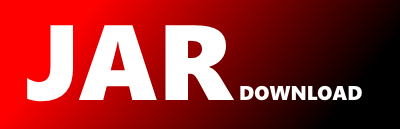
software.amazon.awssdk.services.sts.model.AssumeRoleRequest Maven / Gradle / Ivy
Show all versions of sts Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.sts.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class AssumeRoleRequest extends StsRequest implements
ToCopyableBuilder {
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleArn").getter(getter(AssumeRoleRequest::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleArn").build()).build();
private static final SdkField ROLE_SESSION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RoleSessionName").getter(getter(AssumeRoleRequest::roleSessionName))
.setter(setter(Builder::roleSessionName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleSessionName").build()).build();
private static final SdkField> POLICY_ARNS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("PolicyArns")
.getter(getter(AssumeRoleRequest::policyArns))
.setter(setter(Builder::policyArns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PolicyArns").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PolicyDescriptorType::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField POLICY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Policy")
.getter(getter(AssumeRoleRequest::policy)).setter(setter(Builder::policy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Policy").build()).build();
private static final SdkField DURATION_SECONDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("DurationSeconds").getter(getter(AssumeRoleRequest::durationSeconds))
.setter(setter(Builder::durationSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DurationSeconds").build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(AssumeRoleRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> TRANSITIVE_TAG_KEYS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("TransitiveTagKeys")
.getter(getter(AssumeRoleRequest::transitiveTagKeys))
.setter(setter(Builder::transitiveTagKeys))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TransitiveTagKeys").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField EXTERNAL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ExternalId").getter(getter(AssumeRoleRequest::externalId)).setter(setter(Builder::externalId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExternalId").build()).build();
private static final SdkField SERIAL_NUMBER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SerialNumber").getter(getter(AssumeRoleRequest::serialNumber)).setter(setter(Builder::serialNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SerialNumber").build()).build();
private static final SdkField TOKEN_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TokenCode").getter(getter(AssumeRoleRequest::tokenCode)).setter(setter(Builder::tokenCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TokenCode").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ROLE_ARN_FIELD,
ROLE_SESSION_NAME_FIELD, POLICY_ARNS_FIELD, POLICY_FIELD, DURATION_SECONDS_FIELD, TAGS_FIELD,
TRANSITIVE_TAG_KEYS_FIELD, EXTERNAL_ID_FIELD, SERIAL_NUMBER_FIELD, TOKEN_CODE_FIELD));
private final String roleArn;
private final String roleSessionName;
private final List policyArns;
private final String policy;
private final Integer durationSeconds;
private final List tags;
private final List transitiveTagKeys;
private final String externalId;
private final String serialNumber;
private final String tokenCode;
private AssumeRoleRequest(BuilderImpl builder) {
super(builder);
this.roleArn = builder.roleArn;
this.roleSessionName = builder.roleSessionName;
this.policyArns = builder.policyArns;
this.policy = builder.policy;
this.durationSeconds = builder.durationSeconds;
this.tags = builder.tags;
this.transitiveTagKeys = builder.transitiveTagKeys;
this.externalId = builder.externalId;
this.serialNumber = builder.serialNumber;
this.tokenCode = builder.tokenCode;
}
/**
*
* The Amazon Resource Name (ARN) of the role to assume.
*
*
* @return The Amazon Resource Name (ARN) of the role to assume.
*/
public String roleArn() {
return roleArn;
}
/**
*
* An identifier for the assumed role session.
*
*
* Use the role session name to uniquely identify a session when the same role is assumed by different principals or
* for different reasons. In cross-account scenarios, the role session name is visible to, and can be logged by the
* account that owns the role. The role session name is also used in the ARN of the assumed role principal. This
* means that subsequent cross-account API requests that use the temporary security credentials will expose the role
* session name to the external account in their AWS CloudTrail logs.
*
*
* The regex used to validate this parameter is a string of characters consisting of upper- and lower-case
* alphanumeric characters with no spaces. You can also include underscores or any of the following characters: =,.@-
*
*
* @return An identifier for the assumed role session.
*
* Use the role session name to uniquely identify a session when the same role is assumed by different
* principals or for different reasons. In cross-account scenarios, the role session name is visible to, and
* can be logged by the account that owns the role. The role session name is also used in the ARN of the
* assumed role principal. This means that subsequent cross-account API requests that use the temporary
* security credentials will expose the role session name to the external account in their AWS CloudTrail
* logs.
*
*
* The regex used to validate this parameter is a string of characters consisting of upper- and lower-case
* alphanumeric characters with no spaces. You can also include underscores or any of the following
* characters: =,.@-
*/
public String roleSessionName() {
return roleSessionName;
}
/**
* Returns true if the PolicyArns property was specified by the sender (it may be empty), or false if the sender did
* not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasPolicyArns() {
return policyArns != null && !(policyArns instanceof SdkAutoConstructList);
}
/**
*
* The Amazon Resource Names (ARNs) of the IAM managed policies that you want to use as managed session policies.
* The policies must exist in the same account as the role.
*
*
* This parameter is optional. You can provide up to 10 managed policy ARNs. However, the plain text that you use
* for both inline and managed session policies can't exceed 2,048 characters. For more information about ARNs, see
* Amazon Resource Names (ARNs)
* and AWS Service Namespaces in the AWS General Reference.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format that has a
* separate limit. Your request can fail for this limit even if your plain text meets the other requirements. The
* PackedPolicySize
response element indicates by percentage how close the policies and tags for your
* request are to the upper size limit.
*
*
*
* Passing policies to this operation returns new temporary credentials. The resulting session's permissions are the
* intersection of the role's identity-based policy and the session policies. You can use the role's temporary
* credentials in subsequent AWS API calls to access resources in the account that owns the role. You cannot use
* session policies to grant more permissions than those allowed by the identity-based policy of the role that is
* being assumed. For more information, see Session
* Policies in the IAM User Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasPolicyArns()} to see if a value was sent in this field.
