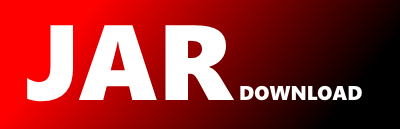
software.amazon.awssdk.services.sqs.model.ReceiveMessageRequest Maven / Gradle / Ivy
Show all versions of sqs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sqs.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReceiveMessageRequest extends SqsRequest implements
ToCopyableBuilder {
private static final SdkField QUEUE_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("QueueUrl").getter(getter(ReceiveMessageRequest::queueUrl)).setter(setter(Builder::queueUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QueueUrl").build()).build();
private static final SdkField> ATTRIBUTE_NAMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AttributeNames")
.getter(getter(ReceiveMessageRequest::attributeNamesAsStrings))
.setter(setter(Builder::attributeNamesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AttributeNames").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).isFlattened(true).build()).build();
private static final SdkField> MESSAGE_SYSTEM_ATTRIBUTE_NAMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MessageSystemAttributeNames")
.getter(getter(ReceiveMessageRequest::messageSystemAttributeNamesAsStrings))
.setter(setter(Builder::messageSystemAttributeNamesWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MessageSystemAttributeNames")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).isFlattened(true).build()).build();
private static final SdkField> MESSAGE_ATTRIBUTE_NAMES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("MessageAttributeNames")
.getter(getter(ReceiveMessageRequest::messageAttributeNames))
.setter(setter(Builder::messageAttributeNames))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MessageAttributeNames").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).isFlattened(true).build()).build();
private static final SdkField MAX_NUMBER_OF_MESSAGES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxNumberOfMessages").getter(getter(ReceiveMessageRequest::maxNumberOfMessages))
.setter(setter(Builder::maxNumberOfMessages))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxNumberOfMessages").build())
.build();
private static final SdkField VISIBILITY_TIMEOUT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("VisibilityTimeout").getter(getter(ReceiveMessageRequest::visibilityTimeout))
.setter(setter(Builder::visibilityTimeout))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VisibilityTimeout").build()).build();
private static final SdkField WAIT_TIME_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("WaitTimeSeconds").getter(getter(ReceiveMessageRequest::waitTimeSeconds))
.setter(setter(Builder::waitTimeSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WaitTimeSeconds").build()).build();
private static final SdkField RECEIVE_REQUEST_ATTEMPT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReceiveRequestAttemptId").getter(getter(ReceiveMessageRequest::receiveRequestAttemptId))
.setter(setter(Builder::receiveRequestAttemptId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReceiveRequestAttemptId").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(QUEUE_URL_FIELD,
ATTRIBUTE_NAMES_FIELD, MESSAGE_SYSTEM_ATTRIBUTE_NAMES_FIELD, MESSAGE_ATTRIBUTE_NAMES_FIELD,
MAX_NUMBER_OF_MESSAGES_FIELD, VISIBILITY_TIMEOUT_FIELD, WAIT_TIME_SECONDS_FIELD, RECEIVE_REQUEST_ATTEMPT_ID_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("QueueUrl", QUEUE_URL_FIELD);
put("AttributeNames", ATTRIBUTE_NAMES_FIELD);
put("MessageSystemAttributeNames", MESSAGE_SYSTEM_ATTRIBUTE_NAMES_FIELD);
put("MessageAttributeNames", MESSAGE_ATTRIBUTE_NAMES_FIELD);
put("MaxNumberOfMessages", MAX_NUMBER_OF_MESSAGES_FIELD);
put("VisibilityTimeout", VISIBILITY_TIMEOUT_FIELD);
put("WaitTimeSeconds", WAIT_TIME_SECONDS_FIELD);
put("ReceiveRequestAttemptId", RECEIVE_REQUEST_ATTEMPT_ID_FIELD);
}
});
private final String queueUrl;
private final List attributeNames;
private final List messageSystemAttributeNames;
private final List messageAttributeNames;
private final Integer maxNumberOfMessages;
private final Integer visibilityTimeout;
private final Integer waitTimeSeconds;
private final String receiveRequestAttemptId;
private ReceiveMessageRequest(BuilderImpl builder) {
super(builder);
this.queueUrl = builder.queueUrl;
this.attributeNames = builder.attributeNames;
this.messageSystemAttributeNames = builder.messageSystemAttributeNames;
this.messageAttributeNames = builder.messageAttributeNames;
this.maxNumberOfMessages = builder.maxNumberOfMessages;
this.visibilityTimeout = builder.visibilityTimeout;
this.waitTimeSeconds = builder.waitTimeSeconds;
this.receiveRequestAttemptId = builder.receiveRequestAttemptId;
}
/**
*
* The URL of the Amazon SQS queue from which messages are received.
*
*
* Queue URLs and names are case-sensitive.
*
*
* @return The URL of the Amazon SQS queue from which messages are received.
*
* Queue URLs and names are case-sensitive.
*/
public final String queueUrl() {
return queueUrl;
}
/**
*
*
* This parameter has been deprecated but will be supported for backward compatibility. To provide attribute names,
* you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the queue
* (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all queues
* but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys. Only
* one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAttributeNames} method.
