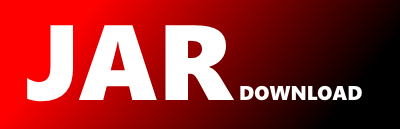
software.amazon.awssdk.services.sqs.DefaultSqsAsyncClient Maven / Gradle / Ivy
Show all versions of sqs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sqs;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
import java.util.function.Function;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.sqs.batchmanager.SqsAsyncBatchManager;
import software.amazon.awssdk.services.sqs.internal.SqsServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.sqs.model.AddPermissionRequest;
import software.amazon.awssdk.services.sqs.model.AddPermissionResponse;
import software.amazon.awssdk.services.sqs.model.BatchEntryIdsNotDistinctException;
import software.amazon.awssdk.services.sqs.model.BatchRequestTooLongException;
import software.amazon.awssdk.services.sqs.model.CancelMessageMoveTaskRequest;
import software.amazon.awssdk.services.sqs.model.CancelMessageMoveTaskResponse;
import software.amazon.awssdk.services.sqs.model.ChangeMessageVisibilityBatchRequest;
import software.amazon.awssdk.services.sqs.model.ChangeMessageVisibilityBatchResponse;
import software.amazon.awssdk.services.sqs.model.ChangeMessageVisibilityRequest;
import software.amazon.awssdk.services.sqs.model.ChangeMessageVisibilityResponse;
import software.amazon.awssdk.services.sqs.model.CreateQueueRequest;
import software.amazon.awssdk.services.sqs.model.CreateQueueResponse;
import software.amazon.awssdk.services.sqs.model.DeleteMessageBatchRequest;
import software.amazon.awssdk.services.sqs.model.DeleteMessageBatchResponse;
import software.amazon.awssdk.services.sqs.model.DeleteMessageRequest;
import software.amazon.awssdk.services.sqs.model.DeleteMessageResponse;
import software.amazon.awssdk.services.sqs.model.DeleteQueueRequest;
import software.amazon.awssdk.services.sqs.model.DeleteQueueResponse;
import software.amazon.awssdk.services.sqs.model.EmptyBatchRequestException;
import software.amazon.awssdk.services.sqs.model.GetQueueAttributesRequest;
import software.amazon.awssdk.services.sqs.model.GetQueueAttributesResponse;
import software.amazon.awssdk.services.sqs.model.GetQueueUrlRequest;
import software.amazon.awssdk.services.sqs.model.GetQueueUrlResponse;
import software.amazon.awssdk.services.sqs.model.InvalidAddressException;
import software.amazon.awssdk.services.sqs.model.InvalidAttributeNameException;
import software.amazon.awssdk.services.sqs.model.InvalidAttributeValueException;
import software.amazon.awssdk.services.sqs.model.InvalidBatchEntryIdException;
import software.amazon.awssdk.services.sqs.model.InvalidIdFormatException;
import software.amazon.awssdk.services.sqs.model.InvalidMessageContentsException;
import software.amazon.awssdk.services.sqs.model.InvalidSecurityException;
import software.amazon.awssdk.services.sqs.model.KmsAccessDeniedException;
import software.amazon.awssdk.services.sqs.model.KmsDisabledException;
import software.amazon.awssdk.services.sqs.model.KmsInvalidKeyUsageException;
import software.amazon.awssdk.services.sqs.model.KmsInvalidStateException;
import software.amazon.awssdk.services.sqs.model.KmsNotFoundException;
import software.amazon.awssdk.services.sqs.model.KmsOptInRequiredException;
import software.amazon.awssdk.services.sqs.model.KmsThrottledException;
import software.amazon.awssdk.services.sqs.model.ListDeadLetterSourceQueuesRequest;
import software.amazon.awssdk.services.sqs.model.ListDeadLetterSourceQueuesResponse;
import software.amazon.awssdk.services.sqs.model.ListMessageMoveTasksRequest;
import software.amazon.awssdk.services.sqs.model.ListMessageMoveTasksResponse;
import software.amazon.awssdk.services.sqs.model.ListQueueTagsRequest;
import software.amazon.awssdk.services.sqs.model.ListQueueTagsResponse;
import software.amazon.awssdk.services.sqs.model.ListQueuesRequest;
import software.amazon.awssdk.services.sqs.model.ListQueuesResponse;
import software.amazon.awssdk.services.sqs.model.MessageNotInflightException;
import software.amazon.awssdk.services.sqs.model.OverLimitException;
import software.amazon.awssdk.services.sqs.model.PurgeQueueInProgressException;
import software.amazon.awssdk.services.sqs.model.PurgeQueueRequest;
import software.amazon.awssdk.services.sqs.model.PurgeQueueResponse;
import software.amazon.awssdk.services.sqs.model.QueueDeletedRecentlyException;
import software.amazon.awssdk.services.sqs.model.QueueDoesNotExistException;
import software.amazon.awssdk.services.sqs.model.QueueNameExistsException;
import software.amazon.awssdk.services.sqs.model.ReceiptHandleIsInvalidException;
import software.amazon.awssdk.services.sqs.model.ReceiveMessageRequest;
import software.amazon.awssdk.services.sqs.model.ReceiveMessageResponse;
import software.amazon.awssdk.services.sqs.model.RemovePermissionRequest;
import software.amazon.awssdk.services.sqs.model.RemovePermissionResponse;
import software.amazon.awssdk.services.sqs.model.RequestThrottledException;
import software.amazon.awssdk.services.sqs.model.ResourceNotFoundException;
import software.amazon.awssdk.services.sqs.model.SendMessageBatchRequest;
import software.amazon.awssdk.services.sqs.model.SendMessageBatchResponse;
import software.amazon.awssdk.services.sqs.model.SendMessageRequest;
import software.amazon.awssdk.services.sqs.model.SendMessageResponse;
import software.amazon.awssdk.services.sqs.model.SetQueueAttributesRequest;
import software.amazon.awssdk.services.sqs.model.SetQueueAttributesResponse;
import software.amazon.awssdk.services.sqs.model.SqsException;
import software.amazon.awssdk.services.sqs.model.StartMessageMoveTaskRequest;
import software.amazon.awssdk.services.sqs.model.StartMessageMoveTaskResponse;
import software.amazon.awssdk.services.sqs.model.TagQueueRequest;
import software.amazon.awssdk.services.sqs.model.TagQueueResponse;
import software.amazon.awssdk.services.sqs.model.TooManyEntriesInBatchRequestException;
import software.amazon.awssdk.services.sqs.model.UnsupportedOperationException;
import software.amazon.awssdk.services.sqs.model.UntagQueueRequest;
import software.amazon.awssdk.services.sqs.model.UntagQueueResponse;
