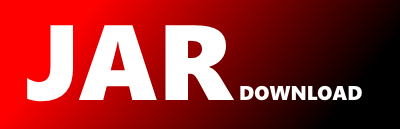
software.amazon.awssdk.services.sqs.model.ListMessageMoveTasksResultEntry Maven / Gradle / Ivy
Show all versions of sqs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sqs.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the details of a message movement task.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListMessageMoveTasksResultEntry implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TASK_HANDLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TaskHandle").getter(getter(ListMessageMoveTasksResultEntry::taskHandle))
.setter(setter(Builder::taskHandle))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TaskHandle").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(ListMessageMoveTasksResultEntry::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField SOURCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceArn").getter(getter(ListMessageMoveTasksResultEntry::sourceArn))
.setter(setter(Builder::sourceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceArn").build()).build();
private static final SdkField DESTINATION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DestinationArn").getter(getter(ListMessageMoveTasksResultEntry::destinationArn))
.setter(setter(Builder::destinationArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DestinationArn").build()).build();
private static final SdkField MAX_NUMBER_OF_MESSAGES_PER_SECOND_FIELD = SdkField
. builder(MarshallingType.INTEGER)
.memberName("MaxNumberOfMessagesPerSecond")
.getter(getter(ListMessageMoveTasksResultEntry::maxNumberOfMessagesPerSecond))
.setter(setter(Builder::maxNumberOfMessagesPerSecond))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxNumberOfMessagesPerSecond")
.build()).build();
private static final SdkField APPROXIMATE_NUMBER_OF_MESSAGES_MOVED_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("ApproximateNumberOfMessagesMoved")
.getter(getter(ListMessageMoveTasksResultEntry::approximateNumberOfMessagesMoved))
.setter(setter(Builder::approximateNumberOfMessagesMoved))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApproximateNumberOfMessagesMoved")
.build()).build();
private static final SdkField APPROXIMATE_NUMBER_OF_MESSAGES_TO_MOVE_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("ApproximateNumberOfMessagesToMove")
.getter(getter(ListMessageMoveTasksResultEntry::approximateNumberOfMessagesToMove))
.setter(setter(Builder::approximateNumberOfMessagesToMove))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApproximateNumberOfMessagesToMove")
.build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FailureReason").getter(getter(ListMessageMoveTasksResultEntry::failureReason))
.setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FailureReason").build()).build();
private static final SdkField STARTED_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("StartedTimestamp").getter(getter(ListMessageMoveTasksResultEntry::startedTimestamp))
.setter(setter(Builder::startedTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartedTimestamp").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TASK_HANDLE_FIELD,
STATUS_FIELD, SOURCE_ARN_FIELD, DESTINATION_ARN_FIELD, MAX_NUMBER_OF_MESSAGES_PER_SECOND_FIELD,
APPROXIMATE_NUMBER_OF_MESSAGES_MOVED_FIELD, APPROXIMATE_NUMBER_OF_MESSAGES_TO_MOVE_FIELD, FAILURE_REASON_FIELD,
STARTED_TIMESTAMP_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("TaskHandle", TASK_HANDLE_FIELD);
put("Status", STATUS_FIELD);
put("SourceArn", SOURCE_ARN_FIELD);
put("DestinationArn", DESTINATION_ARN_FIELD);
put("MaxNumberOfMessagesPerSecond", MAX_NUMBER_OF_MESSAGES_PER_SECOND_FIELD);
put("ApproximateNumberOfMessagesMoved", APPROXIMATE_NUMBER_OF_MESSAGES_MOVED_FIELD);
put("ApproximateNumberOfMessagesToMove", APPROXIMATE_NUMBER_OF_MESSAGES_TO_MOVE_FIELD);
put("FailureReason", FAILURE_REASON_FIELD);
put("StartedTimestamp", STARTED_TIMESTAMP_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String taskHandle;
private final String status;
private final String sourceArn;
private final String destinationArn;
private final Integer maxNumberOfMessagesPerSecond;
private final Long approximateNumberOfMessagesMoved;
private final Long approximateNumberOfMessagesToMove;
private final String failureReason;
private final Long startedTimestamp;
private ListMessageMoveTasksResultEntry(BuilderImpl builder) {
this.taskHandle = builder.taskHandle;
this.status = builder.status;
this.sourceArn = builder.sourceArn;
this.destinationArn = builder.destinationArn;
this.maxNumberOfMessagesPerSecond = builder.maxNumberOfMessagesPerSecond;
this.approximateNumberOfMessagesMoved = builder.approximateNumberOfMessagesMoved;
this.approximateNumberOfMessagesToMove = builder.approximateNumberOfMessagesToMove;
this.failureReason = builder.failureReason;
this.startedTimestamp = builder.startedTimestamp;
}
/**
*
* An identifier associated with a message movement task. When this field is returned in the response of the
* ListMessageMoveTasks
action, it is only populated for tasks that are in RUNNING status.
*
*
* @return An identifier associated with a message movement task. When this field is returned in the response of the
* ListMessageMoveTasks
action, it is only populated for tasks that are in RUNNING status.
*/
public final String taskHandle() {
return taskHandle;
}
/**
*
* The status of the message movement task. Possible values are: RUNNING, COMPLETED, CANCELLING, CANCELLED, and
* FAILED.
