model.RevokeSigningProfileResponse;
import software.amazon.awssdk.services.signer.model.ServiceLimitExceededException;
import software.amazon.awssdk.services.signer.model.SignerException;
import software.amazon.awssdk.services.signer.model.SignerRequest;
import software.amazon.awssdk.services.signer.model.StartSigningJobRequest;
import software.amazon.awssdk.services.signer.model.StartSigningJobResponse;
import software.amazon.awssdk.services.signer.model.TagResourceRequest;
import software.amazon.awssdk.services.signer.model.TagResourceResponse;
import software.amazon.awssdk.services.signer.model.ThrottlingException;
import software.amazon.awssdk.services.signer.model.TooManyRequestsException;
import software.amazon.awssdk.services.signer.model.UntagResourceRequest;
import software.amazon.awssdk.services.signer.model.UntagResourceResponse;
import software.amazon.awssdk.services.signer.model.ValidationException;
import software.amazon.awssdk.services.signer.paginators.ListSigningJobsIterable;
import software.amazon.awssdk.services.signer.paginators.ListSigningPlatformsIterable;
import software.amazon.awssdk.services.signer.paginators.ListSigningProfilesIterable;
import software.amazon.awssdk.services.signer.transform.AddProfilePermissionRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.CancelSigningProfileRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.DescribeSigningJobRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.GetSigningPlatformRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.GetSigningProfileRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.ListProfilePermissionsRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.ListSigningJobsRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.ListSigningPlatformsRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.ListSigningProfilesRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.PutSigningProfileRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.RemoveProfilePermissionRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.RevokeSignatureRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.RevokeSigningProfileRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.StartSigningJobRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.signer.waiters.SignerWaiter;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link SignerClient}.
*
* @see SignerClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultSignerClient implements SignerClient {
private static final Logger log = Logger.loggerFor(DefaultSignerClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultSignerClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Adds cross-account permissions to a signing profile.
*
*
* @param addProfilePermissionRequest
* @return Result of the AddProfilePermission operation returned by the service.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ServiceLimitExceededException
* The client is making a request that exceeds service limits.
* @throws ConflictException
* The resource encountered a conflicting state.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.AddProfilePermission
* @see AWS
* API Documentation
*/
@Override
public AddProfilePermissionResponse addProfilePermission(AddProfilePermissionRequest addProfilePermissionRequest)
throws ValidationException, ResourceNotFoundException, AccessDeniedException, ServiceLimitExceededException,
ConflictException, TooManyRequestsException, InternalServiceErrorException, AwsServiceException, SdkClientException,
SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AddProfilePermissionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addProfilePermissionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddProfilePermission");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AddProfilePermission").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(addProfilePermissionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AddProfilePermissionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Changes the state of an ACTIVE
signing profile to CANCELED
. A canceled profile is still
* viewable with the ListSigningProfiles
operation, but it cannot perform new signing jobs, and is
* deleted two years after cancelation.
*
*
* @param cancelSigningProfileRequest
* @return Result of the CancelSigningProfile operation returned by the service.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.CancelSigningProfile
* @see AWS
* API Documentation
*/
@Override
public CancelSigningProfileResponse cancelSigningProfile(CancelSigningProfileRequest cancelSigningProfileRequest)
throws ResourceNotFoundException, AccessDeniedException, TooManyRequestsException, InternalServiceErrorException,
AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelSigningProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelSigningProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelSigningProfile");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CancelSigningProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(cancelSigningProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CancelSigningProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information about a specific code signing job. You specify the job by using the jobId
value
* that is returned by the StartSigningJob operation.
*
*
* @param describeSigningJobRequest
* @return Result of the DescribeSigningJob operation returned by the service.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.DescribeSigningJob
* @see AWS API
* Documentation
*/
@Override
public DescribeSigningJobResponse describeSigningJob(DescribeSigningJobRequest describeSigningJobRequest)
throws ResourceNotFoundException, AccessDeniedException, TooManyRequestsException, InternalServiceErrorException,
AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSigningJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeSigningJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSigningJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeSigningJob").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeSigningJobRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeSigningJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information on a specific signing platform.