import software.amazon.awssdk.services.sqs.transform.AddPermissionRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.CancelMessageMoveTaskRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.ChangeMessageVisibilityBatchRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.ChangeMessageVisibilityRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.CreateQueueRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.DeleteMessageBatchRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.DeleteMessageRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.DeleteQueueRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.GetQueueAttributesRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.GetQueueUrlRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.ListDeadLetterSourceQueuesRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.ListMessageMoveTasksRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.ListQueueTagsRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.ListQueuesRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.PurgeQueueRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.ReceiveMessageRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.RemovePermissionRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.SendMessageBatchRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.SendMessageRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.SetQueueAttributesRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.StartMessageMoveTaskRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.TagQueueRequestMarshaller;
import software.amazon.awssdk.services.sqs.transform.UntagQueueRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link SqsAsyncClient}.
*
* @see SqsAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultSqsAsyncClient implements SqsAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultSqsAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultSqsAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
/**
*
* Adds a permission to a queue for a specific principal. This allows sharing access
* to the queue.
*
*
* When you create a queue, you have full control access rights for the queue. Only you, the owner of the queue, can
* grant or deny permissions to the queue. For more information about these permissions, see Allow Developers to Write Messages to a Shared Queue in the Amazon SQS Developer Guide.
*
*
*
* -
*
* AddPermission
generates a policy for you. You can use SetQueueAttributes
to
* upload your policy. For more information, see Using Custom Policies with the Amazon SQS Access Policy Language in the Amazon SQS Developer Guide.
*
*
* -
*
* An Amazon SQS policy can have a maximum of seven actions per statement.
*
*
* -
*
* To remove the ability to change queue permissions, you must deny permission to the AddPermission
,
* RemovePermission
, and SetQueueAttributes
actions in your IAM policy.
*
*
* -
*
* Amazon SQS AddPermission
does not support adding a non-account principal.
*
*
*
*
*
* Cross-account permissions don't apply to this action. For more information, see Grant cross-account permissions to a role and a username in the Amazon SQS Developer Guide.
*
*
*
* @param addPermissionRequest
* @return A Java Future containing the result of the AddPermission operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - OverLimitException The specified action violates a limit. For example,
ReceiveMessage
* returns this error if the maximum number of in flight messages is reached and AddPermission
* returns this error if the maximum number of permissions for the queue is reached.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.AddPermission
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture addPermission(AddPermissionRequest addPermissionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addPermissionRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addPermissionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddPermission");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AddPermissionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddPermission").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AddPermissionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(addPermissionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels a specified message movement task. A message movement can only be cancelled when the current status is
* RUNNING. Cancelling a message movement task does not revert the messages that have already been moved. It can
* only stop the messages that have not been moved yet.
*
*
*
* -
*
* This action is currently limited to supporting message redrive from dead-letter queues (DLQs) only. In this context, the source queue is the dead-letter queue (DLQ), while the
* destination queue can be the original source queue (from which the messages were driven to the
* dead-letter-queue), or a custom destination queue.
*
*
* -
*
* Only one active message movement task is supported per queue at any given time.
*
*
*
*
*
* @param cancelMessageMoveTaskRequest
* @return A Java Future containing the result of the CancelMessageMoveTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException One or more specified resources don't exist.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.CancelMessageMoveTask
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture cancelMessageMoveTask(
CancelMessageMoveTaskRequest cancelMessageMoveTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelMessageMoveTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelMessageMoveTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelMessageMoveTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelMessageMoveTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelMessageMoveTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelMessageMoveTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelMessageMoveTaskRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Changes the visibility timeout of a specified message in a queue to a new value. The default visibility timeout
* for a message is 30 seconds. The minimum is 0 seconds. The maximum is 12 hours. For more information, see
* Visibility Timeout in the Amazon SQS Developer Guide.
*
*
* For example, if the default timeout for a queue is 60 seconds, 15 seconds have elapsed since you received the
* message, and you send a ChangeMessageVisibility call with VisibilityTimeout
set to 10 seconds, the
* 10 seconds begin to count from the time that you make the ChangeMessageVisibility
call. Thus, any
* attempt to change the visibility timeout or to delete that message 10 seconds after you initially change the
* visibility timeout (a total of 25 seconds) might result in an error.
*
*
* An Amazon SQS message has three basic states:
*
*
* -
*
* Sent to a queue by a producer.
*
*
* -
*
* Received from the queue by a consumer.
*
*
* -
*
* Deleted from the queue.
