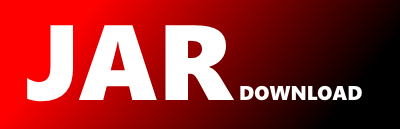
software.amazon.awssdk.services.sms.DefaultSmsClient Maven / Gradle / Ivy
Show all versions of sms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sms;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.sms.model.CreateAppRequest;
import software.amazon.awssdk.services.sms.model.CreateAppResponse;
import software.amazon.awssdk.services.sms.model.CreateReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.CreateReplicationJobResponse;
import software.amazon.awssdk.services.sms.model.DeleteAppLaunchConfigurationRequest;
import software.amazon.awssdk.services.sms.model.DeleteAppLaunchConfigurationResponse;
import software.amazon.awssdk.services.sms.model.DeleteAppReplicationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.DeleteAppReplicationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.DeleteAppRequest;
import software.amazon.awssdk.services.sms.model.DeleteAppResponse;
import software.amazon.awssdk.services.sms.model.DeleteAppValidationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.DeleteAppValidationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.DeleteReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.DeleteReplicationJobResponse;
import software.amazon.awssdk.services.sms.model.DeleteServerCatalogRequest;
import software.amazon.awssdk.services.sms.model.DeleteServerCatalogResponse;
import software.amazon.awssdk.services.sms.model.DisassociateConnectorRequest;
import software.amazon.awssdk.services.sms.model.DisassociateConnectorResponse;
import software.amazon.awssdk.services.sms.model.DryRunOperationException;
import software.amazon.awssdk.services.sms.model.GenerateChangeSetRequest;
import software.amazon.awssdk.services.sms.model.GenerateChangeSetResponse;
import software.amazon.awssdk.services.sms.model.GenerateTemplateRequest;
import software.amazon.awssdk.services.sms.model.GenerateTemplateResponse;
import software.amazon.awssdk.services.sms.model.GetAppLaunchConfigurationRequest;
import software.amazon.awssdk.services.sms.model.GetAppLaunchConfigurationResponse;
import software.amazon.awssdk.services.sms.model.GetAppReplicationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.GetAppReplicationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.GetAppRequest;
import software.amazon.awssdk.services.sms.model.GetAppResponse;
import software.amazon.awssdk.services.sms.model.GetAppValidationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.GetAppValidationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.GetAppValidationOutputRequest;
import software.amazon.awssdk.services.sms.model.GetAppValidationOutputResponse;
import software.amazon.awssdk.services.sms.model.GetConnectorsRequest;
import software.amazon.awssdk.services.sms.model.GetConnectorsResponse;
import software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest;
import software.amazon.awssdk.services.sms.model.GetReplicationJobsResponse;
import software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest;
import software.amazon.awssdk.services.sms.model.GetReplicationRunsResponse;
import software.amazon.awssdk.services.sms.model.GetServersRequest;
import software.amazon.awssdk.services.sms.model.GetServersResponse;
import software.amazon.awssdk.services.sms.model.ImportAppCatalogRequest;
import software.amazon.awssdk.services.sms.model.ImportAppCatalogResponse;
import software.amazon.awssdk.services.sms.model.ImportServerCatalogRequest;
import software.amazon.awssdk.services.sms.model.ImportServerCatalogResponse;
import software.amazon.awssdk.services.sms.model.InternalErrorException;
import software.amazon.awssdk.services.sms.model.InvalidParameterException;
import software.amazon.awssdk.services.sms.model.LaunchAppRequest;
import software.amazon.awssdk.services.sms.model.LaunchAppResponse;
import software.amazon.awssdk.services.sms.model.ListAppsRequest;
import software.amazon.awssdk.services.sms.model.ListAppsResponse;
import software.amazon.awssdk.services.sms.model.MissingRequiredParameterException;
import software.amazon.awssdk.services.sms.model.NoConnectorsAvailableException;
import software.amazon.awssdk.services.sms.model.NotifyAppValidationOutputRequest;
import software.amazon.awssdk.services.sms.model.NotifyAppValidationOutputResponse;
import software.amazon.awssdk.services.sms.model.OperationNotPermittedException;
import software.amazon.awssdk.services.sms.model.PutAppLaunchConfigurationRequest;
import software.amazon.awssdk.services.sms.model.PutAppLaunchConfigurationResponse;
import software.amazon.awssdk.services.sms.model.PutAppReplicationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.PutAppReplicationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.PutAppValidationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.PutAppValidationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.ReplicationJobAlreadyExistsException;
import software.amazon.awssdk.services.sms.model.ReplicationJobNotFoundException;
import software.amazon.awssdk.services.sms.model.ReplicationRunLimitExceededException;
import software.amazon.awssdk.services.sms.model.ServerCannotBeReplicatedException;
import software.amazon.awssdk.services.sms.model.SmsException;
import software.amazon.awssdk.services.sms.model.SmsRequest;
import software.amazon.awssdk.services.sms.model.StartAppReplicationRequest;
import software.amazon.awssdk.services.sms.model.StartAppReplicationResponse;
