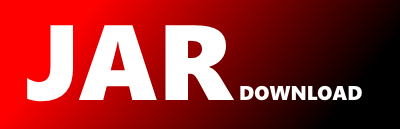
software.amazon.awssdk.services.sns.model.SetTopicAttributesRequest Maven / Gradle / Ivy
Show all versions of sns Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sns.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Input for SetTopicAttributes action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SetTopicAttributesRequest extends SnsRequest implements
ToCopyableBuilder {
private static final SdkField TOPIC_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TopicArn").getter(getter(SetTopicAttributesRequest::topicArn)).setter(setter(Builder::topicArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TopicArn").build()).build();
private static final SdkField ATTRIBUTE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AttributeName").getter(getter(SetTopicAttributesRequest::attributeName))
.setter(setter(Builder::attributeName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AttributeName").build()).build();
private static final SdkField ATTRIBUTE_VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AttributeValue").getter(getter(SetTopicAttributesRequest::attributeValue))
.setter(setter(Builder::attributeValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AttributeValue").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TOPIC_ARN_FIELD,
ATTRIBUTE_NAME_FIELD, ATTRIBUTE_VALUE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String topicArn;
private final String attributeName;
private final String attributeValue;
private SetTopicAttributesRequest(BuilderImpl builder) {
super(builder);
this.topicArn = builder.topicArn;
this.attributeName = builder.attributeName;
this.attributeValue = builder.attributeValue;
}
/**
*
* The ARN of the topic to modify.
*
*
* @return The ARN of the topic to modify.
*/
public final String topicArn() {
return topicArn;
}
/**
*
* A map of attributes with their corresponding values.
*
*
* The following lists the names, descriptions, and values of the special request parameters that the
* SetTopicAttributes
action uses:
*
*
* -
*
* ApplicationSuccessFeedbackRoleArn
– Indicates failed message delivery status for an Amazon SNS topic
* that is subscribed to a platform application endpoint.
*
*
* -
*
* DeliveryPolicy
– The policy that defines how Amazon SNS retries failed deliveries to HTTP/S
* endpoints.
*
*
* -
*
* DisplayName
– The display name to use for a topic with SMS subscriptions.
*
*
* -
*
* Policy
– The policy that defines who can access your topic. By default, only the topic owner can
* publish or subscribe to the topic.
*
*
* -
*
* TracingConfig
– Tracing mode of an Amazon SNS topic. By default TracingConfig
is set to
* PassThrough
, and the topic passes through the tracing header it receives from an Amazon SNS
* publisher to its subscriptions. If set to Active
, Amazon SNS will vend X-Ray segment data to topic
* owner account if the sampled flag in the tracing header is true. This is only supported on standard topics.
*
*
* -
*
* HTTP
*
*
* -
*
* HTTPSuccessFeedbackRoleArn
– Indicates successful message delivery status for an Amazon SNS topic
* that is subscribed to an HTTP endpoint.
*
*
* -
*
* HTTPSuccessFeedbackSampleRate
– Indicates percentage of successful messages to sample for an Amazon
* SNS topic that is subscribed to an HTTP endpoint.
*
*
* -
*
* HTTPFailureFeedbackRoleArn
– Indicates failed message delivery status for an Amazon SNS topic that
* is subscribed to an HTTP endpoint.
*
*
*
*
* -
*
* Amazon Kinesis Data Firehose
*
*
* -
*
* FirehoseSuccessFeedbackRoleArn
– Indicates successful message delivery status for an Amazon SNS
* topic that is subscribed to an Amazon Kinesis Data Firehose endpoint.
*
*
* -
*
* FirehoseSuccessFeedbackSampleRate
– Indicates percentage of successful messages to sample for an
* Amazon SNS topic that is subscribed to an Amazon Kinesis Data Firehose endpoint.
*
*
* -
*
* FirehoseFailureFeedbackRoleArn
– Indicates failed message delivery status for an Amazon SNS topic
* that is subscribed to an Amazon Kinesis Data Firehose endpoint.