*
*
*
*
* A message is considered to be stored after it is sent to a queue by a producer, but not yet received from
* the queue by a consumer (that is, between states 1 and 2). There is no limit to the number of stored messages. A
* message is considered to be in flight after it is received from a queue by a consumer, but not yet deleted
* from the queue (that is, between states 2 and 3). There is a limit to the number of in flight messages.
*
*
* Limits that apply to in flight messages are unrelated to the unlimited number of stored messages.
*
*
* For most standard queues (depending on queue traffic and message backlog), there can be a maximum of
* approximately 120,000 in flight messages (received from a queue by a consumer, but not yet deleted from the
* queue). If you reach this limit, Amazon SQS returns the OverLimit
error message. To avoid reaching
* the limit, you should delete messages from the queue after they're processed. You can also increase the number of
* queues you use to process your messages. To request a limit increase, file a support request.
*
*
* For FIFO queues, there can be a maximum of 20,000 in flight messages (received from a queue by a consumer, but
* not yet deleted from the queue). If you reach this limit, Amazon SQS returns no error messages.
*
*
*
* If you attempt to set the VisibilityTimeout
to a value greater than the maximum time left, Amazon
* SQS returns an error. Amazon SQS doesn't automatically recalculate and increase the timeout to the maximum
* remaining time.
*
*
* Unlike with a queue, when you change the visibility timeout for a specific message the timeout value is applied
* immediately but isn't saved in memory for that message. If you don't delete a message after it is received, the
* visibility timeout for the message reverts to the original timeout value (not to the value you set using the
* ChangeMessageVisibility
action) the next time the message is received.
*
*
*
* @param changeMessageVisibilityRequest
* @return A Java Future containing the result of the ChangeMessageVisibility operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - MessageNotInflightException The specified message isn't in flight.
* - ReceiptHandleIsInvalidException The specified receipt handle isn't valid.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.ChangeMessageVisibility
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture changeMessageVisibility(
ChangeMessageVisibilityRequest changeMessageVisibilityRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(changeMessageVisibilityRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, changeMessageVisibilityRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ChangeMessageVisibility");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ChangeMessageVisibilityResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ChangeMessageVisibility").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ChangeMessageVisibilityRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(changeMessageVisibilityRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Changes the visibility timeout of multiple messages. This is a batch version of
* ChangeMessageVisibility.
The result of the action on each message is reported individually
* in the response. You can send up to 10 ChangeMessageVisibility
requests with each
* ChangeMessageVisibilityBatch
action.
*
*
*
* Because the batch request can result in a combination of successful and unsuccessful actions, you should check
* for batch errors even when the call returns an HTTP status code of 200
.
*
*
*
* @param changeMessageVisibilityBatchRequest
* @return A Java Future containing the result of the ChangeMessageVisibilityBatch operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyEntriesInBatchRequestException The batch request contains more entries than permissible.
* - EmptyBatchRequestException The batch request doesn't contain any entries.
* - BatchEntryIdsNotDistinctException Two or more batch entries in the request have the same
*
Id
.
* - InvalidBatchEntryIdException The
Id
of a batch entry in a batch request doesn't abide by
* the specification.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.ChangeMessageVisibilityBatch
* @see AWS API Documentation
*/
@Override
public CompletableFuture changeMessageVisibilityBatch(
ChangeMessageVisibilityBatchRequest changeMessageVisibilityBatchRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(changeMessageVisibilityBatchRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, changeMessageVisibilityBatchRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ChangeMessageVisibilityBatch");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ChangeMessageVisibilityBatchResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ChangeMessageVisibilityBatch").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ChangeMessageVisibilityBatchRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(changeMessageVisibilityBatchRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new standard or FIFO queue. You can pass one or more attributes in the request. Keep the following in
* mind:
*
*
* -
*
* If you don't specify the FifoQueue
attribute, Amazon SQS creates a standard queue.
*
*
*
* You can't change the queue type after you create it and you can't convert an existing standard queue into a FIFO
* queue. You must either create a new FIFO queue for your application or delete your existing standard queue and
* recreate it as a FIFO queue. For more information, see Moving From a Standard Queue to a FIFO Queue in the Amazon SQS Developer Guide.
*
*
* -
*
* If you don't provide a value for an attribute, the queue is created with the default value for the attribute.
*
*
* -
*
* If you delete a queue, you must wait at least 60 seconds before creating a queue with the same name.
*
*
*
*
* To successfully create a new queue, you must provide a queue name that adheres to the limits
* related to queues and is unique within the scope of your queues.
*
*
*
* After you create a queue, you must wait at least one second after the queue is created to be able to use the
* queue.
*
*
*
* To get the queue URL, use the GetQueueUrl
action. GetQueueUrl
* requires only the QueueName
parameter. be aware of existing queue names:
*
*
* -
*
* If you provide the name of an existing queue along with the exact names and values of all the queue's attributes,
* CreateQueue
returns the queue URL for the existing queue.
*
*
* -
*
* If the queue name, attribute names, or attribute values don't match an existing queue, CreateQueue
* returns an error.
*
*
*
*
*
* Cross-account permissions don't apply to this action. For more information, see Grant cross-account permissions to a role and a username in the Amazon SQS Developer Guide.
*
*
*
* @param createQueueRequest
* @return A Java Future containing the result of the CreateQueue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - QueueDeletedRecentlyException You must wait 60 seconds after deleting a queue before you can create
* another queue with the same name.
* - QueueNameExistsException A queue with this name already exists. Amazon SQS returns this error only if
* the request includes attributes whose values differ from those of the existing queue.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - InvalidAddressException The
accountId
is invalid.
