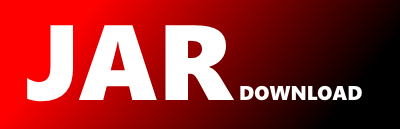
software.amazon.awssdk.services.sms.model.UpdateReplicationJobRequest Maven / Gradle / Ivy
Show all versions of sms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sms.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateReplicationJobRequest extends SmsRequest implements
ToCopyableBuilder {
private static final SdkField REPLICATION_JOB_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("replicationJobId").getter(getter(UpdateReplicationJobRequest::replicationJobId))
.setter(setter(Builder::replicationJobId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("replicationJobId").build()).build();
private static final SdkField FREQUENCY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("frequency").getter(getter(UpdateReplicationJobRequest::frequency)).setter(setter(Builder::frequency))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("frequency").build()).build();
private static final SdkField NEXT_REPLICATION_RUN_START_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("nextReplicationRunStartTime")
.getter(getter(UpdateReplicationJobRequest::nextReplicationRunStartTime))
.setter(setter(Builder::nextReplicationRunStartTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("nextReplicationRunStartTime")
.build()).build();
private static final SdkField LICENSE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("licenseType").getter(getter(UpdateReplicationJobRequest::licenseTypeAsString))
.setter(setter(Builder::licenseType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("licenseType").build()).build();
private static final SdkField ROLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("roleName").getter(getter(UpdateReplicationJobRequest::roleName)).setter(setter(Builder::roleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("roleName").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("description").getter(getter(UpdateReplicationJobRequest::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField NUMBER_OF_RECENT_AMIS_TO_KEEP_FIELD = SdkField
. builder(MarshallingType.INTEGER).memberName("numberOfRecentAmisToKeep")
.getter(getter(UpdateReplicationJobRequest::numberOfRecentAmisToKeep))
.setter(setter(Builder::numberOfRecentAmisToKeep))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("numberOfRecentAmisToKeep").build())
.build();
private static final SdkField ENCRYPTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("encrypted").getter(getter(UpdateReplicationJobRequest::encrypted)).setter(setter(Builder::encrypted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("encrypted").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("kmsKeyId").getter(getter(UpdateReplicationJobRequest::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kmsKeyId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(REPLICATION_JOB_ID_FIELD,
FREQUENCY_FIELD, NEXT_REPLICATION_RUN_START_TIME_FIELD, LICENSE_TYPE_FIELD, ROLE_NAME_FIELD, DESCRIPTION_FIELD,
NUMBER_OF_RECENT_AMIS_TO_KEEP_FIELD, ENCRYPTED_FIELD, KMS_KEY_ID_FIELD));
private final String replicationJobId;
private final Integer frequency;
private final Instant nextReplicationRunStartTime;
private final String licenseType;
private final String roleName;
private final String description;
private final Integer numberOfRecentAmisToKeep;
private final Boolean encrypted;
private final String kmsKeyId;
private UpdateReplicationJobRequest(BuilderImpl builder) {
super(builder);
this.replicationJobId = builder.replicationJobId;
this.frequency = builder.frequency;
this.nextReplicationRunStartTime = builder.nextReplicationRunStartTime;
this.licenseType = builder.licenseType;
this.roleName = builder.roleName;
this.description = builder.description;
this.numberOfRecentAmisToKeep = builder.numberOfRecentAmisToKeep;
this.encrypted = builder.encrypted;
this.kmsKeyId = builder.kmsKeyId;
}
/**
*
* The ID of the replication job.
*
*
* @return The ID of the replication job.
*/
public final String replicationJobId() {
return replicationJobId;
}
/**
*
* The time between consecutive replication runs, in hours.
*
*
* @return The time between consecutive replication runs, in hours.
*/
public final Integer frequency() {
return frequency;
}
/**
*
* The start time of the next replication run.
*
*
* @return The start time of the next replication run.
*/
public final Instant nextReplicationRunStartTime() {
return nextReplicationRunStartTime;
}
/**
*
* The license type to be used for the AMI created by a successful replication run.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #licenseType} will
* return {@link LicenseType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #licenseTypeAsString}.
*
*
* @return The license type to be used for the AMI created by a successful replication run.