*
*
* @return
* This parameter has been deprecated but will be supported for backward compatibility. To provide attribute
* names, you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned
* in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
* @deprecated AttributeNames has been replaced by MessageSystemAttributeNames
*/
@Deprecated
public final List attributeNames() {
return AttributeNameListCopier.copyStringToEnum(attributeNames);
}
/**
* For responses, this returns true if the service returned a value for the AttributeNames property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*
* @deprecated AttributeNames has been replaced by MessageSystemAttributeNames
*/
@Deprecated
public final boolean hasAttributeNames() {
return attributeNames != null && !(attributeNames instanceof SdkAutoConstructList);
}
/**
*
*
* This parameter has been deprecated but will be supported for backward compatibility. To provide attribute names,
* you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the queue
* (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all queues
* but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys. Only
* one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAttributeNames} method.
*
*
* @return
* This parameter has been deprecated but will be supported for backward compatibility. To provide attribute
* names, you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned
* in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
* @deprecated AttributeNames has been replaced by MessageSystemAttributeNames
*/
@Deprecated
public final List attributeNamesAsStrings() {
return attributeNames;
}
/**
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the queue
* (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all queues
* but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys. Only
* one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMessageSystemAttributeNames} method.
*
*
* @return A list of attributes that need to be returned along with each message. These attributes include:
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned
* in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*/
public final List messageSystemAttributeNames() {
return MessageSystemAttributeListCopier.copyStringToEnum(messageSystemAttributeNames);
}
/**
* For responses, this returns true if the service returned a value for the MessageSystemAttributeNames property.
* This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasMessageSystemAttributeNames() {
return messageSystemAttributeNames != null && !(messageSystemAttributeNames instanceof SdkAutoConstructList);
}
/**
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the queue
* (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all queues
* but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys. Only
* one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMessageSystemAttributeNames} method.
*
*
* @return A list of attributes that need to be returned along with each message. These attributes include:
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned
* in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*/
public final List messageSystemAttributeNamesAsStrings() {
return messageSystemAttributeNames;
}
/**
* For responses, this returns true if the service returned a value for the MessageAttributeNames property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasMessageAttributeNames() {
return messageAttributeNames != null && !(messageAttributeNames instanceof SdkAutoConstructList);
}
/**
*
* The name of the message attribute, where N is the index.
*
*
* -
*
* The name can contain alphanumeric characters and the underscore (_
), hyphen (-
), and
* period (.
).
*
*
* -
*
* The name is case-sensitive and must be unique among all attribute names for the message.
*
*
* -
*
* The name must not start with AWS-reserved prefixes such as AWS.
or Amazon.
(or any
* casing variants).
*
*
* -
*
* The name must not start or end with a period (.
), and it should not have periods in succession (
* ..
).
*
*
* -
*
* The name can be up to 256 characters long.
*
*
*
*
* When using ReceiveMessage
, you can send a list of attribute names to receive, or you can return all
* of the attributes by specifying All
or .*
in your request. You can also use all message
* attributes starting with a prefix, for example bar.*
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasMessageAttributeNames} method.
*
*
* @return The name of the message attribute, where N is the index.
*
* -
*
* The name can contain alphanumeric characters and the underscore (_
), hyphen (-
* ), and period (.
).
*
*
* -
*
* The name is case-sensitive and must be unique among all attribute names for the message.
*
*
* -
*
* The name must not start with AWS-reserved prefixes such as AWS.
or Amazon.
(or
* any casing variants).
*
*
* -
*
* The name must not start or end with a period (.
), and it should not have periods in
* succession (..
).
*
*
* -
*
* The name can be up to 256 characters long.
*
*
*
*
* When using ReceiveMessage
, you can send a list of attribute names to receive, or you can
* return all of the attributes by specifying All
or .*
in your request. You can
* also use all message attributes starting with a prefix, for example bar.*
.
*/
public final List messageAttributeNames() {
return messageAttributeNames;
}
/**
*
* The maximum number of messages to return. Amazon SQS never returns more messages than this value (however, fewer
* messages might be returned). Valid values: 1 to 10. Default: 1.
*
*
* @return The maximum number of messages to return. Amazon SQS never returns more messages than this value
* (however, fewer messages might be returned). Valid values: 1 to 10. Default: 1.
*/
public final Integer maxNumberOfMessages() {
return maxNumberOfMessages;
}
/**
*
* The duration (in seconds) that the received messages are hidden from subsequent retrieve requests after being
* retrieved by a ReceiveMessage
request.
*
*
* @return The duration (in seconds) that the received messages are hidden from subsequent retrieve requests after
* being retrieved by a ReceiveMessage
request.
*/
public final Integer visibilityTimeout() {
return visibilityTimeout;
}
/**
*
* The duration (in seconds) for which the call waits for a message to arrive in the queue before returning. If a
* message is available, the call returns sooner than WaitTimeSeconds
. If no messages are available and
* the wait time expires, the call does not return a message list.