*
*
*
*
* -
*
* Lambda
*
*
* -
*
* LambdaSuccessFeedbackRoleArn
– Indicates successful message delivery status for an Amazon SNS topic
* that is subscribed to an Lambda endpoint.
*
*
* -
*
* LambdaSuccessFeedbackSampleRate
– Indicates percentage of successful messages to sample for an
* Amazon SNS topic that is subscribed to an Lambda endpoint.
*
*
* -
*
* LambdaFailureFeedbackRoleArn
– Indicates failed message delivery status for an Amazon SNS topic that
* is subscribed to an Lambda endpoint.
*
*
*
*
* -
*
* Platform application endpoint
*
*
* -
*
* ApplicationSuccessFeedbackRoleArn
– Indicates successful message delivery status for an Amazon SNS
* topic that is subscribed to an Amazon Web Services application endpoint.
*
*
* -
*
* ApplicationSuccessFeedbackSampleRate
– Indicates percentage of successful messages to sample for an
* Amazon SNS topic that is subscribed to an Amazon Web Services application endpoint.
*
*
* -
*
* ApplicationFailureFeedbackRoleArn
– Indicates failed message delivery status for an Amazon SNS topic
* that is subscribed to an Amazon Web Services application endpoint.
*
*
*
*
*
* In addition to being able to configure topic attributes for message delivery status of notification messages sent
* to Amazon SNS application endpoints, you can also configure application attributes for the delivery status of
* push notification messages sent to push notification services.
*
*
* For example, For more information, see Using Amazon SNS Application Attributes for
* Message Delivery Status.
*
*
* -
*
* Amazon SQS
*
*
* -
*
* SQSSuccessFeedbackRoleArn
– Indicates successful message delivery status for an Amazon SNS topic
* that is subscribed to an Amazon SQS endpoint.
*
*
* -
*
* SQSSuccessFeedbackSampleRate
– Indicates percentage of successful messages to sample for an Amazon
* SNS topic that is subscribed to an Amazon SQS endpoint.
*
*
* -
*
* SQSFailureFeedbackRoleArn
– Indicates failed message delivery status for an Amazon SNS topic that is
* subscribed to an Amazon SQS endpoint.
*
*
*
*
*
*
*
* The <ENDPOINT>SuccessFeedbackRoleArn and <ENDPOINT>FailureFeedbackRoleArn attributes are used to give
* Amazon SNS write access to use CloudWatch Logs on your behalf. The <ENDPOINT>SuccessFeedbackSampleRate
* attribute is for specifying the sample rate percentage (0-100) of successfully delivered messages. After you
* configure the <ENDPOINT>FailureFeedbackRoleArn attribute, then all failed message deliveries generate
* CloudWatch Logs.
*
*
*
* The following attribute applies only to server-side-encryption:
*
*
* -
*
* KmsMasterKeyId
– The ID of an Amazon Web Services managed customer master key (CMK) for Amazon SNS
* or a custom CMK. For more information, see Key Terms. For
* more examples, see KeyId in the Key Management Service API Reference.
*
*
* -
*
* SignatureVersion
– The signature version corresponds to the hashing algorithm used while creating
* the signature of the notifications, subscription confirmations, or unsubscribe confirmation messages sent by
* Amazon SNS. By default, SignatureVersion
is set to 1
.
*
*
*
*
* The following attribute applies only to FIFO topics:
*
*
* -
*
* ArchivePolicy
– The policy that sets the retention period for messages stored in the message archive
* of an Amazon SNS FIFO topic.
*
*
* -
*
* ContentBasedDeduplication
– Enables content-based deduplication for FIFO topics.
*
*
* -
*
* By default, ContentBasedDeduplication
is set to false
. If you create a FIFO topic and
* this attribute is false
, you must specify a value for the MessageDeduplicationId
* parameter for the Publish action.
*
*
* -
*
* When you set ContentBasedDeduplication
to true
, Amazon SNS uses a SHA-256 hash to
* generate the MessageDeduplicationId
using the body of the message (but not the attributes of the
* message).