import software.amazon.awssdk.services.sms.model.StartOnDemandAppReplicationRequest;
import software.amazon.awssdk.services.sms.model.StartOnDemandAppReplicationResponse;
import software.amazon.awssdk.services.sms.model.StartOnDemandReplicationRunRequest;
import software.amazon.awssdk.services.sms.model.StartOnDemandReplicationRunResponse;
import software.amazon.awssdk.services.sms.model.StopAppReplicationRequest;
import software.amazon.awssdk.services.sms.model.StopAppReplicationResponse;
import software.amazon.awssdk.services.sms.model.TemporarilyUnavailableException;
import software.amazon.awssdk.services.sms.model.TerminateAppRequest;
import software.amazon.awssdk.services.sms.model.TerminateAppResponse;
import software.amazon.awssdk.services.sms.model.UnauthorizedOperationException;
import software.amazon.awssdk.services.sms.model.UpdateAppRequest;
import software.amazon.awssdk.services.sms.model.UpdateAppResponse;
import software.amazon.awssdk.services.sms.model.UpdateReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.UpdateReplicationJobResponse;
import software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable;
import software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable;
import software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable;
import software.amazon.awssdk.services.sms.paginators.GetServersIterable;
import software.amazon.awssdk.services.sms.transform.CreateAppRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.CreateReplicationJobRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.DeleteAppLaunchConfigurationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.DeleteAppReplicationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.DeleteAppRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.DeleteAppValidationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.DeleteReplicationJobRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.DeleteServerCatalogRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.DisassociateConnectorRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GenerateChangeSetRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GenerateTemplateRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetAppLaunchConfigurationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetAppReplicationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetAppRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetAppValidationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetAppValidationOutputRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetConnectorsRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetReplicationJobsRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetReplicationRunsRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.GetServersRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.ImportAppCatalogRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.ImportServerCatalogRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.LaunchAppRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.ListAppsRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.NotifyAppValidationOutputRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.PutAppLaunchConfigurationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.PutAppReplicationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.PutAppValidationConfigurationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.StartAppReplicationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.StartOnDemandAppReplicationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.StartOnDemandReplicationRunRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.StopAppReplicationRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.TerminateAppRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.UpdateAppRequestMarshaller;
import software.amazon.awssdk.services.sms.transform.UpdateReplicationJobRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link SmsClient}.
*
* @see SmsClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultSmsClient implements SmsClient {
private static final Logger log = Logger.loggerFor(DefaultSmsClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultSmsClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Creates an application. An application consists of one or more server groups. Each server group contain one or
* more servers.
*
*
* @param createAppRequest
* @return Result of the CreateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.CreateApp
* @see AWS API
* Documentation
*/
@Override
public CreateAppResponse createApp(CreateAppRequest createAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateApp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateApp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createAppRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new CreateAppRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a replication job. The replication job schedules periodic replication runs to replicate your server to
* AWS. Each replication run creates an Amazon Machine Image (AMI).
*
*
* @param createReplicationJobRequest
* @return Result of the CreateReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ServerCannotBeReplicatedException
* The specified server cannot be replicated.
* @throws ReplicationJobAlreadyExistsException
* The specified replication job already exists.
* @throws NoConnectorsAvailableException
* There are no connectors available.
* @throws InternalErrorException
* An internal error occurred.