* - InvalidAttributeNameException The specified attribute doesn't exist.
* - InvalidAttributeValueException A queue attribute value is invalid.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.CreateQueue
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createQueue(CreateQueueRequest createQueueRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createQueueRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createQueueRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateQueue");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateQueueResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateQueue").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateQueueRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createQueueRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified message from the specified queue. To select the message to delete, use the
* ReceiptHandle
of the message (not the MessageId
which you receive when you send
* the message). Amazon SQS can delete a message from a queue even if a visibility timeout setting causes the
* message to be locked by another consumer. Amazon SQS automatically deletes messages left in a queue longer than
* the retention period configured for the queue.
*
*
*
* The ReceiptHandle
is associated with a specific instance of receiving a message. If you
* receive a message more than once, the ReceiptHandle
is different each time you receive a message.
* When you use the DeleteMessage
action, you must provide the most recently received
* ReceiptHandle
for the message (otherwise, the request succeeds, but the message will not be
* deleted).
*
*
* For standard queues, it is possible to receive a message even after you delete it. This might happen on rare
* occasions if one of the servers which stores a copy of the message is unavailable when you send the request to
* delete the message. The copy remains on the server and might be returned to you during a subsequent receive
* request. You should ensure that your application is idempotent, so that receiving a message more than once does
* not cause issues.
*
*
*
* @param deleteMessageRequest
* @return A Java Future containing the result of the DeleteMessage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidIdFormatException The specified receipt handle isn't valid for the current version.
* - ReceiptHandleIsInvalidException The specified receipt handle isn't valid.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - InvalidAddressException The
accountId
is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.DeleteMessage
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteMessage(DeleteMessageRequest deleteMessageRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteMessageRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteMessageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteMessage");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteMessageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteMessage").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteMessageRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteMessageRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes up to ten messages from the specified queue. This is a batch version of
* DeleteMessage.
The result of the action on each message is reported individually in the
* response.
*
*
*
* Because the batch request can result in a combination of successful and unsuccessful actions, you should check
* for batch errors even when the call returns an HTTP status code of 200
.
*
*
*
* @param deleteMessageBatchRequest
* @return A Java Future containing the result of the DeleteMessageBatch operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyEntriesInBatchRequestException The batch request contains more entries than permissible.
* - EmptyBatchRequestException The batch request doesn't contain any entries.
* - BatchEntryIdsNotDistinctException Two or more batch entries in the request have the same
*
Id
.
* - InvalidBatchEntryIdException The
Id
of a batch entry in a batch request doesn't abide by
* the specification.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.DeleteMessageBatch
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteMessageBatch(DeleteMessageBatchRequest deleteMessageBatchRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteMessageBatchRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteMessageBatchRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteMessageBatch");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteMessageBatchResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteMessageBatch").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteMessageBatchRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteMessageBatchRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the queue specified by the QueueUrl
, regardless of the queue's contents.
*
*
*
* Be careful with the DeleteQueue
action: When you delete a queue, any messages in the queue are no
* longer available.
*
*
*
* When you delete a queue, the deletion process takes up to 60 seconds. Requests you send involving that queue
* during the 60 seconds might succeed. For example, a SendMessage
request might succeed, but
* after 60 seconds the queue and the message you sent no longer exist.
*
*
* When you delete a queue, you must wait at least 60 seconds before creating a queue with the same name.
*
*
*
* Cross-account permissions don't apply to this action. For more information, see Grant cross-account permissions to a role and a username in the Amazon SQS Developer Guide.
*
*
* The delete operation uses the HTTP GET
verb.
*
*
*
* @param deleteQueueRequest
* @return A Java Future containing the result of the DeleteQueue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - InvalidAddressException The
accountId
is invalid.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.DeleteQueue
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteQueue(DeleteQueueRequest deleteQueueRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteQueueRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteQueueRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteQueue");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteQueueResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteQueue").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteQueueRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteQueueRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets attributes for the specified queue.
*
*
*
* To determine whether a queue is FIFO, you
* can check whether QueueName
ends with the .fifo
suffix.
*
*
*
* @param getQueueAttributesRequest
* @return A Java Future containing the result of the GetQueueAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidAttributeNameException The specified attribute doesn't exist.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - InvalidAddressException The
accountId
is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.GetQueueAttributes
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getQueueAttributes(GetQueueAttributesRequest getQueueAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getQueueAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getQueueAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetQueueAttributes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetQueueAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetQueueAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetQueueAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getQueueAttributesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the URL of an existing Amazon SQS queue.
*
*
* To access a queue that belongs to another AWS account, use the QueueOwnerAWSAccountId
parameter to
* specify the account ID of the queue's owner. The queue's owner must grant you permission to access the queue. For
* more information about shared queue access, see AddPermission
or see Allow Developers to Write Messages to a Shared Queue in the Amazon SQS Developer Guide.
*
*
* @param getQueueUrlRequest
* @return A Java Future containing the result of the GetQueueUrl operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.GetQueueUrl
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getQueueUrl(GetQueueUrlRequest getQueueUrlRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getQueueUrlRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getQueueUrlRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetQueueUrl");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetQueueUrlResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetQueueUrl").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetQueueUrlRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getQueueUrlRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of your queues that have the RedrivePolicy
queue attribute configured with a
* dead-letter queue.
*
*
* The ListDeadLetterSourceQueues
methods supports pagination. Set parameter MaxResults
in
* the request to specify the maximum number of results to be returned in the response. If you do not set
* MaxResults
, the response includes a maximum of 1,000 results. If you set MaxResults
and
* there are additional results to display, the response includes a value for NextToken
. Use
* NextToken
as a parameter in your next request to ListDeadLetterSourceQueues
to receive
* the next page of results.
