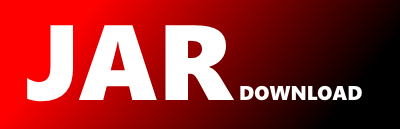
software.amazon.awssdk.services.signer.DefaultSignerAsyncClient Maven / Gradle / Ivy
Show all versions of signer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.signer;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.signer.internal.SignerServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.signer.model.AccessDeniedException;
import software.amazon.awssdk.services.signer.model.AddProfilePermissionRequest;
import software.amazon.awssdk.services.signer.model.AddProfilePermissionResponse;
import software.amazon.awssdk.services.signer.model.BadRequestException;
import software.amazon.awssdk.services.signer.model.CancelSigningProfileRequest;
import software.amazon.awssdk.services.signer.model.CancelSigningProfileResponse;
import software.amazon.awssdk.services.signer.model.ConflictException;
import software.amazon.awssdk.services.signer.model.DescribeSigningJobRequest;
import software.amazon.awssdk.services.signer.model.DescribeSigningJobResponse;
import software.amazon.awssdk.services.signer.model.GetRevocationStatusRequest;
import software.amazon.awssdk.services.signer.model.GetRevocationStatusResponse;
import software.amazon.awssdk.services.signer.model.GetSigningPlatformRequest;
import software.amazon.awssdk.services.signer.model.GetSigningPlatformResponse;
import software.amazon.awssdk.services.signer.model.GetSigningProfileRequest;
import software.amazon.awssdk.services.signer.model.GetSigningProfileResponse;
import software.amazon.awssdk.services.signer.model.InternalServiceErrorException;
import software.amazon.awssdk.services.signer.model.ListProfilePermissionsRequest;
import software.amazon.awssdk.services.signer.model.ListProfilePermissionsResponse;
import software.amazon.awssdk.services.signer.model.ListSigningJobsRequest;
import software.amazon.awssdk.services.signer.model.ListSigningJobsResponse;
import software.amazon.awssdk.services.signer.model.ListSigningPlatformsRequest;
import software.amazon.awssdk.services.signer.model.ListSigningPlatformsResponse;
import software.amazon.awssdk.services.signer.model.ListSigningProfilesRequest;
import software.amazon.awssdk.services.signer.model.ListSigningProfilesResponse;
import software.amazon.awssdk.services.signer.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.signer.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.signer.model.NotFoundException;
import software.amazon.awssdk.services.signer.model.PutSigningProfileRequest;
import software.amazon.awssdk.services.signer.model.PutSigningProfileResponse;
import software.amazon.awssdk.services.signer.model.RemoveProfilePermissionRequest;
import software.amazon.awssdk.services.signer.model.RemoveProfilePermissionResponse;
import software.amazon.awssdk.services.signer.model.ResourceNotFoundException;
import software.amazon.awssdk.services.signer.model.RevokeSignatureRequest;
import software.amazon.awssdk.services.signer.model.RevokeSignatureResponse;
import software.amazon.awssdk.services.signer.model.RevokeSigningProfileRequest;
import software.amazon.awssdk.services.signer.model.RevokeSigningProfileResponse;
import software.amazon.awssdk.services.signer.model.ServiceLimitExceededException;
import software.amazon.awssdk.services.signer.model.SignPayloadRequest;
import software.amazon.awssdk.services.signer.model.SignPayloadResponse;
import software.amazon.awssdk.services.signer.model.SignerException;
import software.amazon.awssdk.services.signer.model.StartSigningJobRequest;
import software.amazon.awssdk.services.signer.model.StartSigningJobResponse;
import software.amazon.awssdk.services.signer.model.TagResourceRequest;
import software.amazon.awssdk.services.signer.model.TagResourceResponse;
import software.amazon.awssdk.services.signer.model.ThrottlingException;
import software.amazon.awssdk.services.signer.model.TooManyRequestsException;
import software.amazon.awssdk.services.signer.model.UntagResourceRequest;
import software.amazon.awssdk.services.signer.model.UntagResourceResponse;
import software.amazon.awssdk.services.signer.model.ValidationException;
import software.amazon.awssdk.services.signer.transform.AddProfilePermissionRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.CancelSigningProfileRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.DescribeSigningJobRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.GetRevocationStatusRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.GetSigningPlatformRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.GetSigningProfileRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.ListProfilePermissionsRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.ListSigningJobsRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.ListSigningPlatformsRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.ListSigningProfilesRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.PutSigningProfileRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.RemoveProfilePermissionRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.RevokeSignatureRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.RevokeSigningProfileRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.SignPayloadRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.StartSigningJobRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.signer.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.signer.waiters.SignerAsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link SignerAsyncClient}.