*
*
* @param getSigningPlatformRequest
* @return Result of the GetSigningPlatform operation returned by the service.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.GetSigningPlatform
* @see AWS API
* Documentation
*/
@Override
public GetSigningPlatformResponse getSigningPlatform(GetSigningPlatformRequest getSigningPlatformRequest)
throws ResourceNotFoundException, AccessDeniedException, TooManyRequestsException, InternalServiceErrorException,
AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetSigningPlatformResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSigningPlatformRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSigningPlatform");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetSigningPlatform").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getSigningPlatformRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetSigningPlatformRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns information on a specific signing profile.
*
*
* @param getSigningProfileRequest
* @return Result of the GetSigningProfile operation returned by the service.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.GetSigningProfile
* @see AWS API
* Documentation
*/
@Override
public GetSigningProfileResponse getSigningProfile(GetSigningProfileRequest getSigningProfileRequest)
throws ResourceNotFoundException, AccessDeniedException, TooManyRequestsException, InternalServiceErrorException,
AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetSigningProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSigningProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSigningProfile");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetSigningProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getSigningProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetSigningProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the cross-account permissions associated with a signing profile.
*
*
* @param listProfilePermissionsRequest
* @return Result of the ListProfilePermissions operation returned by the service.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.ListProfilePermissions
* @see AWS
* API Documentation
*/
@Override
public ListProfilePermissionsResponse listProfilePermissions(ListProfilePermissionsRequest listProfilePermissionsRequest)
throws ValidationException, ResourceNotFoundException, AccessDeniedException, TooManyRequestsException,
InternalServiceErrorException, AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListProfilePermissionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listProfilePermissionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListProfilePermissions");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListProfilePermissions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listProfilePermissionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListProfilePermissionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all your signing jobs. You can use the maxResults
parameter to limit the number of signing
* jobs that are returned in the response. If additional jobs remain to be listed, code signing returns a
* nextToken
value. Use this value in subsequent calls to ListSigningJobs
to fetch the
* remaining values. You can continue calling ListSigningJobs
with your maxResults
* parameter and with new values that code signing returns in the nextToken
parameter until all of your
* signing jobs have been returned.
*
*
* @param listSigningJobsRequest
* @return Result of the ListSigningJobs operation returned by the service.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.ListSigningJobs
* @see AWS API
* Documentation
*/
@Override
public ListSigningJobsResponse listSigningJobs(ListSigningJobsRequest listSigningJobsRequest) throws ValidationException,
AccessDeniedException, TooManyRequestsException, InternalServiceErrorException, AwsServiceException,
SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListSigningJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSigningJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSigningJobs");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListSigningJobs").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listSigningJobsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListSigningJobsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all your signing jobs. You can use the maxResults
parameter to limit the number of signing
* jobs that are returned in the response. If additional jobs remain to be listed, code signing returns a
* nextToken
value. Use this value in subsequent calls to ListSigningJobs
to fetch the
* remaining values. You can continue calling ListSigningJobs
with your maxResults
* parameter and with new values that code signing returns in the nextToken
parameter until all of your
* signing jobs have been returned.
*
*
*
* This is a variant of
* {@link #listSigningJobs(software.amazon.awssdk.services.signer.model.ListSigningJobsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.signer.paginators.ListSigningJobsIterable responses = client.listSigningJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.signer.paginators.ListSigningJobsIterable responses = client
* .listSigningJobsPaginator(request);
* for (software.amazon.awssdk.services.signer.model.ListSigningJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.signer.paginators.ListSigningJobsIterable responses = client.listSigningJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSigningJobs(software.amazon.awssdk.services.signer.model.ListSigningJobsRequest)} operation.