*
*
* For more information about using dead-letter queues, see Using Amazon SQS Dead-Letter Queues in the Amazon SQS Developer Guide.
*
*
* @param listDeadLetterSourceQueuesRequest
* @return A Java Future containing the result of the ListDeadLetterSourceQueues operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - InvalidAddressException The
accountId
is invalid.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.ListDeadLetterSourceQueues
* @see AWS API Documentation
*/
@Override
public CompletableFuture listDeadLetterSourceQueues(
ListDeadLetterSourceQueuesRequest listDeadLetterSourceQueuesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDeadLetterSourceQueuesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDeadLetterSourceQueuesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDeadLetterSourceQueues");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDeadLetterSourceQueuesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDeadLetterSourceQueues").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListDeadLetterSourceQueuesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listDeadLetterSourceQueuesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the most recent message movement tasks (up to 10) under a specific source queue.
*
*
*
* -
*
* This action is currently limited to supporting message redrive from dead-letter queues (DLQs) only. In this context, the source queue is the dead-letter queue (DLQ), while the
* destination queue can be the original source queue (from which the messages were driven to the
* dead-letter-queue), or a custom destination queue.
*
*
* -
*
* Only one active message movement task is supported per queue at any given time.
*
*
*
*
*
* @param listMessageMoveTasksRequest
* @return A Java Future containing the result of the ListMessageMoveTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException One or more specified resources don't exist.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.ListMessageMoveTasks
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listMessageMoveTasks(
ListMessageMoveTasksRequest listMessageMoveTasksRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listMessageMoveTasksRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMessageMoveTasksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMessageMoveTasks");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListMessageMoveTasksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListMessageMoveTasks").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListMessageMoveTasksRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listMessageMoveTasksRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* List all cost allocation tags added to the specified Amazon SQS queue. For an overview, see Tagging
* Your Amazon SQS Queues in the Amazon SQS Developer Guide.
*
*
*
* Cross-account permissions don't apply to this action. For more information, see Grant cross-account permissions to a role and a username in the Amazon SQS Developer Guide.
*
*
*
* @param listQueueTagsRequest
* @return A Java Future containing the result of the ListQueueTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.ListQueueTags
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listQueueTags(ListQueueTagsRequest listQueueTagsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listQueueTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listQueueTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListQueueTags");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListQueueTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListQueueTags").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListQueueTagsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listQueueTagsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of your queues in the current region. The response includes a maximum of 1,000 results. If you
* specify a value for the optional QueueNamePrefix
parameter, only queues with a name that begins with
* the specified value are returned.
*
*
* The listQueues
methods supports pagination. Set parameter MaxResults
in the request to
* specify the maximum number of results to be returned in the response. If you do not set MaxResults
,
* the response includes a maximum of 1,000 results. If you set MaxResults
and there are additional
* results to display, the response includes a value for NextToken
. Use NextToken
as a
* parameter in your next request to listQueues
to receive the next page of results.
*
*
*
* Cross-account permissions don't apply to this action. For more information, see Grant cross-account permissions to a role and a username in the Amazon SQS Developer Guide.
*
*
*
* @param listQueuesRequest
* @return A Java Future containing the result of the ListQueues operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - InvalidAddressException The
accountId
is invalid.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.ListQueues
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listQueues(ListQueuesRequest listQueuesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listQueuesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listQueuesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListQueues");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListQueuesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListQueues")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListQueuesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listQueuesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes available messages in a queue (including in-flight messages) specified by the QueueURL
* parameter.
*
*
*
* When you use the PurgeQueue
action, you can't retrieve any messages deleted from a queue.
*
*
* The message deletion process takes up to 60 seconds. We recommend waiting for 60 seconds regardless of your
* queue's size.
*
*
*
* Messages sent to the queue before you call PurgeQueue
might be received but are deleted
* within the next minute.
*
*
* Messages sent to the queue after you call PurgeQueue
might be deleted while the queue is
* being purged.
*
*
* @param purgeQueueRequest
* @return A Java Future containing the result of the PurgeQueue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - PurgeQueueInProgressException Indicates that the specified queue previously received a
*
PurgeQueue
request within the last 60 seconds (the time it can take to delete the messages
* in the queue).
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.PurgeQueue
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture purgeQueue(PurgeQueueRequest purgeQueueRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(purgeQueueRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, purgeQueueRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PurgeQueue");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PurgeQueueResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("PurgeQueue")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new PurgeQueueRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(purgeQueueRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves one or more messages (up to 10), from the specified queue. Using the WaitTimeSeconds
* parameter enables long-poll support. For more information, see Amazon
* SQS Long Polling in the Amazon SQS Developer Guide.
*
*
* Short poll is the default behavior where a weighted random set of machines is sampled on a
* ReceiveMessage
call. Thus, only the messages on the sampled machines are returned. If the number of
* messages in the queue is small (fewer than 1,000), you most likely get fewer messages than you requested per
* ReceiveMessage
call. If the number of messages in the queue is extremely small, you might not
* receive any messages in a particular ReceiveMessage
response. If this happens, repeat the request.
*
*
* For each message returned, the response includes the following:
*
*
* -
*
* The message body.
*
*
* -
*
* An MD5 digest of the message body. For information about MD5, see RFC1321.
*
*
* -
*
* The MessageId
you received when you sent the message to the queue.
*
*
* -
*
* The receipt handle.
*
*
* -
*
* The message attributes.