*
* @see SignerAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultSignerAsyncClient implements SignerAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultSignerAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultSignerAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
/**
*
* Adds cross-account permissions to a signing profile.
*
*
* @param addProfilePermissionRequest
* @return A Java Future containing the result of the AddProfilePermission operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException You signing certificate could not be validated.
* - ResourceNotFoundException A specified resource could not be found.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ServiceLimitExceededException The client is making a request that exceeds service limits.
* - ConflictException The resource encountered a conflicting state.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.AddProfilePermission
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture addProfilePermission(
AddProfilePermissionRequest addProfilePermissionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addProfilePermissionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addProfilePermissionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddProfilePermission");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AddProfilePermissionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddProfilePermission").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AddProfilePermissionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(addProfilePermissionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Changes the state of an ACTIVE
signing profile to CANCELED
. A canceled profile is still
* viewable with the ListSigningProfiles
operation, but it cannot perform new signing jobs, and is
* deleted two years after cancelation.
*
*
* @param cancelSigningProfileRequest
* @return A Java Future containing the result of the CancelSigningProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException A specified resource could not be found.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.CancelSigningProfile
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture cancelSigningProfile(
CancelSigningProfileRequest cancelSigningProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelSigningProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelSigningProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelSigningProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelSigningProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelSigningProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelSigningProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelSigningProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about a specific code signing job. You specify the job by using the jobId
value
* that is returned by the StartSigningJob operation.
*
*
* @param describeSigningJobRequest
* @return A Java Future containing the result of the DescribeSigningJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException A specified resource could not be found.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.DescribeSigningJob
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeSigningJob(DescribeSigningJobRequest describeSigningJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeSigningJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeSigningJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeSigningJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeSigningJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeSigningJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeSigningJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeSigningJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the revocation status of one or more of the signing profile, signing job, and signing certificate.
*
*
* @param getRevocationStatusRequest
* @return A Java Future containing the result of the GetRevocationStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException You signing certificate could not be validated.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.GetRevocationStatus
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getRevocationStatus(
GetRevocationStatusRequest getRevocationStatusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getRevocationStatusRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRevocationStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRevocationStatus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRevocationStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
String hostPrefix = "verification.";
String resolvedHostExpression = "verification.";
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetRevocationStatus").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetRevocationStatusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.hostPrefixExpression(resolvedHostExpression).withInput(getRevocationStatusRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information on a specific signing platform.
*
*
* @param getSigningPlatformRequest
* @return A Java Future containing the result of the GetSigningPlatform operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException A specified resource could not be found.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.GetSigningPlatform
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getSigningPlatform(GetSigningPlatformRequest getSigningPlatformRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getSigningPlatformRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSigningPlatformRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSigningPlatform");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetSigningPlatformResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSigningPlatform").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetSigningPlatformRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getSigningPlatformRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information on a specific signing profile.
*
*
* @param getSigningProfileRequest
* @return A Java Future containing the result of the GetSigningProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException A specified resource could not be found.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.GetSigningProfile
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getSigningProfile(GetSigningProfileRequest getSigningProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getSigningProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSigningProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSigningProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetSigningProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSigningProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetSigningProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getSigningProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the cross-account permissions associated with a signing profile.
*
*
* @param listProfilePermissionsRequest
* @return A Java Future containing the result of the ListProfilePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException You signing certificate could not be validated.
* - ResourceNotFoundException A specified resource could not be found.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.ListProfilePermissions
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listProfilePermissions(
ListProfilePermissionsRequest listProfilePermissionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listProfilePermissionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listProfilePermissionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListProfilePermissions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListProfilePermissionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListProfilePermissions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListProfilePermissionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listProfilePermissionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all your signing jobs. You can use the maxResults
parameter to limit the number of signing
* jobs that are returned in the response. If additional jobs remain to be listed, AWS Signer returns a
* nextToken
value. Use this value in subsequent calls to ListSigningJobs
to fetch the
* remaining values. You can continue calling ListSigningJobs
with your maxResults
* parameter and with new values that Signer returns in the nextToken
parameter until all of your
* signing jobs have been returned.