* @see LicenseType
*/
public final LicenseType licenseType() {
return LicenseType.fromValue(licenseType);
}
/**
*
* The license type to be used for the AMI created by a successful replication run.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #licenseType} will
* return {@link LicenseType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #licenseTypeAsString}.
*
*
* @return The license type to be used for the AMI created by a successful replication run.
* @see LicenseType
*/
public final String licenseTypeAsString() {
return licenseType;
}
/**
*
* The name of the IAM role to be used by Server Migration Service.
*
*
* @return The name of the IAM role to be used by Server Migration Service.
*/
public final String roleName() {
return roleName;
}
/**
*
* The description of the replication job.
*
*
* @return The description of the replication job.
*/
public final String description() {
return description;
}
/**
*
* The maximum number of SMS-created AMIs to retain. The oldest is deleted after the maximum number is reached and a
* new AMI is created.
*
*
* @return The maximum number of SMS-created AMIs to retain. The oldest is deleted after the maximum number is
* reached and a new AMI is created.
*/
public final Integer numberOfRecentAmisToKeep() {
return numberOfRecentAmisToKeep;
}
/**
*
* When true, the replication job produces encrypted AMIs. For more information, KmsKeyId
.
*
*
* @return When true, the replication job produces encrypted AMIs. For more information, KmsKeyId
.
*/
public final Boolean encrypted() {
return encrypted;
}
/**
*
* The ID of the KMS key for replication jobs that produce encrypted AMIs. This value can be any of the following:
*
*
* -
*
* KMS key ID
*
*
* -
*
* KMS key alias
*
*
* -
*
* ARN referring to the KMS key ID
*
*
* -
*
* ARN referring to the KMS key alias
*
*
*
*
* If encrypted is enabled but a KMS key ID is not specified, the customer's default KMS key for Amazon EBS is used.
*
*
* @return The ID of the KMS key for replication jobs that produce encrypted AMIs. This value can be any of the
* following:
*
* -
*
* KMS key ID
*
*
* -
*
* KMS key alias
*
*
* -
*
* ARN referring to the KMS key ID
*
*
* -
*
* ARN referring to the KMS key alias
*
*
*
*
* If encrypted is enabled but a KMS key ID is not specified, the customer's default KMS key for Amazon EBS
* is used.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(replicationJobId());
hashCode = 31 * hashCode + Objects.hashCode(frequency());
hashCode = 31 * hashCode + Objects.hashCode(nextReplicationRunStartTime());
hashCode = 31 * hashCode + Objects.hashCode(licenseTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(roleName());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(numberOfRecentAmisToKeep());
hashCode = 31 * hashCode + Objects.hashCode(encrypted());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateReplicationJobRequest)) {
return false;
}
UpdateReplicationJobRequest other = (UpdateReplicationJobRequest) obj;
return Objects.equals(replicationJobId(), other.replicationJobId()) && Objects.equals(frequency(), other.frequency())
&& Objects.equals(nextReplicationRunStartTime(), other.nextReplicationRunStartTime())
&& Objects.equals(licenseTypeAsString(), other.licenseTypeAsString())
&& Objects.equals(roleName(), other.roleName()) && Objects.equals(description(), other.description())
&& Objects.equals(numberOfRecentAmisToKeep(), other.numberOfRecentAmisToKeep())
&& Objects.equals(encrypted(), other.encrypted()) && Objects.equals(kmsKeyId(), other.kmsKeyId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateReplicationJobRequest").add("ReplicationJobId", replicationJobId())
.add("Frequency", frequency()).add("NextReplicationRunStartTime", nextReplicationRunStartTime())
.add("LicenseType", licenseTypeAsString()).add("RoleName", roleName()).add("Description", description())
.add("NumberOfRecentAmisToKeep", numberOfRecentAmisToKeep()).add("Encrypted", encrypted())
.add("KmsKeyId", kmsKeyId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "replicationJobId":
return Optional.ofNullable(clazz.cast(replicationJobId()));
case "frequency":
return Optional.ofNullable(clazz.cast(frequency()));
case "nextReplicationRunStartTime":
return Optional.ofNullable(clazz.cast(nextReplicationRunStartTime()));
case "licenseType":
return Optional.ofNullable(clazz.cast(licenseTypeAsString()));