*
*
* (Optional) To override the generated value, you can specify a value for the MessageDeduplicationId
* parameter for the Publish
action.
*
*
*
*
*
*
* @return A map of attributes with their corresponding values.
*
* The following lists the names, descriptions, and values of the special request parameters that the
* SetTopicAttributes
action uses:
*
*
* -
*
* ApplicationSuccessFeedbackRoleArn
– Indicates failed message delivery status for an Amazon
* SNS topic that is subscribed to a platform application endpoint.
*
*
* -
*
* DeliveryPolicy
– The policy that defines how Amazon SNS retries failed deliveries to HTTP/S
* endpoints.
*
*
* -
*
* DisplayName
– The display name to use for a topic with SMS subscriptions.
*
*
* -
*
* Policy
– The policy that defines who can access your topic. By default, only the topic owner
* can publish or subscribe to the topic.
*
*
* -
*
* TracingConfig
– Tracing mode of an Amazon SNS topic. By default TracingConfig
* is set to PassThrough
, and the topic passes through the tracing header it receives from an
* Amazon SNS publisher to its subscriptions. If set to Active
, Amazon SNS will vend X-Ray
* segment data to topic owner account if the sampled flag in the tracing header is true. This is only
* supported on standard topics.
*
*
* -
*
* HTTP
*
*
* -
*
* HTTPSuccessFeedbackRoleArn
– Indicates successful message delivery status for an Amazon SNS
* topic that is subscribed to an HTTP endpoint.
*
*
* -
*
* HTTPSuccessFeedbackSampleRate
– Indicates percentage of successful messages to sample for an
* Amazon SNS topic that is subscribed to an HTTP endpoint.
*
*
* -
*
* HTTPFailureFeedbackRoleArn
– Indicates failed message delivery status for an Amazon SNS
* topic that is subscribed to an HTTP endpoint.
*
*
*
*
* -
*
* Amazon Kinesis Data Firehose
*
*
* -
*
* FirehoseSuccessFeedbackRoleArn
– Indicates successful message delivery status for an Amazon
* SNS topic that is subscribed to an Amazon Kinesis Data Firehose endpoint.
*
*
* -
*
* FirehoseSuccessFeedbackSampleRate
– Indicates percentage of successful messages to sample
* for an Amazon SNS topic that is subscribed to an Amazon Kinesis Data Firehose endpoint.
*
*
* -
*
* FirehoseFailureFeedbackRoleArn
– Indicates failed message delivery status for an Amazon SNS
* topic that is subscribed to an Amazon Kinesis Data Firehose endpoint.
*
*
*
*
* -
*
* Lambda
*
*
* -
*
* LambdaSuccessFeedbackRoleArn
– Indicates successful message delivery status for an Amazon
* SNS topic that is subscribed to an Lambda endpoint.
*
*
* -
*
* LambdaSuccessFeedbackSampleRate
– Indicates percentage of successful messages to sample for
* an Amazon SNS topic that is subscribed to an Lambda endpoint.
*
*
* -
*
* LambdaFailureFeedbackRoleArn
– Indicates failed message delivery status for an Amazon SNS
* topic that is subscribed to an Lambda endpoint.
*
*
*
*
* -
*
* Platform application endpoint
*
*
* -
*
* ApplicationSuccessFeedbackRoleArn
– Indicates successful message delivery status for an
* Amazon SNS topic that is subscribed to an Amazon Web Services application endpoint.
*
*
* -
*
* ApplicationSuccessFeedbackSampleRate
– Indicates percentage of successful messages to sample
* for an Amazon SNS topic that is subscribed to an Amazon Web Services application endpoint.
*
*
* -
*
* ApplicationFailureFeedbackRoleArn
– Indicates failed message delivery status for an Amazon
* SNS topic that is subscribed to an Amazon Web Services application endpoint.
*
*
*
*
*
* In addition to being able to configure topic attributes for message delivery status of notification
* messages sent to Amazon SNS application endpoints, you can also configure application attributes for the
* delivery status of push notification messages sent to push notification services.