*
*
*
* To avoid HTTP errors, ensure that the HTTP response timeout for ReceiveMessage
requests is longer
* than the WaitTimeSeconds
parameter. For example, with the Java SDK, you can set HTTP transport
* settings using the
* NettyNioAsyncHttpClient for asynchronous clients, or the
* ApacheHttpClient for synchronous clients.
*
*
*
* @return The duration (in seconds) for which the call waits for a message to arrive in the queue before returning.
* If a message is available, the call returns sooner than WaitTimeSeconds
. If no messages are
* available and the wait time expires, the call does not return a message list.
*
* To avoid HTTP errors, ensure that the HTTP response timeout for ReceiveMessage
requests is
* longer than the WaitTimeSeconds
parameter. For example, with the Java SDK, you can set HTTP
* transport settings using the NettyNioAsyncHttpClient for asynchronous clients, or the
* ApacheHttpClient for synchronous clients.
*
*/
public final Integer waitTimeSeconds() {
return waitTimeSeconds;
}
/**
*
* This parameter applies only to FIFO (first-in-first-out) queues.
*
*
* The token used for deduplication of ReceiveMessage
calls. If a networking issue occurs after a
* ReceiveMessage
action, and instead of a response you receive a generic error, it is possible to
* retry the same action with an identical ReceiveRequestAttemptId
to retrieve the same set of
* messages, even if their visibility timeout has not yet expired.
*
*
* -
*
* You can use ReceiveRequestAttemptId
only for 5 minutes after a ReceiveMessage
action.
*
*
* -
*
* When you set FifoQueue
, a caller of the ReceiveMessage
action can provide a
* ReceiveRequestAttemptId
explicitly.
*
*
* -
*
* It is possible to retry the ReceiveMessage
action with the same ReceiveRequestAttemptId
* if none of the messages have been modified (deleted or had their visibility changes).
*
*
* -
*
* During a visibility timeout, subsequent calls with the same ReceiveRequestAttemptId
return the same
* messages and receipt handles. If a retry occurs within the deduplication interval, it resets the visibility
* timeout. For more information, see Visibility Timeout in the Amazon SQS Developer Guide.
*
*
*
* If a caller of the ReceiveMessage
action still processes messages when the visibility timeout
* expires and messages become visible, another worker consuming from the same queue can receive the same messages
* and therefore process duplicates. Also, if a consumer whose message processing time is longer than the visibility
* timeout tries to delete the processed messages, the action fails with an error.
*
*
* To mitigate this effect, ensure that your application observes a safe threshold before the visibility timeout
* expires and extend the visibility timeout as necessary.
*
*
* -
*
* While messages with a particular MessageGroupId
are invisible, no more messages belonging to the
* same MessageGroupId
are returned until the visibility timeout expires. You can still receive
* messages with another MessageGroupId
as long as it is also visible.
*
*
* -
*
* If a caller of ReceiveMessage
can't track the ReceiveRequestAttemptId
, no retries work
* until the original visibility timeout expires. As a result, delays might occur but the messages in the queue
* remain in a strict order.
*
*
*
*
* The maximum length of ReceiveRequestAttemptId
is 128 characters.
* ReceiveRequestAttemptId
can contain alphanumeric characters (a-z
, A-Z
,
* 0-9
) and punctuation (!"#$%&'()*+,-./:;<=>?@[\]^_`{|}~
).
*
*
* For best practices of using ReceiveRequestAttemptId
, see Using the ReceiveRequestAttemptId Request Parameter in the Amazon SQS Developer Guide.
*
*
* @return This parameter applies only to FIFO (first-in-first-out) queues.
*
* The token used for deduplication of ReceiveMessage
calls. If a networking issue occurs after
* a ReceiveMessage
action, and instead of a response you receive a generic error, it is
* possible to retry the same action with an identical ReceiveRequestAttemptId
to retrieve the
* same set of messages, even if their visibility timeout has not yet expired.
*
*
* -
*
* You can use ReceiveRequestAttemptId
only for 5 minutes after a ReceiveMessage
* action.
*
*
* -
*
* When you set FifoQueue
, a caller of the ReceiveMessage
action can provide a
* ReceiveRequestAttemptId
explicitly.
*
*
* -
*
* It is possible to retry the ReceiveMessage
action with the same
* ReceiveRequestAttemptId
if none of the messages have been modified (deleted or had their
* visibility changes).
*
*
* -
*
* During a visibility timeout, subsequent calls with the same ReceiveRequestAttemptId
return
* the same messages and receipt handles. If a retry occurs within the deduplication interval, it resets the
* visibility timeout. For more information, see Visibility Timeout in the Amazon SQS Developer Guide.
*
*
*
* If a caller of the ReceiveMessage
action still processes messages when the visibility
* timeout expires and messages become visible, another worker consuming from the same queue can receive the
* same messages and therefore process duplicates. Also, if a consumer whose message processing time is
* longer than the visibility timeout tries to delete the processed messages, the action fails with an
* error.
*
*
* To mitigate this effect, ensure that your application observes a safe threshold before the visibility
* timeout expires and extend the visibility timeout as necessary.
