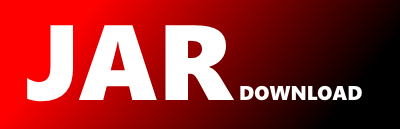
software.amazon.awssdk.services.sms.SmsClient Maven / Gradle / Ivy
Show all versions of sms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sms;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.sms.model.CreateAppRequest;
import software.amazon.awssdk.services.sms.model.CreateAppResponse;
import software.amazon.awssdk.services.sms.model.CreateReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.CreateReplicationJobResponse;
import software.amazon.awssdk.services.sms.model.DeleteAppLaunchConfigurationRequest;
import software.amazon.awssdk.services.sms.model.DeleteAppLaunchConfigurationResponse;
import software.amazon.awssdk.services.sms.model.DeleteAppReplicationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.DeleteAppReplicationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.DeleteAppRequest;
import software.amazon.awssdk.services.sms.model.DeleteAppResponse;
import software.amazon.awssdk.services.sms.model.DeleteAppValidationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.DeleteAppValidationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.DeleteReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.DeleteReplicationJobResponse;
import software.amazon.awssdk.services.sms.model.DeleteServerCatalogRequest;
import software.amazon.awssdk.services.sms.model.DeleteServerCatalogResponse;
import software.amazon.awssdk.services.sms.model.DisassociateConnectorRequest;
import software.amazon.awssdk.services.sms.model.DisassociateConnectorResponse;
import software.amazon.awssdk.services.sms.model.DryRunOperationException;
import software.amazon.awssdk.services.sms.model.GenerateChangeSetRequest;
import software.amazon.awssdk.services.sms.model.GenerateChangeSetResponse;
import software.amazon.awssdk.services.sms.model.GenerateTemplateRequest;
import software.amazon.awssdk.services.sms.model.GenerateTemplateResponse;
import software.amazon.awssdk.services.sms.model.GetAppLaunchConfigurationRequest;
import software.amazon.awssdk.services.sms.model.GetAppLaunchConfigurationResponse;
import software.amazon.awssdk.services.sms.model.GetAppReplicationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.GetAppReplicationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.GetAppRequest;
import software.amazon.awssdk.services.sms.model.GetAppResponse;
import software.amazon.awssdk.services.sms.model.GetAppValidationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.GetAppValidationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.GetAppValidationOutputRequest;
import software.amazon.awssdk.services.sms.model.GetAppValidationOutputResponse;
import software.amazon.awssdk.services.sms.model.GetConnectorsRequest;
import software.amazon.awssdk.services.sms.model.GetConnectorsResponse;
import software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest;
import software.amazon.awssdk.services.sms.model.GetReplicationJobsResponse;
import software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest;
import software.amazon.awssdk.services.sms.model.GetReplicationRunsResponse;
import software.amazon.awssdk.services.sms.model.GetServersRequest;
import software.amazon.awssdk.services.sms.model.GetServersResponse;
import software.amazon.awssdk.services.sms.model.ImportAppCatalogRequest;
import software.amazon.awssdk.services.sms.model.ImportAppCatalogResponse;
import software.amazon.awssdk.services.sms.model.ImportServerCatalogRequest;
import software.amazon.awssdk.services.sms.model.ImportServerCatalogResponse;
import software.amazon.awssdk.services.sms.model.InternalErrorException;
import software.amazon.awssdk.services.sms.model.InvalidParameterException;
import software.amazon.awssdk.services.sms.model.LaunchAppRequest;
import software.amazon.awssdk.services.sms.model.LaunchAppResponse;
import software.amazon.awssdk.services.sms.model.ListAppsRequest;
import software.amazon.awssdk.services.sms.model.ListAppsResponse;
import software.amazon.awssdk.services.sms.model.MissingRequiredParameterException;
import software.amazon.awssdk.services.sms.model.NoConnectorsAvailableException;
import software.amazon.awssdk.services.sms.model.NotifyAppValidationOutputRequest;
import software.amazon.awssdk.services.sms.model.NotifyAppValidationOutputResponse;
import software.amazon.awssdk.services.sms.model.OperationNotPermittedException;
import software.amazon.awssdk.services.sms.model.PutAppLaunchConfigurationRequest;
import software.amazon.awssdk.services.sms.model.PutAppLaunchConfigurationResponse;
import software.amazon.awssdk.services.sms.model.PutAppReplicationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.PutAppReplicationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.PutAppValidationConfigurationRequest;
import software.amazon.awssdk.services.sms.model.PutAppValidationConfigurationResponse;
import software.amazon.awssdk.services.sms.model.ReplicationJobAlreadyExistsException;
import software.amazon.awssdk.services.sms.model.ReplicationJobNotFoundException;
import software.amazon.awssdk.services.sms.model.ReplicationRunLimitExceededException;
import software.amazon.awssdk.services.sms.model.ServerCannotBeReplicatedException;
import software.amazon.awssdk.services.sms.model.SmsException;
import software.amazon.awssdk.services.sms.model.StartAppReplicationRequest;
import software.amazon.awssdk.services.sms.model.StartAppReplicationResponse;
import software.amazon.awssdk.services.sms.model.StartOnDemandAppReplicationRequest;
import software.amazon.awssdk.services.sms.model.StartOnDemandAppReplicationResponse;
import software.amazon.awssdk.services.sms.model.StartOnDemandReplicationRunRequest;
import software.amazon.awssdk.services.sms.model.StartOnDemandReplicationRunResponse;
import software.amazon.awssdk.services.sms.model.StopAppReplicationRequest;
import software.amazon.awssdk.services.sms.model.StopAppReplicationResponse;
import software.amazon.awssdk.services.sms.model.TemporarilyUnavailableException;
import software.amazon.awssdk.services.sms.model.TerminateAppRequest;
import software.amazon.awssdk.services.sms.model.TerminateAppResponse;
import software.amazon.awssdk.services.sms.model.UnauthorizedOperationException;
import software.amazon.awssdk.services.sms.model.UpdateAppRequest;
import software.amazon.awssdk.services.sms.model.UpdateAppResponse;
import software.amazon.awssdk.services.sms.model.UpdateReplicationJobRequest;
import software.amazon.awssdk.services.sms.model.UpdateReplicationJobResponse;
import software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable;
import software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable;
import software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable;
import software.amazon.awssdk.services.sms.paginators.GetServersIterable;
/**
* Service client for accessing SMS. This can be created using the static {@link #builder()} method.
*
*
*
* Product update
*
*
* We recommend Amazon Web Services Application Migration
* Service (Amazon Web Services MGN) as the primary migration service for lift-and-shift migrations. If Amazon Web
* Services MGN is unavailable in a specific Amazon Web Services Region, you can use the Server Migration Service APIs
* through March 2023.
*
*
*
* Server Migration Service (Server Migration Service) makes it easier and faster for you to migrate your on-premises
* workloads to Amazon Web Services. To learn more about Server Migration Service, see the following resources:
*
*
* -
*
*
* -
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface SmsClient extends AwsClient {
String SERVICE_NAME = "sms";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "sms";
/**
*
* Creates an application. An application consists of one or more server groups. Each server group contain one or
* more servers.
*
*
* @param createAppRequest
* @return Result of the CreateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.CreateApp
* @see AWS API
* Documentation
*/
default CreateAppResponse createApp(CreateAppRequest createAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an application. An application consists of one or more server groups. Each server group contain one or
* more servers.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAppRequest.Builder} avoiding the need to
* create one manually via {@link CreateAppRequest#builder()}
*
*
* @param createAppRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.CreateAppRequest.Builder} to create a request.