*
*
* @param listSigningJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.ListSigningJobs
* @see AWS API
* Documentation
*/
@Override
public ListSigningJobsIterable listSigningJobsPaginator(ListSigningJobsRequest listSigningJobsRequest)
throws ValidationException, AccessDeniedException, TooManyRequestsException, InternalServiceErrorException,
AwsServiceException, SdkClientException, SignerException {
return new ListSigningJobsIterable(this, applyPaginatorUserAgent(listSigningJobsRequest));
}
/**
*
* Lists all signing platforms available in code signing that match the request parameters. If additional jobs
* remain to be listed, code signing returns a nextToken
value. Use this value in subsequent calls to
* ListSigningJobs
to fetch the remaining values. You can continue calling ListSigningJobs
* with your maxResults
parameter and with new values that code signing returns in the
* nextToken
parameter until all of your signing jobs have been returned.
*
*
* @param listSigningPlatformsRequest
* @return Result of the ListSigningPlatforms operation returned by the service.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.ListSigningPlatforms
* @see AWS
* API Documentation
*/
@Override
public ListSigningPlatformsResponse listSigningPlatforms(ListSigningPlatformsRequest listSigningPlatformsRequest)
throws ValidationException, AccessDeniedException, TooManyRequestsException, InternalServiceErrorException,
AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSigningPlatformsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSigningPlatformsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSigningPlatforms");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListSigningPlatforms").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listSigningPlatformsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListSigningPlatformsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all signing platforms available in code signing that match the request parameters. If additional jobs
* remain to be listed, code signing returns a nextToken
value. Use this value in subsequent calls to
* ListSigningJobs
to fetch the remaining values. You can continue calling ListSigningJobs
* with your maxResults
parameter and with new values that code signing returns in the
* nextToken
parameter until all of your signing jobs have been returned.
*
*
*
* This is a variant of
* {@link #listSigningPlatforms(software.amazon.awssdk.services.signer.model.ListSigningPlatformsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.signer.paginators.ListSigningPlatformsIterable responses = client.listSigningPlatformsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.signer.paginators.ListSigningPlatformsIterable responses = client
* .listSigningPlatformsPaginator(request);
* for (software.amazon.awssdk.services.signer.model.ListSigningPlatformsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.signer.paginators.ListSigningPlatformsIterable responses = client.listSigningPlatformsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSigningPlatforms(software.amazon.awssdk.services.signer.model.ListSigningPlatformsRequest)}
* operation.
*
*
* @param listSigningPlatformsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.ListSigningPlatforms
* @see AWS
* API Documentation
*/
@Override
public ListSigningPlatformsIterable listSigningPlatformsPaginator(ListSigningPlatformsRequest listSigningPlatformsRequest)
throws ValidationException, AccessDeniedException, TooManyRequestsException, InternalServiceErrorException,
AwsServiceException, SdkClientException, SignerException {
return new ListSigningPlatformsIterable(this, applyPaginatorUserAgent(listSigningPlatformsRequest));
}
/**
*
* Lists all available signing profiles in your AWS account. Returns only profiles with an ACTIVE
* status unless the includeCanceled
request field is set to true
. If additional jobs
* remain to be listed, code signing returns a nextToken
value. Use this value in subsequent calls to
* ListSigningJobs
to fetch the remaining values. You can continue calling ListSigningJobs
* with your maxResults
parameter and with new values that code signing returns in the
* nextToken
parameter until all of your signing jobs have been returned.
*
*
* @param listSigningProfilesRequest
* @return Result of the ListSigningProfiles operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.ListSigningProfiles
* @see AWS
* API Documentation
*/
@Override
public ListSigningProfilesResponse listSigningProfiles(ListSigningProfilesRequest listSigningProfilesRequest)
throws AccessDeniedException, TooManyRequestsException, InternalServiceErrorException, AwsServiceException,
SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSigningProfilesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSigningProfilesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSigningProfiles");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListSigningProfiles").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listSigningProfilesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListSigningProfilesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all available signing profiles in your AWS account. Returns only profiles with an ACTIVE
* status unless the includeCanceled
request field is set to true
. If additional jobs
* remain to be listed, code signing returns a nextToken
value. Use this value in subsequent calls to
* ListSigningJobs
to fetch the remaining values. You can continue calling ListSigningJobs
* with your maxResults
parameter and with new values that code signing returns in the
* nextToken
parameter until all of your signing jobs have been returned.