*
*
* @return The Amazon Resource Names (ARNs) of the IAM managed policies that you want to use as managed session
* policies. The policies must exist in the same account as the role.
*
* This parameter is optional. You can provide up to 10 managed policy ARNs. However, the plain text that
* you use for both inline and managed session policies can't exceed 2,048 characters. For more information
* about ARNs, see Amazon Resource Names
* (ARNs) and AWS Service Namespaces in the AWS General Reference.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format
* that has a separate limit. Your request can fail for this limit even if your plain text meets the other
* requirements. The PackedPolicySize
response element indicates by percentage how close the
* policies and tags for your request are to the upper size limit.
*
*
*
* Passing policies to this operation returns new temporary credentials. The resulting session's permissions
* are the intersection of the role's identity-based policy and the session policies. You can use the role's
* temporary credentials in subsequent AWS API calls to access resources in the account that owns the role.
* You cannot use session policies to grant more permissions than those allowed by the identity-based policy
* of the role that is being assumed. For more information, see Session
* Policies in the IAM User Guide.
*/
public List policyArns() {
return policyArns;
}
/**
*
* An IAM policy in JSON format that you want to use as an inline session policy.
*
*
* This parameter is optional. Passing policies to this operation returns new temporary credentials. The resulting
* session's permissions are the intersection of the role's identity-based policy and the session policies. You can
* use the role's temporary credentials in subsequent AWS API calls to access resources in the account that owns the
* role. You cannot use session policies to grant more permissions than those allowed by the identity-based policy
* of the role that is being assumed. For more information, see Session
* Policies in the IAM User Guide.
*
*
* The plain text that you use for both inline and managed session policies can't exceed 2,048 characters. The JSON
* policy characters can be any ASCII character from the space character to the end of the valid character list (
* through \u00FF). It can also include the tab ( ), linefeed ( ), and carriage return ( ) characters.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format that has a
* separate limit. Your request can fail for this limit even if your plain text meets the other requirements. The
* PackedPolicySize
response element indicates by percentage how close the policies and tags for your
* request are to the upper size limit.
*
*
*
* @return An IAM policy in JSON format that you want to use as an inline session policy.
*
* This parameter is optional. Passing policies to this operation returns new temporary credentials. The
* resulting session's permissions are the intersection of the role's identity-based policy and the session
* policies. You can use the role's temporary credentials in subsequent AWS API calls to access resources in
* the account that owns the role. You cannot use session policies to grant more permissions than those
* allowed by the identity-based policy of the role that is being assumed. For more information, see Session
* Policies in the IAM User Guide.
*
*
* The plain text that you use for both inline and managed session policies can't exceed 2,048 characters.
* The JSON policy characters can be any ASCII character from the space character to the end of the valid
* character list ( through \u00FF). It can also include the tab ( ), linefeed ( ), and carriage return ( )
* characters.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format
* that has a separate limit. Your request can fail for this limit even if your plain text meets the other
* requirements. The PackedPolicySize
response element indicates by percentage how close the
* policies and tags for your request are to the upper size limit.
*
*/
public String policy() {
return policy;
}
/**
*
* The duration, in seconds, of the role session. The value can range from 900 seconds (15 minutes) up to the
* maximum session duration setting for the role. This setting can have a value from 1 hour to 12 hours. If you
* specify a value higher than this setting, the operation fails. For example, if you specify a session duration of
* 12 hours, but your administrator set the maximum session duration to 6 hours, your operation fails. To learn how
* to view the maximum value for your role, see View
* the Maximum Session Duration Setting for a Role in the IAM User Guide.
*
*
* By default, the value is set to 3600
seconds.
*
*
*
* The DurationSeconds
parameter is separate from the duration of a console session that you might
* request using the returned credentials. The request to the federation endpoint for a console sign-in token takes
* a SessionDuration
parameter that specifies the maximum length of the console session. For more
* information, see Creating a URL that Enables Federated Users to Access the AWS Management Console in the IAM User
* Guide.
*
*
*
* @return The duration, in seconds, of the role session. The value can range from 900 seconds (15 minutes) up to
* the maximum session duration setting for the role. This setting can have a value from 1 hour to 12 hours.
* If you specify a value higher than this setting, the operation fails. For example, if you specify a
* session duration of 12 hours, but your administrator set the maximum session duration to 6 hours, your
* operation fails. To learn how to view the maximum value for your role, see View the Maximum Session Duration Setting for a Role in the IAM User Guide.
*
* By default, the value is set to 3600
seconds.
*
*
*
* The DurationSeconds
parameter is separate from the duration of a console session that you
* might request using the returned credentials. The request to the federation endpoint for a console
* sign-in token takes a SessionDuration
parameter that specifies the maximum length of the
* console session. For more information, see Creating a URL that Enables Federated Users to Access the AWS Management Console in the IAM User
* Guide.
*
*/
public Integer durationSeconds() {
return durationSeconds;
}
/**
* Returns true if the Tags property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* A list of session tags that you want to pass. Each session tag consists of a key name and an associated value.
* For more information about session tags, see Tagging AWS STS Sessions in the
* IAM User Guide.
*
*
* This parameter is optional. You can pass up to 50 session tags. The plain text session tag keys can’t exceed 128
* characters, and the values can’t exceed 256 characters. For these and additional limits, see IAM and STS Character Limits in the IAM User Guide.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format that has a
* separate limit. Your request can fail for this limit even if your plain text meets the other requirements. The
* PackedPolicySize
response element indicates by percentage how close the policies and tags for your
* request are to the upper size limit.
*
*
*
* You can pass a session tag with the same key as a tag that is already attached to the role. When you do, session
* tags override a role tag with the same key.
*
*
* Tag key–value pairs are not case sensitive, but case is preserved. This means that you cannot have separate
* Department
and department
tag keys. Assume that the role has the
* Department
=Marketing
tag and you pass the department
=
* engineering
session tag. Department
and department
are not saved as
* separate tags, and the session tag passed in the request takes precedence over the role tag.
