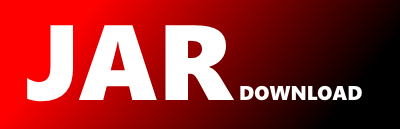
software.amazon.awssdk.services.ssmincidents.model.CreateTimelineEventRequest Maven / Gradle / Ivy
Show all versions of ssmincidents Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ssmincidents.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateTimelineEventRequest extends SsmIncidentsRequest implements
ToCopyableBuilder {
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("clientToken")
.getter(getter(CreateTimelineEventRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientToken").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final SdkField EVENT_DATA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("eventData").getter(getter(CreateTimelineEventRequest::eventData)).setter(setter(Builder::eventData))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventData").build()).build();
private static final SdkField> EVENT_REFERENCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("eventReferences")
.getter(getter(CreateTimelineEventRequest::eventReferences))
.setter(setter(Builder::eventReferences))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventReferences").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(EventReference::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField EVENT_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("eventTime").getter(getter(CreateTimelineEventRequest::eventTime)).setter(setter(Builder::eventTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventTime").build()).build();
private static final SdkField EVENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("eventType").getter(getter(CreateTimelineEventRequest::eventType)).setter(setter(Builder::eventType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("eventType").build()).build();
private static final SdkField INCIDENT_RECORD_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("incidentRecordArn").getter(getter(CreateTimelineEventRequest::incidentRecordArn))
.setter(setter(Builder::incidentRecordArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("incidentRecordArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLIENT_TOKEN_FIELD,
EVENT_DATA_FIELD, EVENT_REFERENCES_FIELD, EVENT_TIME_FIELD, EVENT_TYPE_FIELD, INCIDENT_RECORD_ARN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("clientToken", CLIENT_TOKEN_FIELD);
put("eventData", EVENT_DATA_FIELD);
put("eventReferences", EVENT_REFERENCES_FIELD);
put("eventTime", EVENT_TIME_FIELD);
put("eventType", EVENT_TYPE_FIELD);
put("incidentRecordArn", INCIDENT_RECORD_ARN_FIELD);
}
});
private final String clientToken;
private final String eventData;
private final List eventReferences;
private final Instant eventTime;
private final String eventType;
private final String incidentRecordArn;
private CreateTimelineEventRequest(BuilderImpl builder) {
super(builder);
this.clientToken = builder.clientToken;
this.eventData = builder.eventData;
this.eventReferences = builder.eventReferences;
this.eventTime = builder.eventTime;
this.eventType = builder.eventType;
this.incidentRecordArn = builder.incidentRecordArn;
}
/**
*
* A token that ensures that a client calls the action only once with the specified details.
*
*
* @return A token that ensures that a client calls the action only once with the specified details.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* A short description of the event.
*
*
* @return A short description of the event.
*/
public final String eventData() {
return eventData;
}
/**
* For responses, this returns true if the service returned a value for the EventReferences property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasEventReferences() {
return eventReferences != null && !(eventReferences instanceof SdkAutoConstructList);
}
/**
*
* Adds one or more references to the TimelineEvent
. A reference is an Amazon Web Services resource
* involved or associated with the incident. To specify a reference, enter its Amazon Resource Name (ARN). You can
* also specify a related item associated with a resource. For example, to specify an Amazon DynamoDB (DynamoDB)
* table as a resource, use the table's ARN. You can also specify an Amazon CloudWatch metric associated with the
* DynamoDB table as a related item.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEventReferences} method.
*
*
* @return Adds one or more references to the TimelineEvent
. A reference is an Amazon Web Services
* resource involved or associated with the incident. To specify a reference, enter its Amazon Resource Name
* (ARN). You can also specify a related item associated with a resource. For example, to specify an Amazon
* DynamoDB (DynamoDB) table as a resource, use the table's ARN. You can also specify an Amazon CloudWatch
* metric associated with the DynamoDB table as a related item.
*/
public final List eventReferences() {
return eventReferences;
}
/**
*
* The timestamp for when the event occurred.
*
*
* @return The timestamp for when the event occurred.
*/
public final Instant eventTime() {
return eventTime;
}
/**
*
* The type of event. You can create timeline events of type Custom Event
and Note
.
*
*
* To make a Note-type event appear on the Incident notes panel in the console, specify
* eventType
as Note
and enter the Amazon Resource Name (ARN) of the incident as the value
* for eventReference
.
*
*
* @return The type of event. You can create timeline events of type Custom Event
and Note
* .
*
* To make a Note-type event appear on the Incident notes panel in the console, specify
* eventType
as Note
and enter the Amazon Resource Name (ARN) of the incident as
* the value for eventReference
.
*/
public final String eventType() {
return eventType;
}
/**
*
* The Amazon Resource Name (ARN) of the incident record that the action adds the incident to.
*
*
* @return The Amazon Resource Name (ARN) of the incident record that the action adds the incident to.
*/
public final String incidentRecordArn() {
return incidentRecordArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(eventData());
hashCode = 31 * hashCode + Objects.hashCode(hasEventReferences() ? eventReferences() : null);
hashCode = 31 * hashCode + Objects.hashCode(eventTime());
hashCode = 31 * hashCode + Objects.hashCode(eventType());
hashCode = 31 * hashCode + Objects.hashCode(incidentRecordArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateTimelineEventRequest)) {
return false;
}
CreateTimelineEventRequest other = (CreateTimelineEventRequest) obj;
return Objects.equals(clientToken(), other.clientToken()) && Objects.equals(eventData(), other.eventData())
&& hasEventReferences() == other.hasEventReferences()
&& Objects.equals(eventReferences(), other.eventReferences()) && Objects.equals(eventTime(), other.eventTime())
&& Objects.equals(eventType(), other.eventType())
&& Objects.equals(incidentRecordArn(), other.incidentRecordArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateTimelineEventRequest").add("ClientToken", clientToken()).add("EventData", eventData())
.add("EventReferences", hasEventReferences() ? eventReferences() : null).add("EventTime", eventTime())
.add("EventType", eventType()).add("IncidentRecordArn", incidentRecordArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "clientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "eventData":
return Optional.ofNullable(clazz.cast(eventData()));
case "eventReferences":
return Optional.ofNullable(clazz.cast(eventReferences()));
case "eventTime":
return Optional.ofNullable(clazz.cast(eventTime()));
case "eventType":
return Optional.ofNullable(clazz.cast(eventType()));
case "incidentRecordArn":
return Optional.ofNullable(clazz.cast(incidentRecordArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function