* @throws TemporarilyUnavailableException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.CreateReplicationJob
* @see AWS API
* Documentation
*/
@Override
public CreateReplicationJobResponse createReplicationJob(CreateReplicationJobRequest createReplicationJobRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
OperationNotPermittedException, ServerCannotBeReplicatedException, ReplicationJobAlreadyExistsException,
NoConnectorsAvailableException, InternalErrorException, TemporarilyUnavailableException, AwsServiceException,
SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateReplicationJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createReplicationJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateReplicationJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateReplicationJob").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createReplicationJobRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateReplicationJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified application. Optionally deletes the launched stack associated with the application and all
* AWS SMS replication jobs for servers in the application.
*
*
* @param deleteAppRequest
* @return Result of the DeleteApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteApp
* @see AWS API
* Documentation
*/
@Override
public DeleteAppResponse deleteApp(DeleteAppRequest deleteAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteApp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteAppRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new DeleteAppRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the launch configuration for the specified application.
*
*
* @param deleteAppLaunchConfigurationRequest
* @return Result of the DeleteAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteAppLaunchConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteAppLaunchConfigurationResponse deleteAppLaunchConfiguration(
DeleteAppLaunchConfigurationRequest deleteAppLaunchConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAppLaunchConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAppLaunchConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAppLaunchConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAppLaunchConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteAppLaunchConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteAppLaunchConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the replication configuration for the specified application.
*
*
* @param deleteAppReplicationConfigurationRequest
* @return Result of the DeleteAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteAppReplicationConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteAppReplicationConfigurationResponse deleteAppReplicationConfiguration(
DeleteAppReplicationConfigurationRequest deleteAppReplicationConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAppReplicationConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteAppReplicationConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAppReplicationConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAppReplicationConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteAppReplicationConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteAppReplicationConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the validation configuration for the specified application.
*
*
* @param deleteAppValidationConfigurationRequest
* @return Result of the DeleteAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteAppValidationConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteAppValidationConfigurationResponse deleteAppValidationConfiguration(
DeleteAppValidationConfigurationRequest deleteAppValidationConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteAppValidationConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
deleteAppValidationConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteAppValidationConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteAppValidationConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteAppValidationConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteAppValidationConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes the specified replication job.
*
*
* After you delete a replication job, there are no further replication runs. AWS deletes the contents of the Amazon
* S3 bucket used to store AWS SMS artifacts. The AMIs created by the replication runs are not deleted.
*
*
* @param deleteReplicationJobRequest
* @return Result of the DeleteReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ReplicationJobNotFoundException
* The specified replication job does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteReplicationJob
* @see AWS API
* Documentation
*/
@Override
public DeleteReplicationJobResponse deleteReplicationJob(DeleteReplicationJobRequest deleteReplicationJobRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
OperationNotPermittedException, ReplicationJobNotFoundException, AwsServiceException, SdkClientException,
SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteReplicationJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteReplicationJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteReplicationJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteReplicationJob").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteReplicationJobRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteReplicationJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes all servers from your server catalog.
*
*
* @param deleteServerCatalogRequest
* @return Result of the DeleteServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteServerCatalog
* @see AWS API
* Documentation
*/
@Override
public DeleteServerCatalogResponse deleteServerCatalog(DeleteServerCatalogRequest deleteServerCatalogRequest)
throws UnauthorizedOperationException, OperationNotPermittedException, InvalidParameterException,
MissingRequiredParameterException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteServerCatalogResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteServerCatalogRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteServerCatalog");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteServerCatalog").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteServerCatalogRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteServerCatalogRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociates the specified connector from AWS SMS.
*
*
* After you disassociate a connector, it is no longer available to support replication jobs.
*
*
* @param disassociateConnectorRequest
* @return Result of the DisassociateConnector operation returned by the service.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DisassociateConnector
* @see AWS API
* Documentation
*/
@Override
public DisassociateConnectorResponse disassociateConnector(DisassociateConnectorRequest disassociateConnectorRequest)
throws MissingRequiredParameterException, UnauthorizedOperationException, OperationNotPermittedException,
InvalidParameterException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateConnectorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateConnectorRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateConnector");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DisassociateConnector").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateConnectorRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateConnectorRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Generates a target change set for a currently launched stack and writes it to an Amazon S3 object in the
* customer’s Amazon S3 bucket.