*
*
* -
*
* While messages with a particular MessageGroupId
are invisible, no more messages belonging to
* the same MessageGroupId
are returned until the visibility timeout expires. You can still
* receive messages with another MessageGroupId
as long as it is also visible.
*
*
* -
*
* If a caller of ReceiveMessage
can't track the ReceiveRequestAttemptId
, no
* retries work until the original visibility timeout expires. As a result, delays might occur but the
* messages in the queue remain in a strict order.
*
*
*
*
* The maximum length of ReceiveRequestAttemptId
is 128 characters.
* ReceiveRequestAttemptId
can contain alphanumeric characters (a-z
,
* A-Z
, 0-9
) and punctuation (
* !"#$%&'()*+,-./:;<=>?@[\]^_`{|}~
).
*
*
* For best practices of using ReceiveRequestAttemptId
, see Using the ReceiveRequestAttemptId Request Parameter in the Amazon SQS Developer Guide.
*/
public final String receiveRequestAttemptId() {
return receiveRequestAttemptId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(queueUrl());
hashCode = 31 * hashCode + Objects.hashCode(hasAttributeNames() ? attributeNamesAsStrings() : null);
hashCode = 31 * hashCode
+ Objects.hashCode(hasMessageSystemAttributeNames() ? messageSystemAttributeNamesAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasMessageAttributeNames() ? messageAttributeNames() : null);
hashCode = 31 * hashCode + Objects.hashCode(maxNumberOfMessages());
hashCode = 31 * hashCode + Objects.hashCode(visibilityTimeout());
hashCode = 31 * hashCode + Objects.hashCode(waitTimeSeconds());
hashCode = 31 * hashCode + Objects.hashCode(receiveRequestAttemptId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReceiveMessageRequest)) {
return false;
}
ReceiveMessageRequest other = (ReceiveMessageRequest) obj;
return Objects.equals(queueUrl(), other.queueUrl()) && hasAttributeNames() == other.hasAttributeNames()
&& Objects.equals(attributeNamesAsStrings(), other.attributeNamesAsStrings())
&& hasMessageSystemAttributeNames() == other.hasMessageSystemAttributeNames()
&& Objects.equals(messageSystemAttributeNamesAsStrings(), other.messageSystemAttributeNamesAsStrings())
&& hasMessageAttributeNames() == other.hasMessageAttributeNames()
&& Objects.equals(messageAttributeNames(), other.messageAttributeNames())
&& Objects.equals(maxNumberOfMessages(), other.maxNumberOfMessages())
&& Objects.equals(visibilityTimeout(), other.visibilityTimeout())
&& Objects.equals(waitTimeSeconds(), other.waitTimeSeconds())
&& Objects.equals(receiveRequestAttemptId(), other.receiveRequestAttemptId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("ReceiveMessageRequest")
.add("QueueUrl", queueUrl())
.add("AttributeNames", hasAttributeNames() ? attributeNamesAsStrings() : null)
.add("MessageSystemAttributeNames",
hasMessageSystemAttributeNames() ? messageSystemAttributeNamesAsStrings() : null)
.add("MessageAttributeNames", hasMessageAttributeNames() ? messageAttributeNames() : null)
.add("MaxNumberOfMessages", maxNumberOfMessages()).add("VisibilityTimeout", visibilityTimeout())
.add("WaitTimeSeconds", waitTimeSeconds()).add("ReceiveRequestAttemptId", receiveRequestAttemptId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "QueueUrl":
return Optional.ofNullable(clazz.cast(queueUrl()));
case "AttributeNames":
return Optional.ofNullable(clazz.cast(attributeNamesAsStrings()));
case "MessageSystemAttributeNames":
return Optional.ofNullable(clazz.cast(messageSystemAttributeNamesAsStrings()));
case "MessageAttributeNames":
return Optional.ofNullable(clazz.cast(messageAttributeNames()));
case "MaxNumberOfMessages":
return Optional.ofNullable(clazz.cast(maxNumberOfMessages()));
case "VisibilityTimeout":
return Optional.ofNullable(clazz.cast(visibilityTimeout()));
case "WaitTimeSeconds":
return Optional.ofNullable(clazz.cast(waitTimeSeconds()));
case "ReceiveRequestAttemptId":
return Optional.ofNullable(clazz.cast(receiveRequestAttemptId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* Queue URLs and names are case-sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder queueUrl(String queueUrl);
/**
*
*
* This parameter has been deprecated but will be supported for backward compatibility. To provide attribute
* names, you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* @param attributeNames
*
* This parameter has been deprecated but will be supported for backward compatibility. To provide
* attribute names, you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from
* the queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across
* all queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption
* keys. Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are
* returned in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @deprecated AttributeNames has been replaced by MessageSystemAttributeNames
*/
@Deprecated
Builder attributeNamesWithStrings(Collection attributeNames);
/**
*
*
* This parameter has been deprecated but will be supported for backward compatibility. To provide attribute
* names, you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* @param attributeNames
*
* This parameter has been deprecated but will be supported for backward compatibility. To provide
* attribute names, you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from
* the queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across
* all queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption
* keys. Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are
* returned in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @deprecated AttributeNames has been replaced by MessageSystemAttributeNames
*/
@Deprecated
Builder attributeNamesWithStrings(String... attributeNames);
/**
*
*
* This parameter has been deprecated but will be supported for backward compatibility. To provide attribute
* names, you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* @param attributeNames
*
* This parameter has been deprecated but will be supported for backward compatibility. To provide
* attribute names, you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from
* the queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across
* all queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption
* keys. Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are
* returned in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @deprecated AttributeNames has been replaced by MessageSystemAttributeNames
*/
@Deprecated
Builder attributeNames(Collection attributeNames);
/**
*
*
* This parameter has been deprecated but will be supported for backward compatibility. To provide attribute
* names, you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* @param attributeNames
*
* This parameter has been deprecated but will be supported for backward compatibility. To provide
* attribute names, you are encouraged to use MessageSystemAttributeNames
.