* @return Result of the CreateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.CreateApp
* @see AWS API
* Documentation
*/
default CreateAppResponse createApp(Consumer createAppRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return createApp(CreateAppRequest.builder().applyMutation(createAppRequest).build());
}
/**
*
* Creates a replication job. The replication job schedules periodic replication runs to replicate your server to
* Amazon Web Services. Each replication run creates an Amazon Machine Image (AMI).
*
*
* @param createReplicationJobRequest
* @return Result of the CreateReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ServerCannotBeReplicatedException
* The specified server cannot be replicated.
* @throws ReplicationJobAlreadyExistsException
* The specified replication job already exists.
* @throws NoConnectorsAvailableException
* There are no connectors available.
* @throws InternalErrorException
* An internal error occurred.
* @throws TemporarilyUnavailableException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.CreateReplicationJob
* @see AWS API
* Documentation
*/
default CreateReplicationJobResponse createReplicationJob(CreateReplicationJobRequest createReplicationJobRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
OperationNotPermittedException, ServerCannotBeReplicatedException, ReplicationJobAlreadyExistsException,
NoConnectorsAvailableException, InternalErrorException, TemporarilyUnavailableException, AwsServiceException,
SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a replication job. The replication job schedules periodic replication runs to replicate your server to
* Amazon Web Services. Each replication run creates an Amazon Machine Image (AMI).
*
*
*
* This is a convenience which creates an instance of the {@link CreateReplicationJobRequest.Builder} avoiding the
* need to create one manually via {@link CreateReplicationJobRequest#builder()}
*
*
* @param createReplicationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.CreateReplicationJobRequest.Builder} to create a request.
* @return Result of the CreateReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ServerCannotBeReplicatedException
* The specified server cannot be replicated.
* @throws ReplicationJobAlreadyExistsException
* The specified replication job already exists.
* @throws NoConnectorsAvailableException
* There are no connectors available.
* @throws InternalErrorException
* An internal error occurred.
* @throws TemporarilyUnavailableException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.CreateReplicationJob
* @see AWS API
* Documentation
*/
default CreateReplicationJobResponse createReplicationJob(
Consumer createReplicationJobRequest) throws InvalidParameterException,
MissingRequiredParameterException, UnauthorizedOperationException, OperationNotPermittedException,
ServerCannotBeReplicatedException, ReplicationJobAlreadyExistsException, NoConnectorsAvailableException,
InternalErrorException, TemporarilyUnavailableException, AwsServiceException, SdkClientException, SmsException {
return createReplicationJob(CreateReplicationJobRequest.builder().applyMutation(createReplicationJobRequest).build());
}
/**
*
* Deletes the specified application. Optionally deletes the launched stack associated with the application and all
* Server Migration Service replication jobs for servers in the application.
*
*
* @param deleteAppRequest
* @return Result of the DeleteApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteApp
* @see AWS API
* Documentation
*/
default DeleteAppResponse deleteApp(DeleteAppRequest deleteAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified application. Optionally deletes the launched stack associated with the application and all
* Server Migration Service replication jobs for servers in the application.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAppRequest.Builder} avoiding the need to
* create one manually via {@link DeleteAppRequest#builder()}
*
*
* @param deleteAppRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.DeleteAppRequest.Builder} to create a request.
* @return Result of the DeleteApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteApp
* @see AWS API
* Documentation
*/
default DeleteAppResponse deleteApp(Consumer deleteAppRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return deleteApp(DeleteAppRequest.builder().applyMutation(deleteAppRequest).build());
}
/**
*
* Deletes the launch configuration for the specified application.
*
*
* @param deleteAppLaunchConfigurationRequest
* @return Result of the DeleteAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteAppLaunchConfiguration
* @see AWS API Documentation
*/
default DeleteAppLaunchConfigurationResponse deleteAppLaunchConfiguration(
DeleteAppLaunchConfigurationRequest deleteAppLaunchConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the launch configuration for the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAppLaunchConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link DeleteAppLaunchConfigurationRequest#builder()}
*
*
* @param deleteAppLaunchConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.DeleteAppLaunchConfigurationRequest.Builder} to create a
* request.
* @return Result of the DeleteAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteAppLaunchConfiguration
* @see AWS API Documentation
*/
default DeleteAppLaunchConfigurationResponse deleteAppLaunchConfiguration(
Consumer deleteAppLaunchConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return deleteAppLaunchConfiguration(DeleteAppLaunchConfigurationRequest.builder()
.applyMutation(deleteAppLaunchConfigurationRequest).build());
}
/**
*
* Deletes the replication configuration for the specified application.
*
*
* @param deleteAppReplicationConfigurationRequest
* @return Result of the DeleteAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteAppReplicationConfiguration
* @see AWS API Documentation
*/
default DeleteAppReplicationConfigurationResponse deleteAppReplicationConfiguration(
DeleteAppReplicationConfigurationRequest deleteAppReplicationConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the replication configuration for the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAppReplicationConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link DeleteAppReplicationConfigurationRequest#builder()}
*
*
* @param deleteAppReplicationConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.DeleteAppReplicationConfigurationRequest.Builder} to
* create a request.
* @return Result of the DeleteAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteAppReplicationConfiguration
* @see AWS API Documentation
*/
default DeleteAppReplicationConfigurationResponse deleteAppReplicationConfiguration(
Consumer deleteAppReplicationConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return deleteAppReplicationConfiguration(DeleteAppReplicationConfigurationRequest.builder()
.applyMutation(deleteAppReplicationConfigurationRequest).build());
}
/**
*
* Deletes the validation configuration for the specified application.
*
*
* @param deleteAppValidationConfigurationRequest
* @return Result of the DeleteAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteAppValidationConfiguration
* @see AWS API Documentation
*/
default DeleteAppValidationConfigurationResponse deleteAppValidationConfiguration(
DeleteAppValidationConfigurationRequest deleteAppValidationConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the validation configuration for the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAppValidationConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link DeleteAppValidationConfigurationRequest#builder()}
*
*
* @param deleteAppValidationConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.DeleteAppValidationConfigurationRequest.Builder} to
* create a request.
* @return Result of the DeleteAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteAppValidationConfiguration
* @see AWS API Documentation
*/
default DeleteAppValidationConfigurationResponse deleteAppValidationConfiguration(
Consumer deleteAppValidationConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return deleteAppValidationConfiguration(DeleteAppValidationConfigurationRequest.builder()
.applyMutation(deleteAppValidationConfigurationRequest).build());
}
/**
*
* Deletes the specified replication job.
*
*
* After you delete a replication job, there are no further replication runs. Amazon Web Services deletes the
* contents of the Amazon S3 bucket used to store Server Migration Service artifacts. The AMIs created by the
* replication runs are not deleted.
*
*
* @param deleteReplicationJobRequest
* @return Result of the DeleteReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ReplicationJobNotFoundException
* The specified replication job does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteReplicationJob
* @see AWS API
* Documentation
*/
default DeleteReplicationJobResponse deleteReplicationJob(DeleteReplicationJobRequest deleteReplicationJobRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
OperationNotPermittedException, ReplicationJobNotFoundException, AwsServiceException, SdkClientException,
SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified replication job.