*
*
* -
*
* An MD5 digest of the message attributes.
*
*
*
*
* The receipt handle is the identifier you must provide when deleting the message. For more information, see Queue and Message Identifiers in the Amazon SQS Developer Guide.
*
*
* You can provide the VisibilityTimeout
parameter in your request. The parameter is applied to the
* messages that Amazon SQS returns in the response. If you don't include the parameter, the overall visibility
* timeout for the queue is used for the returned messages. For more information, see Visibility Timeout in the Amazon SQS Developer Guide.
*
*
* A message that isn't deleted or a message whose visibility isn't extended before the visibility timeout expires
* counts as a failed receive. Depending on the configuration of the queue, the message might be sent to the
* dead-letter queue.
*
*
*
* In the future, new attributes might be added. If you write code that calls this action, we recommend that you
* structure your code so that it can handle new attributes gracefully.
*
*
*
* @param receiveMessageRequest
* @return A Java Future containing the result of the ReceiveMessage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - OverLimitException The specified action violates a limit. For example,
ReceiveMessage
* returns this error if the maximum number of in flight messages is reached and AddPermission
* returns this error if the maximum number of permissions for the queue is reached.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - KmsDisabledException The request was denied due to request throttling.
* - KmsInvalidStateException The request was rejected because the state of the specified resource is not
* valid for this request.
* - KmsNotFoundException The request was rejected because the specified entity or resource could not be
* found.
* - KmsOptInRequiredException The request was rejected because the specified key policy isn't
* syntactically or semantically correct.
* - KmsThrottledException Amazon Web Services KMS throttles requests for the following conditions.
* - KmsAccessDeniedException The caller doesn't have the required KMS access.
* - KmsInvalidKeyUsageException The request was rejected for one of the following reasons:
*
* -
*
* The KeyUsage value of the KMS key is incompatible with the API operation.
*
*
* -
*
* The encryption algorithm or signing algorithm specified for the operation is incompatible with the type
* of key material in the KMS key (KeySpec).
*
*
* - InvalidAddressException The
accountId
is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.ReceiveMessage
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture receiveMessage(ReceiveMessageRequest receiveMessageRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(receiveMessageRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, receiveMessageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ReceiveMessage");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ReceiveMessageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ReceiveMessage").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ReceiveMessageRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(receiveMessageRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Revokes any permissions in the queue policy that matches the specified Label
parameter.
*
*
*
* -
*
* Only the owner of a queue can remove permissions from it.
*
*
* -
*
* Cross-account permissions don't apply to this action. For more information, see Grant cross-account permissions to a role and a username in the Amazon SQS Developer Guide.
*
*
* -
*
* To remove the ability to change queue permissions, you must deny permission to the AddPermission
,
* RemovePermission
, and SetQueueAttributes
actions in your IAM policy.
*
*
*
*
*
* @param removePermissionRequest
* @return A Java Future containing the result of the RemovePermission operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidAddressException The
accountId
is invalid.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.RemovePermission
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture removePermission(RemovePermissionRequest removePermissionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(removePermissionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, removePermissionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RemovePermission");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemovePermissionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemovePermission").withProtocolMetadata(protocolMetadata)
.withMarshaller(new RemovePermissionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(removePermissionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Delivers a message to the specified queue.
*
*
*
* A message can include only XML, JSON, and unformatted text. The following Unicode characters are allowed. For
* more information, see the W3C specification for characters.
*
*
* #x9
| #xA
| #xD
| #x20
to #xD7FF
|
* #xE000
to #xFFFD
| #x10000
to #x10FFFF
*
*
* Amazon SQS does not throw an exception or completely reject the message if it contains invalid characters.
* Instead, it replaces those invalid characters with U+FFFD
before storing the message in the queue,
* as long as the message body contains at least one valid character.
*
*
*
* @param sendMessageRequest
* @return A Java Future containing the result of the SendMessage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidMessageContentsException The message contains characters outside the allowed set.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - KmsDisabledException The request was denied due to request throttling.
* - KmsInvalidStateException The request was rejected because the state of the specified resource is not
* valid for this request.
* - KmsNotFoundException The request was rejected because the specified entity or resource could not be
* found.
* - KmsOptInRequiredException The request was rejected because the specified key policy isn't
* syntactically or semantically correct.
* - KmsThrottledException Amazon Web Services KMS throttles requests for the following conditions.
* - KmsAccessDeniedException The caller doesn't have the required KMS access.
* - KmsInvalidKeyUsageException The request was rejected for one of the following reasons:
*
* -
*
* The KeyUsage value of the KMS key is incompatible with the API operation.
*
*
* -
*
* The encryption algorithm or signing algorithm specified for the operation is incompatible with the type
* of key material in the KMS key (KeySpec).
*
*
* - InvalidAddressException The
accountId
is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.SendMessage
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture sendMessage(SendMessageRequest sendMessageRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(sendMessageRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, sendMessageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SendMessage");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
SendMessageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SendMessage").withProtocolMetadata(protocolMetadata)
.withMarshaller(new SendMessageRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(sendMessageRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* You can use SendMessageBatch
to send up to 10 messages to the specified queue by assigning either
* identical or different values to each message (or by not assigning values at all). This is a batch version of
* SendMessage.
For a FIFO queue, multiple messages within a single batch are enqueued in the
* order they are sent.
*
*
* The result of sending each message is reported individually in the response. Because the batch request can result
* in a combination of successful and unsuccessful actions, you should check for batch errors even when the call
* returns an HTTP status code of 200
.
*
*
* The maximum allowed individual message size and the maximum total payload size (the sum of the individual lengths
* of all of the batched messages) are both 256 KiB (262,144 bytes).
