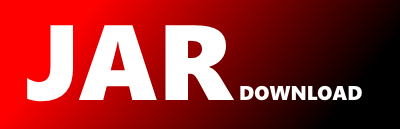
software.amazon.awssdk.services.ssmincidents.model.TimelineEvent Maven / Gradle / Ivy
Show all versions of ssmincidents Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ssmincidents.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A significant event that happened during the incident.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TimelineEvent implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField EVENT_DATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("eventData").getter(getter(TimelineEvent::eventData)).setter(setter(Builder::eventData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventData").build()).build();
private static final SdkField EVENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("eventId").getter(getter(TimelineEvent::eventId)).setter(setter(Builder::eventId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventId").build()).build();
private static final SdkField> EVENT_REFERENCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("eventReferences")
.getter(getter(TimelineEvent::eventReferences))
.setter(setter(Builder::eventReferences))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventReferences").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(EventReference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField EVENT_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("eventTime").getter(getter(TimelineEvent::eventTime)).setter(setter(Builder::eventTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventTime").build()).build();
private static final SdkField EVENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("eventType").getter(getter(TimelineEvent::eventType)).setter(setter(Builder::eventType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventType").build()).build();
private static final SdkField EVENT_UPDATED_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("eventUpdatedTime").getter(getter(TimelineEvent::eventUpdatedTime))
.setter(setter(Builder::eventUpdatedTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventUpdatedTime").build()).build();
private static final SdkField INCIDENT_RECORD_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("incidentRecordArn").getter(getter(TimelineEvent::incidentRecordArn))
.setter(setter(Builder::incidentRecordArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("incidentRecordArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EVENT_DATA_FIELD,
EVENT_ID_FIELD, EVENT_REFERENCES_FIELD, EVENT_TIME_FIELD, EVENT_TYPE_FIELD, EVENT_UPDATED_TIME_FIELD,
INCIDENT_RECORD_ARN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("eventData", EVENT_DATA_FIELD);
put("eventId", EVENT_ID_FIELD);
put("eventReferences", EVENT_REFERENCES_FIELD);
put("eventTime", EVENT_TIME_FIELD);
put("eventType", EVENT_TYPE_FIELD);
put("eventUpdatedTime", EVENT_UPDATED_TIME_FIELD);
put("incidentRecordArn", INCIDENT_RECORD_ARN_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String eventData;
private final String eventId;
private final List eventReferences;
private final Instant eventTime;
private final String eventType;
private final Instant eventUpdatedTime;
private final String incidentRecordArn;
private TimelineEvent(BuilderImpl builder) {
this.eventData = builder.eventData;
this.eventId = builder.eventId;
this.eventReferences = builder.eventReferences;
this.eventTime = builder.eventTime;
this.eventType = builder.eventType;
this.eventUpdatedTime = builder.eventUpdatedTime;
this.incidentRecordArn = builder.incidentRecordArn;
}
/**
*
* A short description of the event.
*
*
* @return A short description of the event.
*/
public final String eventData() {
return eventData;
}
/**
*
* The ID of the timeline event.
*
*
* @return The ID of the timeline event.
*/
public final String eventId() {
return eventId;
}
/**
* For responses, this returns true if the service returned a value for the EventReferences property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasEventReferences() {
return eventReferences != null && !(eventReferences instanceof SdkAutoConstructList);
}
/**
*
* A list of references in a TimelineEvent
.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEventReferences} method.
*
*
* @return A list of references in a TimelineEvent
.
*/
public final List eventReferences() {
return eventReferences;
}
/**
*
* The timestamp for when the event occurred.
*
*
* @return The timestamp for when the event occurred.
*/
public final Instant eventTime() {
return eventTime;
}
/**
*
* The type of event that occurred. Currently Incident Manager supports only the Custom Event
and
* Note
types.
*
*
* @return The type of event that occurred. Currently Incident Manager supports only the Custom Event
* and Note
types.
*/
public final String eventType() {
return eventType;
}
/**
*
* The timestamp for when the timeline event was last updated.
*
*
* @return The timestamp for when the timeline event was last updated.
*/
public final Instant eventUpdatedTime() {
return eventUpdatedTime;
}
/**
*
* The Amazon Resource Name (ARN) of the incident that the event occurred during.
*
*
* @return The Amazon Resource Name (ARN) of the incident that the event occurred during.
*/
public final String incidentRecordArn() {
return incidentRecordArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(eventData());
hashCode = 31 * hashCode + Objects.hashCode(eventId());
hashCode = 31 * hashCode + Objects.hashCode(hasEventReferences() ? eventReferences() : null);
hashCode = 31 * hashCode + Objects.hashCode(eventTime());
hashCode = 31 * hashCode + Objects.hashCode(eventType());
hashCode = 31 * hashCode + Objects.hashCode(eventUpdatedTime());
hashCode = 31 * hashCode + Objects.hashCode(incidentRecordArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TimelineEvent)) {
return false;
}
TimelineEvent other = (TimelineEvent) obj;
return Objects.equals(eventData(), other.eventData()) && Objects.equals(eventId(), other.eventId())
&& hasEventReferences() == other.hasEventReferences()
&& Objects.equals(eventReferences(), other.eventReferences()) && Objects.equals(eventTime(), other.eventTime())
&& Objects.equals(eventType(), other.eventType()) && Objects.equals(eventUpdatedTime(), other.eventUpdatedTime())
&& Objects.equals(incidentRecordArn(), other.incidentRecordArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TimelineEvent").add("EventData", eventData()).add("EventId", eventId())
.add("EventReferences", hasEventReferences() ? eventReferences() : null).add("EventTime", eventTime())
.add("EventType", eventType()).add("EventUpdatedTime", eventUpdatedTime())
.add("IncidentRecordArn", incidentRecordArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "eventData":
return Optional.ofNullable(clazz.cast(eventData()));
case "eventId":
return Optional.ofNullable(clazz.cast(eventId()));
case "eventReferences":
return Optional.ofNullable(clazz.cast(eventReferences()));
case "eventTime":
return Optional.ofNullable(clazz.cast(eventTime()));
case "eventType":
return Optional.ofNullable(clazz.cast(eventType()));
case "eventUpdatedTime":
return Optional.ofNullable(clazz.cast(eventUpdatedTime()));
case "incidentRecordArn":
return Optional.ofNullable(clazz.cast(incidentRecordArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function