*
*
* Additionally, if you used temporary credentials to perform this operation, the new session inherits any
* transitive session tags from the calling session. If you pass a session tag with the same key as an inherited
* tag, the operation fails. To view the inherited tags for a session, see the AWS CloudTrail logs. For more
* information, see Viewing Session
* Tags in CloudTrail in the IAM User Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTags()} to see if a value was sent in this field.
*
*
* @return A list of session tags that you want to pass. Each session tag consists of a key name and an associated
* value. For more information about session tags, see Tagging AWS STS Sessions
* in the IAM User Guide.
*
* This parameter is optional. You can pass up to 50 session tags. The plain text session tag keys can’t
* exceed 128 characters, and the values can’t exceed 256 characters. For these and additional limits, see
* IAM and STS Character Limits in the IAM User Guide.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format
* that has a separate limit. Your request can fail for this limit even if your plain text meets the other
* requirements. The PackedPolicySize
response element indicates by percentage how close the
* policies and tags for your request are to the upper size limit.
*
*
*
* You can pass a session tag with the same key as a tag that is already attached to the role. When you do,
* session tags override a role tag with the same key.
*
*
* Tag key–value pairs are not case sensitive, but case is preserved. This means that you cannot have
* separate Department
and department
tag keys. Assume that the role has the
* Department
=Marketing
tag and you pass the department
=
* engineering
session tag. Department
and department
are not saved
* as separate tags, and the session tag passed in the request takes precedence over the role tag.
*
*
* Additionally, if you used temporary credentials to perform this operation, the new session inherits any
* transitive session tags from the calling session. If you pass a session tag with the same key as an
* inherited tag, the operation fails. To view the inherited tags for a session, see the AWS CloudTrail
* logs. For more information, see Viewing
* Session Tags in CloudTrail in the IAM User Guide.
*/
public List tags() {
return tags;
}
/**
* Returns true if the TransitiveTagKeys property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasTransitiveTagKeys() {
return transitiveTagKeys != null && !(transitiveTagKeys instanceof SdkAutoConstructList);
}
/**
*
* A list of keys for session tags that you want to set as transitive. If you set a tag key as transitive, the
* corresponding key and value passes to subsequent sessions in a role chain. For more information, see Chaining Roles with Session Tags in the IAM User Guide.
*
*
* This parameter is optional. When you set session tags as transitive, the session policy and session tags packed
* binary limit is not affected.
*
*
* If you choose not to specify a transitive tag key, then no tags are passed from this session to any subsequent
* sessions.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasTransitiveTagKeys()} to see if a value was sent in this field.
*
*
* @return A list of keys for session tags that you want to set as transitive. If you set a tag key as transitive,
* the corresponding key and value passes to subsequent sessions in a role chain. For more information, see
* Chaining Roles with Session Tags in the IAM User Guide.
*
* This parameter is optional. When you set session tags as transitive, the session policy and session tags
* packed binary limit is not affected.
*
*
* If you choose not to specify a transitive tag key, then no tags are passed from this session to any
* subsequent sessions.
*/
public List transitiveTagKeys() {
return transitiveTagKeys;
}
/**
*
* A unique identifier that might be required when you assume a role in another account. If the administrator of the
* account to which the role belongs provided you with an external ID, then provide that value in the
* ExternalId
parameter. This value can be any string, such as a passphrase or account number. A
* cross-account role is usually set up to trust everyone in an account. Therefore, the administrator of the
* trusting account might send an external ID to the administrator of the trusted account. That way, only someone
* with the ID can assume the role, rather than everyone in the account. For more information about the external ID,
* see How to
* Use an External ID When Granting Access to Your AWS Resources to a Third Party in the IAM User Guide.
*
*
* The regex used to validate this parameter is a string of characters consisting of upper- and lower-case
* alphanumeric characters with no spaces. You can also include underscores or any of the following characters:
* =,.@:/-
*
*
* @return A unique identifier that might be required when you assume a role in another account. If the
* administrator of the account to which the role belongs provided you with an external ID, then provide
* that value in the ExternalId
parameter. This value can be any string, such as a passphrase
* or account number. A cross-account role is usually set up to trust everyone in an account. Therefore, the
* administrator of the trusting account might send an external ID to the administrator of the trusted
* account. That way, only someone with the ID can assume the role, rather than everyone in the account. For
* more information about the external ID, see How to
* Use an External ID When Granting Access to Your AWS Resources to a Third Party in the IAM User
* Guide.
*
* The regex used to validate this parameter is a string of characters consisting of upper- and lower-case
* alphanumeric characters with no spaces. You can also include underscores or any of the following
* characters: =,.@:/-
*/
public String externalId() {
return externalId;
}
/**
*
* The identification number of the MFA device that is associated with the user who is making the
* AssumeRole
call. Specify this value if the trust policy of the role being assumed includes a
* condition that requires MFA authentication. The value is either the serial number for a hardware device (such as
* GAHT12345678
) or an Amazon Resource Name (ARN) for a virtual device (such as
* arn:aws:iam::123456789012:mfa/user
).
*
*
* The regex used to validate this parameter is a string of characters consisting of upper- and lower-case
* alphanumeric characters with no spaces. You can also include underscores or any of the following characters: =,.@-
*
*
* @return The identification number of the MFA device that is associated with the user who is making the
* AssumeRole
call. Specify this value if the trust policy of the role being assumed includes a
* condition that requires MFA authentication. The value is either the serial number for a hardware device
* (such as GAHT12345678
) or an Amazon Resource Name (ARN) for a virtual device (such as
* arn:aws:iam::123456789012:mfa/user
).
*
* The regex used to validate this parameter is a string of characters consisting of upper- and lower-case
* alphanumeric characters with no spaces. You can also include underscores or any of the following
* characters: =,.@-
*/
public String serialNumber() {
return serialNumber;
}
/**
*
* The value provided by the MFA device, if the trust policy of the role being assumed requires MFA (that is, if the
* policy includes a condition that tests for MFA). If the role being assumed requires MFA and if the
* TokenCode
value is missing or expired, the AssumeRole
call returns an "access denied"
* error.