*
*
* @param generateChangeSetRequest
* @return Result of the GenerateChangeSet operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GenerateChangeSet
* @see AWS API
* Documentation
*/
@Override
public GenerateChangeSetResponse generateChangeSet(GenerateChangeSetRequest generateChangeSetRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GenerateChangeSetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, generateChangeSetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GenerateChangeSet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GenerateChangeSet").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(generateChangeSetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GenerateChangeSetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Generates an AWS CloudFormation template based on the current launch configuration and writes it to an Amazon S3
* object in the customer’s Amazon S3 bucket.
*
*
* @param generateTemplateRequest
* @return Result of the GenerateTemplate operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GenerateTemplate
* @see AWS API
* Documentation
*/
@Override
public GenerateTemplateResponse generateTemplate(GenerateTemplateRequest generateTemplateRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GenerateTemplateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, generateTemplateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GenerateTemplate");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GenerateTemplate").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(generateTemplateRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GenerateTemplateRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieve information about the specified application.
*
*
* @param getAppRequest
* @return Result of the GetApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetApp
* @see AWS API
* Documentation
*/
@Override
public GetAppResponse getApp(GetAppRequest getAppRequest) throws UnauthorizedOperationException, InvalidParameterException,
MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException, AwsServiceException,
SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetApp");
return clientHandler.execute(new ClientExecutionParams().withOperationName("GetApp")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler).withInput(getAppRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new GetAppRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the application launch configuration associated with the specified application.
*
*
* @param getAppLaunchConfigurationRequest
* @return Result of the GetAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppLaunchConfiguration
* @see AWS
* API Documentation
*/
@Override
public GetAppLaunchConfigurationResponse getAppLaunchConfiguration(
GetAppLaunchConfigurationRequest getAppLaunchConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAppLaunchConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAppLaunchConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAppLaunchConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAppLaunchConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getAppLaunchConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetAppLaunchConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the application replication configuration associated with the specified application.
*
*
* @param getAppReplicationConfigurationRequest
* @return Result of the GetAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppReplicationConfiguration
* @see AWS API Documentation
*/
@Override
public GetAppReplicationConfigurationResponse getAppReplicationConfiguration(
GetAppReplicationConfigurationRequest getAppReplicationConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAppReplicationConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getAppReplicationConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAppReplicationConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAppReplicationConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getAppReplicationConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetAppReplicationConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves information about a configuration for validating an application.
*
*
* @param getAppValidationConfigurationRequest
* @return Result of the GetAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppValidationConfiguration
* @see AWS API Documentation
*/
@Override
public GetAppValidationConfigurationResponse getAppValidationConfiguration(
GetAppValidationConfigurationRequest getAppValidationConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAppValidationConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getAppValidationConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAppValidationConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAppValidationConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getAppValidationConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetAppValidationConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves output from validating an application.
*
*
* @param getAppValidationOutputRequest
* @return Result of the GetAppValidationOutput operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppValidationOutput
* @see AWS API
* Documentation
*/
@Override
public GetAppValidationOutputResponse getAppValidationOutput(GetAppValidationOutputRequest getAppValidationOutputRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAppValidationOutputResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAppValidationOutputRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAppValidationOutput");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAppValidationOutput").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getAppValidationOutputRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetAppValidationOutputRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the connectors registered with the AWS SMS.
*
*
* @param getConnectorsRequest
* @return Result of the GetConnectors operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetConnectors
* @see AWS API
* Documentation
*/
@Override
public GetConnectorsResponse getConnectors(GetConnectorsRequest getConnectorsRequest) throws UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetConnectorsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getConnectorsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetConnectors");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetConnectors").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getConnectorsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetConnectorsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the connectors registered with the AWS SMS.
*
*
*
* This is a variant of {@link #getConnectors(software.amazon.awssdk.services.sms.model.GetConnectorsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetConnectorsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getConnectors(software.amazon.awssdk.services.sms.model.GetConnectorsRequest)} operation.
*
*
* @param getConnectorsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetConnectors
* @see AWS API
* Documentation
*/
@Override
public GetConnectorsIterable getConnectorsPaginator(GetConnectorsRequest getConnectorsRequest)
throws UnauthorizedOperationException, AwsServiceException, SdkClientException, SmsException {
return new GetConnectorsIterable(this, applyPaginatorUserAgent(getConnectorsRequest));
}
/**
*
* Describes the specified replication job or all of your replication jobs.