*
*
*
* A message can include only XML, JSON, and unformatted text. The following Unicode characters are allowed. For
* more information, see the W3C specification for characters.
*
*
* #x9
| #xA
| #xD
| #x20
to #xD7FF
|
* #xE000
to #xFFFD
| #x10000
to #x10FFFF
*
*
* Amazon SQS does not throw an exception or completely reject the message if it contains invalid characters.
* Instead, it replaces those invalid characters with U+FFFD
before storing the message in the queue,
* as long as the message body contains at least one valid character.
*
*
*
* If you don't specify the DelaySeconds
parameter for an entry, Amazon SQS uses the default value for
* the queue.
*
*
* @param sendMessageBatchRequest
* @return A Java Future containing the result of the SendMessageBatch operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - TooManyEntriesInBatchRequestException The batch request contains more entries than permissible.
* - EmptyBatchRequestException The batch request doesn't contain any entries.
* - BatchEntryIdsNotDistinctException Two or more batch entries in the request have the same
*
Id
.
* - BatchRequestTooLongException The length of all the messages put together is more than the limit.
* - InvalidBatchEntryIdException The
Id
of a batch entry in a batch request doesn't abide by
* the specification.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - KmsDisabledException The request was denied due to request throttling.
* - KmsInvalidStateException The request was rejected because the state of the specified resource is not
* valid for this request.
* - KmsNotFoundException The request was rejected because the specified entity or resource could not be
* found.
* - KmsOptInRequiredException The request was rejected because the specified key policy isn't
* syntactically or semantically correct.
* - KmsThrottledException Amazon Web Services KMS throttles requests for the following conditions.
* - KmsAccessDeniedException The caller doesn't have the required KMS access.
* - KmsInvalidKeyUsageException The request was rejected for one of the following reasons:
*
* -
*
* The KeyUsage value of the KMS key is incompatible with the API operation.
*
*
* -
*
* The encryption algorithm or signing algorithm specified for the operation is incompatible with the type
* of key material in the KMS key (KeySpec).
*
*
* - InvalidAddressException The
accountId
is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.SendMessageBatch
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture sendMessageBatch(SendMessageBatchRequest sendMessageBatchRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(sendMessageBatchRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, sendMessageBatchRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SendMessageBatch");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SendMessageBatchResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SendMessageBatch").withProtocolMetadata(protocolMetadata)
.withMarshaller(new SendMessageBatchRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(sendMessageBatchRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sets the value of one or more queue attributes, like a policy. When you change a queue's attributes, the change
* can take up to 60 seconds for most of the attributes to propagate throughout the Amazon SQS system. Changes made
* to the MessageRetentionPeriod
attribute can take up to 15 minutes and will impact existing messages
* in the queue potentially causing them to be expired and deleted if the MessageRetentionPeriod
is
* reduced below the age of existing messages.
*
*
*
* -
*
* In the future, new attributes might be added. If you write code that calls this action, we recommend that you
* structure your code so that it can handle new attributes gracefully.
*
*
* -
*
* Cross-account permissions don't apply to this action. For more information, see Grant cross-account permissions to a role and a username in the Amazon SQS Developer Guide.
*
*
* -
*
* To remove the ability to change queue permissions, you must deny permission to the AddPermission
,
* RemovePermission
, and SetQueueAttributes
actions in your IAM policy.
*
*
*
*
*
* @param setQueueAttributesRequest
* @return A Java Future containing the result of the SetQueueAttributes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidAttributeNameException The specified attribute doesn't exist.
* - InvalidAttributeValueException A queue attribute value is invalid.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - OverLimitException The specified action violates a limit. For example,
ReceiveMessage
* returns this error if the maximum number of in flight messages is reached and AddPermission
* returns this error if the maximum number of permissions for the queue is reached.
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.SetQueueAttributes
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture setQueueAttributes(SetQueueAttributesRequest setQueueAttributesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(setQueueAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, setQueueAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetQueueAttributes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetQueueAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetQueueAttributes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new SetQueueAttributesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(setQueueAttributesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Starts an asynchronous task to move messages from a specified source queue to a specified destination queue.
*
*
*
* -
*
* This action is currently limited to supporting message redrive from queues that are configured as dead-letter queues (DLQs) of other Amazon SQS queues only. Non-SQS queue sources of dead-letter queues, such
* as Lambda or Amazon SNS topics, are currently not supported.
*
*
* -
*
* In dead-letter queues redrive context, the StartMessageMoveTask
the source queue is the DLQ, while
* the destination queue can be the original source queue (from which the messages were driven to the
* dead-letter-queue), or a custom destination queue.
*
*
* -
*
* Only one active message movement task is supported per queue at any given time.
*
*
*
*
*
* @param startMessageMoveTaskRequest
* @return A Java Future containing the result of the StartMessageMoveTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException One or more specified resources don't exist.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - InvalidAddressException The
accountId
is invalid.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.StartMessageMoveTask
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture startMessageMoveTask(
StartMessageMoveTaskRequest startMessageMoveTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startMessageMoveTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startMessageMoveTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartMessageMoveTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartMessageMoveTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartMessageMoveTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartMessageMoveTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startMessageMoveTaskRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Add cost allocation tags to the specified Amazon SQS queue. For an overview, see Tagging
* Your Amazon SQS Queues in the Amazon SQS Developer Guide.
*
*
* When you use queue tags, keep the following guidelines in mind:
*
*
* -
*
* Adding more than 50 tags to a queue isn't recommended.
*
*
* -
*
* Tags don't have any semantic meaning. Amazon SQS interprets tags as character strings.