*
*
* After you delete a replication job, there are no further replication runs. Amazon Web Services deletes the
* contents of the Amazon S3 bucket used to store Server Migration Service artifacts. The AMIs created by the
* replication runs are not deleted.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteReplicationJobRequest.Builder} avoiding the
* need to create one manually via {@link DeleteReplicationJobRequest#builder()}
*
*
* @param deleteReplicationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.DeleteReplicationJobRequest.Builder} to create a request.
* @return Result of the DeleteReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ReplicationJobNotFoundException
* The specified replication job does not exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteReplicationJob
* @see AWS API
* Documentation
*/
default DeleteReplicationJobResponse deleteReplicationJob(
Consumer deleteReplicationJobRequest) throws InvalidParameterException,
MissingRequiredParameterException, UnauthorizedOperationException, OperationNotPermittedException,
ReplicationJobNotFoundException, AwsServiceException, SdkClientException, SmsException {
return deleteReplicationJob(DeleteReplicationJobRequest.builder().applyMutation(deleteReplicationJobRequest).build());
}
/**
*
* Deletes all servers from your server catalog.
*
*
* @param deleteServerCatalogRequest
* @return Result of the DeleteServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteServerCatalog
* @see AWS API
* Documentation
*/
default DeleteServerCatalogResponse deleteServerCatalog(DeleteServerCatalogRequest deleteServerCatalogRequest)
throws UnauthorizedOperationException, OperationNotPermittedException, InvalidParameterException,
MissingRequiredParameterException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes all servers from your server catalog.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteServerCatalogRequest.Builder} avoiding the
* need to create one manually via {@link DeleteServerCatalogRequest#builder()}
*
*
* @param deleteServerCatalogRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.DeleteServerCatalogRequest.Builder} to create a request.
* @return Result of the DeleteServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteServerCatalog
* @see AWS API
* Documentation
*/
default DeleteServerCatalogResponse deleteServerCatalog(
Consumer deleteServerCatalogRequest) throws UnauthorizedOperationException,
OperationNotPermittedException, InvalidParameterException, MissingRequiredParameterException, AwsServiceException,
SdkClientException, SmsException {
return deleteServerCatalog(DeleteServerCatalogRequest.builder().applyMutation(deleteServerCatalogRequest).build());
}
/**
*
* Deletes all servers from your server catalog.
*
*
* @return Result of the DeleteServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DeleteServerCatalog
* @see #deleteServerCatalog(DeleteServerCatalogRequest)
* @see AWS API
* Documentation
*/
default DeleteServerCatalogResponse deleteServerCatalog() throws UnauthorizedOperationException,
OperationNotPermittedException, InvalidParameterException, MissingRequiredParameterException, AwsServiceException,
SdkClientException, SmsException {
return deleteServerCatalog(DeleteServerCatalogRequest.builder().build());
}
/**
*
* Disassociates the specified connector from Server Migration Service.
*
*
* After you disassociate a connector, it is no longer available to support replication jobs.
*
*
* @param disassociateConnectorRequest
* @return Result of the DisassociateConnector operation returned by the service.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DisassociateConnector
* @see AWS API
* Documentation
*/
default DisassociateConnectorResponse disassociateConnector(DisassociateConnectorRequest disassociateConnectorRequest)
throws MissingRequiredParameterException, UnauthorizedOperationException, OperationNotPermittedException,
InvalidParameterException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates the specified connector from Server Migration Service.
*
*
* After you disassociate a connector, it is no longer available to support replication jobs.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateConnectorRequest.Builder} avoiding the
* need to create one manually via {@link DisassociateConnectorRequest#builder()}
*
*
* @param disassociateConnectorRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.DisassociateConnectorRequest.Builder} to create a
* request.
* @return Result of the DisassociateConnector operation returned by the service.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.DisassociateConnector
* @see AWS API
* Documentation
*/
default DisassociateConnectorResponse disassociateConnector(
Consumer disassociateConnectorRequest)
throws MissingRequiredParameterException, UnauthorizedOperationException, OperationNotPermittedException,
InvalidParameterException, AwsServiceException, SdkClientException, SmsException {
return disassociateConnector(DisassociateConnectorRequest.builder().applyMutation(disassociateConnectorRequest).build());
}
/**
*
* Generates a target change set for a currently launched stack and writes it to an Amazon S3 object in the
* customer’s Amazon S3 bucket.
*
*
* @param generateChangeSetRequest
* @return Result of the GenerateChangeSet operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GenerateChangeSet
* @see AWS API
* Documentation
*/
default GenerateChangeSetResponse generateChangeSet(GenerateChangeSetRequest generateChangeSetRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Generates a target change set for a currently launched stack and writes it to an Amazon S3 object in the
* customer’s Amazon S3 bucket.
*
*
*
* This is a convenience which creates an instance of the {@link GenerateChangeSetRequest.Builder} avoiding the need
* to create one manually via {@link GenerateChangeSetRequest#builder()}
*
*
* @param generateChangeSetRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GenerateChangeSetRequest.Builder} to create a request.
* @return Result of the GenerateChangeSet operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GenerateChangeSet
* @see AWS API
* Documentation
*/
default GenerateChangeSetResponse generateChangeSet(Consumer generateChangeSetRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return generateChangeSet(GenerateChangeSetRequest.builder().applyMutation(generateChangeSetRequest).build());
}
/**
*
* Generates an CloudFormation template based on the current launch configuration and writes it to an Amazon S3
* object in the customer’s Amazon S3 bucket.
*
*
* @param generateTemplateRequest
* @return Result of the GenerateTemplate operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GenerateTemplate
* @see AWS API
* Documentation
*/
default GenerateTemplateResponse generateTemplate(GenerateTemplateRequest generateTemplateRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Generates an CloudFormation template based on the current launch configuration and writes it to an Amazon S3
* object in the customer’s Amazon S3 bucket.
*
*
*
* This is a convenience which creates an instance of the {@link GenerateTemplateRequest.Builder} avoiding the need
* to create one manually via {@link GenerateTemplateRequest#builder()}
*
*
* @param generateTemplateRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GenerateTemplateRequest.Builder} to create a request.
* @return Result of the GenerateTemplate operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GenerateTemplate
* @see AWS API
* Documentation
*/
default GenerateTemplateResponse generateTemplate(Consumer generateTemplateRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return generateTemplate(GenerateTemplateRequest.builder().applyMutation(generateTemplateRequest).build());
}
/**
*
* Retrieve information about the specified application.
*
*
* @param getAppRequest
* @return Result of the GetApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetApp
* @see AWS API
* Documentation
*/
default GetAppResponse getApp(GetAppRequest getAppRequest) throws UnauthorizedOperationException, InvalidParameterException,
MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException, AwsServiceException,
SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieve information about the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link GetAppRequest.Builder} avoiding the need to create
* one manually via {@link GetAppRequest#builder()}
*
*
* @param getAppRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetAppRequest.Builder} to create a request.
* @return Result of the GetApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetApp
* @see AWS API
* Documentation
*/
default GetAppResponse getApp(Consumer getAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
return getApp(GetAppRequest.builder().applyMutation(getAppRequest).build());
}
/**
*
* Retrieve information about the specified application.