*
*
* @param getReplicationJobsRequest
* @return Result of the GetReplicationJobs operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationJobs
* @see AWS API
* Documentation
*/
@Override
public GetReplicationJobsResponse getReplicationJobs(GetReplicationJobsRequest getReplicationJobsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetReplicationJobsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getReplicationJobsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetReplicationJobs");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetReplicationJobs").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getReplicationJobsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetReplicationJobsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the specified replication job or all of your replication jobs.
*
*
*
* This is a variant of
* {@link #getReplicationJobs(software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client.getReplicationJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client
* .getReplicationJobsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetReplicationJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client.getReplicationJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getReplicationJobs(software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest)} operation.
*
*
* @param getReplicationJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationJobs
* @see AWS API
* Documentation
*/
@Override
public GetReplicationJobsIterable getReplicationJobsPaginator(GetReplicationJobsRequest getReplicationJobsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
return new GetReplicationJobsIterable(this, applyPaginatorUserAgent(getReplicationJobsRequest));
}
/**
*
* Describes the replication runs for the specified replication job.
*
*
* @param getReplicationRunsRequest
* @return Result of the GetReplicationRuns operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationRuns
* @see AWS API
* Documentation
*/
@Override
public GetReplicationRunsResponse getReplicationRuns(GetReplicationRunsRequest getReplicationRunsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetReplicationRunsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getReplicationRunsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetReplicationRuns");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetReplicationRuns").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getReplicationRunsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetReplicationRunsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the replication runs for the specified replication job.
*
*
*
* This is a variant of
* {@link #getReplicationRuns(software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable responses = client.getReplicationRunsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable responses = client
* .getReplicationRunsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetReplicationRunsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable responses = client.getReplicationRunsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getReplicationRuns(software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest)} operation.
*
*
* @param getReplicationRunsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationRuns
* @see AWS API
* Documentation
*/
@Override
public GetReplicationRunsIterable getReplicationRunsPaginator(GetReplicationRunsRequest getReplicationRunsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
return new GetReplicationRunsIterable(this, applyPaginatorUserAgent(getReplicationRunsRequest));
}
/**
*
* Describes the servers in your server catalog.
*
*
* Before you can describe your servers, you must import them using ImportServerCatalog.
*
*
* @param getServersRequest
* @return Result of the GetServers operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetServers
* @see AWS API
* Documentation
*/
@Override
public GetServersResponse getServers(GetServersRequest getServersRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, AwsServiceException,
SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetServersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getServersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetServers");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("GetServers")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getServersRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetServersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the servers in your server catalog.
*
*
* Before you can describe your servers, you must import them using ImportServerCatalog.
*
*
*
* This is a variant of {@link #getServers(software.amazon.awssdk.services.sms.model.GetServersRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetServersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getServers(software.amazon.awssdk.services.sms.model.GetServersRequest)} operation.
*
*
* @param getServersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetServers
* @see AWS API
* Documentation
*/
@Override
public GetServersIterable getServersPaginator(GetServersRequest getServersRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, AwsServiceException,
SdkClientException, SmsException {
return new GetServersIterable(this, applyPaginatorUserAgent(getServersRequest));
}
/**
*
* Allows application import from AWS Migration Hub.
*
*
* @param importAppCatalogRequest
* @return Result of the ImportAppCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ImportAppCatalog
* @see AWS API
* Documentation
*/
@Override
public ImportAppCatalogResponse importAppCatalog(ImportAppCatalogRequest importAppCatalogRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ImportAppCatalogResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, importAppCatalogRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ImportAppCatalog");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ImportAppCatalog").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(importAppCatalogRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ImportAppCatalogRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gathers a complete list of on-premises servers. Connectors must be installed and monitoring all servers to
* import.
*
*
* This call returns immediately, but might take additional time to retrieve all the servers.
*
*
* @param importServerCatalogRequest
* @return Result of the ImportServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws NoConnectorsAvailableException
* There are no connectors available.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ImportServerCatalog
* @see AWS API
* Documentation
*/
@Override
public ImportServerCatalogResponse importServerCatalog(ImportServerCatalogRequest importServerCatalogRequest)
throws UnauthorizedOperationException, OperationNotPermittedException, InvalidParameterException,
MissingRequiredParameterException, NoConnectorsAvailableException, AwsServiceException, SdkClientException,
SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ImportServerCatalogResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, importServerCatalogRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ImportServerCatalog");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ImportServerCatalog").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(importServerCatalogRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ImportServerCatalogRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Launches the specified application as a stack in AWS CloudFormation.
