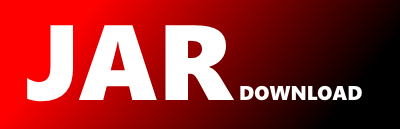
software.amazon.awssdk.services.sms.model.VmServer Maven / Gradle / Ivy
Show all versions of sms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sms.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a VM server.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class VmServer implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField VM_SERVER_ADDRESS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("vmServerAddress")
.getter(getter(VmServer::vmServerAddress)).setter(setter(Builder::vmServerAddress))
.constructor(VmServerAddress::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vmServerAddress").build()).build();
private static final SdkField VM_NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("vmName")
.getter(getter(VmServer::vmName)).setter(setter(Builder::vmName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vmName").build()).build();
private static final SdkField VM_MANAGER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("vmManagerName").getter(getter(VmServer::vmManagerName)).setter(setter(Builder::vmManagerName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vmManagerName").build()).build();
private static final SdkField VM_MANAGER_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("vmManagerType").getter(getter(VmServer::vmManagerTypeAsString)).setter(setter(Builder::vmManagerType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vmManagerType").build()).build();
private static final SdkField VM_PATH_FIELD = SdkField. builder(MarshallingType.STRING).memberName("vmPath")
.getter(getter(VmServer::vmPath)).setter(setter(Builder::vmPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vmPath").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(VM_SERVER_ADDRESS_FIELD,
VM_NAME_FIELD, VM_MANAGER_NAME_FIELD, VM_MANAGER_TYPE_FIELD, VM_PATH_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("vmServerAddress", VM_SERVER_ADDRESS_FIELD);
put("vmName", VM_NAME_FIELD);
put("vmManagerName", VM_MANAGER_NAME_FIELD);
put("vmManagerType", VM_MANAGER_TYPE_FIELD);
put("vmPath", VM_PATH_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final VmServerAddress vmServerAddress;
private final String vmName;
private final String vmManagerName;
private final String vmManagerType;
private final String vmPath;
private VmServer(BuilderImpl builder) {
this.vmServerAddress = builder.vmServerAddress;
this.vmName = builder.vmName;
this.vmManagerName = builder.vmManagerName;
this.vmManagerType = builder.vmManagerType;
this.vmPath = builder.vmPath;
}
/**
*
* The VM server location.
*
*
* @return The VM server location.
*/
public final VmServerAddress vmServerAddress() {
return vmServerAddress;
}
/**
*
* The name of the VM.
*
*
* @return The name of the VM.
*/
public final String vmName() {
return vmName;
}
/**
*
* The name of the VM manager.
*
*
* @return The name of the VM manager.
*/
public final String vmManagerName() {
return vmManagerName;
}
/**
*
* The type of VM management product.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #vmManagerType}
* will return {@link VmManagerType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #vmManagerTypeAsString}.
*
*
* @return The type of VM management product.
* @see VmManagerType
*/
public final VmManagerType vmManagerType() {
return VmManagerType.fromValue(vmManagerType);
}
/**
*
* The type of VM management product.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #vmManagerType}
* will return {@link VmManagerType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #vmManagerTypeAsString}.
*
*
* @return The type of VM management product.
* @see VmManagerType
*/
public final String vmManagerTypeAsString() {
return vmManagerType;
}
/**
*
* The VM folder path in the vCenter Server virtual machine inventory tree.
*
*
* @return The VM folder path in the vCenter Server virtual machine inventory tree.
*/
public final String vmPath() {
return vmPath;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(vmServerAddress());
hashCode = 31 * hashCode + Objects.hashCode(vmName());
hashCode = 31 * hashCode + Objects.hashCode(vmManagerName());
hashCode = 31 * hashCode + Objects.hashCode(vmManagerTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(vmPath());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof VmServer)) {
return false;
}
VmServer other = (VmServer) obj;
return Objects.equals(vmServerAddress(), other.vmServerAddress()) && Objects.equals(vmName(), other.vmName())
&& Objects.equals(vmManagerName(), other.vmManagerName())
&& Objects.equals(vmManagerTypeAsString(), other.vmManagerTypeAsString())
&& Objects.equals(vmPath(), other.vmPath());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("VmServer").add("VmServerAddress", vmServerAddress()).add("VmName", vmName())
.add("VmManagerName", vmManagerName()).add("VmManagerType", vmManagerTypeAsString()).add("VmPath", vmPath())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "vmServerAddress":
return Optional.ofNullable(clazz.cast(vmServerAddress()));
case "vmName":
return Optional.ofNullable(clazz.cast(vmName()));
case "vmManagerName":
return Optional.ofNullable(clazz.cast(vmManagerName()));
case "vmManagerType":
return Optional.ofNullable(clazz.cast(vmManagerTypeAsString()));
case "vmPath":
return Optional.ofNullable(clazz.cast(vmPath()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function