*
*
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from
* the queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across
* all queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption
* keys. Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are
* returned in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @deprecated AttributeNames has been replaced by MessageSystemAttributeNames
*/
@Deprecated
Builder attributeNames(QueueAttributeName... attributeNames);
/**
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* @param messageSystemAttributeNames
* A list of attributes that need to be returned along with each message. These attributes include:
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from
* the queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across
* all queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption
* keys. Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are
* returned in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder messageSystemAttributeNamesWithStrings(Collection messageSystemAttributeNames);
/**
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* @param messageSystemAttributeNames
* A list of attributes that need to be returned along with each message. These attributes include:
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from
* the queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across
* all queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption
* keys. Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are
* returned in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder messageSystemAttributeNamesWithStrings(String... messageSystemAttributeNames);
/**
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* @param messageSystemAttributeNames
* A list of attributes that need to be returned along with each message. These attributes include:
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from
* the queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across
* all queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption
* keys. Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are
* returned in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder messageSystemAttributeNames(Collection messageSystemAttributeNames);
/**
*
* A list of attributes that need to be returned along with each message. These attributes include:
*
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from the
* queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across all
* queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption keys.
* Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are returned in
* sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
*
*
* @param messageSystemAttributeNames
* A list of attributes that need to be returned along with each message. These attributes include:
*
* -
*
* All
– Returns all values.
*
*
* -
*
* ApproximateFirstReceiveTimestamp
– Returns the time the message was first received from
* the queue (epoch time in milliseconds).
*
*
* -
*
* ApproximateReceiveCount
– Returns the number of times a message has been received across
* all queues but not deleted.
*
*
* -
*
* AWSTraceHeader
– Returns the X-Ray trace header string.
*
*
* -
*
* SenderId
*
*
* -
*
* For a user, returns the user ID, for example ABCDEFGHI1JKLMNOPQ23R
.
*
*
* -
*
* For an IAM role, returns the IAM role ID, for example ABCDE1F2GH3I4JK5LMNOP:i-a123b456
.
*
*
*
*
* -
*
* SentTimestamp
– Returns the time the message was sent to the queue (epoch time in milliseconds).
*
*
* -
*
* SqsManagedSseEnabled
– Enables server-side queue encryption using SQS owned encryption
* keys. Only one server-side encryption option is supported per queue (for example, SSE-KMS or SSE-SQS).
*
*
* -
*
* MessageDeduplicationId
– Returns the value provided by the producer that calls the
* SendMessage
action.
*
*
* -
*
* MessageGroupId
– Returns the value provided by the producer that calls the
* SendMessage
action. Messages with the same MessageGroupId
are
* returned in sequence.
*
*
* -
*
* SequenceNumber
– Returns the value provided by Amazon SQS.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder messageSystemAttributeNames(MessageSystemAttributeName... messageSystemAttributeNames);
/**
*
* The name of the message attribute, where N is the index.
*
*
* -
*
* The name can contain alphanumeric characters and the underscore (_
), hyphen (-
),
* and period (.
).
*
*
* -
*
* The name is case-sensitive and must be unique among all attribute names for the message.
*
*
* -
*
* The name must not start with AWS-reserved prefixes such as AWS.
or Amazon.
(or any
* casing variants).
*
*
* -
*
* The name must not start or end with a period (.
), and it should not have periods in succession (
* ..
).
*
*
* -
*
* The name can be up to 256 characters long.
*
*
*
*
* When using ReceiveMessage
, you can send a list of attribute names to receive, or you can return
* all of the attributes by specifying All
or .*
in your request. You can also use all
* message attributes starting with a prefix, for example bar.*
.
*
*
* @param messageAttributeNames
* The name of the message attribute, where N is the index.
*
* -
*
* The name can contain alphanumeric characters and the underscore (_
), hyphen (
* -
), and period (.
).
*
*
* -
*
* The name is case-sensitive and must be unique among all attribute names for the message.
*
*
* -
*
* The name must not start with AWS-reserved prefixes such as AWS.
or Amazon.
* (or any casing variants).
*
*
* -
*
* The name must not start or end with a period (.
), and it should not have periods in
* succession (..