*
*
* The format for this parameter, as described by its regex pattern, is a sequence of six numeric digits.
*
*
* @return The value provided by the MFA device, if the trust policy of the role being assumed requires MFA (that
* is, if the policy includes a condition that tests for MFA). If the role being assumed requires MFA and if
* the TokenCode
value is missing or expired, the AssumeRole
call returns an
* "access denied" error.
*
* The format for this parameter, as described by its regex pattern, is a sequence of six numeric digits.
*/
public String tokenCode() {
return tokenCode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(roleSessionName());
hashCode = 31 * hashCode + Objects.hashCode(policyArns());
hashCode = 31 * hashCode + Objects.hashCode(policy());
hashCode = 31 * hashCode + Objects.hashCode(durationSeconds());
hashCode = 31 * hashCode + Objects.hashCode(tags());
hashCode = 31 * hashCode + Objects.hashCode(transitiveTagKeys());
hashCode = 31 * hashCode + Objects.hashCode(externalId());
hashCode = 31 * hashCode + Objects.hashCode(serialNumber());
hashCode = 31 * hashCode + Objects.hashCode(tokenCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AssumeRoleRequest)) {
return false;
}
AssumeRoleRequest other = (AssumeRoleRequest) obj;
return Objects.equals(roleArn(), other.roleArn()) && Objects.equals(roleSessionName(), other.roleSessionName())
&& Objects.equals(policyArns(), other.policyArns()) && Objects.equals(policy(), other.policy())
&& Objects.equals(durationSeconds(), other.durationSeconds()) && Objects.equals(tags(), other.tags())
&& Objects.equals(transitiveTagKeys(), other.transitiveTagKeys())
&& Objects.equals(externalId(), other.externalId()) && Objects.equals(serialNumber(), other.serialNumber())
&& Objects.equals(tokenCode(), other.tokenCode());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("AssumeRoleRequest").add("RoleArn", roleArn()).add("RoleSessionName", roleSessionName())
.add("PolicyArns", policyArns()).add("Policy", policy()).add("DurationSeconds", durationSeconds())
.add("Tags", tags()).add("TransitiveTagKeys", transitiveTagKeys()).add("ExternalId", externalId())
.add("SerialNumber", serialNumber()).add("TokenCode", tokenCode()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RoleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "RoleSessionName":
return Optional.ofNullable(clazz.cast(roleSessionName()));
case "PolicyArns":
return Optional.ofNullable(clazz.cast(policyArns()));
case "Policy":
return Optional.ofNullable(clazz.cast(policy()));
case "DurationSeconds":
return Optional.ofNullable(clazz.cast(durationSeconds()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "TransitiveTagKeys":
return Optional.ofNullable(clazz.cast(transitiveTagKeys()));
case "ExternalId":
return Optional.ofNullable(clazz.cast(externalId()));
case "SerialNumber":
return Optional.ofNullable(clazz.cast(serialNumber()));
case "TokenCode":
return Optional.ofNullable(clazz.cast(tokenCode()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Use the role session name to uniquely identify a session when the same role is assumed by different
* principals or for different reasons. In cross-account scenarios, the role session name is visible to,
* and can be logged by the account that owns the role. The role session name is also used in the ARN of
* the assumed role principal. This means that subsequent cross-account API requests that use the
* temporary security credentials will expose the role session name to the external account in their AWS
* CloudTrail logs.
*
*
* The regex used to validate this parameter is a string of characters consisting of upper- and
* lower-case alphanumeric characters with no spaces. You can also include underscores or any of the
* following characters: =,.@-
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder roleSessionName(String roleSessionName);
/**
*
* The Amazon Resource Names (ARNs) of the IAM managed policies that you want to use as managed session
* policies. The policies must exist in the same account as the role.
*
*
* This parameter is optional. You can provide up to 10 managed policy ARNs. However, the plain text that you
* use for both inline and managed session policies can't exceed 2,048 characters. For more information about
* ARNs, see Amazon
* Resource Names (ARNs) and AWS Service Namespaces in the AWS General Reference.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format that
* has a separate limit. Your request can fail for this limit even if your plain text meets the other
* requirements. The PackedPolicySize
response element indicates by percentage how close the
* policies and tags for your request are to the upper size limit.
*
*
*
* Passing policies to this operation returns new temporary credentials. The resulting session's permissions are
* the intersection of the role's identity-based policy and the session policies. You can use the role's
* temporary credentials in subsequent AWS API calls to access resources in the account that owns the role. You
* cannot use session policies to grant more permissions than those allowed by the identity-based policy of the
* role that is being assumed. For more information, see Session
* Policies in the IAM User Guide.
*
*
* @param policyArns
* The Amazon Resource Names (ARNs) of the IAM managed policies that you want to use as managed session
* policies. The policies must exist in the same account as the role.
*
* This parameter is optional. You can provide up to 10 managed policy ARNs. However, the plain text that
* you use for both inline and managed session policies can't exceed 2,048 characters. For more
* information about ARNs, see Amazon Resource
* Names (ARNs) and AWS Service Namespaces in the AWS General Reference.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format
* that has a separate limit. Your request can fail for this limit even if your plain text meets the
* other requirements. The PackedPolicySize
response element indicates by percentage how
* close the policies and tags for your request are to the upper size limit.
*
*
*
* Passing policies to this operation returns new temporary credentials. The resulting session's
* permissions are the intersection of the role's identity-based policy and the session policies. You can
* use the role's temporary credentials in subsequent AWS API calls to access resources in the account
* that owns the role. You cannot use session policies to grant more permissions than those allowed by
* the identity-based policy of the role that is being assumed. For more information, see Session
* Policies in the IAM User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder policyArns(Collection policyArns);
/**
*
* The Amazon Resource Names (ARNs) of the IAM managed policies that you want to use as managed session
* policies. The policies must exist in the same account as the role.