*
*
* @return Result of the GetApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetApp
* @see #getApp(GetAppRequest)
* @see AWS API
* Documentation
*/
default GetAppResponse getApp() throws UnauthorizedOperationException, InvalidParameterException,
MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException, AwsServiceException,
SdkClientException, SmsException {
return getApp(GetAppRequest.builder().build());
}
/**
*
* Retrieves the application launch configuration associated with the specified application.
*
*
* @param getAppLaunchConfigurationRequest
* @return Result of the GetAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppLaunchConfiguration
* @see AWS
* API Documentation
*/
default GetAppLaunchConfigurationResponse getAppLaunchConfiguration(
GetAppLaunchConfigurationRequest getAppLaunchConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the application launch configuration associated with the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link GetAppLaunchConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link GetAppLaunchConfigurationRequest#builder()}
*
*
* @param getAppLaunchConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetAppLaunchConfigurationRequest.Builder} to create a
* request.
* @return Result of the GetAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppLaunchConfiguration
* @see AWS
* API Documentation
*/
default GetAppLaunchConfigurationResponse getAppLaunchConfiguration(
Consumer getAppLaunchConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return getAppLaunchConfiguration(GetAppLaunchConfigurationRequest.builder()
.applyMutation(getAppLaunchConfigurationRequest).build());
}
/**
*
* Retrieves the application launch configuration associated with the specified application.
*
*
* @return Result of the GetAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppLaunchConfiguration
* @see #getAppLaunchConfiguration(GetAppLaunchConfigurationRequest)
* @see AWS
* API Documentation
*/
default GetAppLaunchConfigurationResponse getAppLaunchConfiguration() throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
return getAppLaunchConfiguration(GetAppLaunchConfigurationRequest.builder().build());
}
/**
*
* Retrieves the application replication configuration associated with the specified application.
*
*
* @param getAppReplicationConfigurationRequest
* @return Result of the GetAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppReplicationConfiguration
* @see AWS API Documentation
*/
default GetAppReplicationConfigurationResponse getAppReplicationConfiguration(
GetAppReplicationConfigurationRequest getAppReplicationConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the application replication configuration associated with the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link GetAppReplicationConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link GetAppReplicationConfigurationRequest#builder()}
*
*
* @param getAppReplicationConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetAppReplicationConfigurationRequest.Builder} to create
* a request.
* @return Result of the GetAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppReplicationConfiguration
* @see AWS API Documentation
*/
default GetAppReplicationConfigurationResponse getAppReplicationConfiguration(
Consumer getAppReplicationConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return getAppReplicationConfiguration(GetAppReplicationConfigurationRequest.builder()
.applyMutation(getAppReplicationConfigurationRequest).build());
}
/**
*
* Retrieves the application replication configuration associated with the specified application.
*
*
* @return Result of the GetAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppReplicationConfiguration
* @see #getAppReplicationConfiguration(GetAppReplicationConfigurationRequest)
* @see AWS API Documentation
*/
default GetAppReplicationConfigurationResponse getAppReplicationConfiguration() throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
return getAppReplicationConfiguration(GetAppReplicationConfigurationRequest.builder().build());
}
/**
*
* Retrieves information about a configuration for validating an application.
*
*
* @param getAppValidationConfigurationRequest
* @return Result of the GetAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppValidationConfiguration
* @see AWS API Documentation
*/
default GetAppValidationConfigurationResponse getAppValidationConfiguration(
GetAppValidationConfigurationRequest getAppValidationConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about a configuration for validating an application.
*
*
*
* This is a convenience which creates an instance of the {@link GetAppValidationConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link GetAppValidationConfigurationRequest#builder()}
*
*
* @param getAppValidationConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetAppValidationConfigurationRequest.Builder} to create a
* request.
* @return Result of the GetAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppValidationConfiguration
* @see AWS API Documentation
*/
default GetAppValidationConfigurationResponse getAppValidationConfiguration(
Consumer getAppValidationConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return getAppValidationConfiguration(GetAppValidationConfigurationRequest.builder()
.applyMutation(getAppValidationConfigurationRequest).build());
}
/**
*
* Retrieves output from validating an application.
*
*
* @param getAppValidationOutputRequest
* @return Result of the GetAppValidationOutput operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppValidationOutput
* @see AWS
* API Documentation
*/
default GetAppValidationOutputResponse getAppValidationOutput(GetAppValidationOutputRequest getAppValidationOutputRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves output from validating an application.
*
*
*
* This is a convenience which creates an instance of the {@link GetAppValidationOutputRequest.Builder} avoiding the
* need to create one manually via {@link GetAppValidationOutputRequest#builder()}
*
*
* @param getAppValidationOutputRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetAppValidationOutputRequest.Builder} to create a
* request.
* @return Result of the GetAppValidationOutput operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetAppValidationOutput
* @see AWS
* API Documentation
*/
default GetAppValidationOutputResponse getAppValidationOutput(
Consumer getAppValidationOutputRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
return getAppValidationOutput(GetAppValidationOutputRequest.builder().applyMutation(getAppValidationOutputRequest)
.build());
}
/**
*
* Describes the connectors registered with the Server Migration Service.
*
*
* @param getConnectorsRequest
* @return Result of the GetConnectors operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetConnectors
* @see AWS API
* Documentation
*/
default GetConnectorsResponse getConnectors(GetConnectorsRequest getConnectorsRequest) throws UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the connectors registered with the Server Migration Service.
*
*
*
* This is a convenience which creates an instance of the {@link GetConnectorsRequest.Builder} avoiding the need to
* create one manually via {@link GetConnectorsRequest#builder()}
*
*
* @param getConnectorsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetConnectorsRequest.Builder} to create a request.
* @return Result of the GetConnectors operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetConnectors
* @see AWS API
* Documentation
*/
default GetConnectorsResponse getConnectors(Consumer getConnectorsRequest)
throws UnauthorizedOperationException, AwsServiceException, SdkClientException, SmsException {
return getConnectors(GetConnectorsRequest.builder().applyMutation(getConnectorsRequest).build());
}
/**
*
* Describes the connectors registered with the Server Migration Service.
*
*
* @return Result of the GetConnectors operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetConnectors
* @see #getConnectors(GetConnectorsRequest)
* @see AWS API
* Documentation
*/
default GetConnectorsResponse getConnectors() throws UnauthorizedOperationException, AwsServiceException, SdkClientException,
SmsException {
return getConnectors(GetConnectorsRequest.builder().build());
}
/**
*
* This is a variant of {@link #getConnectors(software.amazon.awssdk.services.sms.model.GetConnectorsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetConnectorsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getConnectors(software.amazon.awssdk.services.sms.model.GetConnectorsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetConnectors
* @see #getConnectorsPaginator(GetConnectorsRequest)
* @see AWS API
* Documentation
*/
default GetConnectorsIterable getConnectorsPaginator() throws UnauthorizedOperationException, AwsServiceException,
SdkClientException, SmsException {
return getConnectorsPaginator(GetConnectorsRequest.builder().build());
}
/**
*
* This is a variant of {@link #getConnectors(software.amazon.awssdk.services.sms.model.GetConnectorsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetConnectorsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getConnectors(software.amazon.awssdk.services.sms.model.GetConnectorsRequest)} operation.
