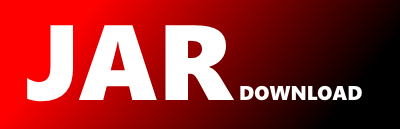
software.amazon.awssdk.services.sso.model.RoleCredentials Maven / Gradle / Ivy
Show all versions of sso Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sso.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information about the role credentials that are assigned to the user.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RoleCredentials implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ACCESS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RoleCredentials::accessKeyId)).setter(setter(Builder::accessKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("accessKeyId").build()).build();
private static final SdkField SECRET_ACCESS_KEY_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RoleCredentials::secretAccessKey)).setter(setter(Builder::secretAccessKey))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("secretAccessKey").build()).build();
private static final SdkField SESSION_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(RoleCredentials::sessionToken)).setter(setter(Builder::sessionToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sessionToken").build()).build();
private static final SdkField EXPIRATION_FIELD = SdkField. builder(MarshallingType.LONG)
.getter(getter(RoleCredentials::expiration)).setter(setter(Builder::expiration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("expiration").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACCESS_KEY_ID_FIELD,
SECRET_ACCESS_KEY_FIELD, SESSION_TOKEN_FIELD, EXPIRATION_FIELD));
private static final long serialVersionUID = 1L;
private final String accessKeyId;
private final String secretAccessKey;
private final String sessionToken;
private final Long expiration;
private RoleCredentials(BuilderImpl builder) {
this.accessKeyId = builder.accessKeyId;
this.secretAccessKey = builder.secretAccessKey;
this.sessionToken = builder.sessionToken;
this.expiration = builder.expiration;
}
/**
*
* The identifier used for the temporary security credentials. For more information, see Using Temporary
* Security Credentials to Request Access to AWS Resources in the AWS IAM User Guide.
*
*
* @return The identifier used for the temporary security credentials. For more information, see Using
* Temporary Security Credentials to Request Access to AWS Resources in the AWS IAM User Guide.
*/
public String accessKeyId() {
return accessKeyId;
}
/**
*
* The key that is used to sign the request. For more information, see Using Temporary
* Security Credentials to Request Access to AWS Resources in the AWS IAM User Guide.
*
*
* @return The key that is used to sign the request. For more information, see Using
* Temporary Security Credentials to Request Access to AWS Resources in the AWS IAM User Guide.
*/
public String secretAccessKey() {
return secretAccessKey;
}
/**
*
* The token used for temporary credentials. For more information, see Using Temporary
* Security Credentials to Request Access to AWS Resources in the AWS IAM User Guide.
*
*
* @return The token used for temporary credentials. For more information, see Using
* Temporary Security Credentials to Request Access to AWS Resources in the AWS IAM User Guide.
*/
public String sessionToken() {
return sessionToken;
}
/**
*
* The date on which temporary security credentials expire.
*
*
* @return The date on which temporary security credentials expire.
*/
public Long expiration() {
return expiration;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(accessKeyId());
hashCode = 31 * hashCode + Objects.hashCode(secretAccessKey());
hashCode = 31 * hashCode + Objects.hashCode(sessionToken());
hashCode = 31 * hashCode + Objects.hashCode(expiration());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RoleCredentials)) {
return false;
}
RoleCredentials other = (RoleCredentials) obj;
return Objects.equals(accessKeyId(), other.accessKeyId()) && Objects.equals(secretAccessKey(), other.secretAccessKey())
&& Objects.equals(sessionToken(), other.sessionToken()) && Objects.equals(expiration(), other.expiration());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("RoleCredentials").add("AccessKeyId", accessKeyId())
.add("SecretAccessKey", secretAccessKey() == null ? null : "*** Sensitive Data Redacted ***")
.add("SessionToken", sessionToken() == null ? null : "*** Sensitive Data Redacted ***")
.add("Expiration", expiration()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "accessKeyId":
return Optional.ofNullable(clazz.cast(accessKeyId()));
case "secretAccessKey":
return Optional.ofNullable(clazz.cast(secretAccessKey()));
case "sessionToken":
return Optional.ofNullable(clazz.cast(sessionToken()));
case "expiration":
return Optional.ofNullable(clazz.cast(expiration()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function