*
*
* @param launchAppRequest
* @return Result of the LaunchApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.LaunchApp
* @see AWS API
* Documentation
*/
@Override
public LaunchAppResponse launchApp(LaunchAppRequest launchAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
LaunchAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, launchAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "LaunchApp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("LaunchApp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(launchAppRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new LaunchAppRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves summaries for all applications.
*
*
* @param listAppsRequest
* @return Result of the ListApps operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ListApps
* @see AWS API
* Documentation
*/
@Override
public ListAppsResponse listApps(ListAppsRequest listAppsRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListAppsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAppsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListApps");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListApps").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listAppsRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new ListAppsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides information to AWS SMS about whether application validation is successful.
*
*
* @param notifyAppValidationOutputRequest
* @return Result of the NotifyAppValidationOutput operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.NotifyAppValidationOutput
* @see AWS
* API Documentation
*/
@Override
public NotifyAppValidationOutputResponse notifyAppValidationOutput(
NotifyAppValidationOutputRequest notifyAppValidationOutputRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, NotifyAppValidationOutputResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, notifyAppValidationOutputRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "NotifyAppValidationOutput");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("NotifyAppValidationOutput").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(notifyAppValidationOutputRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new NotifyAppValidationOutputRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates the launch configuration for the specified application.
*
*
* @param putAppLaunchConfigurationRequest
* @return Result of the PutAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.PutAppLaunchConfiguration
* @see AWS
* API Documentation
*/
@Override
public PutAppLaunchConfigurationResponse putAppLaunchConfiguration(
PutAppLaunchConfigurationRequest putAppLaunchConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAppLaunchConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putAppLaunchConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAppLaunchConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAppLaunchConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putAppLaunchConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutAppLaunchConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates the replication configuration for the specified application.
*
*
* @param putAppReplicationConfigurationRequest
* @return Result of the PutAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.PutAppReplicationConfiguration
* @see AWS API Documentation
*/
@Override
public PutAppReplicationConfigurationResponse putAppReplicationConfiguration(
PutAppReplicationConfigurationRequest putAppReplicationConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAppReplicationConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putAppReplicationConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAppReplicationConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAppReplicationConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putAppReplicationConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutAppReplicationConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates a validation configuration for the specified application.
*
*
* @param putAppValidationConfigurationRequest
* @return Result of the PutAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.PutAppValidationConfiguration
* @see AWS API Documentation
*/
@Override
public PutAppValidationConfigurationResponse putAppValidationConfiguration(
PutAppValidationConfigurationRequest putAppValidationConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutAppValidationConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
putAppValidationConfigurationRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutAppValidationConfiguration");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("PutAppValidationConfiguration").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putAppValidationConfigurationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutAppValidationConfigurationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Starts replicating the specified application by creating replication jobs for each server in the application.
*
*
* @param startAppReplicationRequest
* @return Result of the StartAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StartAppReplication
* @see AWS API
* Documentation
*/
@Override
public StartAppReplicationResponse startAppReplication(StartAppReplicationRequest startAppReplicationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartAppReplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startAppReplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartAppReplication");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StartAppReplication").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(startAppReplicationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartAppReplicationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Starts an on-demand replication run for the specified application.
*
*
* @param startOnDemandAppReplicationRequest
* @return Result of the StartOnDemandAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StartOnDemandAppReplication
* @see AWS API Documentation
*/
@Override
public StartOnDemandAppReplicationResponse startOnDemandAppReplication(
StartOnDemandAppReplicationRequest startOnDemandAppReplicationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartOnDemandAppReplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startOnDemandAppReplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartOnDemandAppReplication");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartOnDemandAppReplication").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(startOnDemandAppReplicationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartOnDemandAppReplicationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Starts an on-demand replication run for the specified replication job. This replication run starts immediately.
* This replication run is in addition to the ones already scheduled.
*
*
* There is a limit on the number of on-demand replications runs that you can request in a 24-hour period.