*
*
* -
*
* Tags are case-sensitive.
*
*
* -
*
* A new tag with a key identical to that of an existing tag overwrites the existing tag.
*
*
*
*
* For a full list of tag restrictions, see Quotas related to queues in the Amazon SQS Developer Guide.
*
*
*
* Cross-account permissions don't apply to this action. For more information, see Grant cross-account permissions to a role and a username in the Amazon SQS Developer Guide.
*
*
*
* @param tagQueueRequest
* @return A Java Future containing the result of the TagQueue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidAddressException The
accountId
is invalid.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.TagQueue
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagQueue(TagQueueRequest tagQueueRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagQueueRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagQueueRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagQueue");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagQueueResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("TagQueue")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new TagQueueRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(tagQueueRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Remove cost allocation tags from the specified Amazon SQS queue. For an overview, see Tagging
* Your Amazon SQS Queues in the Amazon SQS Developer Guide.
*
*
*
* Cross-account permissions don't apply to this action. For more information, see Grant cross-account permissions to a role and a username in the Amazon SQS Developer Guide.
*
*
*
* @param untagQueueRequest
* @return A Java Future containing the result of the UntagQueue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidAddressException The
accountId
is invalid.
* - RequestThrottledException The request was denied due to request throttling.
*
* -
*
* The rate of requests per second exceeds the Amazon Web Services KMS request quota for an account and
* Region.
*
*
* -
*
* A burst or sustained high rate of requests to change the state of the same KMS key. This condition is
* often known as a "hot key."
*
*
* -
*
* Requests for operations on KMS keys in a Amazon Web Services CloudHSM key store might be throttled at a
* lower-than-expected rate when the Amazon Web Services CloudHSM cluster associated with the Amazon Web
* Services CloudHSM key store is processing numerous commands, including those unrelated to the Amazon Web
* Services CloudHSM key store.
*
*
* - QueueDoesNotExistException The specified queue doesn't exist.
* - InvalidSecurityException When the request to a queue is not HTTPS and SigV4.
* - UnsupportedOperationException Error code 400. Unsupported operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SqsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SqsAsyncClient.UntagQueue
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture untagQueue(UntagQueueRequest untagQueueRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(untagQueueRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagQueueRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SQS");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagQueue");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagQueueResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("UntagQueue")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new UntagQueueRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(untagQueueRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public SqsAsyncBatchManager batchManager() {
return SqsAsyncBatchManager.builder().client(this).scheduledExecutor(executorService).build();
}
@Override
public final SqsServiceClientConfiguration serviceClientConfiguration() {
return new SqsServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(SqsException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.0")
.hasAwsQueryCompatible(true)
.registerModeledException(
ExceptionMetadata.builder().errorCode("PurgeQueueInProgress")
.exceptionBuilderSupplier(PurgeQueueInProgressException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("KmsInvalidKeyUsage")
.exceptionBuilderSupplier(KmsInvalidKeyUsageException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidAttributeName")
.exceptionBuilderSupplier(InvalidAttributeNameException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("RequestThrottled")
.exceptionBuilderSupplier(RequestThrottledException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BatchEntryIdsNotDistinct")
.exceptionBuilderSupplier(BatchEntryIdsNotDistinctException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyEntriesInBatchRequest")
.exceptionBuilderSupplier(TooManyEntriesInBatchRequestException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("BatchRequestTooLong")
.exceptionBuilderSupplier(BatchRequestTooLongException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnsupportedOperation")
.exceptionBuilderSupplier(UnsupportedOperationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("KmsInvalidState")
.exceptionBuilderSupplier(KmsInvalidStateException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidIdFormat")
.exceptionBuilderSupplier(InvalidIdFormatException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("KmsNotFound")
.exceptionBuilderSupplier(KmsNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("KmsThrottled")
.exceptionBuilderSupplier(KmsThrottledException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("KmsOptInRequired")
.exceptionBuilderSupplier(KmsOptInRequiredException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("KmsAccessDenied")
.exceptionBuilderSupplier(KmsAccessDeniedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("OverLimit").exceptionBuilderSupplier(OverLimitException::builder)
.httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("QueueDoesNotExist")
.exceptionBuilderSupplier(QueueDoesNotExistException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("KmsDisabled")
.exceptionBuilderSupplier(KmsDisabledException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("QueueNameExists")
.exceptionBuilderSupplier(QueueNameExistsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("MessageNotInflight")
.exceptionBuilderSupplier(MessageNotInflightException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ReceiptHandleIsInvalid")
.exceptionBuilderSupplier(ReceiptHandleIsInvalidException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidAttributeValue")
.exceptionBuilderSupplier(InvalidAttributeValueException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidMessageContents")
.exceptionBuilderSupplier(InvalidMessageContentsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("QueueDeletedRecently")
.exceptionBuilderSupplier(QueueDeletedRecentlyException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("EmptyBatchRequest")
.exceptionBuilderSupplier(EmptyBatchRequestException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSecurity")
.exceptionBuilderSupplier(InvalidSecurityException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidAddress")
.exceptionBuilderSupplier(InvalidAddressException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidBatchEntryId")
.exceptionBuilderSupplier(InvalidBatchEntryIdException::builder).httpStatusCode(400).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
SqsServiceClientConfigurationBuilder serviceConfigBuilder = new SqsServiceClientConfigurationBuilder(configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata, Function> exceptionMetadataMapper) {
return protocolFactory.createErrorResponseHandler(operationMetadata, exceptionMetadataMapper);
}
@Override
public void close() {
clientHandler.close();
}
}