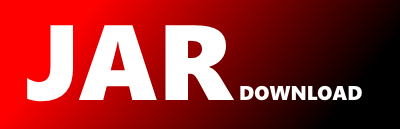
software.amazon.awssdk.services.signer.model.GetRevocationStatusRequest Maven / Gradle / Ivy
Show all versions of signer Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.signer.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetRevocationStatusRequest extends SignerRequest implements
ToCopyableBuilder {
private static final SdkField SIGNATURE_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("signatureTimestamp").getter(getter(GetRevocationStatusRequest::signatureTimestamp))
.setter(setter(Builder::signatureTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("signatureTimestamp").build())
.build();
private static final SdkField PLATFORM_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("platformId").getter(getter(GetRevocationStatusRequest::platformId)).setter(setter(Builder::platformId))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("platformId").build()).build();
private static final SdkField PROFILE_VERSION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("profileVersionArn").getter(getter(GetRevocationStatusRequest::profileVersionArn))
.setter(setter(Builder::profileVersionArn))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("profileVersionArn").build())
.build();
private static final SdkField JOB_ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("jobArn")
.getter(getter(GetRevocationStatusRequest::jobArn)).setter(setter(Builder::jobArn))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("jobArn").build()).build();
private static final SdkField> CERTIFICATE_HASHES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("certificateHashes")
.getter(getter(GetRevocationStatusRequest::certificateHashes))
.setter(setter(Builder::certificateHashes))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("certificateHashes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SIGNATURE_TIMESTAMP_FIELD,
PLATFORM_ID_FIELD, PROFILE_VERSION_ARN_FIELD, JOB_ARN_FIELD, CERTIFICATE_HASHES_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final Instant signatureTimestamp;
private final String platformId;
private final String profileVersionArn;
private final String jobArn;
private final List certificateHashes;
private GetRevocationStatusRequest(BuilderImpl builder) {
super(builder);
this.signatureTimestamp = builder.signatureTimestamp;
this.platformId = builder.platformId;
this.profileVersionArn = builder.profileVersionArn;
this.jobArn = builder.jobArn;
this.certificateHashes = builder.certificateHashes;
}
/**
*
* The timestamp of the signature that validates the profile or job.
*
*
* @return The timestamp of the signature that validates the profile or job.
*/
public final Instant signatureTimestamp() {
return signatureTimestamp;
}
/**
*
* The ID of a signing platform.
*
*
* @return The ID of a signing platform.
*/
public final String platformId() {
return platformId;
}
/**
*
* The version of a signing profile.
*
*
* @return The version of a signing profile.
*/
public final String profileVersionArn() {
return profileVersionArn;
}
/**
*
* The ARN of a signing job.
*
*
* @return The ARN of a signing job.
*/
public final String jobArn() {
return jobArn;
}
/**
* For responses, this returns true if the service returned a value for the CertificateHashes property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCertificateHashes() {
return certificateHashes != null && !(certificateHashes instanceof SdkAutoConstructList);
}
/**
*
* A list of composite signed hashes that identify certificates.
*
*
* A certificate identifier consists of a subject certificate TBS hash (signed by the parent CA) combined with a
* parent CA TBS hash (signed by the parent CA’s CA). Root certificates are defined as their own CA.
*
*
* The following example shows how to calculate a hash for this parameter using OpenSSL commands:
*
*
* openssl asn1parse -in childCert.pem -strparse 4 -out childCert.tbs
*
*
* openssl sha384 < childCert.tbs -binary > childCertTbsHash
*
*
* openssl asn1parse -in parentCert.pem -strparse 4 -out parentCert.tbs
*
*
* openssl sha384 < parentCert.tbs -binary > parentCertTbsHash xxd -p childCertTbsHash > certificateHash.hex xxd -p parentCertTbsHash >> certificateHash.hex
*
*
* cat certificateHash.hex | tr -d '\n'
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCertificateHashes} method.