).
*
*
* -
*
* The name can be up to 256 characters long.
*
*
*
*
* When using ReceiveMessage
, you can send a list of attribute names to receive, or you can
* return all of the attributes by specifying All
or .*
in your request. You
* can also use all message attributes starting with a prefix, for example bar.*
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder messageAttributeNames(Collection messageAttributeNames);
/**
*
* The name of the message attribute, where N is the index.
*
*
* -
*
* The name can contain alphanumeric characters and the underscore (_
), hyphen (-
),
* and period (.
).
*
*
* -
*
* The name is case-sensitive and must be unique among all attribute names for the message.
*
*
* -
*
* The name must not start with AWS-reserved prefixes such as AWS.
or Amazon.
(or any
* casing variants).
*
*
* -
*
* The name must not start or end with a period (.
), and it should not have periods in succession (
* ..
).
*
*
* -
*
* The name can be up to 256 characters long.
*
*
*
*
* When using ReceiveMessage
, you can send a list of attribute names to receive, or you can return
* all of the attributes by specifying All
or .*
in your request. You can also use all
* message attributes starting with a prefix, for example bar.*
.
*
*
* @param messageAttributeNames
* The name of the message attribute, where N is the index.
*
* -
*
* The name can contain alphanumeric characters and the underscore (_
), hyphen (
* -
), and period (.
).
*
*
* -
*
* The name is case-sensitive and must be unique among all attribute names for the message.
*
*
* -
*
* The name must not start with AWS-reserved prefixes such as AWS.
or Amazon.
* (or any casing variants).
*
*
* -
*
* The name must not start or end with a period (.
), and it should not have periods in
* succession (..
).
*
*
* -
*
* The name can be up to 256 characters long.
*
*
*
*
* When using ReceiveMessage
, you can send a list of attribute names to receive, or you can
* return all of the attributes by specifying All
or .*
in your request. You
* can also use all message attributes starting with a prefix, for example bar.*
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder messageAttributeNames(String... messageAttributeNames);
/**
*
* The maximum number of messages to return. Amazon SQS never returns more messages than this value (however,
* fewer messages might be returned). Valid values: 1 to 10. Default: 1.
*
*
* @param maxNumberOfMessages
* The maximum number of messages to return. Amazon SQS never returns more messages than this value
* (however, fewer messages might be returned). Valid values: 1 to 10. Default: 1.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder maxNumberOfMessages(Integer maxNumberOfMessages);
/**
*
* The duration (in seconds) that the received messages are hidden from subsequent retrieve requests after being
* retrieved by a ReceiveMessage
request.
*
*
* @param visibilityTimeout
* The duration (in seconds) that the received messages are hidden from subsequent retrieve requests
* after being retrieved by a ReceiveMessage
request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder visibilityTimeout(Integer visibilityTimeout);
/**
*
* The duration (in seconds) for which the call waits for a message to arrive in the queue before returning. If
* a message is available, the call returns sooner than WaitTimeSeconds
. If no messages are
* available and the wait time expires, the call does not return a message list.
*
*
*
* To avoid HTTP errors, ensure that the HTTP response timeout for ReceiveMessage
requests is
* longer than the WaitTimeSeconds
parameter. For example, with the Java SDK, you can set HTTP
* transport settings using the NettyNioAsyncHttpClient for asynchronous clients, or the
* ApacheHttpClient for synchronous clients.
*
*
*
* @param waitTimeSeconds
* The duration (in seconds) for which the call waits for a message to arrive in the queue before
* returning. If a message is available, the call returns sooner than WaitTimeSeconds
. If no
* messages are available and the wait time expires, the call does not return a message list.
*
*
* To avoid HTTP errors, ensure that the HTTP response timeout for ReceiveMessage
requests
* is longer than the WaitTimeSeconds
parameter. For example, with the Java SDK, you can set
* HTTP transport settings using the NettyNioAsyncHttpClient for asynchronous clients, or the
* ApacheHttpClient for synchronous clients.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder waitTimeSeconds(Integer waitTimeSeconds);
/**
*
* This parameter applies only to FIFO (first-in-first-out) queues.
*
*
* The token used for deduplication of ReceiveMessage
calls. If a networking issue occurs after a
* ReceiveMessage
action, and instead of a response you receive a generic error, it is possible to
* retry the same action with an identical ReceiveRequestAttemptId
to retrieve the same set of
* messages, even if their visibility timeout has not yet expired.
*
*
* -
*
* You can use ReceiveRequestAttemptId
only for 5 minutes after a ReceiveMessage
* action.
*
*
* -
*
* When you set FifoQueue
, a caller of the ReceiveMessage
action can provide a
* ReceiveRequestAttemptId
explicitly.
*
*
* -
*
* It is possible to retry the ReceiveMessage
action with the same
* ReceiveRequestAttemptId
if none of the messages have been modified (deleted or had their
* visibility changes).
*
*
* -
*
* During a visibility timeout, subsequent calls with the same ReceiveRequestAttemptId
return the
* same messages and receipt handles. If a retry occurs within the deduplication interval, it resets the
* visibility timeout. For more information, see Visibility Timeout in the Amazon SQS Developer Guide.