*
*
*
* This is a variant of
* {@link #listSigningProfiles(software.amazon.awssdk.services.signer.model.ListSigningProfilesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.signer.paginators.ListSigningProfilesIterable responses = client.listSigningProfilesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.signer.paginators.ListSigningProfilesIterable responses = client
* .listSigningProfilesPaginator(request);
* for (software.amazon.awssdk.services.signer.model.ListSigningProfilesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.signer.paginators.ListSigningProfilesIterable responses = client.listSigningProfilesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSigningProfiles(software.amazon.awssdk.services.signer.model.ListSigningProfilesRequest)}
* operation.
*
*
* @param listSigningProfilesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.ListSigningProfiles
* @see AWS
* API Documentation
*/
@Override
public ListSigningProfilesIterable listSigningProfilesPaginator(ListSigningProfilesRequest listSigningProfilesRequest)
throws AccessDeniedException, TooManyRequestsException, InternalServiceErrorException, AwsServiceException,
SdkClientException, SignerException {
return new ListSigningProfilesIterable(this, applyPaginatorUserAgent(listSigningProfilesRequest));
}
/**
*
* Returns a list of the tags associated with a signing profile resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws BadRequestException
* The request contains invalid parameters for the ARN or tags. This exception also occurs when you call a
* tagging API on a cancelled signing profile.
* @throws NotFoundException
* The signing profile was not found.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InternalServiceErrorException, BadRequestException, NotFoundException, TooManyRequestsException,
AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a signing profile. A signing profile is a code signing template that can be used to carry out a
* pre-defined signing job. For more information, see http://docs.aws.amazon.com/signer/latest/developerguide/gs-profile.html
*
*
* @param putSigningProfileRequest
* @return Result of the PutSigningProfile operation returned by the service.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.PutSigningProfile
* @see AWS API
* Documentation
*/
@Override
public PutSigningProfileResponse putSigningProfile(PutSigningProfileRequest putSigningProfileRequest)
throws ResourceNotFoundException, AccessDeniedException, ValidationException, TooManyRequestsException,
InternalServiceErrorException, AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutSigningProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putSigningProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutSigningProfile");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutSigningProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putSigningProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutSigningProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes cross-account permissions from a signing profile.
*
*
* @param removeProfilePermissionRequest
* @return Result of the RemoveProfilePermission operation returned by the service.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ConflictException
* The resource encountered a conflicting state.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.RemoveProfilePermission
* @see AWS API Documentation
*/
@Override
public RemoveProfilePermissionResponse removeProfilePermission(RemoveProfilePermissionRequest removeProfilePermissionRequest)
throws ValidationException, ResourceNotFoundException, AccessDeniedException, ConflictException,
TooManyRequestsException, InternalServiceErrorException, AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemoveProfilePermissionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeProfilePermissionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveProfilePermission");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveProfilePermission").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(removeProfilePermissionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RemoveProfilePermissionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Changes the state of a signing job to REVOKED. This indicates that the signature is no longer valid.
*
*
* @param revokeSignatureRequest
* @return Result of the RevokeSignature operation returned by the service.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.RevokeSignature
* @see AWS API
* Documentation
*/
@Override
public RevokeSignatureResponse revokeSignature(RevokeSignatureRequest revokeSignatureRequest) throws ValidationException,
AccessDeniedException, ResourceNotFoundException, TooManyRequestsException, InternalServiceErrorException,
AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RevokeSignatureResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, revokeSignatureRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RevokeSignature");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RevokeSignature").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(revokeSignatureRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RevokeSignatureRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Changes the state of a signing profile to REVOKED. This indicates that signatures generated using the signing
* profile after an effective start date are no longer valid.