*
*
* @return A list of composite signed hashes that identify certificates.
*
* A certificate identifier consists of a subject certificate TBS hash (signed by the parent CA) combined
* with a parent CA TBS hash (signed by the parent CA’s CA). Root certificates are defined as their own CA.
*
*
* The following example shows how to calculate a hash for this parameter using OpenSSL commands:
*
*
* openssl asn1parse -in childCert.pem -strparse 4 -out childCert.tbs
*
*
* openssl sha384 < childCert.tbs -binary > childCertTbsHash
*
*
* openssl asn1parse -in parentCert.pem -strparse 4 -out parentCert.tbs
*
*
* openssl sha384 < parentCert.tbs -binary > parentCertTbsHash xxd -p childCertTbsHash > certificateHash.hex xxd -p parentCertTbsHash >> certificateHash.hex
*
*
* cat certificateHash.hex | tr -d '\n'
*/
public final List certificateHashes() {
return certificateHashes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(signatureTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(platformId());
hashCode = 31 * hashCode + Objects.hashCode(profileVersionArn());
hashCode = 31 * hashCode + Objects.hashCode(jobArn());
hashCode = 31 * hashCode + Objects.hashCode(hasCertificateHashes() ? certificateHashes() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetRevocationStatusRequest)) {
return false;
}
GetRevocationStatusRequest other = (GetRevocationStatusRequest) obj;
return Objects.equals(signatureTimestamp(), other.signatureTimestamp())
&& Objects.equals(platformId(), other.platformId())
&& Objects.equals(profileVersionArn(), other.profileVersionArn()) && Objects.equals(jobArn(), other.jobArn())
&& hasCertificateHashes() == other.hasCertificateHashes()
&& Objects.equals(certificateHashes(), other.certificateHashes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetRevocationStatusRequest").add("SignatureTimestamp", signatureTimestamp())
.add("PlatformId", platformId()).add("ProfileVersionArn", profileVersionArn()).add("JobArn", jobArn())
.add("CertificateHashes", hasCertificateHashes() ? certificateHashes() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "signatureTimestamp":
return Optional.ofNullable(clazz.cast(signatureTimestamp()));
case "platformId":
return Optional.ofNullable(clazz.cast(platformId()));
case "profileVersionArn":
return Optional.ofNullable(clazz.cast(profileVersionArn()));
case "jobArn":
return Optional.ofNullable(clazz.cast(jobArn()));
case "certificateHashes":
return Optional.ofNullable(clazz.cast(certificateHashes()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("signatureTimestamp", SIGNATURE_TIMESTAMP_FIELD);
map.put("platformId", PLATFORM_ID_FIELD);
map.put("profileVersionArn", PROFILE_VERSION_ARN_FIELD);
map.put("jobArn", JOB_ARN_FIELD);
map.put("certificateHashes", CERTIFICATE_HASHES_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
*
* A certificate identifier consists of a subject certificate TBS hash (signed by the parent CA) combined
* with a parent CA TBS hash (signed by the parent CA’s CA). Root certificates are defined as their own
* CA.
*
*
* The following example shows how to calculate a hash for this parameter using OpenSSL commands:
*
*
* openssl asn1parse -in childCert.pem -strparse 4 -out childCert.tbs
*
*
* openssl sha384 < childCert.tbs -binary > childCertTbsHash
*
*
* openssl asn1parse -in parentCert.pem -strparse 4 -out parentCert.tbs
*
*
* openssl sha384 < parentCert.tbs -binary > parentCertTbsHash xxd -p childCertTbsHash > certificateHash.hex xxd -p parentCertTbsHash >> certificateHash.hex
*
*
* cat certificateHash.hex | tr -d '\n'
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder certificateHashes(Collection certificateHashes);
/**
*
* A list of composite signed hashes that identify certificates.
*
*
* A certificate identifier consists of a subject certificate TBS hash (signed by the parent CA) combined with a
* parent CA TBS hash (signed by the parent CA’s CA). Root certificates are defined as their own CA.