*
*
* @param getConnectorsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetConnectors
* @see AWS API
* Documentation
*/
default GetConnectorsIterable getConnectorsPaginator(GetConnectorsRequest getConnectorsRequest)
throws UnauthorizedOperationException, AwsServiceException, SdkClientException, SmsException {
return new GetConnectorsIterable(this, getConnectorsRequest);
}
/**
*
* This is a variant of {@link #getConnectors(software.amazon.awssdk.services.sms.model.GetConnectorsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetConnectorsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetConnectorsIterable responses = client.getConnectorsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getConnectors(software.amazon.awssdk.services.sms.model.GetConnectorsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetConnectorsRequest.Builder} avoiding the need to
* create one manually via {@link GetConnectorsRequest#builder()}
*
*
* @param getConnectorsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetConnectorsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetConnectors
* @see AWS API
* Documentation
*/
default GetConnectorsIterable getConnectorsPaginator(Consumer getConnectorsRequest)
throws UnauthorizedOperationException, AwsServiceException, SdkClientException, SmsException {
return getConnectorsPaginator(GetConnectorsRequest.builder().applyMutation(getConnectorsRequest).build());
}
/**
*
* Describes the specified replication job or all of your replication jobs.
*
*
* @param getReplicationJobsRequest
* @return Result of the GetReplicationJobs operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationJobs
* @see AWS API
* Documentation
*/
default GetReplicationJobsResponse getReplicationJobs(GetReplicationJobsRequest getReplicationJobsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the specified replication job or all of your replication jobs.
*
*
*
* This is a convenience which creates an instance of the {@link GetReplicationJobsRequest.Builder} avoiding the
* need to create one manually via {@link GetReplicationJobsRequest#builder()}
*
*
* @param getReplicationJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest.Builder} to create a request.
* @return Result of the GetReplicationJobs operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationJobs
* @see AWS API
* Documentation
*/
default GetReplicationJobsResponse getReplicationJobs(Consumer getReplicationJobsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
return getReplicationJobs(GetReplicationJobsRequest.builder().applyMutation(getReplicationJobsRequest).build());
}
/**
*
* Describes the specified replication job or all of your replication jobs.
*
*
* @return Result of the GetReplicationJobs operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationJobs
* @see #getReplicationJobs(GetReplicationJobsRequest)
* @see AWS API
* Documentation
*/
default GetReplicationJobsResponse getReplicationJobs() throws InvalidParameterException, MissingRequiredParameterException,
UnauthorizedOperationException, AwsServiceException, SdkClientException, SmsException {
return getReplicationJobs(GetReplicationJobsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #getReplicationJobs(software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client.getReplicationJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client
* .getReplicationJobsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetReplicationJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client.getReplicationJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getReplicationJobs(software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationJobs
* @see #getReplicationJobsPaginator(GetReplicationJobsRequest)
* @see AWS API
* Documentation
*/
default GetReplicationJobsIterable getReplicationJobsPaginator() throws InvalidParameterException,
MissingRequiredParameterException, UnauthorizedOperationException, AwsServiceException, SdkClientException,
SmsException {
return getReplicationJobsPaginator(GetReplicationJobsRequest.builder().build());
}
/**
*
* This is a variant of
* {@link #getReplicationJobs(software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client.getReplicationJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client
* .getReplicationJobsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetReplicationJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client.getReplicationJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getReplicationJobs(software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest)} operation.
*
*
* @param getReplicationJobsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationJobs
* @see AWS API
* Documentation
*/
default GetReplicationJobsIterable getReplicationJobsPaginator(GetReplicationJobsRequest getReplicationJobsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
return new GetReplicationJobsIterable(this, getReplicationJobsRequest);
}
/**
*
* This is a variant of
* {@link #getReplicationJobs(software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client.getReplicationJobsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client
* .getReplicationJobsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetReplicationJobsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationJobsIterable responses = client.getReplicationJobsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getReplicationJobs(software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetReplicationJobsRequest.Builder} avoiding the
* need to create one manually via {@link GetReplicationJobsRequest#builder()}
*
*
* @param getReplicationJobsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetReplicationJobsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationJobs
* @see AWS API
* Documentation
*/
default GetReplicationJobsIterable getReplicationJobsPaginator(
Consumer getReplicationJobsRequest) throws InvalidParameterException,
MissingRequiredParameterException, UnauthorizedOperationException, AwsServiceException, SdkClientException,
SmsException {
return getReplicationJobsPaginator(GetReplicationJobsRequest.builder().applyMutation(getReplicationJobsRequest).build());
}
/**
*
* Describes the replication runs for the specified replication job.
*
*
* @param getReplicationRunsRequest
* @return Result of the GetReplicationRuns operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationRuns
* @see AWS API
* Documentation
*/
default GetReplicationRunsResponse getReplicationRuns(GetReplicationRunsRequest getReplicationRunsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the replication runs for the specified replication job.
*
*
*
* This is a convenience which creates an instance of the {@link GetReplicationRunsRequest.Builder} avoiding the
* need to create one manually via {@link GetReplicationRunsRequest#builder()}
*
*
* @param getReplicationRunsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest.Builder} to create a request.
* @return Result of the GetReplicationRuns operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationRuns
* @see AWS API
* Documentation
*/
default GetReplicationRunsResponse getReplicationRuns(Consumer getReplicationRunsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
return getReplicationRuns(GetReplicationRunsRequest.builder().applyMutation(getReplicationRunsRequest).build());
}
/**
*
* This is a variant of
* {@link #getReplicationRuns(software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable responses = client.getReplicationRunsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable responses = client
* .getReplicationRunsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetReplicationRunsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable responses = client.getReplicationRunsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getReplicationRuns(software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest)} operation.
*
*
* @param getReplicationRunsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationRuns
* @see AWS API
* Documentation
*/
default GetReplicationRunsIterable getReplicationRunsPaginator(GetReplicationRunsRequest getReplicationRunsRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
AwsServiceException, SdkClientException, SmsException {
return new GetReplicationRunsIterable(this, getReplicationRunsRequest);
}
/**
*
* This is a variant of
* {@link #getReplicationRuns(software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest)} operation. The
* return type is a custom iterable that can be used to iterate through all the pages. SDK will internally handle
* making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable responses = client.getReplicationRunsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable responses = client
* .getReplicationRunsPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetReplicationRunsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetReplicationRunsIterable responses = client.getReplicationRunsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getReplicationRuns(software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetReplicationRunsRequest.Builder} avoiding the
* need to create one manually via {@link GetReplicationRunsRequest#builder()}
*
*
* @param getReplicationRunsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetReplicationRunsRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetReplicationRuns
* @see AWS API
* Documentation
*/
default GetReplicationRunsIterable getReplicationRunsPaginator(
Consumer getReplicationRunsRequest) throws InvalidParameterException,
MissingRequiredParameterException, UnauthorizedOperationException, AwsServiceException, SdkClientException,
SmsException {
return getReplicationRunsPaginator(GetReplicationRunsRequest.builder().applyMutation(getReplicationRunsRequest).build());
}
/**
*
* Describes the servers in your server catalog.
*
*
* Before you can describe your servers, you must import them using ImportServerCatalog.
*
*
* @param getServersRequest
* @return Result of the GetServers operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetServers
* @see AWS API
* Documentation
*/
default GetServersResponse getServers(GetServersRequest getServersRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, AwsServiceException,
SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the servers in your server catalog.
*
*
* Before you can describe your servers, you must import them using ImportServerCatalog.