*
*
* For example, For more information, see Using Amazon SNS Application
* Attributes for Message Delivery Status.
*
*
* -
*
* Amazon SQS
*
*
* -
*
* SQSSuccessFeedbackRoleArn
– Indicates successful message delivery status for an Amazon SNS
* topic that is subscribed to an Amazon SQS endpoint.
*
*
* -
*
* SQSSuccessFeedbackSampleRate
– Indicates percentage of successful messages to sample for an
* Amazon SNS topic that is subscribed to an Amazon SQS endpoint.
*
*
* -
*
* SQSFailureFeedbackRoleArn
– Indicates failed message delivery status for an Amazon SNS topic
* that is subscribed to an Amazon SQS endpoint.
*
*
*
*
*
*
*
* The <ENDPOINT>SuccessFeedbackRoleArn and <ENDPOINT>FailureFeedbackRoleArn attributes are used
* to give Amazon SNS write access to use CloudWatch Logs on your behalf. The
* <ENDPOINT>SuccessFeedbackSampleRate attribute is for specifying the sample rate percentage (0-100)
* of successfully delivered messages. After you configure the <ENDPOINT>FailureFeedbackRoleArn
* attribute, then all failed message deliveries generate CloudWatch Logs.
*
*
*
* The following attribute applies only to server-side-encryption:
*
*
* -
*
* KmsMasterKeyId
– The ID of an Amazon Web Services managed customer master key (CMK) for
* Amazon SNS or a custom CMK. For more information, see Key
* Terms. For more examples, see KeyId in the Key Management Service API Reference.
*
*
* -
*
* SignatureVersion
– The signature version corresponds to the hashing algorithm used while
* creating the signature of the notifications, subscription confirmations, or unsubscribe confirmation
* messages sent by Amazon SNS. By default, SignatureVersion
is set to 1
.
*
*
*
*
* The following attribute applies only to FIFO topics:
*
*
* -
*
* ArchivePolicy
– The policy that sets the retention period for messages stored in the message
* archive of an Amazon SNS FIFO topic.
*
*
* -
*
* ContentBasedDeduplication
– Enables content-based deduplication for FIFO topics.
*
*
* -
*
* By default, ContentBasedDeduplication
is set to false
. If you create a FIFO
* topic and this attribute is false
, you must specify a value for the
* MessageDeduplicationId
parameter for the Publish action.
*
*
* -
*
* When you set ContentBasedDeduplication
to true
, Amazon SNS uses a SHA-256 hash
* to generate the MessageDeduplicationId
using the body of the message (but not the attributes
* of the message).
*
*
* (Optional) To override the generated value, you can specify a value for the
* MessageDeduplicationId
parameter for the Publish
action.
*
*
*
*
*/
public final String attributeName() {
return attributeName;
}
/**
*
* The new value for the attribute.
*
*
* @return The new value for the attribute.
*/
public final String attributeValue() {
return attributeValue;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(topicArn());
hashCode = 31 * hashCode + Objects.hashCode(attributeName());
hashCode = 31 * hashCode + Objects.hashCode(attributeValue());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SetTopicAttributesRequest)) {
return false;
}
SetTopicAttributesRequest other = (SetTopicAttributesRequest) obj;
return Objects.equals(topicArn(), other.topicArn()) && Objects.equals(attributeName(), other.attributeName())
&& Objects.equals(attributeValue(), other.attributeValue());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SetTopicAttributesRequest").add("TopicArn", topicArn()).add("AttributeName", attributeName())
.add("AttributeValue", attributeValue()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TopicArn":
return Optional.ofNullable(clazz.cast(topicArn()));
case "AttributeName":
return Optional.ofNullable(clazz.cast(attributeName()));
case "AttributeValue":
return Optional.ofNullable(clazz.cast(attributeValue()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("TopicArn", TOPIC_ARN_FIELD);
map.put("AttributeName", ATTRIBUTE_NAME_FIELD);
map.put("AttributeValue", ATTRIBUTE_VALUE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function