*
*
* This parameter is optional. You can provide up to 10 managed policy ARNs. However, the plain text that you
* use for both inline and managed session policies can't exceed 2,048 characters. For more information about
* ARNs, see Amazon
* Resource Names (ARNs) and AWS Service Namespaces in the AWS General Reference.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format that
* has a separate limit. Your request can fail for this limit even if your plain text meets the other
* requirements. The PackedPolicySize
response element indicates by percentage how close the
* policies and tags for your request are to the upper size limit.
*
*
*
* Passing policies to this operation returns new temporary credentials. The resulting session's permissions are
* the intersection of the role's identity-based policy and the session policies. You can use the role's
* temporary credentials in subsequent AWS API calls to access resources in the account that owns the role. You
* cannot use session policies to grant more permissions than those allowed by the identity-based policy of the
* role that is being assumed. For more information, see Session
* Policies in the IAM User Guide.
*
*
* @param policyArns
* The Amazon Resource Names (ARNs) of the IAM managed policies that you want to use as managed session
* policies. The policies must exist in the same account as the role.
*
* This parameter is optional. You can provide up to 10 managed policy ARNs. However, the plain text that
* you use for both inline and managed session policies can't exceed 2,048 characters. For more
* information about ARNs, see Amazon Resource
* Names (ARNs) and AWS Service Namespaces in the AWS General Reference.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format
* that has a separate limit. Your request can fail for this limit even if your plain text meets the
* other requirements. The PackedPolicySize
response element indicates by percentage how
* close the policies and tags for your request are to the upper size limit.
*
*
*
* Passing policies to this operation returns new temporary credentials. The resulting session's
* permissions are the intersection of the role's identity-based policy and the session policies. You can
* use the role's temporary credentials in subsequent AWS API calls to access resources in the account
* that owns the role. You cannot use session policies to grant more permissions than those allowed by
* the identity-based policy of the role that is being assumed. For more information, see Session
* Policies in the IAM User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder policyArns(PolicyDescriptorType... policyArns);
/**
*
* The Amazon Resource Names (ARNs) of the IAM managed policies that you want to use as managed session
* policies. The policies must exist in the same account as the role.
*
*
* This parameter is optional. You can provide up to 10 managed policy ARNs. However, the plain text that you
* use for both inline and managed session policies can't exceed 2,048 characters. For more information about
* ARNs, see Amazon
* Resource Names (ARNs) and AWS Service Namespaces in the AWS General Reference.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format that
* has a separate limit. Your request can fail for this limit even if your plain text meets the other
* requirements. The PackedPolicySize
response element indicates by percentage how close the
* policies and tags for your request are to the upper size limit.
*
*
*
* Passing policies to this operation returns new temporary credentials. The resulting session's permissions are
* the intersection of the role's identity-based policy and the session policies. You can use the role's
* temporary credentials in subsequent AWS API calls to access resources in the account that owns the role. You
* cannot use session policies to grant more permissions than those allowed by the identity-based policy of the
* role that is being assumed. For more information, see Session
* Policies in the IAM User Guide.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the
* need to create one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately
* and its result is passed to {@link #policyArns(List)}.
*
* @param policyArns
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #policyArns(List)
*/
Builder policyArns(Consumer... policyArns);
/**
*
* An IAM policy in JSON format that you want to use as an inline session policy.
*
*
* This parameter is optional. Passing policies to this operation returns new temporary credentials. The
* resulting session's permissions are the intersection of the role's identity-based policy and the session
* policies. You can use the role's temporary credentials in subsequent AWS API calls to access resources in the
* account that owns the role. You cannot use session policies to grant more permissions than those allowed by
* the identity-based policy of the role that is being assumed. For more information, see Session
* Policies in the IAM User Guide.
*
*
* The plain text that you use for both inline and managed session policies can't exceed 2,048 characters. The
* JSON policy characters can be any ASCII character from the space character to the end of the valid character
* list ( through \u00FF). It can also include the tab ( ), linefeed ( ), and carriage return ( ) characters.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format that
* has a separate limit. Your request can fail for this limit even if your plain text meets the other
* requirements. The PackedPolicySize
response element indicates by percentage how close the
* policies and tags for your request are to the upper size limit.
*
*
*
* @param policy
* An IAM policy in JSON format that you want to use as an inline session policy.
*
* This parameter is optional. Passing policies to this operation returns new temporary credentials. The
* resulting session's permissions are the intersection of the role's identity-based policy and the
* session policies. You can use the role's temporary credentials in subsequent AWS API calls to access
* resources in the account that owns the role. You cannot use session policies to grant more permissions
* than those allowed by the identity-based policy of the role that is being assumed. For more
* information, see Session
* Policies in the IAM User Guide.
*
*
* The plain text that you use for both inline and managed session policies can't exceed 2,048
* characters. The JSON policy characters can be any ASCII character from the space character to the end
* of the valid character list ( through \u00FF). It can also include the tab ( ), linefeed ( ), and
* carriage return ( ) characters.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format
* that has a separate limit. Your request can fail for this limit even if your plain text meets the
* other requirements. The PackedPolicySize
response element indicates by percentage how
* close the policies and tags for your request are to the upper size limit.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder policy(String policy);
/**
*
* The duration, in seconds, of the role session. The value can range from 900 seconds (15 minutes) up to the
* maximum session duration setting for the role. This setting can have a value from 1 hour to 12 hours. If you
* specify a value higher than this setting, the operation fails. For example, if you specify a session duration
* of 12 hours, but your administrator set the maximum session duration to 6 hours, your operation fails. To
* learn how to view the maximum value for your role, see View
* the Maximum Session Duration Setting for a Role in the IAM User Guide.
*
*
* By default, the value is set to 3600
seconds.
*
*
*
* The DurationSeconds
parameter is separate from the duration of a console session that you might
* request using the returned credentials. The request to the federation endpoint for a console sign-in token
* takes a SessionDuration
parameter that specifies the maximum length of the console session. For
* more information, see Creating a URL that Enables Federated Users to Access the AWS Management Console in the IAM User
* Guide.