*
*
*
* This is a convenience which creates an instance of the {@link GetServersRequest.Builder} avoiding the need to
* create one manually via {@link GetServersRequest#builder()}
*
*
* @param getServersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetServersRequest.Builder} to create a request.
* @return Result of the GetServers operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetServers
* @see AWS API
* Documentation
*/
default GetServersResponse getServers(Consumer getServersRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, AwsServiceException, SdkClientException, SmsException {
return getServers(GetServersRequest.builder().applyMutation(getServersRequest).build());
}
/**
*
* Describes the servers in your server catalog.
*
*
* Before you can describe your servers, you must import them using ImportServerCatalog.
*
*
* @return Result of the GetServers operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetServers
* @see #getServers(GetServersRequest)
* @see AWS API
* Documentation
*/
default GetServersResponse getServers() throws UnauthorizedOperationException, InvalidParameterException,
MissingRequiredParameterException, InternalErrorException, AwsServiceException, SdkClientException, SmsException {
return getServers(GetServersRequest.builder().build());
}
/**
*
* This is a variant of {@link #getServers(software.amazon.awssdk.services.sms.model.GetServersRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetServersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getServers(software.amazon.awssdk.services.sms.model.GetServersRequest)} operation.
*
*
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetServers
* @see #getServersPaginator(GetServersRequest)
* @see AWS API
* Documentation
*/
default GetServersIterable getServersPaginator() throws UnauthorizedOperationException, InvalidParameterException,
MissingRequiredParameterException, InternalErrorException, AwsServiceException, SdkClientException, SmsException {
return getServersPaginator(GetServersRequest.builder().build());
}
/**
*
* This is a variant of {@link #getServers(software.amazon.awssdk.services.sms.model.GetServersRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetServersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getServers(software.amazon.awssdk.services.sms.model.GetServersRequest)} operation.
*
*
* @param getServersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetServers
* @see AWS API
* Documentation
*/
default GetServersIterable getServersPaginator(GetServersRequest getServersRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, AwsServiceException,
SdkClientException, SmsException {
return new GetServersIterable(this, getServersRequest);
}
/**
*
* This is a variant of {@link #getServers(software.amazon.awssdk.services.sms.model.GetServersRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* for (software.amazon.awssdk.services.sms.model.GetServersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sms.paginators.GetServersIterable responses = client.getServersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getServers(software.amazon.awssdk.services.sms.model.GetServersRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link GetServersRequest.Builder} avoiding the need to
* create one manually via {@link GetServersRequest#builder()}
*
*
* @param getServersRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.GetServersRequest.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.GetServers
* @see AWS API
* Documentation
*/
default GetServersIterable getServersPaginator(Consumer getServersRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, AwsServiceException, SdkClientException, SmsException {
return getServersPaginator(GetServersRequest.builder().applyMutation(getServersRequest).build());
}
/**
*
* Allows application import from Migration Hub.
*
*
* @param importAppCatalogRequest
* @return Result of the ImportAppCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ImportAppCatalog
* @see AWS API
* Documentation
*/
default ImportAppCatalogResponse importAppCatalog(ImportAppCatalogRequest importAppCatalogRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Allows application import from Migration Hub.
*
*
*
* This is a convenience which creates an instance of the {@link ImportAppCatalogRequest.Builder} avoiding the need
* to create one manually via {@link ImportAppCatalogRequest#builder()}
*
*
* @param importAppCatalogRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.ImportAppCatalogRequest.Builder} to create a request.
* @return Result of the ImportAppCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ImportAppCatalog
* @see AWS API
* Documentation
*/
default ImportAppCatalogResponse importAppCatalog(Consumer importAppCatalogRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return importAppCatalog(ImportAppCatalogRequest.builder().applyMutation(importAppCatalogRequest).build());
}
/**
*
* Gathers a complete list of on-premises servers. Connectors must be installed and monitoring all servers to
* import.
*
*
* This call returns immediately, but might take additional time to retrieve all the servers.
*
*
* @param importServerCatalogRequest
* @return Result of the ImportServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws NoConnectorsAvailableException
* There are no connectors available.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ImportServerCatalog
* @see AWS API
* Documentation
*/
default ImportServerCatalogResponse importServerCatalog(ImportServerCatalogRequest importServerCatalogRequest)
throws UnauthorizedOperationException, OperationNotPermittedException, InvalidParameterException,
MissingRequiredParameterException, NoConnectorsAvailableException, AwsServiceException, SdkClientException,
SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Gathers a complete list of on-premises servers. Connectors must be installed and monitoring all servers to
* import.
*
*
* This call returns immediately, but might take additional time to retrieve all the servers.
*
*
*
* This is a convenience which creates an instance of the {@link ImportServerCatalogRequest.Builder} avoiding the
* need to create one manually via {@link ImportServerCatalogRequest#builder()}
*
*
* @param importServerCatalogRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.ImportServerCatalogRequest.Builder} to create a request.
* @return Result of the ImportServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws NoConnectorsAvailableException
* There are no connectors available.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ImportServerCatalog
* @see AWS API
* Documentation
*/
default ImportServerCatalogResponse importServerCatalog(
Consumer importServerCatalogRequest) throws UnauthorizedOperationException,
OperationNotPermittedException, InvalidParameterException, MissingRequiredParameterException,
NoConnectorsAvailableException, AwsServiceException, SdkClientException, SmsException {
return importServerCatalog(ImportServerCatalogRequest.builder().applyMutation(importServerCatalogRequest).build());
}
/**
*
* Gathers a complete list of on-premises servers. Connectors must be installed and monitoring all servers to
* import.
*
*
* This call returns immediately, but might take additional time to retrieve all the servers.
*
*
* @return Result of the ImportServerCatalog operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws NoConnectorsAvailableException
* There are no connectors available.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ImportServerCatalog
* @see #importServerCatalog(ImportServerCatalogRequest)
* @see AWS API
* Documentation
*/
default ImportServerCatalogResponse importServerCatalog() throws UnauthorizedOperationException,
OperationNotPermittedException, InvalidParameterException, MissingRequiredParameterException,
NoConnectorsAvailableException, AwsServiceException, SdkClientException, SmsException {
return importServerCatalog(ImportServerCatalogRequest.builder().build());
}
/**
*
* Launches the specified application as a stack in CloudFormation.
*
*
* @param launchAppRequest
* @return Result of the LaunchApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.LaunchApp
* @see AWS API
* Documentation
*/
default LaunchAppResponse launchApp(LaunchAppRequest launchAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Launches the specified application as a stack in CloudFormation.
*
*
*
* This is a convenience which creates an instance of the {@link LaunchAppRequest.Builder} avoiding the need to
* create one manually via {@link LaunchAppRequest#builder()}
*
*
* @param launchAppRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.LaunchAppRequest.Builder} to create a request.
* @return Result of the LaunchApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.LaunchApp
* @see AWS API
* Documentation
*/
default LaunchAppResponse launchApp(Consumer launchAppRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return launchApp(LaunchAppRequest.builder().applyMutation(launchAppRequest).build());
}
/**
*
* Retrieves summaries for all applications.
*
*
* @param listAppsRequest
* @return Result of the ListApps operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ListApps
* @see AWS API
* Documentation
*/
default ListAppsResponse listApps(ListAppsRequest listAppsRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves summaries for all applications.
*
*
*
* This is a convenience which creates an instance of the {@link ListAppsRequest.Builder} avoiding the need to
* create one manually via {@link ListAppsRequest#builder()}
*
*
* @param listAppsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.ListAppsRequest.Builder} to create a request.
