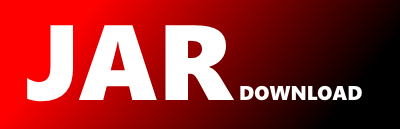
software.amazon.awssdk.services.sso.DefaultSsoClient Maven / Gradle / Ivy
Show all versions of sso Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sso;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.interceptor.SdkInternalExecutionAttribute;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.sso.model.GetRoleCredentialsRequest;
import software.amazon.awssdk.services.sso.model.GetRoleCredentialsResponse;
import software.amazon.awssdk.services.sso.model.InvalidRequestException;
import software.amazon.awssdk.services.sso.model.ListAccountRolesRequest;
import software.amazon.awssdk.services.sso.model.ListAccountRolesResponse;
import software.amazon.awssdk.services.sso.model.ListAccountsRequest;
import software.amazon.awssdk.services.sso.model.ListAccountsResponse;
import software.amazon.awssdk.services.sso.model.LogoutRequest;
import software.amazon.awssdk.services.sso.model.LogoutResponse;
import software.amazon.awssdk.services.sso.model.ResourceNotFoundException;
import software.amazon.awssdk.services.sso.model.SsoException;
import software.amazon.awssdk.services.sso.model.SsoRequest;
import software.amazon.awssdk.services.sso.model.TooManyRequestsException;
import software.amazon.awssdk.services.sso.model.UnauthorizedException;
import software.amazon.awssdk.services.sso.paginators.ListAccountRolesIterable;
import software.amazon.awssdk.services.sso.paginators.ListAccountsIterable;
import software.amazon.awssdk.services.sso.transform.GetRoleCredentialsRequestMarshaller;
import software.amazon.awssdk.services.sso.transform.ListAccountRolesRequestMarshaller;
import software.amazon.awssdk.services.sso.transform.ListAccountsRequestMarshaller;
import software.amazon.awssdk.services.sso.transform.LogoutRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link SsoClient}.
*
* @see SsoClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultSsoClient implements SsoClient {
private static final Logger log = Logger.loggerFor(DefaultSsoClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultSsoClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Returns the STS short-term credentials for a given role name that is assigned to the user.
*
*
* @param getRoleCredentialsRequest
* @return Result of the GetRoleCredentials operation returned by the service.
* @throws InvalidRequestException
* Indicates that a problem occurred with the input to the request. For example, a required parameter might
* be missing or out of range.
* @throws UnauthorizedException
* Indicates that the request is not authorized. This can happen due to an invalid access token in the
* request.
* @throws TooManyRequestsException
* Indicates that the request is being made too frequently and is more than what the server can handle.
* @throws ResourceNotFoundException
* The specified resource doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SsoException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SsoClient.GetRoleCredentials
* @see AWS API
* Documentation
*/
@Override
public GetRoleCredentialsResponse getRoleCredentials(GetRoleCredentialsRequest getRoleCredentialsRequest)
throws InvalidRequestException, UnauthorizedException, TooManyRequestsException, ResourceNotFoundException,
AwsServiceException, SdkClientException, SsoException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRoleCredentialsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRoleCredentialsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SSO");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRoleCredentials");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetRoleCredentials").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getRoleCredentialsRequest)
.withMetricCollector(apiCallMetricCollector)
.putExecutionAttribute(SdkInternalExecutionAttribute.IS_NONE_AUTH_TYPE_REQUEST, false)
.withMarshaller(new GetRoleCredentialsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all roles that are assigned to the user for a given Amazon Web Services account.
*
*
* @param listAccountRolesRequest
* @return Result of the ListAccountRoles operation returned by the service.
* @throws InvalidRequestException
* Indicates that a problem occurred with the input to the request. For example, a required parameter might
* be missing or out of range.
* @throws UnauthorizedException
* Indicates that the request is not authorized. This can happen due to an invalid access token in the
* request.
* @throws TooManyRequestsException
* Indicates that the request is being made too frequently and is more than what the server can handle.