*
*
* @param revokeSigningProfileRequest
* @return Result of the RevokeSigningProfile operation returned by the service.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.RevokeSigningProfile
* @see AWS
* API Documentation
*/
@Override
public RevokeSigningProfileResponse revokeSigningProfile(RevokeSigningProfileRequest revokeSigningProfileRequest)
throws ValidationException, AccessDeniedException, ResourceNotFoundException, TooManyRequestsException,
InternalServiceErrorException, AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RevokeSigningProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, revokeSigningProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RevokeSigningProfile");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RevokeSigningProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(revokeSigningProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RevokeSigningProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Initiates a signing job to be performed on the code provided. Signing jobs are viewable by the
* ListSigningJobs
operation for two years after they are performed. Note the following requirements:
*
*
* -
*
* You must create an Amazon S3 source bucket. For more information, see Create a Bucket in the Amazon
* S3 Getting Started Guide.
*
*
* -
*
* Your S3 source bucket must be version enabled.
*
*
* -
*
* You must create an S3 destination bucket. Code signing uses your S3 destination bucket to write your signed code.
*
*
* -
*
* You specify the name of the source and destination buckets when calling the StartSigningJob
* operation.
*
*
* -
*
* You must also specify a request token that identifies your request to code signing.
*
*
*
*
* You can call the DescribeSigningJob and the ListSigningJobs actions after you call
* StartSigningJob
.
*
*
* For a Java example that shows how to use this action, see http://docs.aws.amazon.com/acm/latest/userguide/
*
*
* @param startSigningJobRequest
* @return Result of the StartSigningJob operation returned by the service.
* @throws ValidationException
* You signing certificate could not be validated.
* @throws ResourceNotFoundException
* A specified resource could not be found.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
*
* Instead of this error, TooManyRequestsException
should be used.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
*
* This error supersedes the error ThrottlingException
.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.StartSigningJob
* @see AWS API
* Documentation
*/
@Override
public StartSigningJobResponse startSigningJob(StartSigningJobRequest startSigningJobRequest) throws ValidationException,
ResourceNotFoundException, AccessDeniedException, ThrottlingException, TooManyRequestsException,
InternalServiceErrorException, AwsServiceException, SdkClientException, SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartSigningJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startSigningJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartSigningJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StartSigningJob").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(startSigningJobRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartSigningJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds one or more tags to a signing profile. Tags are labels that you can use to identify and organize your AWS
* resources. Each tag consists of a key and an optional value. To specify the signing profile, use its Amazon
* Resource Name (ARN). To specify the tag, use a key-value pair.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws BadRequestException
* The request contains invalid parameters for the ARN or tags. This exception also occurs when you call a
* tagging API on a cancelled signing profile.
* @throws NotFoundException
* The signing profile was not found.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InternalServiceErrorException,
BadRequestException, NotFoundException, TooManyRequestsException, AwsServiceException, SdkClientException,
SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes one or more tags from a signing profile. To remove the tags, specify a list of tag keys.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServiceErrorException
* An internal error occurred.
* @throws BadRequestException
* The request contains invalid parameters for the ARN or tags. This exception also occurs when you call a
* tagging API on a cancelled signing profile.
* @throws NotFoundException
* The signing profile was not found.
* @throws TooManyRequestsException
* The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SignerException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SignerClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InternalServiceErrorException,
BadRequestException, NotFoundException, TooManyRequestsException, AwsServiceException, SdkClientException,
SignerException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(SignerException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceLimitExceededException")
.exceptionBuilderSupplier(ServiceLimitExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NotFoundException")
.exceptionBuilderSupplier(NotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServiceErrorException")
.exceptionBuilderSupplier(InternalServiceErrorException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRequestsException")
.exceptionBuilderSupplier(TooManyRequestsException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BadRequestException")
.exceptionBuilderSupplier(BadRequestException::builder).httpStatusCode(400).build());
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
@Override
public SignerWaiter waiter() {
return SignerWaiter.builder().client(this).build();
}
}