* @return Result of the ListApps operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ListApps
* @see AWS API
* Documentation
*/
default ListAppsResponse listApps(Consumer listAppsRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
return listApps(ListAppsRequest.builder().applyMutation(listAppsRequest).build());
}
/**
*
* Retrieves summaries for all applications.
*
*
* @return Result of the ListApps operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.ListApps
* @see #listApps(ListAppsRequest)
* @see AWS API
* Documentation
*/
default ListAppsResponse listApps() throws UnauthorizedOperationException, InvalidParameterException,
MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException, AwsServiceException,
SdkClientException, SmsException {
return listApps(ListAppsRequest.builder().build());
}
/**
*
* Provides information to Server Migration Service about whether application validation is successful.
*
*
* @param notifyAppValidationOutputRequest
* @return Result of the NotifyAppValidationOutput operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.NotifyAppValidationOutput
* @see AWS
* API Documentation
*/
default NotifyAppValidationOutputResponse notifyAppValidationOutput(
NotifyAppValidationOutputRequest notifyAppValidationOutputRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Provides information to Server Migration Service about whether application validation is successful.
*
*
*
* This is a convenience which creates an instance of the {@link NotifyAppValidationOutputRequest.Builder} avoiding
* the need to create one manually via {@link NotifyAppValidationOutputRequest#builder()}
*
*
* @param notifyAppValidationOutputRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.NotifyAppValidationOutputRequest.Builder} to create a
* request.
* @return Result of the NotifyAppValidationOutput operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.NotifyAppValidationOutput
* @see AWS
* API Documentation
*/
default NotifyAppValidationOutputResponse notifyAppValidationOutput(
Consumer notifyAppValidationOutputRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return notifyAppValidationOutput(NotifyAppValidationOutputRequest.builder()
.applyMutation(notifyAppValidationOutputRequest).build());
}
/**
*
* Creates or updates the launch configuration for the specified application.
*
*
* @param putAppLaunchConfigurationRequest
* @return Result of the PutAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.PutAppLaunchConfiguration
* @see AWS
* API Documentation
*/
default PutAppLaunchConfigurationResponse putAppLaunchConfiguration(
PutAppLaunchConfigurationRequest putAppLaunchConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the launch configuration for the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link PutAppLaunchConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link PutAppLaunchConfigurationRequest#builder()}
*
*
* @param putAppLaunchConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.PutAppLaunchConfigurationRequest.Builder} to create a
* request.
* @return Result of the PutAppLaunchConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.PutAppLaunchConfiguration
* @see AWS
* API Documentation
*/
default PutAppLaunchConfigurationResponse putAppLaunchConfiguration(
Consumer putAppLaunchConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return putAppLaunchConfiguration(PutAppLaunchConfigurationRequest.builder()
.applyMutation(putAppLaunchConfigurationRequest).build());
}
/**
*
* Creates or updates the replication configuration for the specified application.
*
*
* @param putAppReplicationConfigurationRequest
* @return Result of the PutAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.PutAppReplicationConfiguration
* @see AWS API Documentation
*/
default PutAppReplicationConfigurationResponse putAppReplicationConfiguration(
PutAppReplicationConfigurationRequest putAppReplicationConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates the replication configuration for the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link PutAppReplicationConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutAppReplicationConfigurationRequest#builder()}
*
*
* @param putAppReplicationConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.PutAppReplicationConfigurationRequest.Builder} to create
* a request.
* @return Result of the PutAppReplicationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.PutAppReplicationConfiguration
* @see AWS API Documentation
*/
default PutAppReplicationConfigurationResponse putAppReplicationConfiguration(
Consumer putAppReplicationConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return putAppReplicationConfiguration(PutAppReplicationConfigurationRequest.builder()
.applyMutation(putAppReplicationConfigurationRequest).build());
}
/**
*
* Creates or updates a validation configuration for the specified application.
*
*
* @param putAppValidationConfigurationRequest
* @return Result of the PutAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.PutAppValidationConfiguration
* @see AWS API Documentation
*/
default PutAppValidationConfigurationResponse putAppValidationConfiguration(
PutAppValidationConfigurationRequest putAppValidationConfigurationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates a validation configuration for the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link PutAppValidationConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link PutAppValidationConfigurationRequest#builder()}
*
*
* @param putAppValidationConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.PutAppValidationConfigurationRequest.Builder} to create a
* request.
* @return Result of the PutAppValidationConfiguration operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.PutAppValidationConfiguration
* @see AWS API Documentation
*/
default PutAppValidationConfigurationResponse putAppValidationConfiguration(
Consumer putAppValidationConfigurationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return putAppValidationConfiguration(PutAppValidationConfigurationRequest.builder()
.applyMutation(putAppValidationConfigurationRequest).build());
}
/**
*
* Starts replicating the specified application by creating replication jobs for each server in the application.
*
*
* @param startAppReplicationRequest
* @return Result of the StartAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StartAppReplication
* @see AWS API
* Documentation
*/
default StartAppReplicationResponse startAppReplication(StartAppReplicationRequest startAppReplicationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Starts replicating the specified application by creating replication jobs for each server in the application.
*
*
*
* This is a convenience which creates an instance of the {@link StartAppReplicationRequest.Builder} avoiding the
* need to create one manually via {@link StartAppReplicationRequest#builder()}
*
*
* @param startAppReplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.StartAppReplicationRequest.Builder} to create a request.
* @return Result of the StartAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StartAppReplication
* @see AWS API
* Documentation
*/
default StartAppReplicationResponse startAppReplication(
Consumer startAppReplicationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
return startAppReplication(StartAppReplicationRequest.builder().applyMutation(startAppReplicationRequest).build());
}
/**
*
* Starts an on-demand replication run for the specified application.
*
*
* @param startOnDemandAppReplicationRequest
* @return Result of the StartOnDemandAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StartOnDemandAppReplication
* @see AWS API Documentation
*/
default StartOnDemandAppReplicationResponse startOnDemandAppReplication(
StartOnDemandAppReplicationRequest startOnDemandAppReplicationRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Starts an on-demand replication run for the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link StartOnDemandAppReplicationRequest.Builder}
* avoiding the need to create one manually via {@link StartOnDemandAppReplicationRequest#builder()}
*
*
* @param startOnDemandAppReplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.StartOnDemandAppReplicationRequest.Builder} to create a
* request.
* @return Result of the StartOnDemandAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StartOnDemandAppReplication
* @see AWS API Documentation
*/
default StartOnDemandAppReplicationResponse startOnDemandAppReplication(
Consumer startOnDemandAppReplicationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return startOnDemandAppReplication(StartOnDemandAppReplicationRequest.builder()
.applyMutation(startOnDemandAppReplicationRequest).build());
}
/**
*
* Starts an on-demand replication run for the specified replication job. This replication run starts immediately.
* This replication run is in addition to the ones already scheduled.
*
*
* There is a limit on the number of on-demand replications runs that you can request in a 24-hour period.
*
*
* @param startOnDemandReplicationRunRequest
* @return Result of the StartOnDemandReplicationRun operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ReplicationRunLimitExceededException
* You have exceeded the number of on-demand replication runs you can request in a 24-hour period.