*
*
*
* @param durationSeconds
* The duration, in seconds, of the role session. The value can range from 900 seconds (15 minutes) up to
* the maximum session duration setting for the role. This setting can have a value from 1 hour to 12
* hours. If you specify a value higher than this setting, the operation fails. For example, if you
* specify a session duration of 12 hours, but your administrator set the maximum session duration to 6
* hours, your operation fails. To learn how to view the maximum value for your role, see View the Maximum Session Duration Setting for a Role in the IAM User Guide.
*
* By default, the value is set to 3600
seconds.
*
*
*
* The DurationSeconds
parameter is separate from the duration of a console session that you
* might request using the returned credentials. The request to the federation endpoint for a console
* sign-in token takes a SessionDuration
parameter that specifies the maximum length of the
* console session. For more information, see Creating a URL that Enables Federated Users to Access the AWS Management Console in the IAM
* User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder durationSeconds(Integer durationSeconds);
/**
*
* A list of session tags that you want to pass. Each session tag consists of a key name and an associated
* value. For more information about session tags, see Tagging AWS STS Sessions in
* the IAM User Guide.
*
*
* This parameter is optional. You can pass up to 50 session tags. The plain text session tag keys can’t exceed
* 128 characters, and the values can’t exceed 256 characters. For these and additional limits, see IAM and STS Character Limits in the IAM User Guide.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format that
* has a separate limit. Your request can fail for this limit even if your plain text meets the other
* requirements. The PackedPolicySize
response element indicates by percentage how close the
* policies and tags for your request are to the upper size limit.
*
*
*
* You can pass a session tag with the same key as a tag that is already attached to the role. When you do,
* session tags override a role tag with the same key.
*
*
* Tag key–value pairs are not case sensitive, but case is preserved. This means that you cannot have separate
* Department
and department
tag keys. Assume that the role has the
* Department
=Marketing
tag and you pass the department
=
* engineering
session tag. Department
and department
are not saved as
* separate tags, and the session tag passed in the request takes precedence over the role tag.
*
*
* Additionally, if you used temporary credentials to perform this operation, the new session inherits any
* transitive session tags from the calling session. If you pass a session tag with the same key as an inherited
* tag, the operation fails. To view the inherited tags for a session, see the AWS CloudTrail logs. For more
* information, see Viewing
* Session Tags in CloudTrail in the IAM User Guide.
*
*
* @param tags
* A list of session tags that you want to pass. Each session tag consists of a key name and an
* associated value. For more information about session tags, see Tagging AWS STS
* Sessions in the IAM User Guide.
*
* This parameter is optional. You can pass up to 50 session tags. The plain text session tag keys can’t
* exceed 128 characters, and the values can’t exceed 256 characters. For these and additional limits,
* see IAM and STS Character Limits in the IAM User Guide.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format
* that has a separate limit. Your request can fail for this limit even if your plain text meets the
* other requirements. The PackedPolicySize
response element indicates by percentage how
* close the policies and tags for your request are to the upper size limit.
*
*
*
* You can pass a session tag with the same key as a tag that is already attached to the role. When you
* do, session tags override a role tag with the same key.
*
*
* Tag key–value pairs are not case sensitive, but case is preserved. This means that you cannot have
* separate Department
and department
tag keys. Assume that the role has the
* Department
=Marketing
tag and you pass the department
=
* engineering
session tag. Department
and department
are not
* saved as separate tags, and the session tag passed in the request takes precedence over the role tag.
*
*
* Additionally, if you used temporary credentials to perform this operation, the new session inherits
* any transitive session tags from the calling session. If you pass a session tag with the same key as
* an inherited tag, the operation fails. To view the inherited tags for a session, see the AWS
* CloudTrail logs. For more information, see Viewing Session Tags in CloudTrail in the IAM User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* A list of session tags that you want to pass. Each session tag consists of a key name and an associated
* value. For more information about session tags, see Tagging AWS STS Sessions in
* the IAM User Guide.
*
*
* This parameter is optional. You can pass up to 50 session tags. The plain text session tag keys can’t exceed
* 128 characters, and the values can’t exceed 256 characters. For these and additional limits, see IAM and STS Character Limits in the IAM User Guide.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format that
* has a separate limit. Your request can fail for this limit even if your plain text meets the other
* requirements. The PackedPolicySize
response element indicates by percentage how close the
* policies and tags for your request are to the upper size limit.
*
*
*
* You can pass a session tag with the same key as a tag that is already attached to the role. When you do,
* session tags override a role tag with the same key.
*
*
* Tag key–value pairs are not case sensitive, but case is preserved. This means that you cannot have separate
* Department
and department
tag keys. Assume that the role has the
* Department
=Marketing
tag and you pass the department
=
* engineering
session tag. Department
and department
are not saved as
* separate tags, and the session tag passed in the request takes precedence over the role tag.
*
*
* Additionally, if you used temporary credentials to perform this operation, the new session inherits any
* transitive session tags from the calling session. If you pass a session tag with the same key as an inherited
* tag, the operation fails. To view the inherited tags for a session, see the AWS CloudTrail logs. For more
* information, see Viewing
* Session Tags in CloudTrail in the IAM User Guide.
*
*
* @param tags
* A list of session tags that you want to pass. Each session tag consists of a key name and an
* associated value. For more information about session tags, see Tagging AWS STS
* Sessions in the IAM User Guide.
*
* This parameter is optional. You can pass up to 50 session tags. The plain text session tag keys can’t
* exceed 128 characters, and the values can’t exceed 256 characters. For these and additional limits,
* see IAM and STS Character Limits in the IAM User Guide.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format
* that has a separate limit. Your request can fail for this limit even if your plain text meets the
* other requirements. The PackedPolicySize
response element indicates by percentage how
* close the policies and tags for your request are to the upper size limit.
*
*
*
* You can pass a session tag with the same key as a tag that is already attached to the role. When you
* do, session tags override a role tag with the same key.
*
*
* Tag key–value pairs are not case sensitive, but case is preserved. This means that you cannot have
* separate Department
and department
tag keys. Assume that the role has the
* Department
=Marketing
tag and you pass the department
=
* engineering
session tag. Department
and department
are not
* saved as separate tags, and the session tag passed in the request takes precedence over the role tag.
