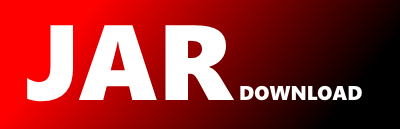
software.amazon.awssdk.services.sso.SsoAsyncClient Maven / Gradle / Ivy
Show all versions of sso Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sso;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.sso.model.GetRoleCredentialsRequest;
import software.amazon.awssdk.services.sso.model.GetRoleCredentialsResponse;
import software.amazon.awssdk.services.sso.model.ListAccountRolesRequest;
import software.amazon.awssdk.services.sso.model.ListAccountRolesResponse;
import software.amazon.awssdk.services.sso.model.ListAccountsRequest;
import software.amazon.awssdk.services.sso.model.ListAccountsResponse;
import software.amazon.awssdk.services.sso.model.LogoutRequest;
import software.amazon.awssdk.services.sso.model.LogoutResponse;
import software.amazon.awssdk.services.sso.paginators.ListAccountRolesPublisher;
import software.amazon.awssdk.services.sso.paginators.ListAccountsPublisher;
/**
* Service client for accessing SSO asynchronously. This can be created using the static {@link #builder()} method.
*
*
* AWS Single Sign-On Portal is a web service that makes it easy for you to assign user access to AWS SSO resources such
* as the user portal. Users can get AWS account applications and roles assigned to them and get federated into the
* application.
*
*
* For general information about AWS SSO, see What is AWS Single Sign-On? in the
* AWS SSO User Guide.
*
*
* This API reference guide describes the AWS SSO Portal operations that you can call programatically and includes
* detailed information on data types and errors.
*
*
*
* AWS provides SDKs that consist of libraries and sample code for various programming languages and platforms, such as
* Java, Ruby, .Net, iOS, or Android. The SDKs provide a convenient way to create programmatic access to AWS SSO and
* other AWS services. For more information about the AWS SDKs, including how to download and install them, see Tools for Amazon Web Services.
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface SsoAsyncClient extends SdkClient {
String SERVICE_NAME = "awsssoportal";
/**
* Create a {@link SsoAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static SsoAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link SsoAsyncClient}.
*/
static SsoAsyncClientBuilder builder() {
return new DefaultSsoAsyncClientBuilder();
}
/**
*
* Returns the STS short-term credentials for a given role name that is assigned to the user.
*
*
* @param getRoleCredentialsRequest
* @return A Java Future containing the result of the GetRoleCredentials operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - ResourceNotFoundException The specified resource doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.GetRoleCredentials
* @see AWS API
* Documentation
*/
default CompletableFuture getRoleCredentials(GetRoleCredentialsRequest getRoleCredentialsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns the STS short-term credentials for a given role name that is assigned to the user.
*
*
*
* This is a convenience which creates an instance of the {@link GetRoleCredentialsRequest.Builder} avoiding the
* need to create one manually via {@link GetRoleCredentialsRequest#builder()}
*
*
* @param getRoleCredentialsRequest
* A {@link Consumer} that will call methods on {@link GetRoleCredentialsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetRoleCredentials operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - ResourceNotFoundException The specified resource doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.GetRoleCredentials
* @see AWS API
* Documentation
*/
default CompletableFuture getRoleCredentials(
Consumer getRoleCredentialsRequest) {
return getRoleCredentials(GetRoleCredentialsRequest.builder().applyMutation(getRoleCredentialsRequest).build());
}
/**
*
* Lists all roles that are assigned to the user for a given AWS account.
*
*
* @param listAccountRolesRequest
* @return A Java Future containing the result of the ListAccountRoles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - ResourceNotFoundException The specified resource doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.ListAccountRoles
* @see AWS API
* Documentation
*/
default CompletableFuture listAccountRoles(ListAccountRolesRequest listAccountRolesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all roles that are assigned to the user for a given AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccountRolesRequest.Builder} avoiding the need
* to create one manually via {@link ListAccountRolesRequest#builder()}
*
*
* @param listAccountRolesRequest
* A {@link Consumer} that will call methods on {@link ListAccountRolesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListAccountRoles operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - ResourceNotFoundException The specified resource doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.ListAccountRoles
* @see AWS API
* Documentation
*/
default CompletableFuture listAccountRoles(
Consumer listAccountRolesRequest) {
return listAccountRoles(ListAccountRolesRequest.builder().applyMutation(listAccountRolesRequest).build());
}
/**
*
* Lists all roles that are assigned to the user for a given AWS account.
*
*
*
* This is a variant of {@link #listAccountRoles(software.amazon.awssdk.services.sso.model.ListAccountRolesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountRolesPublisher publisher = client.listAccountRolesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountRolesPublisher publisher = client.listAccountRolesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sso.model.ListAccountRolesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccountRoles(software.amazon.awssdk.services.sso.model.ListAccountRolesRequest)} operation.
*
*
* @param listAccountRolesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - ResourceNotFoundException The specified resource doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.ListAccountRoles
* @see AWS API
* Documentation
*/
default ListAccountRolesPublisher listAccountRolesPaginator(ListAccountRolesRequest listAccountRolesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all roles that are assigned to the user for a given AWS account.