* @throws DryRunOperationException
* The user has the required permissions, so the request would have succeeded, but a dry run was performed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StartOnDemandReplicationRun
* @see AWS API Documentation
*/
default StartOnDemandReplicationRunResponse startOnDemandReplicationRun(
StartOnDemandReplicationRunRequest startOnDemandReplicationRunRequest) throws InvalidParameterException,
MissingRequiredParameterException, UnauthorizedOperationException, OperationNotPermittedException,
ReplicationRunLimitExceededException, DryRunOperationException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Starts an on-demand replication run for the specified replication job. This replication run starts immediately.
* This replication run is in addition to the ones already scheduled.
*
*
* There is a limit on the number of on-demand replications runs that you can request in a 24-hour period.
*
*
*
* This is a convenience which creates an instance of the {@link StartOnDemandReplicationRunRequest.Builder}
* avoiding the need to create one manually via {@link StartOnDemandReplicationRunRequest#builder()}
*
*
* @param startOnDemandReplicationRunRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.StartOnDemandReplicationRunRequest.Builder} to create a
* request.
* @return Result of the StartOnDemandReplicationRun operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws ReplicationRunLimitExceededException
* You have exceeded the number of on-demand replication runs you can request in a 24-hour period.
* @throws DryRunOperationException
* The user has the required permissions, so the request would have succeeded, but a dry run was performed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StartOnDemandReplicationRun
* @see AWS API Documentation
*/
default StartOnDemandReplicationRunResponse startOnDemandReplicationRun(
Consumer startOnDemandReplicationRunRequest)
throws InvalidParameterException, MissingRequiredParameterException, UnauthorizedOperationException,
OperationNotPermittedException, ReplicationRunLimitExceededException, DryRunOperationException, AwsServiceException,
SdkClientException, SmsException {
return startOnDemandReplicationRun(StartOnDemandReplicationRunRequest.builder()
.applyMutation(startOnDemandReplicationRunRequest).build());
}
/**
*
* Stops replicating the specified application by deleting the replication job for each server in the application.
*
*
* @param stopAppReplicationRequest
* @return Result of the StopAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StopAppReplication
* @see AWS API
* Documentation
*/
default StopAppReplicationResponse stopAppReplication(StopAppReplicationRequest stopAppReplicationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Stops replicating the specified application by deleting the replication job for each server in the application.
*
*
*
* This is a convenience which creates an instance of the {@link StopAppReplicationRequest.Builder} avoiding the
* need to create one manually via {@link StopAppReplicationRequest#builder()}
*
*
* @param stopAppReplicationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.StopAppReplicationRequest.Builder} to create a request.
* @return Result of the StopAppReplication operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.StopAppReplication
* @see AWS API
* Documentation
*/
default StopAppReplicationResponse stopAppReplication(Consumer stopAppReplicationRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return stopAppReplication(StopAppReplicationRequest.builder().applyMutation(stopAppReplicationRequest).build());
}
/**
*
* Terminates the stack for the specified application.
*
*
* @param terminateAppRequest
* @return Result of the TerminateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.TerminateApp
* @see AWS API
* Documentation
*/
default TerminateAppResponse terminateApp(TerminateAppRequest terminateAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Terminates the stack for the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link TerminateAppRequest.Builder} avoiding the need to
* create one manually via {@link TerminateAppRequest#builder()}
*
*
* @param terminateAppRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.TerminateAppRequest.Builder} to create a request.
* @return Result of the TerminateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.TerminateApp
* @see AWS API
* Documentation
*/
default TerminateAppResponse terminateApp(Consumer terminateAppRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return terminateApp(TerminateAppRequest.builder().applyMutation(terminateAppRequest).build());
}
/**
*
* Updates the specified application.
*
*
* @param updateAppRequest
* @return Result of the UpdateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.UpdateApp
* @see AWS API
* Documentation
*/
default UpdateAppResponse updateApp(UpdateAppRequest updateAppRequest) throws UnauthorizedOperationException,
InvalidParameterException, MissingRequiredParameterException, InternalErrorException, OperationNotPermittedException,
AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified application.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAppRequest.Builder} avoiding the need to
* create one manually via {@link UpdateAppRequest#builder()}
*
*
* @param updateAppRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.UpdateAppRequest.Builder} to create a request.
* @return Result of the UpdateApp operation returned by the service.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws InternalErrorException
* An internal error occurred.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.UpdateApp
* @see AWS API
* Documentation
*/
default UpdateAppResponse updateApp(Consumer updateAppRequest)
throws UnauthorizedOperationException, InvalidParameterException, MissingRequiredParameterException,
InternalErrorException, OperationNotPermittedException, AwsServiceException, SdkClientException, SmsException {
return updateApp(UpdateAppRequest.builder().applyMutation(updateAppRequest).build());
}
/**
*
* Updates the specified settings for the specified replication job.
*
*
* @param updateReplicationJobRequest
* @return Result of the UpdateReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws ServerCannotBeReplicatedException
* The specified server cannot be replicated.
* @throws ReplicationJobNotFoundException
* The specified replication job does not exist.
* @throws InternalErrorException
* An internal error occurred.
* @throws TemporarilyUnavailableException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.UpdateReplicationJob
* @see AWS API
* Documentation
*/
default UpdateReplicationJobResponse updateReplicationJob(UpdateReplicationJobRequest updateReplicationJobRequest)
throws InvalidParameterException, MissingRequiredParameterException, OperationNotPermittedException,
UnauthorizedOperationException, ServerCannotBeReplicatedException, ReplicationJobNotFoundException,
InternalErrorException, TemporarilyUnavailableException, AwsServiceException, SdkClientException, SmsException {
throw new UnsupportedOperationException();
}
/**
*
* Updates the specified settings for the specified replication job.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateReplicationJobRequest.Builder} avoiding the
* need to create one manually via {@link UpdateReplicationJobRequest#builder()}
*
*
* @param updateReplicationJobRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.sms.model.UpdateReplicationJobRequest.Builder} to create a request.
* @return Result of the UpdateReplicationJob operation returned by the service.
* @throws InvalidParameterException
* A specified parameter is not valid.
* @throws MissingRequiredParameterException
* A required parameter is missing.
* @throws OperationNotPermittedException
* This operation is not allowed.
* @throws UnauthorizedOperationException
* You lack permissions needed to perform this operation. Check your IAM policies, and ensure that you are
* using the correct access keys.
* @throws ServerCannotBeReplicatedException
* The specified server cannot be replicated.
* @throws ReplicationJobNotFoundException
* The specified replication job does not exist.
* @throws InternalErrorException
* An internal error occurred.
* @throws TemporarilyUnavailableException
* The service is temporarily unavailable.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SmsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SmsClient.UpdateReplicationJob
* @see AWS API
* Documentation
*/
default UpdateReplicationJobResponse updateReplicationJob(
Consumer updateReplicationJobRequest) throws InvalidParameterException,
MissingRequiredParameterException, OperationNotPermittedException, UnauthorizedOperationException,
ServerCannotBeReplicatedException, ReplicationJobNotFoundException, InternalErrorException,
TemporarilyUnavailableException, AwsServiceException, SdkClientException, SmsException {
return updateReplicationJob(UpdateReplicationJobRequest.builder().applyMutation(updateReplicationJobRequest).build());
}
/**
* Create a {@link SmsClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static SmsClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link SmsClient}.
*/
static SmsClientBuilder builder() {
return new DefaultSmsClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default SmsServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}