*
*
*
* If a caller of the ReceiveMessage
action still processes messages when the visibility timeout
* expires and messages become visible, another worker consuming from the same queue can receive the same
* messages and therefore process duplicates. Also, if a consumer whose message processing time is longer than
* the visibility timeout tries to delete the processed messages, the action fails with an error.
*
*
* To mitigate this effect, ensure that your application observes a safe threshold before the visibility timeout
* expires and extend the visibility timeout as necessary.
*
*
* -
*
* While messages with a particular MessageGroupId
are invisible, no more messages belonging to the
* same MessageGroupId
are returned until the visibility timeout expires. You can still receive
* messages with another MessageGroupId
as long as it is also visible.
*
*
* -
*
* If a caller of ReceiveMessage
can't track the ReceiveRequestAttemptId
, no retries
* work until the original visibility timeout expires. As a result, delays might occur but the messages in the
* queue remain in a strict order.
*
*
*
*
* The maximum length of ReceiveRequestAttemptId
is 128 characters.
* ReceiveRequestAttemptId
can contain alphanumeric characters (a-z
, A-Z
,
* 0-9
) and punctuation (!"#$%&'()*+,-./:;<=>?@[\]^_`{|}~
).
*
*
* For best practices of using ReceiveRequestAttemptId
, see Using the ReceiveRequestAttemptId Request Parameter in the Amazon SQS Developer Guide.
*
*
* @param receiveRequestAttemptId
* This parameter applies only to FIFO (first-in-first-out) queues.
*
* The token used for deduplication of ReceiveMessage
calls. If a networking issue occurs
* after a ReceiveMessage
action, and instead of a response you receive a generic error, it
* is possible to retry the same action with an identical ReceiveRequestAttemptId
to
* retrieve the same set of messages, even if their visibility timeout has not yet expired.
*
*
* -
*
* You can use ReceiveRequestAttemptId
only for 5 minutes after a
* ReceiveMessage
action.
*
*
* -
*
* When you set FifoQueue
, a caller of the ReceiveMessage
action can provide a
* ReceiveRequestAttemptId
explicitly.
*
*
* -
*
* It is possible to retry the ReceiveMessage
action with the same
* ReceiveRequestAttemptId
if none of the messages have been modified (deleted or had their
* visibility changes).
*
*
* -
*
* During a visibility timeout, subsequent calls with the same ReceiveRequestAttemptId
* return the same messages and receipt handles. If a retry occurs within the deduplication interval, it
* resets the visibility timeout. For more information, see Visibility Timeout in the Amazon SQS Developer Guide.
*
*
*
* If a caller of the ReceiveMessage
action still processes messages when the visibility
* timeout expires and messages become visible, another worker consuming from the same queue can receive
* the same messages and therefore process duplicates. Also, if a consumer whose message processing time
* is longer than the visibility timeout tries to delete the processed messages, the action fails with an
* error.
*
*
* To mitigate this effect, ensure that your application observes a safe threshold before the visibility
* timeout expires and extend the visibility timeout as necessary.
*
*
* -
*
* While messages with a particular MessageGroupId
are invisible, no more messages belonging
* to the same MessageGroupId
are returned until the visibility timeout expires. You can
* still receive messages with another MessageGroupId
as long as it is also visible.
*
*
* -
*
* If a caller of ReceiveMessage
can't track the ReceiveRequestAttemptId
, no
* retries work until the original visibility timeout expires. As a result, delays might occur but the
* messages in the queue remain in a strict order.
*
*
*
*
* The maximum length of ReceiveRequestAttemptId
is 128 characters.
* ReceiveRequestAttemptId
can contain alphanumeric characters (a-z
,
* A-Z
, 0-9
) and punctuation (
* !"#$%&'()*+,-./:;<=>?@[\]^_`{|}~
).