*
*
* Additionally, if you used temporary credentials to perform this operation, the new session inherits
* any transitive session tags from the calling session. If you pass a session tag with the same key as
* an inherited tag, the operation fails. To view the inherited tags for a session, see the AWS
* CloudTrail logs. For more information, see Viewing Session Tags in CloudTrail in the IAM User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* A list of session tags that you want to pass. Each session tag consists of a key name and an associated
* value. For more information about session tags, see Tagging AWS STS Sessions in
* the IAM User Guide.
*
*
* This parameter is optional. You can pass up to 50 session tags. The plain text session tag keys can’t exceed
* 128 characters, and the values can’t exceed 256 characters. For these and additional limits, see IAM and STS Character Limits in the IAM User Guide.
*
*
*
* An AWS conversion compresses the passed session policies and session tags into a packed binary format that
* has a separate limit. Your request can fail for this limit even if your plain text meets the other
* requirements. The PackedPolicySize
response element indicates by percentage how close the
* policies and tags for your request are to the upper size limit.
*
*
*
* You can pass a session tag with the same key as a tag that is already attached to the role. When you do,
* session tags override a role tag with the same key.
*
*
* Tag key–value pairs are not case sensitive, but case is preserved. This means that you cannot have separate
* Department
and department
tag keys. Assume that the role has the
* Department
=Marketing
tag and you pass the department
=
* engineering
session tag. Department
and department
are not saved as
* separate tags, and the session tag passed in the request takes precedence over the role tag.
*
*
* Additionally, if you used temporary credentials to perform this operation, the new session inherits any
* transitive session tags from the calling session. If you pass a session tag with the same key as an inherited
* tag, the operation fails. To view the inherited tags for a session, see the AWS CloudTrail logs. For more
* information, see Viewing
* Session Tags in CloudTrail in the IAM User Guide.
*
* This is a convenience that creates an instance of the {@link List.Builder} avoiding the need to create
* one manually via {@link List#builder()}.
*
* When the {@link Consumer} completes, {@link List.Builder#build()} is called immediately and its result
* is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on {@link List.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(List)
*/
Builder tags(Consumer... tags);
/**
*
* A list of keys for session tags that you want to set as transitive. If you set a tag key as transitive, the
* corresponding key and value passes to subsequent sessions in a role chain. For more information, see Chaining Roles with Session Tags in the IAM User Guide.
*
*
* This parameter is optional. When you set session tags as transitive, the session policy and session tags
* packed binary limit is not affected.
*
*
* If you choose not to specify a transitive tag key, then no tags are passed from this session to any
* subsequent sessions.
*
*
* @param transitiveTagKeys
* A list of keys for session tags that you want to set as transitive. If you set a tag key as
* transitive, the corresponding key and value passes to subsequent sessions in a role chain. For more
* information, see Chaining Roles with Session Tags in the IAM User Guide.
*
* This parameter is optional. When you set session tags as transitive, the session policy and session
* tags packed binary limit is not affected.
*
*
* If you choose not to specify a transitive tag key, then no tags are passed from this session to any
* subsequent sessions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder transitiveTagKeys(Collection transitiveTagKeys);
/**
*
* A list of keys for session tags that you want to set as transitive. If you set a tag key as transitive, the
* corresponding key and value passes to subsequent sessions in a role chain. For more information, see Chaining Roles with Session Tags in the IAM User Guide.
*
*
* This parameter is optional. When you set session tags as transitive, the session policy and session tags
* packed binary limit is not affected.
*
*
* If you choose not to specify a transitive tag key, then no tags are passed from this session to any
* subsequent sessions.
*
*
* @param transitiveTagKeys
* A list of keys for session tags that you want to set as transitive. If you set a tag key as
* transitive, the corresponding key and value passes to subsequent sessions in a role chain. For more
* information, see Chaining Roles with Session Tags in the IAM User Guide.
*
* This parameter is optional. When you set session tags as transitive, the session policy and session
* tags packed binary limit is not affected.
*
*
* If you choose not to specify a transitive tag key, then no tags are passed from this session to any
* subsequent sessions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder transitiveTagKeys(String... transitiveTagKeys);
/**
*
* A unique identifier that might be required when you assume a role in another account. If the administrator of
* the account to which the role belongs provided you with an external ID, then provide that value in the
* ExternalId
parameter. This value can be any string, such as a passphrase or account number. A
* cross-account role is usually set up to trust everyone in an account. Therefore, the administrator of the
* trusting account might send an external ID to the administrator of the trusted account. That way, only
* someone with the ID can assume the role, rather than everyone in the account. For more information about the
* external ID, see How to Use
* an External ID When Granting Access to Your AWS Resources to a Third Party in the IAM User Guide.
*
*
* The regex used to validate this parameter is a string of characters consisting of upper- and lower-case
* alphanumeric characters with no spaces. You can also include underscores or any of the following characters:
* =,.@:/-
*
*
* @param externalId
* A unique identifier that might be required when you assume a role in another account. If the
* administrator of the account to which the role belongs provided you with an external ID, then provide
* that value in the ExternalId
parameter. This value can be any string, such as a
* passphrase or account number. A cross-account role is usually set up to trust everyone in an account.
* Therefore, the administrator of the trusting account might send an external ID to the administrator of
* the trusted account. That way, only someone with the ID can assume the role, rather than everyone in
* the account. For more information about the external ID, see How
* to Use an External ID When Granting Access to Your AWS Resources to a Third Party in the IAM
* User Guide.
*
* The regex used to validate this parameter is a string of characters consisting of upper- and
* lower-case alphanumeric characters with no spaces. You can also include underscores or any of the
* following characters: =,.@:/-
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder externalId(String externalId);
/**
*
* The identification number of the MFA device that is associated with the user who is making the
* AssumeRole
call. Specify this value if the trust policy of the role being assumed includes a
* condition that requires MFA authentication. The value is either the serial number for a hardware device (such
* as GAHT12345678
) or an Amazon Resource Name (ARN) for a virtual device (such as
* arn:aws:iam::123456789012:mfa/user
).