case "roleName":
return Optional.ofNullable(clazz.cast(roleName()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "numberOfRecentAmisToKeep":
return Optional.ofNullable(clazz.cast(numberOfRecentAmisToKeep()));
case "encrypted":
return Optional.ofNullable(clazz.cast(encrypted()));
case "kmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* -
*
* KMS key ID
*
*
* -
*
* KMS key alias
*
*
* -
*
* ARN referring to the KMS key ID
*
*
* -
*
* ARN referring to the KMS key alias
*
*
*
*
* If encrypted is enabled but a KMS key ID is not specified, the customer's default KMS key for Amazon
* EBS is used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder kmsKeyId(String kmsKeyId);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends SmsRequest.BuilderImpl implements Builder {
private String replicationJobId;
private Integer frequency;
private Instant nextReplicationRunStartTime;
private String licenseType;
private String roleName;
private String description;
private Integer numberOfRecentAmisToKeep;
private Boolean encrypted;
private String kmsKeyId;
private BuilderImpl() {
}
private BuilderImpl(UpdateReplicationJobRequest model) {
super(model);
replicationJobId(model.replicationJobId);
frequency(model.frequency);
nextReplicationRunStartTime(model.nextReplicationRunStartTime);
licenseType(model.licenseType);
roleName(model.roleName);
description(model.description);
numberOfRecentAmisToKeep(model.numberOfRecentAmisToKeep);
encrypted(model.encrypted);
kmsKeyId(model.kmsKeyId);
}
public final String getReplicationJobId() {
return replicationJobId;
}
public final void setReplicationJobId(String replicationJobId) {
this.replicationJobId = replicationJobId;
}
@Override
public final Builder replicationJobId(String replicationJobId) {
this.replicationJobId = replicationJobId;
return this;
}
public final Integer getFrequency() {
return frequency;
}
public final void setFrequency(Integer frequency) {
this.frequency = frequency;
}
@Override
public final Builder frequency(Integer frequency) {
this.frequency = frequency;
return this;
}
public final Instant getNextReplicationRunStartTime() {
return nextReplicationRunStartTime;
}
public final void setNextReplicationRunStartTime(Instant nextReplicationRunStartTime) {
this.nextReplicationRunStartTime = nextReplicationRunStartTime;
}
@Override
public final Builder nextReplicationRunStartTime(Instant nextReplicationRunStartTime) {
this.nextReplicationRunStartTime = nextReplicationRunStartTime;
return this;
}
public final String getLicenseType() {
return licenseType;
}
public final void setLicenseType(String licenseType) {
this.licenseType = licenseType;
}
@Override
public final Builder licenseType(String licenseType) {
this.licenseType = licenseType;
return this;
}
@Override
public final Builder licenseType(LicenseType licenseType) {
this.licenseType(licenseType == null ? null : licenseType.toString());
return this;
}
public final String getRoleName() {
return roleName;
}
public final void setRoleName(String roleName) {
this.roleName = roleName;
}
@Override
public final Builder roleName(String roleName) {
this.roleName = roleName;
return this;
}
public final String getDescription() {
return description;
}
public final void setDescription(String description) {
this.description = description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final Integer getNumberOfRecentAmisToKeep() {
return numberOfRecentAmisToKeep;
}
public final void setNumberOfRecentAmisToKeep(Integer numberOfRecentAmisToKeep) {
this.numberOfRecentAmisToKeep = numberOfRecentAmisToKeep;
}
@Override
public final Builder numberOfRecentAmisToKeep(Integer numberOfRecentAmisToKeep) {
this.numberOfRecentAmisToKeep = numberOfRecentAmisToKeep;
return this;
}
public final Boolean getEncrypted() {
return encrypted;
}
public final void setEncrypted(Boolean encrypted) {
this.encrypted = encrypted;
}
@Override
public final Builder encrypted(Boolean encrypted) {
this.encrypted = encrypted;
return this;
}
public final String getKmsKeyId() {
return kmsKeyId;
}
public final void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
@Override
public final Builder kmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public UpdateReplicationJobRequest build() {
return new UpdateReplicationJobRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}