*
*
* For best practices of using ReceiveRequestAttemptId
, see Using the ReceiveRequestAttemptId Request Parameter in the Amazon SQS Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder receiveRequestAttemptId(String receiveRequestAttemptId);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends SqsRequest.BuilderImpl implements Builder {
private String queueUrl;
private List attributeNames = DefaultSdkAutoConstructList.getInstance();
private List messageSystemAttributeNames = DefaultSdkAutoConstructList.getInstance();
private List messageAttributeNames = DefaultSdkAutoConstructList.getInstance();
private Integer maxNumberOfMessages;
private Integer visibilityTimeout;
private Integer waitTimeSeconds;
private String receiveRequestAttemptId;
private BuilderImpl() {
}
private BuilderImpl(ReceiveMessageRequest model) {
super(model);
queueUrl(model.queueUrl);
attributeNamesWithStrings(model.attributeNames);
messageSystemAttributeNamesWithStrings(model.messageSystemAttributeNames);
messageAttributeNames(model.messageAttributeNames);
maxNumberOfMessages(model.maxNumberOfMessages);
visibilityTimeout(model.visibilityTimeout);
waitTimeSeconds(model.waitTimeSeconds);
receiveRequestAttemptId(model.receiveRequestAttemptId);
}
public final String getQueueUrl() {
return queueUrl;
}
public final void setQueueUrl(String queueUrl) {
this.queueUrl = queueUrl;
}
@Override
public final Builder queueUrl(String queueUrl) {
this.queueUrl = queueUrl;
return this;
}
@Deprecated
public final Collection getAttributeNames() {
if (attributeNames instanceof SdkAutoConstructList) {
return null;
}
return attributeNames;
}
@Deprecated
public final void setAttributeNames(Collection attributeNames) {
this.attributeNames = AttributeNameListCopier.copy(attributeNames);
}
@Override
@Deprecated
public final Builder attributeNamesWithStrings(Collection attributeNames) {
this.attributeNames = AttributeNameListCopier.copy(attributeNames);
return this;
}
@Override
@SafeVarargs
@Deprecated
public final Builder attributeNamesWithStrings(String... attributeNames) {
attributeNamesWithStrings(Arrays.asList(attributeNames));
return this;
}
@Override
@Deprecated
public final Builder attributeNames(Collection attributeNames) {
this.attributeNames = AttributeNameListCopier.copyEnumToString(attributeNames);
return this;
}
@Override
@SafeVarargs
@Deprecated
public final Builder attributeNames(QueueAttributeName... attributeNames) {
attributeNames(Arrays.asList(attributeNames));
return this;
}
public final Collection getMessageSystemAttributeNames() {
if (messageSystemAttributeNames instanceof SdkAutoConstructList) {
return null;
}
return messageSystemAttributeNames;
}
public final void setMessageSystemAttributeNames(Collection messageSystemAttributeNames) {
this.messageSystemAttributeNames = MessageSystemAttributeListCopier.copy(messageSystemAttributeNames);
}
@Override
public final Builder messageSystemAttributeNamesWithStrings(Collection messageSystemAttributeNames) {
this.messageSystemAttributeNames = MessageSystemAttributeListCopier.copy(messageSystemAttributeNames);
return this;
}
@Override
@SafeVarargs
public final Builder messageSystemAttributeNamesWithStrings(String... messageSystemAttributeNames) {
messageSystemAttributeNamesWithStrings(Arrays.asList(messageSystemAttributeNames));
return this;
}
@Override
public final Builder messageSystemAttributeNames(Collection messageSystemAttributeNames) {
this.messageSystemAttributeNames = MessageSystemAttributeListCopier.copyEnumToString(messageSystemAttributeNames);
return this;
}
@Override
@SafeVarargs
public final Builder messageSystemAttributeNames(MessageSystemAttributeName... messageSystemAttributeNames) {
messageSystemAttributeNames(Arrays.asList(messageSystemAttributeNames));
return this;
}
public final Collection getMessageAttributeNames() {
if (messageAttributeNames instanceof SdkAutoConstructList) {
return null;
}
return messageAttributeNames;
}
public final void setMessageAttributeNames(Collection messageAttributeNames) {
this.messageAttributeNames = MessageAttributeNameListCopier.copy(messageAttributeNames);
}
@Override
public final Builder messageAttributeNames(Collection messageAttributeNames) {
this.messageAttributeNames = MessageAttributeNameListCopier.copy(messageAttributeNames);
return this;
}
@Override
@SafeVarargs
public final Builder messageAttributeNames(String... messageAttributeNames) {
messageAttributeNames(Arrays.asList(messageAttributeNames));
return this;
}
public final Integer getMaxNumberOfMessages() {
return maxNumberOfMessages;
}
public final void setMaxNumberOfMessages(Integer maxNumberOfMessages) {
this.maxNumberOfMessages = maxNumberOfMessages;
}
@Override
public final Builder maxNumberOfMessages(Integer maxNumberOfMessages) {
this.maxNumberOfMessages = maxNumberOfMessages;
return this;
}
public final Integer getVisibilityTimeout() {
return visibilityTimeout;
}
public final void setVisibilityTimeout(Integer visibilityTimeout) {
this.visibilityTimeout = visibilityTimeout;
}
@Override
public final Builder visibilityTimeout(Integer visibilityTimeout) {
this.visibilityTimeout = visibilityTimeout;
return this;
}
public final Integer getWaitTimeSeconds() {
return waitTimeSeconds;
}
public final void setWaitTimeSeconds(Integer waitTimeSeconds) {
this.waitTimeSeconds = waitTimeSeconds;
}
@Override
public final Builder waitTimeSeconds(Integer waitTimeSeconds) {
this.waitTimeSeconds = waitTimeSeconds;
return this;
}
public final String getReceiveRequestAttemptId() {
return receiveRequestAttemptId;
}
public final void setReceiveRequestAttemptId(String receiveRequestAttemptId) {
this.receiveRequestAttemptId = receiveRequestAttemptId;
}
@Override
public final Builder receiveRequestAttemptId(String receiveRequestAttemptId) {
this.receiveRequestAttemptId = receiveRequestAttemptId;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public ReceiveMessageRequest build() {
return new ReceiveMessageRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}