*
*
* @param startOnDemandReplicationRunRequest
* @return Result of the StartOnDemandReplicationRun operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ReplicationRunLimitExceededException
* You have exceeded the number of on-demand replication runs you can request in a 24-hour period.
* @throws DryRunOperationException
* The user has the required permissions, so the request would have succeeded, but a dry run was performed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StartOnDemandReplicationRun
* @see AWS API Documentation
*/
@Override
public StartOnDemandReplicationRunResponse startOnDemandReplicationRun(
StartOnDemandReplicationRunRequest startOnDemandReplicationRunRequest) throws InvalidParameterException,
MissingRequiredParameterException, UnauthorizedOperationException, OperationNotPermittedException,
ReplicationRunLimitExceededException, DryRunOperationException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartOnDemandReplicationRunResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startOnDemandReplicationRunRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartOnDemandReplicationRun");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartOnDemandReplicationRun").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(startOnDemandReplicationRunRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartOnDemandReplicationRunRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Stops replicating the specified application by deleting the replication job for each server in the application.
*
*
* @param stopAppReplicationRequest
* @return Result of the StopAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StopAppReplication
* @see AWS API
* Documentation
*/
@Override
public StopAppReplicationResponse stopAppReplication(StopAppReplicationRequest stopAppReplicationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StopAppReplicationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, stopAppReplicationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StopAppReplication");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StopAppReplication").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(stopAppReplicationRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StopAppReplicationRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Terminates the stack for the specified application.
*
*
* @param terminateAppRequest
* @return Result of the TerminateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.TerminateApp
* @see AWS API
* Documentation
*/
@Override
public TerminateAppResponse terminateApp(TerminateAppRequest terminateAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TerminateAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, terminateAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TerminateApp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TerminateApp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(terminateAppRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TerminateAppRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the specified application.
*
*
* @param updateAppRequest
* @return Result of the UpdateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.UpdateApp
* @see AWS API
* Documentation
*/
@Override
public UpdateAppResponse updateApp(UpdateAppRequest updateAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateApp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateApp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateAppRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new UpdateAppRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the specified settings for the specified replication job.
*
*
* @param updateReplicationJobRequest
* @return Result of the UpdateReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws ServerCannotBeReplicatedException
* The specified server cannot be replicated.
* @throws ReplicationJobNotFoundException
* The specified replication job does not exist.
* @throws InternalErrorException
* An internal error occurred.
* @throws TemporarilyUnavailableException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.UpdateReplicationJob
* @see AWS API
* Documentation
*/
@Override
public UpdateReplicationJobResponse updateReplicationJob(UpdateReplicationJobRequest updateReplicationJobRequest)
throws InvalidParameterException, MissingRequiredParameterException, OperationNotPermittedException,
UnauthorizedOperationException, ServerCannotBeReplicatedException, ReplicationJobNotFoundException,
InternalErrorException, TemporarilyUnavailableException, AwsServiceException, SdkClientException, SmsException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateReplicationJobResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateReplicationJobRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SMS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateReplicationJob");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateReplicationJob").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateReplicationJobRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateReplicationJobRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(SmsException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidParameterException")
.exceptionBuilderSupplier(InvalidParameterException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TemporarilyUnavailableException")
.exceptionBuilderSupplier(TemporarilyUnavailableException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("MissingRequiredParameterException")
.exceptionBuilderSupplier(MissingRequiredParameterException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ReplicationJobNotFoundException")
.exceptionBuilderSupplier(ReplicationJobNotFoundException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("OperationNotPermittedException")
.exceptionBuilderSupplier(OperationNotPermittedException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalError")
.exceptionBuilderSupplier(InternalErrorException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServerCannotBeReplicatedException")
.exceptionBuilderSupplier(ServerCannotBeReplicatedException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ReplicationRunLimitExceededException")
.exceptionBuilderSupplier(ReplicationRunLimitExceededException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnauthorizedOperationException")
.exceptionBuilderSupplier(UnauthorizedOperationException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ReplicationJobAlreadyExistsException")
.exceptionBuilderSupplier(ReplicationJobAlreadyExistsException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("NoConnectorsAvailableException")
.exceptionBuilderSupplier(NoConnectorsAvailableException::builder).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DryRunOperationException")
.exceptionBuilderSupplier(DryRunOperationException::builder).build());
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
}