* @throws ResourceNotFoundException
* The specified resource doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SsoException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SsoClient.ListAccountRoles
* @see AWS API
* Documentation
*/
@Override
public ListAccountRolesResponse listAccountRoles(ListAccountRolesRequest listAccountRolesRequest)
throws InvalidRequestException, UnauthorizedException, TooManyRequestsException, ResourceNotFoundException,
AwsServiceException, SdkClientException, SsoException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListAccountRolesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAccountRolesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SSO");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAccountRoles");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListAccountRoles").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listAccountRolesRequest)
.withMetricCollector(apiCallMetricCollector)
.putExecutionAttribute(SdkInternalExecutionAttribute.IS_NONE_AUTH_TYPE_REQUEST, false)
.withMarshaller(new ListAccountRolesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all roles that are assigned to the user for a given Amazon Web Services account.
*
*
*
* This is a variant of {@link #listAccountRoles(software.amazon.awssdk.services.sso.model.ListAccountRolesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountRolesIterable responses = client.listAccountRolesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sso.paginators.ListAccountRolesIterable responses = client.listAccountRolesPaginator(request);
* for (software.amazon.awssdk.services.sso.model.ListAccountRolesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountRolesIterable responses = client.listAccountRolesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccountRoles(software.amazon.awssdk.services.sso.model.ListAccountRolesRequest)} operation.
*
*
* @param listAccountRolesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidRequestException
* Indicates that a problem occurred with the input to the request. For example, a required parameter might
* be missing or out of range.
* @throws UnauthorizedException
* Indicates that the request is not authorized. This can happen due to an invalid access token in the
* request.
* @throws TooManyRequestsException
* Indicates that the request is being made too frequently and is more than what the server can handle.
* @throws ResourceNotFoundException
* The specified resource doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SsoException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SsoClient.ListAccountRoles
* @see AWS API
* Documentation
*/
@Override
public ListAccountRolesIterable listAccountRolesPaginator(ListAccountRolesRequest listAccountRolesRequest)
throws InvalidRequestException, UnauthorizedException, TooManyRequestsException, ResourceNotFoundException,
AwsServiceException, SdkClientException, SsoException {
return new ListAccountRolesIterable(this, applyPaginatorUserAgent(listAccountRolesRequest));
}
/**
*
* Lists all Amazon Web Services accounts assigned to the user. These Amazon Web Services accounts are assigned by
* the administrator of the account. For more information, see Assign User
* Access in the Amazon Web Services SSO User Guide. This operation returns a paginated response.
*
*
* @param listAccountsRequest
* @return Result of the ListAccounts operation returned by the service.
* @throws InvalidRequestException
* Indicates that a problem occurred with the input to the request. For example, a required parameter might
* be missing or out of range.
* @throws UnauthorizedException
* Indicates that the request is not authorized. This can happen due to an invalid access token in the
* request.
* @throws TooManyRequestsException
* Indicates that the request is being made too frequently and is more than what the server can handle.
* @throws ResourceNotFoundException
* The specified resource doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SsoException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SsoClient.ListAccounts
* @see AWS API
* Documentation
*/
@Override
public ListAccountsResponse listAccounts(ListAccountsRequest listAccountsRequest) throws InvalidRequestException,
UnauthorizedException, TooManyRequestsException, ResourceNotFoundException, AwsServiceException, SdkClientException,
SsoException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListAccountsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAccountsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SSO");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAccounts");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListAccounts").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listAccountsRequest)
.withMetricCollector(apiCallMetricCollector)
.putExecutionAttribute(SdkInternalExecutionAttribute.IS_NONE_AUTH_TYPE_REQUEST, false)
.withMarshaller(new ListAccountsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all Amazon Web Services accounts assigned to the user. These Amazon Web Services accounts are assigned by
* the administrator of the account. For more information, see Assign User
* Access in the Amazon Web Services SSO User Guide. This operation returns a paginated response.