*
*
*
* This is a variant of {@link #listAccountRoles(software.amazon.awssdk.services.sso.model.ListAccountRolesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountRolesPublisher publisher = client.listAccountRolesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountRolesPublisher publisher = client.listAccountRolesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sso.model.ListAccountRolesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccountRoles(software.amazon.awssdk.services.sso.model.ListAccountRolesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListAccountRolesRequest.Builder} avoiding the need
* to create one manually via {@link ListAccountRolesRequest#builder()}
*
*
* @param listAccountRolesRequest
* A {@link Consumer} that will call methods on {@link ListAccountRolesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - ResourceNotFoundException The specified resource doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.ListAccountRoles
* @see AWS API
* Documentation
*/
default ListAccountRolesPublisher listAccountRolesPaginator(Consumer listAccountRolesRequest) {
return listAccountRolesPaginator(ListAccountRolesRequest.builder().applyMutation(listAccountRolesRequest).build());
}
/**
*
* Lists all AWS accounts assigned to the user. These AWS accounts are assigned by the administrator of the account.
* For more information, see Assign User
* Access in the AWS SSO User Guide. This operation returns a paginated response.
*
*
* @param listAccountsRequest
* @return A Java Future containing the result of the ListAccounts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - ResourceNotFoundException The specified resource doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.ListAccounts
* @see AWS API
* Documentation
*/
default CompletableFuture listAccounts(ListAccountsRequest listAccountsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all AWS accounts assigned to the user. These AWS accounts are assigned by the administrator of the account.
* For more information, see Assign User
* Access in the AWS SSO User Guide. This operation returns a paginated response.
*
*
*
* This is a convenience which creates an instance of the {@link ListAccountsRequest.Builder} avoiding the need to
* create one manually via {@link ListAccountsRequest#builder()}
*
*
* @param listAccountsRequest
* A {@link Consumer} that will call methods on {@link ListAccountsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListAccounts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - ResourceNotFoundException The specified resource doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.ListAccounts
* @see AWS API
* Documentation
*/
default CompletableFuture listAccounts(Consumer listAccountsRequest) {
return listAccounts(ListAccountsRequest.builder().applyMutation(listAccountsRequest).build());
}
/**
*
* Lists all AWS accounts assigned to the user. These AWS accounts are assigned by the administrator of the account.
* For more information, see Assign User
* Access in the AWS SSO User Guide. This operation returns a paginated response.
*
*
*
* This is a variant of {@link #listAccounts(software.amazon.awssdk.services.sso.model.ListAccountsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountsPublisher publisher = client.listAccountsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountsPublisher publisher = client.listAccountsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sso.model.ListAccountsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccounts(software.amazon.awssdk.services.sso.model.ListAccountsRequest)} operation.
*
*
* @param listAccountsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - ResourceNotFoundException The specified resource doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.ListAccounts
* @see AWS API
* Documentation
*/
default ListAccountsPublisher listAccountsPaginator(ListAccountsRequest listAccountsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all AWS accounts assigned to the user. These AWS accounts are assigned by the administrator of the account.
* For more information, see Assign User
* Access in the AWS SSO User Guide. This operation returns a paginated response.
*
*
*
* This is a variant of {@link #listAccounts(software.amazon.awssdk.services.sso.model.ListAccountsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountsPublisher publisher = client.listAccountsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountsPublisher publisher = client.listAccountsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.sso.model.ListAccountsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccounts(software.amazon.awssdk.services.sso.model.ListAccountsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListAccountsRequest.Builder} avoiding the need to
* create one manually via {@link ListAccountsRequest#builder()}
*
*
* @param listAccountsRequest
* A {@link Consumer} that will call methods on {@link ListAccountsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - ResourceNotFoundException The specified resource doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.ListAccounts
* @see AWS API
* Documentation
*/
default ListAccountsPublisher listAccountsPaginator(Consumer listAccountsRequest) {
return listAccountsPaginator(ListAccountsRequest.builder().applyMutation(listAccountsRequest).build());
}
/**
*
* Removes the client- and server-side session that is associated with the user.
*
*
* @param logoutRequest
* @return A Java Future containing the result of the Logout operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.Logout
* @see AWS API
* Documentation
*/
default CompletableFuture logout(LogoutRequest logoutRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes the client- and server-side session that is associated with the user.
*
*
*
* This is a convenience which creates an instance of the {@link LogoutRequest.Builder} avoiding the need to create
* one manually via {@link LogoutRequest#builder()}
*
*
* @param logoutRequest
* A {@link Consumer} that will call methods on {@link LogoutRequest.Builder} to create a request.
* @return A Java Future containing the result of the Logout operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException Indicates that a problem occurred with the input to the request. For example,
* a required parameter might be missing or out of range.
* - UnauthorizedException Indicates that the request is not authorized. This can happen due to an invalid
* access token in the request.
* - TooManyRequestsException Indicates that the request is being made too frequently and is more than
* what the server can handle.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - SsoException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample SsoAsyncClient.Logout
* @see AWS API
* Documentation
*/
default CompletableFuture logout(Consumer logoutRequest) {
return logout(LogoutRequest.builder().applyMutation(logoutRequest).build());
}
}