*
*
* @param listSigningJobsRequest
* @return A Java Future containing the result of the ListSigningJobs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException You signing certificate could not be validated.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.ListSigningJobs
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listSigningJobs(ListSigningJobsRequest listSigningJobsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listSigningJobsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSigningJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSigningJobs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSigningJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSigningJobs").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListSigningJobsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listSigningJobsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all signing platforms available in AWS Signer that match the request parameters. If additional jobs remain
* to be listed, Signer returns a nextToken
value. Use this value in subsequent calls to
* ListSigningJobs
to fetch the remaining values. You can continue calling ListSigningJobs
* with your maxResults
parameter and with new values that Signer returns in the nextToken
* parameter until all of your signing jobs have been returned.
*
*
* @param listSigningPlatformsRequest
* @return A Java Future containing the result of the ListSigningPlatforms operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException You signing certificate could not be validated.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.ListSigningPlatforms
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listSigningPlatforms(
ListSigningPlatformsRequest listSigningPlatformsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listSigningPlatformsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSigningPlatformsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSigningPlatforms");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSigningPlatformsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSigningPlatforms").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListSigningPlatformsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listSigningPlatformsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all available signing profiles in your AWS account. Returns only profiles with an ACTIVE
* status unless the includeCanceled
request field is set to true
. If additional jobs
* remain to be listed, AWS Signer returns a nextToken
value. Use this value in subsequent calls to
* ListSigningJobs
to fetch the remaining values. You can continue calling ListSigningJobs
* with your maxResults
parameter and with new values that Signer returns in the nextToken
* parameter until all of your signing jobs have been returned.
*
*
* @param listSigningProfilesRequest
* @return A Java Future containing the result of the ListSigningProfiles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have sufficient access to perform this action.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.ListSigningProfiles
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listSigningProfiles(
ListSigningProfilesRequest listSigningProfilesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listSigningProfilesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSigningProfilesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSigningProfiles");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSigningProfilesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSigningProfiles").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListSigningProfilesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listSigningProfilesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of the tags associated with a signing profile resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceErrorException An internal error occurred.
* - BadRequestException The request contains invalid parameters for the ARN or tags. This exception also
* occurs when you call a tagging API on a cancelled signing profile.
* - NotFoundException The signing profile was not found.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a signing profile. A signing profile is a code-signing template that can be used to carry out a
* pre-defined signing job.
*
*
* @param putSigningProfileRequest
* @return A Java Future containing the result of the PutSigningProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException A specified resource could not be found.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ValidationException You signing certificate could not be validated.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.PutSigningProfile
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture putSigningProfile(PutSigningProfileRequest putSigningProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putSigningProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putSigningProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutSigningProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutSigningProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutSigningProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new PutSigningProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(putSigningProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes cross-account permissions from a signing profile.
*
*
* @param removeProfilePermissionRequest
* @return A Java Future containing the result of the RemoveProfilePermission operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException You signing certificate could not be validated.
* - ResourceNotFoundException A specified resource could not be found.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException The resource encountered a conflicting state.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.RemoveProfilePermission
* @see AWS API Documentation
*/
@Override
public CompletableFuture removeProfilePermission(
RemoveProfilePermissionRequest removeProfilePermissionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(removeProfilePermissionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, removeProfilePermissionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemoveProfilePermission");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemoveProfilePermissionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveProfilePermission").withProtocolMetadata(protocolMetadata)
.withMarshaller(new RemoveProfilePermissionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(removeProfilePermissionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Changes the state of a signing job to REVOKED. This indicates that the signature is no longer valid.
*
*
* @param revokeSignatureRequest
* @return A Java Future containing the result of the RevokeSignature operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException You signing certificate could not be validated.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException A specified resource could not be found.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.RevokeSignature
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture revokeSignature(RevokeSignatureRequest revokeSignatureRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(revokeSignatureRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, revokeSignatureRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RevokeSignature");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RevokeSignatureResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RevokeSignature").withProtocolMetadata(protocolMetadata)
.withMarshaller(new RevokeSignatureRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(revokeSignatureRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Changes the state of a signing profile to REVOKED. This indicates that signatures generated using the signing
* profile after an effective start date are no longer valid.