*
*
* @return The status of the message movement task. Possible values are: RUNNING, COMPLETED, CANCELLING, CANCELLED,
* and FAILED.
*/
public final String status() {
return status;
}
/**
*
* The ARN of the queue that contains the messages to be moved to another queue.
*
*
* @return The ARN of the queue that contains the messages to be moved to another queue.
*/
public final String sourceArn() {
return sourceArn;
}
/**
*
* The ARN of the destination queue if it has been specified in the StartMessageMoveTask
request. If a
* DestinationArn
has not been specified in the StartMessageMoveTask
request, this field
* value will be NULL.
*
*
* @return The ARN of the destination queue if it has been specified in the StartMessageMoveTask
* request. If a DestinationArn
has not been specified in the StartMessageMoveTask
* request, this field value will be NULL.
*/
public final String destinationArn() {
return destinationArn;
}
/**
*
* The number of messages to be moved per second (the message movement rate), if it has been specified in the
* StartMessageMoveTask
request. If a MaxNumberOfMessagesPerSecond
has not been specified
* in the StartMessageMoveTask
request, this field value will be NULL.
*
*
* @return The number of messages to be moved per second (the message movement rate), if it has been specified in
* the StartMessageMoveTask
request. If a MaxNumberOfMessagesPerSecond
has not
* been specified in the StartMessageMoveTask
request, this field value will be NULL.
*/
public final Integer maxNumberOfMessagesPerSecond() {
return maxNumberOfMessagesPerSecond;
}
/**
*
* The approximate number of messages already moved to the destination queue.
*
*
* @return The approximate number of messages already moved to the destination queue.
*/
public final Long approximateNumberOfMessagesMoved() {
return approximateNumberOfMessagesMoved;
}
/**
*
* The number of messages to be moved from the source queue. This number is obtained at the time of starting the
* message movement task and is only included after the message movement task is selected to start.
*
*
* @return The number of messages to be moved from the source queue. This number is obtained at the time of starting
* the message movement task and is only included after the message movement task is selected to start.
*/
public final Long approximateNumberOfMessagesToMove() {
return approximateNumberOfMessagesToMove;
}
/**
*
* The task failure reason (only included if the task status is FAILED).
*
*
* @return The task failure reason (only included if the task status is FAILED).
*/
public final String failureReason() {
return failureReason;
}
/**
*
* The timestamp of starting the message movement task.
*
*
* @return The timestamp of starting the message movement task.
*/
public final Long startedTimestamp() {
return startedTimestamp;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(taskHandle());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(sourceArn());
hashCode = 31 * hashCode + Objects.hashCode(destinationArn());
hashCode = 31 * hashCode + Objects.hashCode(maxNumberOfMessagesPerSecond());
hashCode = 31 * hashCode + Objects.hashCode(approximateNumberOfMessagesMoved());
hashCode = 31 * hashCode + Objects.hashCode(approximateNumberOfMessagesToMove());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(startedTimestamp());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListMessageMoveTasksResultEntry)) {
return false;
}
ListMessageMoveTasksResultEntry other = (ListMessageMoveTasksResultEntry) obj;
return Objects.equals(taskHandle(), other.taskHandle()) && Objects.equals(status(), other.status())
&& Objects.equals(sourceArn(), other.sourceArn()) && Objects.equals(destinationArn(), other.destinationArn())
&& Objects.equals(maxNumberOfMessagesPerSecond(), other.maxNumberOfMessagesPerSecond())
&& Objects.equals(approximateNumberOfMessagesMoved(), other.approximateNumberOfMessagesMoved())
&& Objects.equals(approximateNumberOfMessagesToMove(), other.approximateNumberOfMessagesToMove())
&& Objects.equals(failureReason(), other.failureReason())
&& Objects.equals(startedTimestamp(), other.startedTimestamp());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListMessageMoveTasksResultEntry").add("TaskHandle", taskHandle()).add("Status", status())
.add("SourceArn", sourceArn()).add("DestinationArn", destinationArn())
.add("MaxNumberOfMessagesPerSecond", maxNumberOfMessagesPerSecond())
.add("ApproximateNumberOfMessagesMoved", approximateNumberOfMessagesMoved())
.add("ApproximateNumberOfMessagesToMove", approximateNumberOfMessagesToMove())
.add("FailureReason", failureReason()).add("StartedTimestamp", startedTimestamp()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "TaskHandle":
return Optional.ofNullable(clazz.cast(taskHandle()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "SourceArn":
return Optional.ofNullable(clazz.cast(sourceArn()));
case "DestinationArn":
return Optional.ofNullable(clazz.cast(destinationArn()));
case "MaxNumberOfMessagesPerSecond":
return Optional.ofNullable(clazz.cast(maxNumberOfMessagesPerSecond()));
case "ApproximateNumberOfMessagesMoved":
return Optional.ofNullable(clazz.cast(approximateNumberOfMessagesMoved()));
case "ApproximateNumberOfMessagesToMove":
return Optional.ofNullable(clazz.cast(approximateNumberOfMessagesToMove()));
case "FailureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "StartedTimestamp":
return Optional.ofNullable(clazz.cast(startedTimestamp()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function