*
*
* The regex used to validate this parameter is a string of characters consisting of upper- and lower-case
* alphanumeric characters with no spaces. You can also include underscores or any of the following characters:
* =,.@-
*
*
* @param serialNumber
* The identification number of the MFA device that is associated with the user who is making the
* AssumeRole
call. Specify this value if the trust policy of the role being assumed
* includes a condition that requires MFA authentication. The value is either the serial number for a
* hardware device (such as GAHT12345678
) or an Amazon Resource Name (ARN) for a virtual
* device (such as arn:aws:iam::123456789012:mfa/user
).
*
* The regex used to validate this parameter is a string of characters consisting of upper- and
* lower-case alphanumeric characters with no spaces. You can also include underscores or any of the
* following characters: =,.@-
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder serialNumber(String serialNumber);
/**
*
* The value provided by the MFA device, if the trust policy of the role being assumed requires MFA (that is, if
* the policy includes a condition that tests for MFA). If the role being assumed requires MFA and if the
* TokenCode
value is missing or expired, the AssumeRole
call returns an
* "access denied" error.
*
*
* The format for this parameter, as described by its regex pattern, is a sequence of six numeric digits.
*
*
* @param tokenCode
* The value provided by the MFA device, if the trust policy of the role being assumed requires MFA (that
* is, if the policy includes a condition that tests for MFA). If the role being assumed requires MFA and
* if the TokenCode
value is missing or expired, the AssumeRole
call returns an
* "access denied" error.
*
* The format for this parameter, as described by its regex pattern, is a sequence of six numeric digits.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tokenCode(String tokenCode);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends StsRequest.BuilderImpl implements Builder {
private String roleArn;
private String roleSessionName;
private List policyArns = DefaultSdkAutoConstructList.getInstance();
private String policy;
private Integer durationSeconds;
private List tags = DefaultSdkAutoConstructList.getInstance();
private List transitiveTagKeys = DefaultSdkAutoConstructList.getInstance();
private String externalId;
private String serialNumber;
private String tokenCode;
private BuilderImpl() {
}
private BuilderImpl(AssumeRoleRequest model) {
super(model);
roleArn(model.roleArn);
roleSessionName(model.roleSessionName);
policyArns(model.policyArns);
policy(model.policy);
durationSeconds(model.durationSeconds);
tags(model.tags);
transitiveTagKeys(model.transitiveTagKeys);
externalId(model.externalId);
serialNumber(model.serialNumber);
tokenCode(model.tokenCode);
}
public final String getRoleArn() {
return roleArn;
}
@Override
public final Builder roleArn(String roleArn) {
this.roleArn = roleArn;
return this;
}
public final void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
public final String getRoleSessionName() {
return roleSessionName;
}
@Override
public final Builder roleSessionName(String roleSessionName) {
this.roleSessionName = roleSessionName;
return this;
}
public final void setRoleSessionName(String roleSessionName) {
this.roleSessionName = roleSessionName;
}
public final Collection getPolicyArns() {
return policyArns != null ? policyArns.stream().map(PolicyDescriptorType::toBuilder).collect(Collectors.toList())
: null;
}
@Override
public final Builder policyArns(Collection policyArns) {
this.policyArns = _policyDescriptorListTypeCopier.copy(policyArns);
return this;
}
@Override
@SafeVarargs
public final Builder policyArns(PolicyDescriptorType... policyArns) {
policyArns(Arrays.asList(policyArns));
return this;
}
@Override
@SafeVarargs
public final Builder policyArns(Consumer... policyArns) {
policyArns(Stream.of(policyArns).map(c -> PolicyDescriptorType.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
public final void setPolicyArns(Collection policyArns) {
this.policyArns = _policyDescriptorListTypeCopier.copyFromBuilder(policyArns);
}
public final String getPolicy() {
return policy;
}
@Override
public final Builder policy(String policy) {
this.policy = policy;
return this;
}
public final void setPolicy(String policy) {
this.policy = policy;
}
public final Integer getDurationSeconds() {
return durationSeconds;
}
@Override
public final Builder durationSeconds(Integer durationSeconds) {
this.durationSeconds = durationSeconds;
return this;
}
public final void setDurationSeconds(Integer durationSeconds) {
this.durationSeconds = durationSeconds;
}
public final Collection getTags() {
return tags != null ? tags.stream().map(Tag::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder tags(Collection tags) {
this.tags = _tagListTypeCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setTags(Collection tags) {
this.tags = _tagListTypeCopier.copyFromBuilder(tags);
}
public final Collection getTransitiveTagKeys() {
return transitiveTagKeys;
}
@Override
public final Builder transitiveTagKeys(Collection transitiveTagKeys) {
this.transitiveTagKeys = _tagKeyListTypeCopier.copy(transitiveTagKeys);
return this;
}
@Override
@SafeVarargs
public final Builder transitiveTagKeys(String... transitiveTagKeys) {
transitiveTagKeys(Arrays.asList(transitiveTagKeys));
return this;
}
public final void setTransitiveTagKeys(Collection transitiveTagKeys) {
this.transitiveTagKeys = _tagKeyListTypeCopier.copy(transitiveTagKeys);
}
public final String getExternalId() {
return externalId;
}
@Override
public final Builder externalId(String externalId) {
this.externalId = externalId;
return this;
}
public final void setExternalId(String externalId) {
this.externalId = externalId;
}
public final String getSerialNumber() {
return serialNumber;
}
@Override
public final Builder serialNumber(String serialNumber) {
this.serialNumber = serialNumber;
return this;
}
public final void setSerialNumber(String serialNumber) {
this.serialNumber = serialNumber;
}
public final String getTokenCode() {
return tokenCode;
}
@Override
public final Builder tokenCode(String tokenCode) {
this.tokenCode = tokenCode;
return this;
}
public final void setTokenCode(String tokenCode) {
this.tokenCode = tokenCode;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public AssumeRoleRequest build() {
return new AssumeRoleRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}