*
*
* @param revokeSigningProfileRequest
* @return A Java Future containing the result of the RevokeSigningProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException You signing certificate could not be validated.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException A specified resource could not be found.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.RevokeSigningProfile
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture revokeSigningProfile(
RevokeSigningProfileRequest revokeSigningProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(revokeSigningProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, revokeSigningProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RevokeSigningProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RevokeSigningProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RevokeSigningProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new RevokeSigningProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(revokeSigningProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Signs a binary payload and returns a signature envelope.
*
*
* @param signPayloadRequest
* @return A Java Future containing the result of the SignPayload operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException You signing certificate could not be validated.
* - ResourceNotFoundException A specified resource could not be found.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.SignPayload
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture signPayload(SignPayloadRequest signPayloadRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(signPayloadRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, signPayloadRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SignPayload");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
SignPayloadResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SignPayload").withProtocolMetadata(protocolMetadata)
.withMarshaller(new SignPayloadRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(signPayloadRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Initiates a signing job to be performed on the code provided. Signing jobs are viewable by the
* ListSigningJobs
operation for two years after they are performed. Note the following requirements:
*
*
* -
*
* You must create an Amazon S3 source bucket. For more information, see Creating a Bucket in the Amazon
* S3 Getting Started Guide.
*
*
* -
*
* Your S3 source bucket must be version enabled.
*
*
* -
*
* You must create an S3 destination bucket. AWS Signer uses your S3 destination bucket to write your signed code.
*
*
* -
*
* You specify the name of the source and destination buckets when calling the StartSigningJob
* operation.
*
*
* -
*
* You must ensure the S3 buckets are from the same Region as the signing profile. Cross-Region signing isn't
* supported.
*
*
* -
*
* You must also specify a request token that identifies your request to Signer.
*
*
*
*
* You can call the DescribeSigningJob and the ListSigningJobs actions after you call
* StartSigningJob
.
*
*
* For a Java example that shows how to use this action, see StartSigningJob.
*
*
* @param startSigningJobRequest
* @return A Java Future containing the result of the StartSigningJob operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException You signing certificate could not be validated.
* - ResourceNotFoundException A specified resource could not be found.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
*
* Instead of this error, TooManyRequestsException
should be used.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - InternalServiceErrorException An internal error occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.StartSigningJob
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture startSigningJob(StartSigningJobRequest startSigningJobRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startSigningJobRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startSigningJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartSigningJob");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartSigningJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartSigningJob").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartSigningJobRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startSigningJobRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds one or more tags to a signing profile. Tags are labels that you can use to identify and organize your AWS
* resources. Each tag consists of a key and an optional value. To specify the signing profile, use its Amazon
* Resource Name (ARN). To specify the tag, use a key-value pair.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceErrorException An internal error occurred.
* - BadRequestException The request contains invalid parameters for the ARN or tags. This exception also
* occurs when you call a tagging API on a cancelled signing profile.
* - NotFoundException The signing profile was not found.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(tagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes one or more tags from a signing profile. To remove the tags, specify a list of tag keys.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServiceErrorException An internal error occurred.
* - BadRequestException The request contains invalid parameters for the ARN or tags. This exception also
* occurs when you call a tagging API on a cancelled signing profile.
* - NotFoundException The signing profile was not found.
* - TooManyRequestsException The allowed number of job-signing requests has been exceeded.
*
* This error supersedes the error ThrottlingException
.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SignerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample SignerAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(untagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "signer");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(untagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public SignerAsyncWaiter waiter() {
return SignerAsyncWaiter.builder().client(this).scheduledExecutorService(executorService).build();
}
@Override
public final SignerServiceClientConfiguration serviceClientConfiguration() {
return new SignerServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(SignerException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceLimitExceededException")
.exceptionBuilderSupplier(ServiceLimitExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NotFoundException")
.exceptionBuilderSupplier(NotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServiceErrorException")
.exceptionBuilderSupplier(InternalServiceErrorException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRequestsException")
.exceptionBuilderSupplier(TooManyRequestsException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BadRequestException")
.exceptionBuilderSupplier(BadRequestException::builder).httpStatusCode(400).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
SignerServiceClientConfigurationBuilder serviceConfigBuilder = new SignerServiceClientConfigurationBuilder(configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
@Override
public void close() {
clientHandler.close();
}
}