*
*
*
* This is a variant of {@link #listAccounts(software.amazon.awssdk.services.sso.model.ListAccountsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountsIterable responses = client.listAccountsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.sso.paginators.ListAccountsIterable responses = client.listAccountsPaginator(request);
* for (software.amazon.awssdk.services.sso.model.ListAccountsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.sso.paginators.ListAccountsIterable responses = client.listAccountsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccounts(software.amazon.awssdk.services.sso.model.ListAccountsRequest)} operation.
*
*
* @param listAccountsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidRequestException
* Indicates that a problem occurred with the input to the request. For example, a required parameter might
* be missing or out of range.
* @throws UnauthorizedException
* Indicates that the request is not authorized. This can happen due to an invalid access token in the
* request.
* @throws TooManyRequestsException
* Indicates that the request is being made too frequently and is more than what the server can handle.
* @throws ResourceNotFoundException
* The specified resource doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SsoException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SsoClient.ListAccounts
* @see AWS API
* Documentation
*/
@Override
public ListAccountsIterable listAccountsPaginator(ListAccountsRequest listAccountsRequest) throws InvalidRequestException,
UnauthorizedException, TooManyRequestsException, ResourceNotFoundException, AwsServiceException, SdkClientException,
SsoException {
return new ListAccountsIterable(this, applyPaginatorUserAgent(listAccountsRequest));
}
/**
*
* Removes the locally stored SSO tokens from the client-side cache and sends an API call to the Amazon Web Services
* SSO service to invalidate the corresponding server-side Amazon Web Services SSO sign in session.
*
*
*
* If a user uses Amazon Web Services SSO to access the AWS CLI, the user’s Amazon Web Services SSO sign in session
* is used to obtain an IAM session, as specified in the corresponding Amazon Web Services SSO permission set. More
* specifically, Amazon Web Services SSO assumes an IAM role in the target account on behalf of the user, and the
* corresponding temporary Amazon Web Services credentials are returned to the client.
*
*
* After user logout, any existing IAM role sessions that were created by using Amazon Web Services SSO permission
* sets continue based on the duration configured in the permission set. For more information, see User authentications in the
* Amazon Web Services SSO User Guide.
*
*
*
* @param logoutRequest
* @return Result of the Logout operation returned by the service.
* @throws InvalidRequestException
* Indicates that a problem occurred with the input to the request. For example, a required parameter might
* be missing or out of range.
* @throws UnauthorizedException
* Indicates that the request is not authorized. This can happen due to an invalid access token in the
* request.
* @throws TooManyRequestsException
* Indicates that the request is being made too frequently and is more than what the server can handle.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws SsoException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample SsoClient.Logout
* @see AWS API
* Documentation
*/
@Override
public LogoutResponse logout(LogoutRequest logoutRequest) throws InvalidRequestException, UnauthorizedException,
TooManyRequestsException, AwsServiceException, SdkClientException, SsoException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
LogoutResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, logoutRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "SSO");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "Logout");
return clientHandler.execute(new ClientExecutionParams().withOperationName("Logout")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler).withInput(logoutRequest)
.withMetricCollector(apiCallMetricCollector)
.putExecutionAttribute(SdkInternalExecutionAttribute.IS_NONE_AUTH_TYPE_REQUEST, false)
.withMarshaller(new LogoutRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(SsoException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("TooManyRequestsException")
.exceptionBuilderSupplier(TooManyRequestsException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnauthorizedException")
.exceptionBuilderSupplier(UnauthorizedException::builder).httpStatusCode(401).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidRequestException")
.exceptionBuilderSupplier(InvalidRequestException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build());
}
@Override
public void close() {
clientHandler.close();
}
private T applyPaginatorUserAgent(T request) {
Consumer userAgentApplier = b -> b.addApiName(ApiName.builder()
.version(VersionInfo.SDK_VERSION).name("PAGINATED").build());
AwsRequestOverrideConfiguration overrideConfiguration = request.overrideConfiguration()
.map(c -> c.toBuilder().applyMutation(userAgentApplier).build())
.orElse((AwsRequestOverrideConfiguration.builder().applyMutation(userAgentApplier).build()));
return (T) request.toBuilder().overrideConfiguration(overrideConfiguration).build();
}
}