*
*
* The following example shows how to calculate a hash for this parameter using OpenSSL commands:
*
*
* openssl asn1parse -in childCert.pem -strparse 4 -out childCert.tbs
*
*
* openssl sha384 < childCert.tbs -binary > childCertTbsHash
*
*
* openssl asn1parse -in parentCert.pem -strparse 4 -out parentCert.tbs
*
*
* openssl sha384 < parentCert.tbs -binary > parentCertTbsHash xxd -p childCertTbsHash > certificateHash.hex xxd -p parentCertTbsHash >> certificateHash.hex
*
*
* cat certificateHash.hex | tr -d '\n'
*
*
* @param certificateHashes
* A list of composite signed hashes that identify certificates.
*
* A certificate identifier consists of a subject certificate TBS hash (signed by the parent CA) combined
* with a parent CA TBS hash (signed by the parent CA’s CA). Root certificates are defined as their own
* CA.
*
*
* The following example shows how to calculate a hash for this parameter using OpenSSL commands:
*
*
* openssl asn1parse -in childCert.pem -strparse 4 -out childCert.tbs
*
*
* openssl sha384 < childCert.tbs -binary > childCertTbsHash
*
*
* openssl asn1parse -in parentCert.pem -strparse 4 -out parentCert.tbs
*
*
* openssl sha384 < parentCert.tbs -binary > parentCertTbsHash xxd -p childCertTbsHash > certificateHash.hex xxd -p parentCertTbsHash >> certificateHash.hex
*
*
* cat certificateHash.hex | tr -d '\n'
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder certificateHashes(String... certificateHashes);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends SignerRequest.BuilderImpl implements Builder {
private Instant signatureTimestamp;
private String platformId;
private String profileVersionArn;
private String jobArn;
private List certificateHashes = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(GetRevocationStatusRequest model) {
super(model);
signatureTimestamp(model.signatureTimestamp);
platformId(model.platformId);
profileVersionArn(model.profileVersionArn);
jobArn(model.jobArn);
certificateHashes(model.certificateHashes);
}
public final Instant getSignatureTimestamp() {
return signatureTimestamp;
}
public final void setSignatureTimestamp(Instant signatureTimestamp) {
this.signatureTimestamp = signatureTimestamp;
}
@Override
public final Builder signatureTimestamp(Instant signatureTimestamp) {
this.signatureTimestamp = signatureTimestamp;
return this;
}
public final String getPlatformId() {
return platformId;
}
public final void setPlatformId(String platformId) {
this.platformId = platformId;
}
@Override
public final Builder platformId(String platformId) {
this.platformId = platformId;
return this;
}
public final String getProfileVersionArn() {
return profileVersionArn;
}
public final void setProfileVersionArn(String profileVersionArn) {
this.profileVersionArn = profileVersionArn;
}
@Override
public final Builder profileVersionArn(String profileVersionArn) {
this.profileVersionArn = profileVersionArn;
return this;
}
public final String getJobArn() {
return jobArn;
}
public final void setJobArn(String jobArn) {
this.jobArn = jobArn;
}
@Override
public final Builder jobArn(String jobArn) {
this.jobArn = jobArn;
return this;
}
public final Collection getCertificateHashes() {
if (certificateHashes instanceof SdkAutoConstructList) {
return null;
}
return certificateHashes;
}
public final void setCertificateHashes(Collection certificateHashes) {
this.certificateHashes = CertificateHashesCopier.copy(certificateHashes);
}
@Override
public final Builder certificateHashes(Collection certificateHashes) {
this.certificateHashes = CertificateHashesCopier.copy(certificateHashes);
return this;
}
@Override
@SafeVarargs
public final Builder certificateHashes(String... certificateHashes) {
certificateHashes(Arrays.asList(certificateHashes));
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public GetRevocationStatusRequest build() {
return new GetRevocationStatusRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}